When outputting knowledge from PowerShell you typically must concatenate two or extra strings or variables. Becoming a member of strings in PowerShell is primarily carried out utilizing the +
operator. However there are different methods to concatenate strings as effectively.
The issue with becoming a member of strings or variables in PowerShell is commonly discovering the proper output technique. You may get want additional house between the 2 strings, otherwise you get the + signal within the output, which you don’t need after all.
On this article, we’ll have a look at the totally different strategies to hitch a number of strings in PowerShell.
Powershell Concatenate String
The essential technique to concatenate a string in PowerShell is to make use of the +
operator. When utilizing the +
operator, the 2 strings will merely be joined collectively. Let’s take the next two strings to start out with:
$string1 = "The short brown fox" $string2 = "jumps over the lazy canine"
Now if we need to output these strings collectively within the PowerShell console, folks typically are likely to do the next:
Write-Host $string1 + $string2 # Consequence The short brown fox + jumps over the lazy canine
However should you have a look at the outcome, you will notice that the + signal can be within the output, which we don’t need. The right option to output each strings to the console is by merely putting them after one another.
Write-Host $string1 $string2 # Consequence The short brown fox jumps over the lazy canine
However this isn’t concatenating strings. To truly be part of the strings collectively we are able to retailer the outcome first in one other variable utilizing the +
operator:
$string1 = "The short brown fox" $string2 = "jumps over the lazy canine" $outcome = $string1 + $string2 Write-Host $outcome # Consequence The short brown foxjumps over the lazy canine
The one concern right here is that you simply miss the house between the 2 strings. So to resolve that you can concatenate additionally an area between the 2 strings:
$outcome = $string1 + " " + $string2
Utilizing the Be part of Operator
One other technique to hitch strings in PowerShell is to make use of the Be part of Operator. The be part of
operators permit you to concatenate two or extra strings with a specified separator. Taking our two instance strings, we are able to do the next:
$outcome = $string1,$string2 -join " " # Consequence The short brown fox jumps over the lazy canine
An alternative choice is to make use of the .Internet be part of technique. The principal and outcomes are the identical because the be part of operator, solely the writing type is a bit totally different:
[string]::Be part of(' ',$string1,$string2)
Utilizing the Format Operator
When you should insert a number of variables right into a string, then utilizing the string format operator is likely to be a very good choice to make use of. The benefit of the format operator -f
is that it’s simpler to learn and format with longer strings or a number of variables.
The format operator makes use of placeholders to find out the place you need to insert a string or variable. The placeholders are numbered upwards from 0 and positioned between curly brackets.
Take the instance beneath, we’ve a PSCusstomObject
with a few strings. We are able to concatenate these strings in PowerShell into one other string utilizing the string format operator.
$obj = [PSCustomObject]@{ Identify="Lazy Canine" Motion = 'leap over' Merchandise = "3 fences" } write-host ("can the {0} actually {1} greater than {2}" -f $obj.identify, $obj.motion, $obj.merchandise) # Consequence can the Lazy Canine actually leap over greater than 3 fences
You don’t want to make use of variables for the correct aspect of the -f
operator. You may also insert strings or integers straight:
$obj = [PSCustomObject]@{ Identify="Lazy Canine" Motion = 'leap over' Merchandise = "fences" } write-host ("can the {0} actually {1} {2} {3} {4}" -f $obj.identify, $obj.motion, 'lower than', 5, $obj.merchandise) # Consequence can the Lazy Canine actually leap over lower than 5 fences
In case you have an array with strings that you simply need to concatenate in PowerShell, then you can too use the format operator, with out the necessity of specifying every particular person worth for the placeholder.
$values = @( "Lazy Canine" "Jumps" "fences" ) 'Do you know that the {0} {1} 5 {2}?' -f $values # Consequence Do you know that the Lazy Canine Jumps 5 fences?
Concatenate String with Variable
Concatenating strings with variables, and particularly objects or hashtables, is all the time a bit difficult. In case you have a single variable that you simply wish to insert right into a string, then you’ll be able to merely place the string between double quotes “, and insert the variable straight:
$var="fox" Write-Host "The short brown $var jumps over the lazy canine" # Consequence The short brown fox jumps over the lazy canine
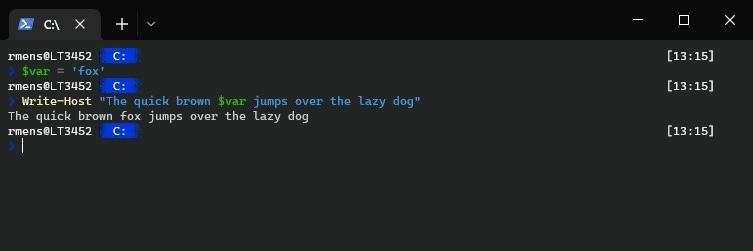
However if you do that with the properties of an object, then it gained’t work. To unravel this you will have to make use of variable substitution, the place you place the variable inside $()
.
$obj = [PSCustomObject]@{ Identify="Lazy Canine" Motion = 'leap over' Merchandise = "fences" } Write-Host "The short brown fox jumps over the $($obj.identify)" # Consequence The short brown fox jumps over the Lazy Canine
Utilizing the Concat Methodology
In PowerShell, we are able to additionally use the .Internet concat technique to concatenate strings. The benefit of the Be part of operator or technique is that you simply don’t must specify a separator, however that additionally makes the use case a bit restricted.
$values = @( "Lazy Canine" "Jumps" "fences" ) [string]::Concat('Lazy Canine','Jumps','fences') # Or when you have got an array with values: [string]::Concat($values) # Consequence for each strategies: Lazy DogJumpsfences
Wrapping Up
There are quite a lot of methods in PowerShell to concatenate a string, much more than I’ve described right here. Between these strategies, there may be little distinction in terms of efficiency to be used instances. So the tactic to make use of actually is dependent upon the scenario and writing type.
While you solely must concatenate a few strings, then utilizing the + operator is commonly the popular method. When you should be part of a number of strings or values, then the format operator (placeholder technique) is commonly simpler to learn.
Additionally remember that if you need to output object properties, you will have to wrap them into parentheses $()
and use double-quotes to wrap the string.
I hope this text helped you with becoming a member of strings in PowerShell. In case you have any questions, simply drop a remark beneath.