Some of the frequent duties when writing a script is to examine if a variable is NULL or Empty in PowerShell. Null or empty values are at all times a bit difficult, as a result of what is precisely null?
Checking if a worth is null or not has develop into quite a bit simpler in PowerShell 7 with the Null-coalescing operators. This operator eliminates the necessity for if-else statements to examine if a worth is Null or not.
On this article, I’ll clarify examine if a worth is null, not null, or simply empty. We have a look at the brand new Null-coalescing operator and why it’s essential to start out with the $null
variable within the comparability.
What’s Null
Once we are speaking about NULL in a programming or scripting language, then we will assume it’s a variable that doesn’t have any worth. In PowerShell nonetheless, Null can both be an empty worth or an unknown worth.
The NULL worth is written as $null
in PowerShell, and it’s one of many computerized variables. It’s an object with the worth NULL. This lets you use $null
as a placeholder in collections or assign it to a variable.
A great way to reveal that is to create a group and depend the size of it. As you’ll be able to see within the instance under, the $null
object is counted as one of many objects within the assortment:
$fruits = "Apple", $null, "Pear" $fruits.depend 3
NULL vs Empty
Apart from $null
values, we will even have empty values in PowerShell. The distinction between the 2 is that an empty worth is a variable that’s declared and assigned an precise empty worth.
If we have a look at the instance under, then the primary variable is $null
, regardless that we haven’t assigned the NULL variable to it. The opposite variables usually are not null however are empty.
# Null variable $variableIsNull # Empty string variable $emptyString = "" # Empty array variable $emptyArray = @()
Examine if Null in PowerShell
There are a few methods to examine if a variable or result’s Null in PowerShell. The beneficial option to examine if a worth is NULL is by evaluating $null
with the worth:
if ($null -eq $worth) { Write-Host 'Variable is null'; }
Vital to notice that the tactic above, returns to true on two events. When the worth is NULL, as you’ll count on, but additionally when the variable is just not outlined.
Once you wish to examine if a worth is Not Null, then you should utilize the comparability operator -ne
as an alternative:
if ($null -ne $worth) { Write-Host 'Variable is just not null'; }
One other frequent option to examine if a worth is Null is to easily use an if assertion with none comparisons. This additionally works, however it does greater than checking if a worth is null. The comparability under will truly do the next:
- not Null
- not empty
- not 0
- not an array,
- Size is just not equal to 0
- not $false
if ($worth) { Write-Host 'Variable is just not null or empty'; }
Null on the left-hand facet
If you find yourself utilizing Visible Studio code or one other IDE to jot down your PowerShell scripts, you’re going to get a warning once you place the $null
variable on the right-hand facet in a comparability. That is for a superb cause.
In some circumstances, inserting them $null
on the right-hand facet may end up in sudden outcomes. Take for instance the array under. If we place $null
on the left facet, it’ll examine if $null
is just not equal to our $array
, which it isn’t, so it returns true.
# Array $a = 1, 2, $null, 4, $null, 6 # Examine if the Array is just not empty (null) $null -ne $a # returns true # Return all the things from the array that isn't Null $a -ne $null # Returns 1 2 4 6
However once you place $null
it on the right-hand facet, then it’ll return all the things from the array, that doesn’t equal $null.
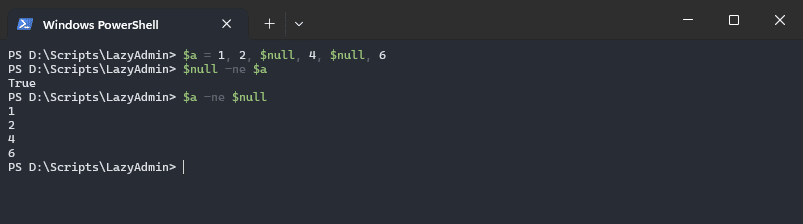
Null-coalescing Operator
If you’re utilizing PowerShell 7, and also you truly ought to, then you should utilize one of many new null conditional operators to rapidly examine if a variable or property is null or not. This manner you don’t want to jot down If statements each time you solely wish to examine if a variable is null or not.
The Null coalescing operator ??
returns the worth on the left facet if it’s not null. If the worth on the left is null, then it returns the outcomes on the correct facet.
$nullableVariable = $null # If $nullableVariable equal NULL, return "Default Worth" $defaultValue = $nullableVariable ?? "Default Worth" # Results of $defaultValue The worth is: Default Worth
We are able to additionally use this methodology to examine the results of a operate and name one other operate or cmdlet if the outcomes are null:
operate Get-LastUsedDate { return $null } # Use null-coalescing operator to deal with null return worth $outcome = (Get-LastUsedDate) ?? (Get-Date).ToShortDateString()
It’s additionally attainable to chain a number of Null Coalescing operators collectively to search out or return the primary variable that isn’t null.
# Outline a sequence of variables the place one could also be null $firstVariable = $null $secondVariable = "Worth 2" # Use null-coalescing operator to decide on the primary non-null worth $chosenValue = $firstVariable ?? $secondVariable ?? "No worth discovered" # Output the chosen worth Write-Host "Chosen worth: $chosenValue"
Null-coalescing Task Operator
The Null-coalescing task operator ??=
makes it simpler to examine if a variable on the left-hand facet of the operator is null or not, and assign the worth of the right-hand facet to it if it’s null.
Within the instance under, we examine if the variable $existingValue is null or not. If it’s null, then we set the variable to the default worth:
# Variable that will or could not have a worth $existingValue = "Present Worth" # Assign a brand new worth utilizing the Null-coalescing task operator $existingValue ??= "Default Worth" # Results of $existingValue Present Worth
Null-conditional operators ?.
and ?[]
New in PowerShell 7.1 are the Null-conditional operators ?.
and ?[]
. These operators can help you safely entry properties and parts of an object that is perhaps null.
If you happen to entry a property or ingredient with out the null-conditional operator, you usually gained’t get an error. Solely if you’re utilizing Strict mode you do get an error. So in these circumstances, it’s essential to examine if you’re not attempting to entry a member of a null-valued object.
# Empty object $cities = $null # Set strictmode for check Set-StrictMode -Model 2 # Will throw an error $cities.language # No error with null-conditional operator ${cities}?.language
Examine if Empty in PowerShell
Apart from checking if a worth is Null one other frequent apply is to examine if a worth is empty. You possibly can solely examine if a string or array is empty in PowerShell.
To examine if a string is empty, merely examine it with ""
$string = "" if ($string -eq "") { write-host "Empty string discovered" }
If you wish to examine if an array is empty, the best choice is to examine the size of the array:
$array = @() if ($array.Rely -gt 0) { write-host "Array is just not empty" }
IsNullOrEmpty
When working with strings, you may as well use the static string operate to examine if the worth is both null or empty. If you wish to know if the worth is just not null or empty, you then solely want to put -not
in entrance of it
$string = $null if ([String]::IsNullOrEmpty($string)) { write-host "Empty string" } # Examine if not empty or null if (-not [String]::IsNullOrEmpty($string)) { write-host "Striong is Not empty" }
Examine for Null in a Perform
If you find yourself creating your personal capabilities in PowerShell, you’ll be able to make sure that a parameter is just not null or empty by utilizing the validator ValidateNotNullOrEmpty
. This manner you don’t should do further checks within the operate.
operate Take a look at-Enter { param( [Parameter(Mandatory)] [ValidateNotNullOrEmpty()] [string]$enter ) Write-Host "Enter acquired: $enter" } # Take a look at the operate Take a look at-Enter -input "Hi there"
Wrapping Up
Checking for Null values makes your script extra sturdy and can end in fewer errors in your script. Just be sure you check out the brand new PowerShell null-coalescing operators as a result of they’re fairly helpful to work with.
Additionally, just remember to at all times preserve the $null
values on the left-hand facet of the comparability.
I hope you appreciated this text, in case you have any questions, simply drop a remark under!