Downside Formulation and Answer Overview
Sooner or later, you could want to put in writing the contents of a flat-text file from one location to a different. This may be completed in varied methods, as proven beneath in our examples.
For this text, a flat-text file about Albert Einstein is saved to the present working folder. This file known as albert.txt
. Contents of identical beneath.
Albert Einstein (4 March 1879 – 18 April 1955) was a German-born theoretical physicist extensively often called one of many biggest and most influential physicists of all time. Einstein is finest recognized for growing the idea of relativity, however he additionally contributed considerably to growing the idea of quantum mechanics. Relativity and quantum mechanics are collectively the 2 pillars of recent physics. His mass-energy equivalence formulation E = mc2, which arises from relativity principle, known as “the world’s most well-known equation.”
Technique 1: Use Absolute Path
This instance makes use of an absolute path to save lots of a flat-text file positioned within the present working folder to a distinct folder.
with open('albert.txt', 'r') as fp1, open('c:mr_smithessaysalbert.txt', 'w') as fp2: outcomes = fp1.learn() fp2.write(outcomes)
This code begins through the use of a with open()
assertion to open two (2) flat-text recordsdata. One file is opened in learn mode (r
) and the opposite in write mode (w
). These two (2) recordsdata save as file objects to fp1
and fp2
, respectively.
If output to the terminal, the contents of fp1
and fp2
might be much like beneath.
<_io.TextIOWrapper identify="einstein.txt" mode="r" encoding='cp1252'>
|
Subsequent, the file object fp1
reads within the contents of the primary file and saves the output to outcomes
. This knowledge is then written to the file object fp2
and positioned within the folder indicated above.
What occurs if the referenced folder or file doesn’t exist?
Add a strive
/besides
assertion to the code to catch and show any exception errors.
strive: with open('albert.txt', 'r') as fp1, open('c:mr_smithessaysalbert.txt', 'w') as fp2: outcomes = fp1.learn() fp2.write(outcomes) besides Exception as e: print('Error: ' + str(e))
If profitable, the above file ought to reside within the file path proven above.
Technique 2: Use os.path
This instance makes use of os.path
to save lots of a flat-text file positioned within the present working folder to a distinct folder.
import os.path folder="c:mr_smithessays" file_name="albert.txt" file_path = os.path.be part of(folder, file_name) if not os.path.isdir(folder): os.mkdir(folder) with open(file_name, 'r') as fp1, open(file_path, 'w') as fp2: outcomes = fp1.learn() fp2.write(outcomes)
The primary line within the above code imports Python’s built-in os library. This library permits customers to work together with and manipulate recordsdata and folders.
The next two (2) strains declare the folder location and the filename. These save to the variables folder
and file_name
, respectively.
Subsequent, os.path.be part of()
known as and handed to the variables folder
and file_name
. This operate concatenates the file path and the filename and saves it to file_path
.
Then, the if
assertion checks for the existence of the desired folder. If this folder does not exist, one is created calling os.mkdir()
and passing the folder to create as an argument.
The next part opens the 2 (2) recordsdata as indicated above in Technique 1 and writes the contents of file 1 (fp1
) to file 2 (fp2
).
If profitable, the above file ought to reside within the file path indicated above.
💡Be aware: Click on right here to learn extra about opening a number of recordsdata concurrently.
Technique 3: Use shutil.copy()
This instance makes use of shutil
.copy() from the shutil
library to repeat a flat-text file positioned within the present working folder to a distinct folder.
import shutil current_loc="albert.txt" new_loc="c:mr_smithessays" + current_loc shutil.copy(current_loc, new_loc)
The above code imports the shutil
library, which affords a number of capabilities. One, specifically, permits us to repeat a file from one location to a different.
This code first declares the variable current_loc
, which accommodates the direct path to the file to be copied (present working folder, plus the file identify).
Subsequent, a variable new_loc
is said and configured to repeat the present flat-text file to a distinct folder.
The ultimate line copies the present file from the present working folder to the brand new location indicated above.
Technique 4: Use Path
This instance makes use of Path
from the pathlib
library to save lots of a flat-text file positioned within the present working folder to a distinct folder.
from pathlib import Path with open('albert.txt', 'r') as fp1: outcomes = fp1.learn() p = Path('c:mr_smithessays') p.mkdir(exist_ok=True) with (p / 'albert.txt').open('w') as fp2: fp2.write(outcomes)
The above code imports Path
from the pathlib
library.
The next two (2) strains open and browse (r
) within the flat-text file positioned within the present working folder. The contents of this file are saved to outcomes
.
Subsequent, the trail (or folder identify) is said the place the contents of outcomes might be written to. If this folder doesn’t exist, it’s created.
Lastly, the above flat-text file is opened in write (w) mode and the contents of outcomes is written to this file.
Abstract
This text has supplied 4 (4) methods to save lots of a flat-text file to a different folder to pick one of the best match in your coding necessities.
Good Luck & Completely happy Coding!
Programming Humor
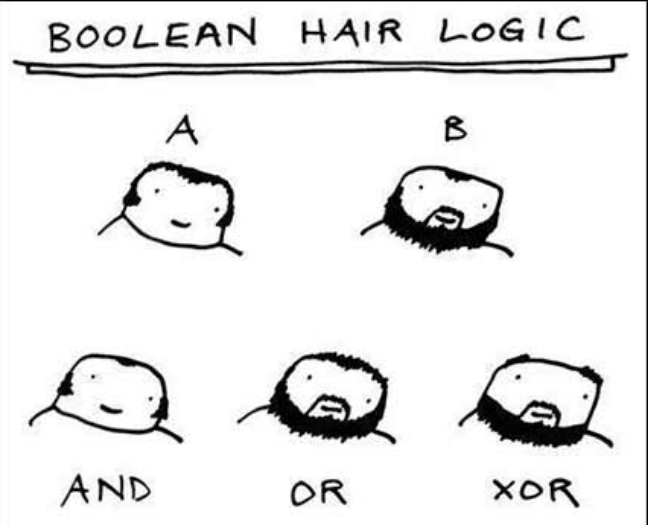

At college, I discovered my love of writing and coding. Each of which I used to be ready to make use of in my profession.
In the course of the previous 15 years, I’ve held numerous positions akin to:
In-house Company Technical Author for varied software program applications akin to Navision and Microsoft CRM
Company Coach (workers of 30+)
Programming Teacher
Implementation Specialist for Navision and Microsoft CRM
Senior PHP Coder