Sequence Index
Python and Go: Half I – gRPC
Python and Go: Half II – Extending Python With Go
Python and Go: Half III – Packaging Python Code
Python and Go: Half IV – Utilizing Python in Reminiscence
Introduction
In a earlier put up we used gRPC to name Python code from Go. gRPC is a superb framework, however there’s a efficiency price to it. Each operate name must marshal the arguments utilizing protobuf, make a community name over HTTP/2, after which un-marshal the consequence utilizing protobuf
.
On this weblog put up, we’ll do away with the networking layer and to some extent, the marshalling. We’ll do that by utilizing cgo to work together with Python as a shared library.
I’m not going to cowl the entire code intimately as a way to preserve this weblog dimension down. You could find all of the code on github and I did my finest to supply correct documentation. Be at liberty to succeed in out and ask me questions in case you don’t perceive one thing.
And at last, if you wish to observe alongside, you’ll want to put in the next (other than Go):
- Python 3.8
- numpy
- A C compiler (equivalent to gcc)
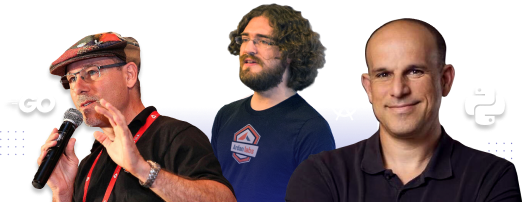
A Crash Course in Python Internals
The model of Python most of us use is known as CPython
. It’s written in C and is designed to be prolonged and embedded utilizing C. On this part, we’ll cowl some matters that may aid you perceive the code I’m going to indicate.
Notice: The Python C API is properly documented, and there’s even a e book within the works.
In CPython, each worth is a PyObject *
and most of Python’s API features will return a PyObject *
or will obtain a PyObject *
as an argument. Additionally, errors are signaled by returning NULL
, and you should utilize the PyErr_Occurred
operate to get the final exception raised.
CPython makes use of a reference counting rubbish collector which signifies that each PyObject *
has a counter for what number of variables are referencing it. As soon as the reference counter reaches 0, Python frees the item’s reminiscence. As a programmer, it’s good to take care to decrement the reference counter utilizing the Py_DECREF
C macro when you’re accomplished with an object.
From Go to Python and Again Once more
Our code tries to reduce reminiscence allocations and keep away from pointless serialization. In an effort to do that, we’ll share reminiscence between Go and Python as a substitute of allocating new reminiscence and copying that information on both sides of the operate name and return. Sharing reminiscence between two runtimes is hard and it’s good to pay quite a lot of consideration to who owns what piece of reminiscence and when every runtime is allowed to launch it.
Determine 1
Determine 1 exhibits the move of information from the Go operate to the Python operate.
Our enter is a Go slice ([]float64
) which has an underlying array in reminiscence managed by Go. We’ll go a pointer to the slice’s underlying array to C, which in flip will create a numpy array that may use the identical underlying array in reminiscence. It’s this numpy array that will likely be handed as enter to the Python outliers detection operate referred to as detect
.
Determine 2
Determine 2 exhibits the move of information from the Python operate again to the Go operate.
When the Python detect
operate completes, it returns a brand new numpy array whose underlying reminiscence is allotted and managed by Python. Like we did between Go and Python, we’ll share the reminiscence again to Go by passing the Python pointer to the underlying numpy array (by way of C).
In an effort to simplify reminiscence administration, on the Go aspect as soon as we’ve entry to the numpy array pointer, we create a brand new Go slice ([]int
) and replica the content material of the numpy array inside.. Then we inform Python it could possibly free the reminiscence it allotted for the numpy array.
After the decision to detect
completes, the one reminiscence we’re left with is the enter ([]float64
) and the output ([]int
) slices each being managed by Go. Any Python reminiscence allocations must be launched.
Code Overview
Our Go code goes to load and initialize a Python shared library so it could possibly name the detect
operate that makes use of numpy to carry out outlier detection on a sequence of floating level values.
These are the steps that we’ll observe:
- Convert the Go slice
[]float64
parameter to a Cdouble *
(outliers.go
) - Create a numpy array from the C
double *
(glue.c
) - Name the Python operate with the numpy array (
glue.c
) - Get again a numpy array with indices of outliers (
glue.c
) - Extract C
lengthy *
from the numpy array (glue.c
) - Convert the C
lengthy *
to a Go slice[]int
and return it from the Go operate
(outliers.go
)
The Go code is in outliers.go
, there’s some C code in glue.c
, and eventually the outlier detection Python operate is in outliers.py
. I’m not going to indicate the C code, however in case you’re interested by it take a look at glue.c.
Itemizing 1: Instance Utilization
15 o, err := NewOutliers("outliers", "detect")
16 if err != nil {
17 return err
18 }
19 defer o.Shut()
20 indices, err := o.Detect(information)
21 if err != nil {
22 return err
23 }
24 fmt.Printf("outliers at: %vn", indices)
25 return nil
Itemizing 1 exhibits an instance of use what we’re going to construct in Go. On line 15, we create an Outliers
object which makes use of the operate detect
from the outliers
Python module. On line 19, we be certain that to free the Python object. On line 20, we name the Detect
technique and get the indices of the outliers within the information.
Code Highlights
Itemizing 2: outliers.go [code]
19 var (
20 initOnce sync.As soon as
21 initErr error
22 )
23
24 // initialize Python & numpy, idempotent
25 func initialize() {
26 initOnce.Do(func() {
27 C.init_python()
28 initErr = pyLastError()
29 })
30 }
Itemizing 2 exhibits how we initialize Python to be used in our Go program. On line 20, we declare a variable of sort sync.As soon as that will likely be used to verify we initialize Python solely as soon as. On line 25, we create a operate to initialize Python. On line 26, we name the Do
technique to name the initialization code and on line 28, we set the initErr
variable to the final Python error.
Itemizing 3: outliers.go [code]
32 // Outliers does outlier detection
33 sort Outliers struct {
34 fn *C.PyObject // Outlier detection Python operate object
35 }
Itemizing 3 exhibits the definition of the Outliers
struct. It has one subject on line 34 which is a pointer to the Python operate object that does the precise outlier detection.
Itemizing 4: outliers.go [code]
37 // NewOutliers returns an new Outliers utilizing moduleName.funcName Python operate
38 func NewOutliers(moduleName, funcName string) (*Outliers, error) {
39 initialize()
40 if initErr != nil {
41 return nil, initErr
42 }
43
44 fn, err := loadPyFunc(moduleName, funcName)
45 if err != nil {
46 return nil, err
47 }
48
49 return &Outliers{fn}, nil
50 }
Itemizing 4 exhibits the NewOutliers
operate that created an Outliers
struct. On strains 39-42, we be certain that Python is initialized and there’s no error. On line 44, we get a pointer to the Python detect
operate. This is similar as doing an import
assertion in Python. On line 49, we save this Python pointer for later use within the Outliers
struct.
Itemizing 5: outliers.go [code]
52 // Detect returns slice of outliers indices
53 func (o *Outliers) Detect(information []float64) ([]int, error) {
54 if o.fn == nil {
55 return nil, fmt.Errorf("closed")
56 }
57
58 if len(information) == 0 { // Quick path
59 return nil, nil
60 }
61
62 // Convert []float64 to C double*
63 carr := (*C.double)(&(information[0]))
64 res := C.detect(o.fn, carr, (C.lengthy)(len(information)))
65
66 // Inform Go's GC to maintain information alive till right here
67 runtime.KeepAlive(information)
68 if res.err != 0 {
69 return nil, pyLastError()
70 }
71
72 indices, err := cArrToSlice(res.indices, res.dimension)
73 if err != nil {
74 return nil, err
75 }
76
77 // Free Python array object
78 C.py_decref(res.obj)
79 return indices, nil
80 }
Itemizing 5 exhibits the code for the Outliers.Detect
technique. On line 63, we convert Go’s []float64
slice to a C double *
by taking the tackle of the primary factor within the underlying slice worth. On line 64, we name the Python detect
operate by way of CGO and we get again a consequence. On line 67, we inform Go’s rubbish collector that it could possibly’t reclaim the reminiscence for information
earlier than this level in this system. On strains 68-70, we examine if there was an error calling detect
. On strains 72, we convert the C double *
to a Go []int
slice. On line 79, we decrement the Python return worth reference depend.
Itemizing 6: outliers.go [code]
82 // Shut frees the underlying Python operate
83 // You'll be able to't use the item after closing it
84 func (o *Outliers) Shut() {
85 if o.fn == nil {
86 return
87 }
88 C.py_decref(o.fn)
89 o.fn = nil
90 }
Itemizing 6 exhibits the Outliers.Shut
technique. On line 88, we decrement the Python operate object reference depend and on line 89, we set the fn
subject to nil
to sign the Outliers
object is closed.
Constructing
The glue code is utilizing header information from Python and numpy. In an effort to construct, we have to inform cgo the place to search out these header information.
Itemizing 7: outliers.go [code]
11 /*
12 #cgo pkg-config: python3
13 #cgo LDFLAGS: -lpython3.8
14
15 #embrace "glue.h"
16 */
17 import "C"
Itemizing 7 exhibits the cgo
directives.
On line 12, we use pkg-config to search out C compiler directives for Python. On line 13, we inform cgo
to make use of the Python 3.8 shared library. On line 15, we import the C code definitions from glue.h
and on line 17, we’ve the import "C"
directive that should come proper after the remark for utilizing cgo
.
Telling cgo the place to search out the numpy headers is hard since numpy doesn’t include a pkg-config
file, however has a Python operate that may inform you the place the headers are. For safety causes, cgo
received’t run arbitrary instructions. I opted to ask the consumer to set the CGO_CFLAGS
setting variable earlier than constructing or putting in the package deal.
Itemizing 8: Construct instructions
01 $ export CGO_CFLAGS="-I $(python -c 'import numpy; print(numpy.get_include())'"
02 $ go construct
Itemizing 8 exhibits construct the package deal. On line 01, we set CGO_CFLAGS
to a worth printed from a brief Python program that prints the placement of the numpy header information. On line 02, we construct the package deal.
I like to make use of make to automate such duties. Take a look on the Makefile to be taught extra.
Conclusion
I’d like to begin by thanking the superior folks on the (aptly named) #darkarts
channel in Gophers Slack for his or her assist and insights.
The code we wrote right here is hard and error susceptible so it is best to have some tight efficiency objectives earlier than happening this path. Benchmarking on my machine exhibits this code is about 45 instances quicker than the equal gRPC code code, the operate name overhead (with out the outliers calculation time) is about 237 instances quicker. Despite the fact that I’m programming in Go for 10 years and in Python near 25 – I discovered some new issues.