Drawback Formulation and Resolution Overview
To make it extra fascinating, we’ve the next working state of affairs:
Technique 1: Use Slicing
This instance makes use of slicing together with the addition operator +
to replace an present tuple aspect and insert a further aspect.
nums_5day = (11, 12, 13, 14, 15) nums_6day = nums_5day[:2] + (18, 21) + nums_5day[3:] print(nums_6day)
The above code declares a Tuple containing projected temperatures for the subsequent 5 (5) days. The outcomes save to nums_5day
.
The next line updates this Tuple by changing a component and inserting a further aspect utilizing slicing. Thus making it projected temperatures for the subsequent six (6
) days. The outcomes save to nums_6day
and are output to the terminal.
Technique 2: Use Unpack and Slicing
This instance makes use of the unpack operator (*) and slicing to switch one (1) worth from an present tuple.
nums_5day = (11, 12, 13, 14, 15) new_5day = (30, *nums_5day[1:]) print(new_5day)
The above code declares a Tuple containing projected temperatures for the subsequent 5 (5) days. The outcomes save to nums_5day
.
The next line replaces the present temperature of 11 to 30. Then, the unpack (*
) operator and slicing are used to maintain the remaining parts. The outcomes save to new_5day
and are output to the terminal.
Technique 3: Use Record and Record Comprehension
This instance makes use of listing and listing comprehension to switch one (1) tuple worth.
nums_5day = listing((11, 12, 13, 14, 15)) new_5day = tuple([x + 5 if x == 13 else x for x in nums_5day]) print(new_5day)
The above code declares a Tuple containing projected temperatures for the subsequent 5 (5) days. That is transformed to a listing and saved to nums_5day
.
The next line makes use of listing comprehension to switch the listing aspect with the worth of 13 to 18 (x+5
). The outcomes are transformed to a Tuple, saved to new_5day
and output to the terminal.
Technique 4: Use map() and a lambda
This instance makes use of the map()
perform and a lambda
to switch three (3) tuple values.
nums_5day = listing((11, 12, 13, 14, 15)) new_5day = tuple(map(lambda x: x+3 if x >= 12 and x <= 14 else x, nums_5day)) print(new_5day)
The above code declares a Tuple containing projected temperatures for the subsequent 5 (5) days. That is transformed to a listing and saved to nums_5day
.
The next line calls the map()
perform and passes it one (1) argument, a lambda
. This consequence returns a map object. If this object was output to the terminal, an object reference much like beneath would show.
<map object at 0x0000021A7F45B190> |
This object is then transformed from the map object to a Tuple and output to the terminal.
Abstract
This text has supplied 4 (4) methods to alter Tuple values to pick the most effective match to your coding necessities.
Good Luck & Comfortable Coding!
Programming Humor – Python
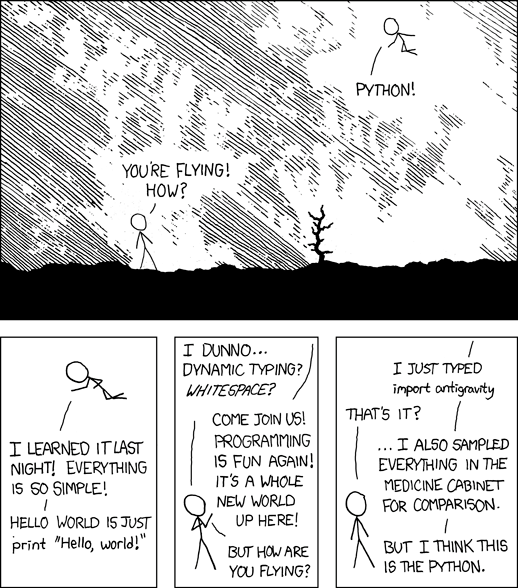

At college, I discovered my love of writing and coding. Each of which I used to be in a position to make use of in my profession.
Through the previous 15 years, I’ve held a variety of positions akin to:
In-house Company Technical Author for varied software program packages akin to Navision and Microsoft CRM
Company Coach (workers of 30+)
Programming Teacher
Implementation Specialist for Navision and Microsoft CRM
Senior PHP Coder