The problem for this month’s Train is to calculate the usual deviation for a given set of information factors. It’s a “complete inhabitants calculation” as a result of all the information factors are current. The trick is to observe the equation, reworking it from cryptic math mumbo-jumbo into C code.
Determine 1 exhibits the equation I used, which follows these steps:
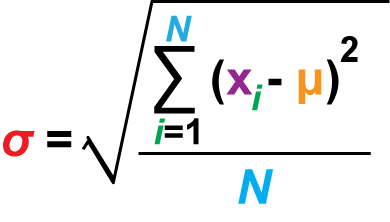
Determine 1. The mathematical monster used to calculate customary deviation (full set).
- Calculate the imply for the values, what I might usually name the “common.” Tally up all the information factors and divide by the variety of information factors.
- Subtract the imply from every component within the information set and sq. the outcome. These values are totaled to acquire their imply. The result’s the variance.
- Get hold of the sq. root of the variance to get the usual deviation.
The pattern code skeleton supplied within the Train submit listed the values[]
array as the information set. Variable gadgets
is about to the variety of gadgets within the information set: gadgets = sizeof(values)/sizeof(int);
These two variables are handed to the stddev() perform the place they’re manipulated to calculate and return the usual deviation.
Right here’s my answer, which follows the steps outlined above:
2024_05-Train.c
#embody <stdio.h> #embody <math.h> double stddev(int v[],int gadgets) { int x,complete; double imply,variance; complete = 0; for( x=0; x<gadgets; x++ ) complete += v[x]; imply = (double)complete/gadgets; complete = 0; for( x=0; x<gadgets; x++ ) complete += (v[x]-mean)*(v[x]-mean); variance = (double)complete/gadgets; return( sqrt(variance) ); } int essential() { int values[] = { 10, 12, 23, 23, 16, 23, 21, 16 }; int x,gadgets; gadgets = sizeof(values)/sizeof(int); printf("Values:"); for( x=0; x<gadgets; x++ ) { printf(" %2nd",values[x]); } putchar('n'); printf("The usual deviation is %.4fn", stddev(values,gadgets) ); return 0; }
The primary for loop within the stddev() perform obtains the overall of all values within the information set, v[]
. The imply is calculated: imply = (double)complete/gadgets;
The double forged is required as imply
is a double variable.
The following for loop calculates the deviation: Variable complete
tallies the squares of the distinction between every worth v[x]
and the imply: complete += (v[x]-mean)*(v[x]-mean);
The multiplication expression squares the values, which is my most popular methodology over utilizing the pow() perform.
Variable variance
is assigned the worth of the overall of the squares divided by the variety of gadgets.
Lastly, the return assertion returns the sq. root of variable variance
, which is the entire inhabitants customary deviation worth. Bear in mind to hyperlink within the math library to help with the sqrt() perform.
Right here’s a pattern run:
Values: 10 12 23 23 16 23 21 16
The usual deviation is 4.8990
I checked my answer’s outcome with the usual deviation calculated by ChatGPT on the identical information set and obtained the identical worth. So I feel I’m good. I hope you’re answer additionally met met with success and that you just didn’t use ChatGPT to write down it for you.