TL;DR: Discover international Gross Nationwide Revenue (GNI) per capita and earnings thresholds by a line chart created with Syncfusion’s .NET MAUI Cartesian Chart management. Uncover the way to collect and hyperlink knowledge to the chart whereas enhancing visualization by customizing parts resembling title, axis, and tooltip.
Welcome to our Chart of the Week weblog sequence!
At the moment, we’ll use Syncfusion’s .NET MAUI Cartesian Chart management to create a line chart with plot bands. This chart will assist us discover international Gross Nationwide Revenue (GNI) per capita alongside earnings thresholds.
GNI represents the full earnings earned by folks and companies in a rustic. We are going to analyze GNI values from 2000 to 2018, categorized by nation. This management is appropriate with desktop platforms resembling Home windows and Mac, in addition to cellular platforms like Android and iOS.
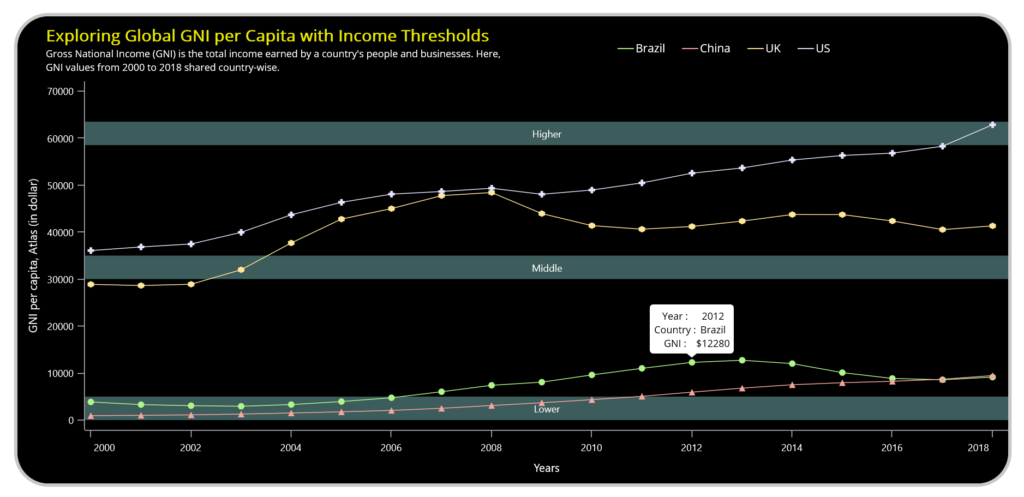
Let’s get began!
Step 1: Gathering GNI knowledge by nation
First, collect knowledge on the GNI per capita. Select the international locations during which you want knowledge and the format for downloading it. For instance, get hold of GNI knowledge for Brazil, China, the UK, and the US, and obtain it in CSV format. After downloading, use the info from 2000 to 2018.
Step 2: Making ready knowledge for the chart
Create a mannequin class that features properties for storing details about GNI (Gross Nationwide Revenue) country-by-country, together with the corresponding 12 months.
Discuss with the next code instance.
public class Mannequin { public DateTime Yr { get; set; } public double BrazilGNI { get; set; } public double ChinaGNI { get; set; } public double UKGNI { get; set; } public double USGNI { get; set; } public Mannequin(DateTime 12 months, double brazilGNI, double chinaGNI, double ukGNI, double usGNI) { Yr = 12 months; BrazilGNI = brazilGNI; ChinaGNI = chinaGNI; UKGNI = ukGNI; USGNI = usGNI; } }
Subsequent, learn the CSV file and retailer the GNI values country-wise with the corresponding 12 months within the observable assortment of the EconomicRate property. Discuss with the next code instance.
public class ViewModel { non-public DateTime 12 months; public ViewModel() { EconomicRate = new ObservableCollection(); ReadCSVFile(); } non-public void ReadCSVFile() { Meeting executingAssembly = typeof(App).GetTypeInfo().Meeting; Stream inputStream = executingAssembly.GetManifestResourceStream("EconomicRatesDemo.Assets.dataset.csv"); string line; ObservableCollection traces = new ObservableCollection(); if (inputStream != null) { utilizing (StreamReader reader = new StreamReader(inputStream)) { whereas ((line = reader.ReadLine()) != null) { traces.Add(line); } } traces.RemoveAt(0); foreach (var objects in traces) { string[] knowledge = objects.Cut up(','); if (int.TryParse(knowledge[0], out int yearValue)) { 12 months = new DateTime(yearValue, 1, 1); } double brazilGNI = Convert.ToDouble(knowledge[1]); double chinaGNI = Convert.ToDouble(knowledge[2]); double ukGNI = Convert.ToDouble(knowledge[3]); double usGNI = Convert.ToDouble(knowledge[4]); EconomicRate.Add(new Mannequin(12 months, brazilGNI, chinaGNI, ukGNI, usGNI)); } } } }
Step 3: Structure definition
Outline the format utilizing a Border ingredient. Contained in the border, we’ll make the most of a Grid to position our parts.
Discuss with the next code instance.
<Border Background="#e8ebe0" StrokeThickness="4" StrokeShape="{OnPlatform Default= RoundRectangle 40, Android= RoundRectangle 35}" Margin="{OnPlatform Default=30, Android= 10}"> <Grid RowDefinitions="80,*" ColumnDefinitions="*,*" BackgroundColor="Black"> <VerticalStackLayout Grid.Row="0" Grid.Column="0"> … </VerticalStackLayout> <HorizontalStackLayout Grid.Row="0" Grid.Column="1"> … </HorizontalStackLayout> </Grid> </Border>
Step 4: Configuring the Syncfusion .NET MAUI Cartesian Charts
Now, configure the Syncfusion .NET MAUI Cartesian Charts management utilizing this documentation.
Discuss with the next code instance.
<chart:SfCartesianChart Grid.Row="1" Grid.ColumnSpan="2"> <chart:SfCartesianChart.XAxes> <chart:DateTimeAxis > … </chart:DateTimeAxis> </chart:SfCartesianChart.XAxes> <chart:SfCartesianChart.YAxes> <chart:NumericalAxis > … </chart:NumericalAxis> </chart:SfCartesianChart.YAxes> </chart:SfCartesianChart>
Step 5: Binding knowledge to the chart
We’ll make the most of the Syncfusion Line sequence cases to visualise the GNI per nation yearly. Bind the ViewModel to the web page’s binding context to allow entry to ViewModel properties. Then, bind the Yr and GNI properties to the X and Y axes utilizing the XBindingPath and YBindingPath respectively. Moreover, bind the gathering to ItemsSource to the EconomicRate.
Discuss with this code instance.
<ContentPage.BindingContext> <native:ViewModel/> </ContentPage.BindingContext> <chart:SfCartesianChart Grid.Row="1" Grid.ColumnSpan="2"> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="BrazilGNI" /> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="ChinaGNI" /> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="UKGNI" /> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="USGNI" /> </chart:SfCartesianChart>
Step 6: Setting plot bands to the Y-axis.
We use NumericalPlotBand to shade three areas: decrease GNI, center GNI, and higher GNI areas and likewise add corresponding textual content. These plot bands are created and added to the NumericalPlotBandCollection. The Begin and Finish APIs decide every shading area’s starting and finish positions. We will customise the textual content for the plot bands utilizing ChartPlotBandLabelStyle.
Discuss with the next code instance.
<chart:SfCartesianChart.YAxes> <chart:NumericalAxis > <!—Decrease GNI plot band--> <chart:NumericalAxis.PlotBands> <chart:NumericalPlotBandCollection> <chart:NumericalPlotBand Begin="0" Finish="5000" Fill="#3d5c5c" Textual content="Decrease"> <chart:NumericalPlotBand.LabelStyle> <chart:ChartPlotBandLabelStyle TextColor="White"/> </chart:NumericalPlotBand.LabelStyle> </chart:NumericalPlotBand> <!--Center GNI plot band--> <chart:NumericalPlotBand Begin="30000" Finish="35000" Fill="#3d5c5c" Textual content="Center"> <chart:NumericalPlotBand.LabelStyle> <chart:ChartPlotBandLabelStyle TextColor="White"/> </chart:NumericalPlotBand.LabelStyle> </chart:NumericalPlotBand> <!--Larger GNI plot band--> <chart:NumericalPlotBand Begin="58500" Finish="63500" Fill="#3d5c5c" Textual content="Larger"> <chart:NumericalPlotBand.LabelStyle> <chart:ChartPlotBandLabelStyle TextColor="White"/> </chart:NumericalPlotBand.LabelStyle> </chart:NumericalPlotBand> </chart:NumericalPlotBandCollection> </chart:NumericalAxis.PlotBands> </chart:NumericalAxis> </chart:SfCartesianChart.YAxes>
Step 7: Customizing the chart look
Let’s improve the visible enchantment of the road sequence together with plot bands and their parts. We’ll customise the look of the road graph by adjusting numerous properties and kinds.
Including chart title
Within the VerticalStackLayout, a Label ingredient is used to position the chart title and outline vertically. Within the HorizontalStackLayout, BoxView parts and labels are added to point which international locations have which line sequence, utilizing colour within the BoxView. Customise the chart title like within the following code instance.
<VerticalStackLayout Grid.Row="0" Grid.Column="0"> <Label Textual content="Exploring International GNI per Capita with Revenue Thresholds" TextColor="#e6e600" FontAttributes="Daring" FontSize="{OnPlatform Android=15, iOS= 15, Default= 20 }" Margin="{OnPlatform Default="35,10,0,0"}"/> <Label Textual content="Gross Nationwide Revenue (GNI) is the full earnings earned by a rustic's folks and companies. Right here, GNI values from 2000 to 2018 shared country-wise." TextColor="White" FontSize="12" Margin="{OnPlatform Default="35,0,0,0"}"/> </VerticalStackLayout> <HorizontalStackLayout Grid.Row="0" Grid.Column="1" HeightRequest="40" WidthRequest="350"> <BoxView HeightRequest="1" WidthRequest="20" BackgroundColor="#b1f48a"/> <Label Textual content="Brazil" TextColor="White" Margin="{OnPlatform Default="2,9,0,0"}" /> <BoxView HeightRequest="1" WidthRequest="20" BackgroundColor="#f7a7a1" Margin="{OnPlatform Default="20,0,0,0"}"/> <Label Textual content="China" TextColor="White" Margin="{OnPlatform Default="2,9,0,0"}"/> <BoxView HeightRequest="1" WidthRequest="20" BackgroundColor="#ffe799" Margin="{OnPlatform Default="20,0,0,0"}"/> <Label Textual content="UK" TextColor="White" Margin="{OnPlatform Default="2,9,0,0"}"/> <BoxView HeightRequest="1" WidthRequest="20" BackgroundColor="#e6e6ff" Margin="{OnPlatform Default="20,0,0,0"}"/> <Label Textual content="US" TextColor="White" Margin="{OnPlatform Default="2,9,0,0"}"/> </HorizontalStackLayout>
Customizing the axes
Let’s customise the first and secondary axes utilizing the next properties:
ShowMajorGridLines – Management the visibility of grid traces.
PlotOffsetStart – Used to supply padding to the axis initially place.
PlotOffserEnd – Used to supply padding to the axis on the finish place.
Title – Used to set titles for chart axes.
ChartAxisLabelStyle – Used to customise axis labels.
Discuss with the next code instance.
<chart:SfCartesianChart.XAxes> <chart:DateTimeAxis ShowMajorGridLines="False" PlotOffsetStart="7" PlotOffsetEnd="20"> <chart:DateTimeAxis.Title> <chart:ChartAxisTitle Textual content="Years" TextColor="White"/> </chart:DateTimeAxis.Title> <chart:DateTimeAxis.LabelStyle> <chart:ChartAxisLabelStyle TextColor="White"/> </chart:DateTimeAxis.LabelStyle> </chart:DateTimeAxis> </chart:SfCartesianChart.XAxes> <chart:SfCartesianChart.YAxes> <chart:NumericalAxis ShowMajorGridLines="False" PlotOffsetStart="{OnPlatform Android=5}" > <chart:NumericalAxis.Title> <chart:ChartAxisTitle Textual content="GNI per capita, Atlas (in greenback)" TextColor="White"/> </chart:NumericalAxis.Title> <chart:NumericalAxis.LabelStyle> <chart:ChartAxisLabelStyle TextColor="White"/> </chart:NumericalAxis.LabelStyle> </chart:NumericalAxis> </chart:SfCartesianChart.YAxes>
Customizing the road sequence marker
Let’s customise the road sequence marker utilizing the next properties:
ShowMarker: Used to focus on the place of the info level.
MarkerSettings: To alter the sequence markers’ look, create an occasion of the MarkerSettings property. The next properties are used to customise marker look.
- Kind: Used to explain the marker form.
- Peak: Signifies the peak of the marker.
- Width: Signifies the width of the marker.
Discuss with the next code instance.
<chart:SfCartesianChart> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="BrazilGNI" ShowMarkers="True"> <chart:LineSeries.MarkerSettings> <chart:ChartMarkerSettings Kind="Circle" Peak="8" Width="8" /> </chart:LineSeries.MarkerSettings> </chart:LineSeries> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="ChinaGNI" ShowMarkers="True"> <chart:LineSeries.MarkerSettings> <chart:ChartMarkerSettings Kind="Triangle" Peak="8" Width="8"/> </chart:LineSeries.MarkerSettings> </chart:LineSeries> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="UKGNI" ShowMarkers="True"> <chart:LineSeries.MarkerSettings> <chart:ChartMarkerSettings Kind="Hexagon" Peak="8" Width="8"/> </chart:LineSeries.MarkerSettings> </chart:LineSeries> <chart:LineSeries ItemsSource="{Binding EconomicRate}" XBindingPath="Yr" YBindingPath="USGNI" ShowMarkers="True"> <chart:LineSeries.MarkerSettings> <chart:ChartMarkerSettings Kind="Plus"/> </chart:LineSeries.MarkerSettings> </chart:LineSeries> </chart:SfCartesianChart>
Customizing the road sequence look
Utilizing the PaletteBrushes property, we will apply customized brushes to line sequence. This property is sure to a set of brushes named CustomBrushes.
<chart:SfCartesianChart PaletteBrushes="{Binding CustomBrushes}"> … </chart:SfCartesianChart>
public Checklist<Brush> CustomBrushes { get; set; } CustomBrushes = new Checklist<Brush>() { new SolidColorBrush(Colour.FromArgb("#b1f48a")), new SolidColorBrush(Colour.FromArgb("#f7a7a1")), new SolidColorBrush(Colour.FromArgb("#ffe799")), new SolidColorBrush(Colour.FromArgb("#e6e6ff")), };
Customizing the tooltip utilizing a template
Utilizing the TooltipTemplate property within the sequence permits customization of the tooltip’s look. A knowledge template with the info factors’ 12 months, nation identify, and GNI values is created and sure to the TooltipTemplate property, with the tooltip enabled. Every line sequence knowledge level shows its data within the tooltip template.
<chart:SfCartesianChart> <chart:SfCartesianChart.Assets> <!--BrazilGNI line sequence tooltip template--> <DataTemplate x:Key="tooltipTemplate1"> <Grid RowDefinitions="*,*,*" ColumnDefinitions="Auto,*"> <Label Grid.Row="0" Grid.Column="0" Textual content="Yr : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="0" Grid.Column="1" Textual content="{Binding Merchandise.Yr,StringFormat="{0:yyyy}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="0" Textual content="Nation : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="1" Textual content="Brazil" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart" /> <Label Grid.Row="2" Grid.Column="0" Textual content="GNI : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="2" Grid.Column="1" Textual content="{Binding Merchandise.BrazilGNI,StringFormat="${0}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> </Grid> </DataTemplate> <!--ChinaGNI line sequence tooltip template--> <DataTemplate x:Key="tooltipTemplate2"> <Grid RowDefinitions="*,*,*" ColumnDefinitions="Auto,*" > <Label Grid.Row="0" Grid.Column="0" Textual content="Yr :" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="0" Grid.Column="1" Textual content="{Binding Merchandise.Yr,StringFormat="{0:yyyy}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="0" Textual content="Nation : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="1" Textual content="China" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="2" Grid.Column="0" Textual content="GNI : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="2" Grid.Column="1" Textual content="{Binding Merchandise.ChinaGNI,StringFormat="${0}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> </Grid> </DataTemplate> <!--UKGNI line sequence tooltip template--> <DataTemplate x:Key="tooltipTemplate3"> <Grid RowDefinitions="*,*,*" ColumnDefinitions="Auto,*" > <Label Grid.Row="0" Grid.Column="0" Textual content="Yr :" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="0" Grid.Column="1" Textual content="{Binding Merchandise.Yr,StringFormat="{0:yyyy}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="0" Textual content="Nation : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="1" Textual content="UK" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Begin"/> <Label Grid.Row="2" Grid.Column="0" Textual content="GNI : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="2" Grid.Column="1" Textual content="{Binding Merchandise.UKGNI,StringFormat="${0}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> </Grid> </DataTemplate> <!--USGNI line sequence tooltip template--> <DataTemplate x:Key="tooltipTemplate4"> <Grid RowDefinitions="*,*,*" ColumnDefinitions="Auto,*" > <Label Grid.Row="0" Grid.Column="0" Textual content="Yr :" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="0" Grid.Column="1" Textual content="{Binding Merchandise.Yr,StringFormat="{0:yyyy}"}" TextColor="Black" FontSize="12"/> <Label Grid.Row="1" Grid.Column="0" Textual content="Nation : " TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="1" Grid.Column="1" Textual content="US" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Begin"/> <Label Grid.Row="2" Grid.Column="0" Textual content="GNI :" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> <Label Grid.Row="2" Grid.Column="1" Textual content="{Binding Merchandise.USGNI,StringFormat="${0}"}" TextColor="Black" FontSize="12" VerticalTextAlignment="Heart" HorizontalTextAlignment="Heart"/> </Grid> </DataTemplate> </chart:SfCartesianChart.Assets> <chart:SfCartesianChart.TooltipBehavior> <chart:ChartTooltipBehavior Background="White"/> </chart:SfCartesianChart.TooltipBehavior> <chart:LineSeries EnableTooltip="True" TooltipTemplate="{StaticResource tooltipTemplate1}"/> <chart:LineSeries EnableTooltip="True" TooltipTemplate="{StaticResource tooltipTemplate2}"/> <chart:LineSeries EnableTooltip="True" TooltipTemplate="{StaticResource tooltipTemplate3}"/> <chart:LineSeries EnableTooltip="True" TooltipTemplate="{StaticResource tooltipTemplate4}"/> </chart:SfCartesianChart>
After executing the above code examples, we are going to get the output that resembles the next picture.
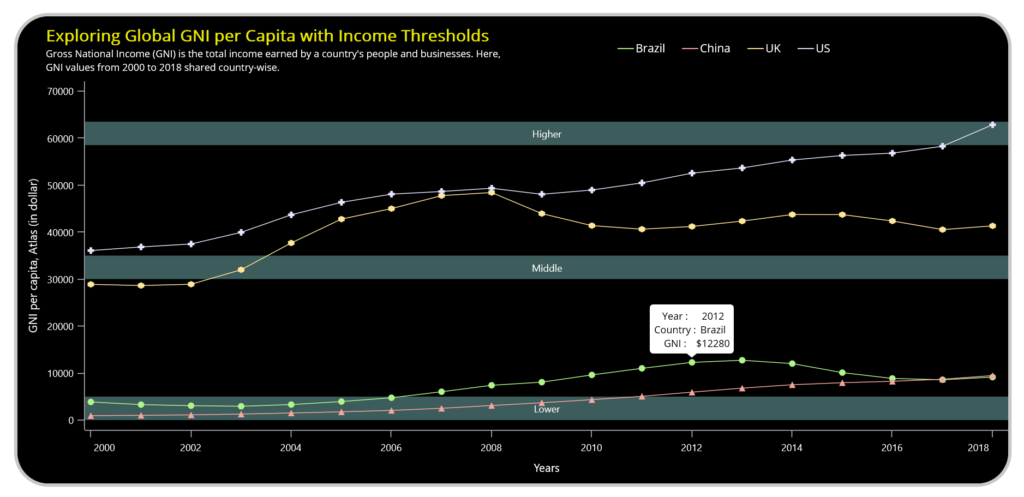
GitHub reference
For extra particulars, confer with the Line chart with plot bands to discover international GNI per capita with earnings thresholds demo.
Conclusion
Thanks for studying! We now have demonstrated the way to discover international GNI per capita with an earnings thresholds line chart utilizing the Syncfusion .NET MAUI Cartesian Charts management. We encourage you to observe these steps and share your ideas on the expertise within the remark part under.
The present prospects can obtain the newest model of Important Studio from the License and Downloads web page. In case you are new, attempt our 30-day free trial to discover our unimaginable options.
If you happen to require help, please don’t hesitate to contact us through our help boards, help portal, or suggestions portal. We’re all the time keen that can assist you!