Use Python’s built-in min()
operate with the key
argument to seek out the shortest string in a set. Name min(my_set, key=len)
to return the shortest string within the set utilizing the built-in len()
operate to find out the burden of every string—the shortest string has minimal size.
👉 Really helpful Tutorial: How you can Discover the Shortest String in a Python Listing?
Downside Formulation
Given a Python set of strings. Discover the string with the minimal variety of characters—the shortest string within the set.
Listed here are just a few instance units of strings and the specified output:
# {'Alice', 'Bob', 'Pete'} – --> 'Bob' # {'aaa', 'aaaa', 'aa'} – --> 'aa' # {''} – --> '' # {} – --> ''
Technique 1: min() Perform with Key Argument Set to len()
Use Python’s built-in min()
operate with a key argument to seek out the shortest string in a set like so: min(s, key=len)
. This returns the shortest string within the set s
utilizing the built-in len()
operate to find out the burden of every string—the shortest string would be the minimal.
Right here’s the code definition of the get_min_str()
operate that takes a set of strings as enter and returns the shortest string within the listing or a ValueError
if the set is empty.
def get_min_str(my_set): return min(my_set, key=len)
Right here’s the output we get hold of when working our desired examples:
print(get_min_str({'Alice', 'Bob', 'Pete'})) # 'Bob' print(get_min_str({'aaa', 'aaaa', 'aa'})) # 'aa' print(get_min_str({''})) # ''
When you go an empty set, Python will elevate a ValueError: min() arg is an empty sequence
since you can’t go an empty iterable into the min()
operate.
print(get_min_str({})) # ValueError: min() arg is an empty sequence
Technique 2: Dealing with Empty Units
If you wish to return an alternate worth in case the set is empty, you possibly can modify the get_min_str()
operate to incorporate a second elective argument:
def get_min_str(my_set, fallback=''): return min(my_set, key=len) if my_set else fallback print(get_min_str({})) # '' print(get_min_str({}, fallback='EMPTY!!!!!!')) # EMPTY!!!!!!
Technique 3: Not-So-Pythonic with For Loop
A much less Pythonic however, for newbie coders, extra readable model is the next loop-based answer:
def get_min_str(my_set, fallback=''): if not my_set: return fallback min_str="" # set will not be empty for x in my_set: if len(x) < len(max_str): min_str = x return min_str print(get_min_str({'Alice', 'Bob', 'Pete'})) # 'Bob' print(get_min_str({'aaa', 'aaaa', 'aa'})) # 'aa' print(get_min_str({''})) # '' print(get_min_str({}, fallback='EMPTY!!!!!!')) # EMPTY!!!!!!
Technique 4: Python Min Size of String in Set
To search out the minimal size of a string in a given set, use the min(my_set, key=len)
operate to acquire the string with the minimal size after which go this min string into the len()
operate to acquire the variety of characters.
len(min(my_set, key=len))
Right here’s a extra detailed instance:
def get_min_str_length(my_set): return len(max(my_set, key=len)) print(get_min_str_length({'Alice', 'Bob', 'Pete'})) # 3 print(get_min_str_length({'aaa', 'aaaa', 'aa'})) # 2 print(get_min_str_length({''})) # 0 print(get_min_str_length({})) # ValueError: min() arg is an empty sequence
Thanks for taking the time to learn this text! 🙂 Be happy to affix us – it’s enjoyable and we’ve got cheat sheets!
👉 Really helpful Tutorial: How you can Get the Most String in a Python Set?
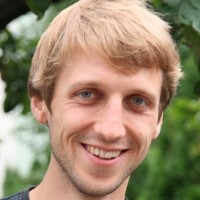
Whereas working as a researcher in distributed programs, Dr. Christian Mayer discovered his love for instructing laptop science college students.
To assist college students attain greater ranges of Python success, he based the programming training web site Finxter.com. He’s creator of the favored programming ebook Python One-Liners (NoStarch 2020), coauthor of the Espresso Break Python collection of self-published books, laptop science fanatic, freelancer, and proprietor of one of many high 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his biggest ardour is to serve aspiring coders via Finxter and assist them to spice up their expertise. You’ll be able to be a part of his free electronic mail academy right here.