With visible guides in easy language

TypeScript could be riddled with errors and warnings, for seemingly each little factor. Take this perform for instance:
(In the event you’re unsure what :quantity
would possibly imply inside the perform arguments, I cowl that, and extra in an earlier chapter of this information. However no worries, we’ll revisit it frivolously on this article as nicely.)
In strict mode, with a TS eslint setting, TypeScript will really shout warnings at you for this completely working perform that even makes use of TypeScript! The error would appear to be this: Lacking return kind on perform
.
Rummaging by errors generally is a headache, so on this article, we’re going to cowl a listing of errors and tips on how to perceive and resolve them, in depth. Since there are a ton of various errors in TypeScript, this chapter might be damaged into many components in an effort to cowl a variety of errors. Be happy to bookmark this and revisit it in case you run into any of those pesky errors sooner or later. Let’s start!
1. “Lacking return kind on perform”
Motive:
We didn’t inform TypeScript what we anticipate to be returned from the perform. Lots of occasions, TypeScript can assume this, however in strict mode, it should anticipate you to declare this.
Resolution:
Place a colon after the closing param of your perform argument, and write the kind(s) that you simply anticipate the perform to return, like so:
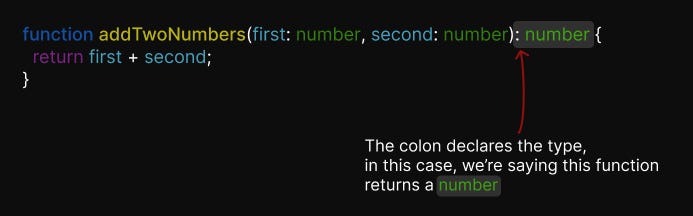
Tip: In the event you’re unsure which sort(s) could be returned out of your perform, you possibly can hover over the perform identify in your textual content editor, and TypeScript will present you a return kind that can be utilized. This may work 99% of the time!

Why returning a sort is essential:
Features can doubtlessly return a number of totally different outcomes. Even one thing easy like addTwoNumbers
might return quantity
,NaN
,null
, void
, or perhaps a string
relying on the way you design it. Thus, TS desires you to be clear on itemizing the entire doable return values it ought to anticipate.
That is particularly helpful for those who’re constructing an API that different folks will work with. Serving to them reliably know what information kind(s) to anticipate can distinguish your API from others that don’t.
The cool a part of returning a sort is that as you refactor or replace a perform, for those who by accident (or unknowingly) neglect to return an essential, anticipated property, TS will let . Moreover, it may auto-complete a listing of accessible properties for you that will help you uncover extra in regards to the information you’re engaged on throughout the code. We’ll cowl all of this shortly.
We’re going to make use of this small software I made for this text to see a number of different errors (and advantages) in motion. Be happy to poke round in there… we’ll cowl it intimately.
Let’s unpack the applying above. On line 25
, we’re utilizing a getUserByRole
perform to return some person information primarily based on their function
. On this software, the person can both be an admin
, or a visitor
.
Utilizing an interface, we will describe what an AdminUser
and a GuestUser
appear to be and talk that to our perform by placing :AdminUser | GuestUser
after its argument physique. That is how I like to visualise it:

Since we outlined what an AdminUser
and a GuestUser
appears like, TypeScript can do a number of cool issues for us. Take this code for instance:
Simply by writing if (function === 'admin')
, TypeScript can inform that we’re speaking in regards to the AdminUser
and put together to anticipate their particular form of knowledge to be returned. That is referred to as Kind Narrowing. It’s once you slender down a listing of sorts (formally referred to as a union, it appears like this → type1 | type2
) to a smaller, extra particular listing reminiscent of AdminUser | GuestUser
to simply AdminUser
. TypeScript can determine this out by evaluating the properties belonging to the categories that we offered.

TypeScript helps to supply a listing of properties obtainable for the AdminUser
. This can provide us helpful perception into the item.
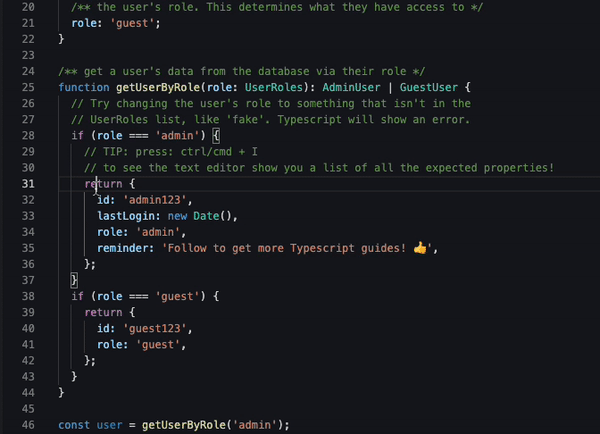
Attempt it for your self! Within the software under, remark strains 33–35.
Then, whereas your cursor is inside the item’s physique, press ctrl + I
or cmd + I
(for Mac) to get a full listing of the properties that belong to the AdminUser
!
Be aware: The properties listing will solely present the item properties that aren’t at present listed. So, for those who’re already returning all of its properties, that command gained’t present any extra obtainable properties.
What if we tried to examine for a person with a job that doesn’t exist, like “author”? Resembling if (function === ‘author’)
. That will lead us to our subsequent error.
2. This situation will at all times return ‘false’ because the sorts ‘UserRoles’ and ‘“author
”’ haven’t any overlap.
Motive:
One (or extra) of the values we tried to match don’t align with what TypeScript was informed. Within the software instance above, it’s because we solely have two roles, “admin” and “visitor.” A “author” function doesn’t exist in our definition. Right here’s how I like to visualise it:

This situation will at all times return ‘false’ because the sorts ‘UserRoles’ and ‘“author”’ haven't any overlap
Let’s break the error down phrase for phrase.
This situation
— The conditional analysis. That’s the if
block, or it might be a ternary
, and so on. Something that compares values and ends in true
or false
.
will at all times return ‘false’
— It’s by no means going to run that physique of code! It gained’t step inside the conditional, as a result of it is at all times false… principally this: if(false) {/* won't ever run */}
.
because the sorts ‘UserRoles’
— On this case, ‘UserRoles’ is the worth that TypeScript is referencing as its supply of reality. Please be aware that UserRoles
is the identify that I selected, it might be something you need. In your software, this may match whichever kind was offered to TS.
and ‘“author”’
— That is the worth you’re attempting to make use of, which precipitated the error.
haven't any overlap
— The worth you’re attempting to make use of doesn’t exist throughout the supply of reality (UserRoles
) that TypeScript is referencing. You’ll be able to both add it to the listing that was offered to TS or persist with utilizing one of many values within the union (UserRoles
).
Resolution:
One of many following will work:
A. Replace the kind UserRoles
(on this instance) to incorporate “author”, like so: kind UserRoles = ‘admin’ | ‘visitor’ | ‘author’
.
Or
B. Fallback to utilizing a price offered in UserRoles
(on this case, ‘admin’ or ‘visitor’) so we get “overlap” within the values used.
For the subsequent error (one of the widespread in TS), hop into the textual content editor under and alter any string worth to a distinct information kind, like a quantity. For instance, on line 40
, change guest123
to a quantity, like 2
.
3. Kind ‘quantity’ is just not assignable to kind ‘string’
Or Kind ‘[TYPE_1]’ is just not assignable to kind ‘[TYPE_2]’
Motive:
We’re making an attempt to make use of a sort that doesn’t match what TypeScript is anticipating. The primary kind, TYPE_1
, is what we try to make use of. The opposite kind, TYPE_2
, is the one which TypeScript anticipated. Right here’s how I like to visualise this error:

We informed TypeScript that the function
might solely be “visitor,” then we rotated and tried to set a quantity, 78
, as the worth for function
. This occurs typically, particularly with complicated information and APIs that we didn’t construct.
Resolution:
Make your kind match what is predicted, or replace the expectations. Within the instance above, one answer might be to vary the quantity 78
to "visitor"
or, if we wished to permit the quantity 78
particularly. We might replace the GuestUser
interface to permit 78
or any generic quantity
worth. Right here’s an instance: