Loops permit us to cycle by way of objects in arrays or objects and do issues like print them, modify them, or carry out different kinds of duties or actions. There are totally different sorts of loops, and the for loop in JavaScript permits us to iterate by way of a set (equivalent to an array).
On this article, we’ll study concerning the for
loop JavaScript gives. We’ll have a look at how for...in
loop statements are utilized in JavaScript, the syntax, examples of the way it works, when to make use of or keep away from it, and what different sorts of loops we will use as an alternative.
Why Use For Loops in JavaScript Code
In JavaScript, simply as in different programming languages, we use loops to learn or entry the objects of a set. The gathering will be an array or an object. Each time the loop assertion cycles by way of the objects in a set, we name it an iteration.
There are two methods to entry an merchandise in a set. The primary means is through its key within the assortment, which is an index in an array or a property in an object. The second means is through the merchandise itself, with no need the important thing.
Definition of the for…in Loop
The JavaScript for
loop goes by way of or iterates keys of a set. Utilizing these keys, you’ll be able to then entry the merchandise it represents within the assortment.
The gathering of things will be both arrays, objects, and even strings.
Syntax of the for…in Loop
The for
loop has the next syntax or construction:
for (let key in worth) {
}
On this code block, worth
is the gathering of things we’re iterating over. It may be an object, array, string, and so forth. key
would be the key of every merchandise in worth
, altering on every iteration to the subsequent key within the record.
Observe that we use let
or const
to declare key
.
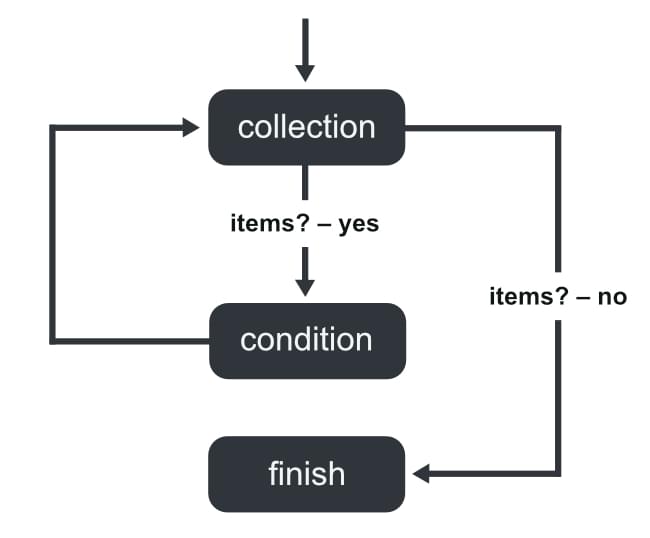
Utilizing the for Loop with Objects
When utilizing for...in
loop to iterate an object in JavaScript, the iterated keys or properties — which, within the snippet above, are represented by the key
variable — are the article’s personal properties.
As objects would possibly inherit objects by way of the prototype chain, which incorporates the default strategies and properties of Objects in addition to Object prototypes we’d outline, we should always then use hasOwnProperty.
For loop instance over an iterable object
Within the following instance, we’re looping over the variable obj
and logging every property and worth:
const obj = {
"a": "JavaScript",
1: "PHP",
"b": "Python",
2: "Java"
};
for (let key in obj) {
console.log(key + ": " + obj[key] )
}
Discover that the order of the iteration is ascending for the keys (that’s, beginning with digits in numerical order after which letters in alphabetical order). Nonetheless, this outputted order is totally different from the index order of the objects as created when initializing the article.
See the Pen
Looping Objects by SitePoint (@SitePoint)
on CodePen.
Utilizing a for…in Loop with Arrays
When utilizing the for...in
loop to iterate arrays in JavaScript, key
on this case would be the indices of the weather. Nonetheless, the indices is likely to be iterated in a random order.
So, if the worth
variable within the for...in
loop syntax construction we confirmed above was an array of 5 objects, key
wouldn’t be assured to be 0 to 4. Some indices would possibly precede others. Particulars on when this would possibly occur is defined later on this article.
For…in loop array instance
Within the instance beneath, we’re looping over the next variable arr
:
const arr = ["JavaScript", "PHP", "Python", "Java"];
for (let key in arr) {
console.log(key + ": " + arr[key])
}
And within the loop, we’re rendering the index and the worth of every array component.
See the Pen
Looping Arrays by SitePoint (@SitePoint)
on CodePen.
Utilizing a for…in Loop with Strings
You’ll be able to loop over a string with the JavaScript for...in
loop. Nonetheless, it’s not really helpful to take action, as you’ll be looping over the indices of the characters relatively than the characters themselves.
A for…in loop string instance
Within the instance beneath, we’re looping over the next variable str
:
const str = "Good day!";
for (let key in str) {
console.log(key + ": " + str.charAt(key));
}
Contained in the loop, we’re rendering the important thing, or index of every character, and the character at that index.
See the Pen
Strings Loop by SitePoint (@SitePoint)
on CodePen.
Let’s have a look at the conditions that the JavaScript for...in
loop is finest suited to.
Iterating objects with a JavaScript for…in loop
As a result of the for...in
loop solely iterates the enumerable properties of an object — that are the article’s personal properties relatively than properties like toString
which can be a part of the article’s prototype — it’s good to make use of a for...in
loop to iterate objects. A for...in
loop gives a straightforward approach to iterate over an object’s properties and finally its values.
Debugging with a for…in loop
One other good use case for the JavaScript for...in
loop is debugging. For instance, chances are you’ll wish to print to the console or an HTML component the properties of an object and its values. On this case, the for...in
loop is an efficient selection.
When utilizing the for...in
loop for debugging objects and their values, it is best to all the time needless to say iterations will not be orderly, that means that the order of things that the loop iterates will be random. So, the order of the properties accessed may not be as anticipated.
When To not Use a JavaScript for…in Loop
Now let’s have a look at conditions the place a for...in
loop isn’t the best choice.
Orderly iterating of arrays
Because the index order within the iterations shouldn’t be assured when utilizing the for...in
loop, it’s really helpful to not iterate arrays if sustaining the order is important.
That is particularly important for those who’re seeking to help browsers like IE, which iterates within the order the objects are created relatively than within the order of the indices. That is totally different from the best way present fashionable browsers work, which iterate arrays primarily based on the indices in ascending order.
So, for instance, you probably have an array of 4 objects and also you insert an merchandise into place three, in fashionable browsers the for...in
loop will nonetheless iterate the array so as from 0 to 4. In IE, when utilizing a for...in
loop, it’ll iterate the 4 objects that have been initially within the array to start with after which attain the merchandise that was added at place three.
Making modifications whereas iterating
Any addition, deletion, or modification to properties doesn’t assure an ordered iteration. Making modifications to properties in a for...in
loop must be prevented. That is largely attributable to its unordered nature.
So, for those who delete an merchandise earlier than you attain it within the iteration, the merchandise won’t be visited in any respect in all the loop.
Equally, for those who make modifications to a property, it doesn’t assure that the merchandise received’t be revisited once more. So, if a property is modified it is likely to be visited twice within the loop relatively than as soon as.
As well as, if a property is added throughout iteration, it’d or may not be visited in the course of the iteration in any respect.
As a consequence of these conditions, it’s finest to keep away from making any alterations, deletions, or additions to an object in a for...in
loop.
Right here’s an instance of including a component in a for...in
loop. We are able to see the results of the primary loop then the second after making additions within the first loop.
See the Pen
Addition in Object Loop by SitePoint (@SitePoint)
on CodePen.
As you’ll be able to see within the above instance, the weather that have been added weren’t iterated over.
Alternate options to For Loops in JavaScript
So in these conditions the place a for...in
loop isn’t the best choice, what must you use as an alternative? Let’s have a look.
Utilizing a for loop with arrays
It’s by no means incorrect to make use of a for
loop! The JavaScript for
loop is without doubt one of the most simple instruments for looping over array components. The for
loop means that you can take full management of the indices as you iterate an array.
Which means when utilizing the for
loop, you’ll be able to transfer forwards and backwards, change objects within the array, add objects, and extra, whereas nonetheless sustaining the order of the array.
The next assertion creates a loop that iterates over an array and prints its values to the console.
for (let i = 0; i < arr.size; i++) {
console.log(arr[i]);
}
forEach technique for arrays and objects
forEach in JavaScript is a technique on array prototypes that permits us to iterate over the weather of an array and their indices in a callback perform.
Callback features are features that you simply move to a different technique or perform to be executed as a part of the execution of that technique or perform. In terms of forEach
in JavaScript, it signifies that the callback perform will likely be executed for every iteration receiving the present merchandise within the iteration as a parameter.
For instance, the next assertion iterates over the variable arr
and prints its values within the console utilizing forEach
:
arr.forEach((worth) => console.log(worth));
You too can entry the index of the array:
arr.forEach((worth, index) => console.log(worth, index));
JavaScript forEach
loops can be used to iterate objects through the use of Object.keys(), passing it the article you wish to iterate over, which returns an array of the article’s personal properties:
Object.keys(obj).forEach((key) => console.log(obj[key]));
Alternatively, you’ll be able to forEach
to loop over the values of properties instantly for those who don’t want entry to the properties utilizing Object.values():
Object.values(obj).forEach((worth) => console.log(worth));
Observe that Object.values()
returns the objects in the identical order as for...in
.
Conclusion
Through the use of the JavaScript for...in
loop, we will loop by way of keys or properties of an object. It may be helpful when iterating object properties or for debugging, however must be prevented when iterating arrays or making modifications to the article. I hope you’ve discovered the above examples and explanations helpful.