Surroundings variables are utilized by the working system and functions to retailer configuration settings, system paths, and different info. All packages in your pc can entry this knowledge. We will use PowerShell to view, change, or set an surroundings variable.
To set surroundings (env) variables you generally use the System Properties display, however when it is advisable to add or change a variable on a number of computer systems, then it’s simpler to make use of PowerShell.
On this article, I’ll clarify the totally different env variables and learn how to set surroundings variables with PowerShell.
Surroundings Variables
Earlier than we’re going to have a look at learn how to handle the variables with PowerShell, let’s first take a more in-depth have a look at the surroundings (env) variables to get a greater understanding of them. Surroundings variables are mainly key-value pairs which are a part of the surroundings during which a course of runs.
There are three scopes on Home windows the place an surroundings variable may be outlined:
- Consumer scope
- Machine (System) scope
- Course of scope
The consumer scope incorporates principally paths to the consumer’s profile folder, like OneDrive, Appdata, or temp folder. Within the machine scope, you will see details about the machine, and essential variables for the system to search out and execute instructions or packages with out requiring the complete path to be specified every time.
The method scope incorporates the variables from the present course of (or PowerShell session). It can additionally checklist all variables from the consumer and machine scopes. Customized variables added to the method scope shall be misplaced when the session is closed.
So to set surroundings variables that stay out there after a reboot, and for all customers, you will have to retailer them within the machine scope. To do that you will have to have the right permissions to do that.
Get Surroundings Variables with PowerShell
Earlier than we’re going to add new surroundings variables to our system, let’s first check out learn how to get the variables in PowerShell. We will use two strategies to get and set surroundings variables in Powershell, the .NET technique [System.Environment]
and the PowerShell Surroundings supplier $env:
.
To checklist all surroundings variables you should utilize one in every of these instructions:
# .NET technique [System.Environment]::GetEnvironmentVariables() # Surroundings supplier, sorting them by identify Get-Merchandise env:* | sort-object identify # Or in brief: gi env:* | kind identify # Utilizing the drive technique dir env:
The benefit of the .NET technique is you could specify the scope for the variable. This fashion we are able to solely checklist the consumer surroundings variables for instance. However when you already know which variable you want, or wish to view all of them, then the Surroundings supplier is quite a bit shorter in fact.
So to view solely the consumer surroundings variables, we are able to specify the consumer scope within the .NET technique. Different choices are Machine
or Course of
# Exchange Consumer with Machine or Course of to view the opposite scopes [System.Environment]::GetEnvironmentVariables([System.EnvironmentVariableTarget]::Consumer)
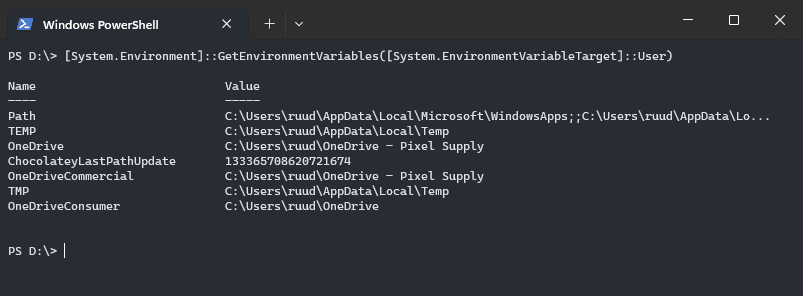
View Particular Surroundings Variable
If you wish to examine the worth of a selected variable, then you will have to know the identify a minimum of. We will then use the $env:
technique to view the worth of the variable:
# Get the OneDrive folder path $env:OneDrive
For those who don’t know the precise identify of the variable, otherwise you wish to view all OneDrive-related variables for instance, then you should utilize the Get-Merchandise
cmdlet mixed with a wildcard *
:
# Get all variables that begin with OneDrive Get-Merchandise env:OneDrive* # Consequence Title Worth ---- ----- OneDrive C:UsersruudOneDrive - Pixel Provide OneDriveConsumer C:UsersruudOneDrive OneDriveCommercial C:UsersruudOneDrive - Pixel Provide # Or get all variables that include the phrase Path Get-Merchandise env:*Path*
We will additionally get the surroundings variable with the .NET technique. For this, you will have to make use of the command [System.Environment]::GetEnvironmentVariable
. Notice that this command seems virtually an identical to the .NET technique, besides the s
is lacking on the finish of it.
To checklist the OneDrive variable worth, we are able to use the next command. Notice that we are able to additionally specify the scope of the variable, however that isn’t mandatory.
[System.Environment]::GetEnvironmentVariable("OneDrive", "Consumer")
Set ENV Variable in PowerShell
To set an surroundings (env) variable in PowerShell you should utilize two strategies, the Surroundings supplier or the [System.Environment]::SetEnvironmentVariable
technique. The latter is advisable, as a result of it means that you can set and alter variables within the machine (system) scope as effectively.
For instance, to create a brand new surroundings variable TenantId
, with our tenant ID as a price, we are able to use the next command:
[System.Environment]::SetEnvironmentVariable('TenantId','11e1234-28gv-4413-aaf8-a1e8b7f4c3d6')
Now if we have a look at the variable, then we are able to see that it’s set to the specified worth:
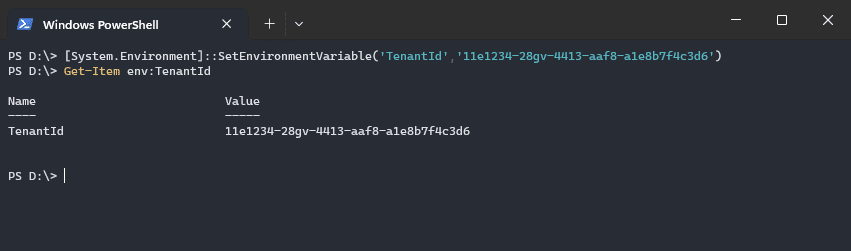
However there’s one drawback, in case you shut PowerShell, then the surroundings variable is gone. The rationale for that is that any variable you add is saved within the course of scope by default. We will remedy this by including the required scope, for instance, the machine scope:
Notice
So as to add or change variables within the machine scope, you will have to have elavated permissions. Run PowerShell in elevated (admin) mode, in any other case you get the error “Requested registry entry isn’t allowed.”
[System.Environment]::SetEnvironmentVariable('TenantId','11e1234-28gv-4413-aaf8-a1e8b7f4c3d6', 'Machine')
If we now have a look at the env variables from the machine scope, then you will notice that it’s listed:
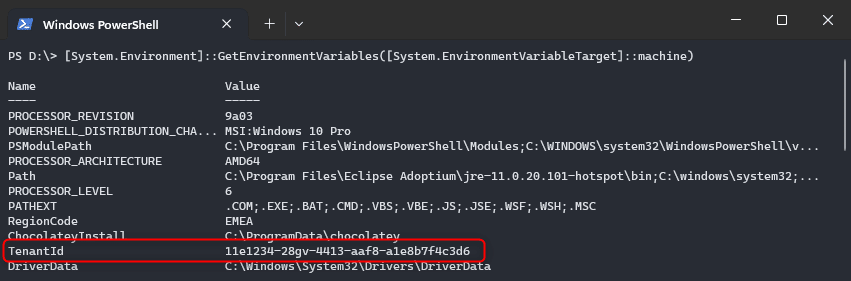
Another choice so as to add a persistent surroundings variable is so as to add the variable in your PowerShell Profile. This fashion the surroundings variable will get added/up to date each time you open PowerShell.
For instance, so as to add a brand new variable and to replace the default Path variable you’ll be able to add the next two traces in your Powershell profile:
$Env:UserPrincipalName="[email protected]" $Env:Path += ';C:Instruments
Including Momentary ENV Variables
While you don’t specify a scope when including a variable, then it’s routinely saved within the course of scope. Which means that the variable is misplaced once you shut your PowerShell session. So this technique is nice when it is advisable to add a brief surroundings variable.
To do that we are able to use the .NET technique described earlier, or we are able to use the variable syntax or Surroundings supplier. The variable syntax $Env:
is the best technique to shortly set or replace a variable:
$env:UserPrincipalName="[email protected]"
If it is advisable to replace the worth, you’ll be able to merely change it by setting a brand new worth. The values are at all times a string, which suggests we are able to append values to the variable with the +=
operator. This lets you add a path to the prevailing PATH variable, for instance.
# Change the worth of the variable $env:UserPrincipalName="[email protected]" # Including a price to the prevailing one: $env:Path += ';d:scripts'
One other technique to create or replace the variables is to make use of the Surroundings supplier. For instance, to shortly add an surroundings variable you should utilize the New-Merchandise
cmdlet:
New-Merchandise -Path Env:UserPrincipalname -Worth '[email protected]' # Consequence Title Worth ---- ----- UserPrincipalname [email protected]
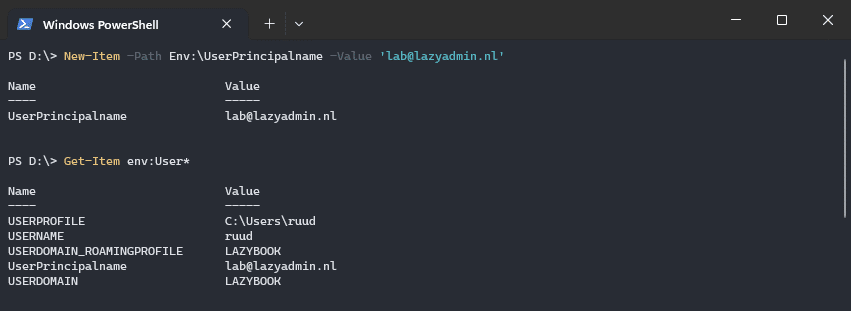
To replace or change the worth of the variable you should utilize the Set-Merchandise
cmdlet:
# Change the variable Set-Merchandise -Path Env:UserPrincipalname -Worth '[email protected]'
Eradicating Env Variables
An surroundings variable can’t be empty. So to take away it, we are able to both set the worth to an empty string (or $null
), or we are able to use the Take away-Merchandise
cmdlet to take away the variable.
To set an env variable in PowerShell to an empty string you should utilize one of many strategies described earlier:
# Set the variable to $null $env:UserPrincipalName = $null # Change it to an empty string $env:UserPrincipalName="" # Set it to an empty string with the .NET technique [Environment]::SetEnvironmentVariable('UserPrincipalName ', '', 'Machine') # Or use the Surroundings supplier Set-Merchandise -Path Env:UserPrincipalname -Worth ''
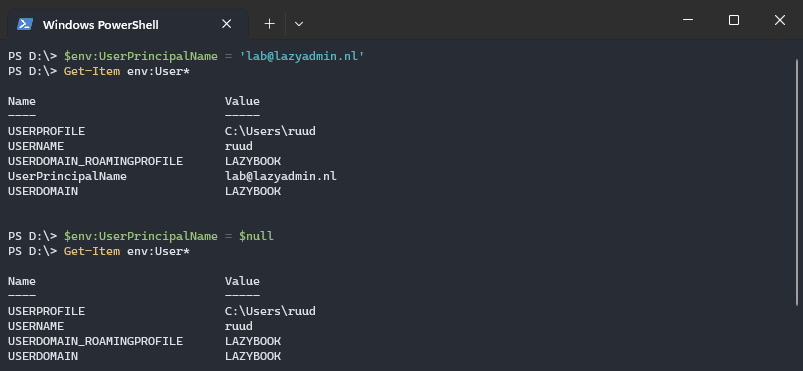
Another choice is to make use of the Take away-Merchandise
cmdlet. It can have the identical impact because the strategies above, however it might perhaps a bit extra clear that you’re truly eradicating the variable.
Take away-Merchandise -Path Env:UserPrincipalname
Wrapping Up
There are totally different strategies to set an env variable PowerShell. The simplest technique is in fact to make use of the surroundings variable, $env:
. However if you wish to create a persistent variable, then you will have to make use of the .Internet
technique and set the scope to machine.
Consider when making a variable that double examine if the variable not already exists. Overwriting system variables can have destructive results on different packages.
I hope this text helped you, when you have any questions, simply drop a remark beneath.