I’ve typically advised those who I spend my day in a PowerShell immediate. I run nearly my whole day with PowerShell. I’ve shared lots of the instruments I take advantage of every day on Github. In the present day, I wish to share one other approach I’ve PowerShell work the way in which I would like it, with minimal effort. This particular process facilities on information and folders.
ManageEngine ADManager Plus – Obtain Free Trial
As you may anticipate, I’m continuously creating, modifying, and managing information. I do all of this from a PowerShell immediate. I hardly ever use the beginning menu to discover a program to launch. My problem has at all times been discovering the information and folders I’ve lately been utilizing. Get-ChildItem is of course the PowerShell software of alternative, however I’ve lastly gotten round to creating it work the way in which I want.
Get-ChildItem Defaults
The default habits for Get-ChildItem has at all times been to kind by identify.
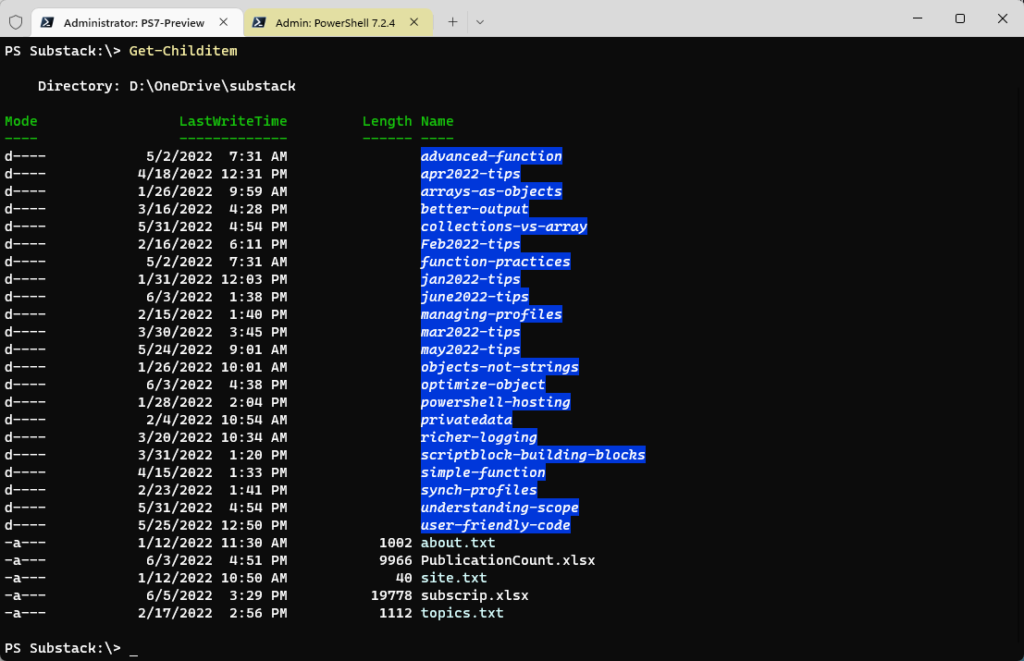
Directories are listed first, sorted by identify, after which information, additionally sorted by identify. Typically, I’m attempting to recollect what folder I used to be utilizing, and this output doesn’t make that simple for me. What I would like is a form by the LastWriteTime property. However I nonetheless need folders listed first, adopted by information.
Making a New Command
I can’t use a customized format view as a result of that received’t do the sorting. As well as, I wish to make this a straightforward course of. I do know I can run a PowerShell expression like this to get the specified outcome.
Get-ChildItem | Type-Object -Property { -Not $_.psiscontainer }, LastWritetime
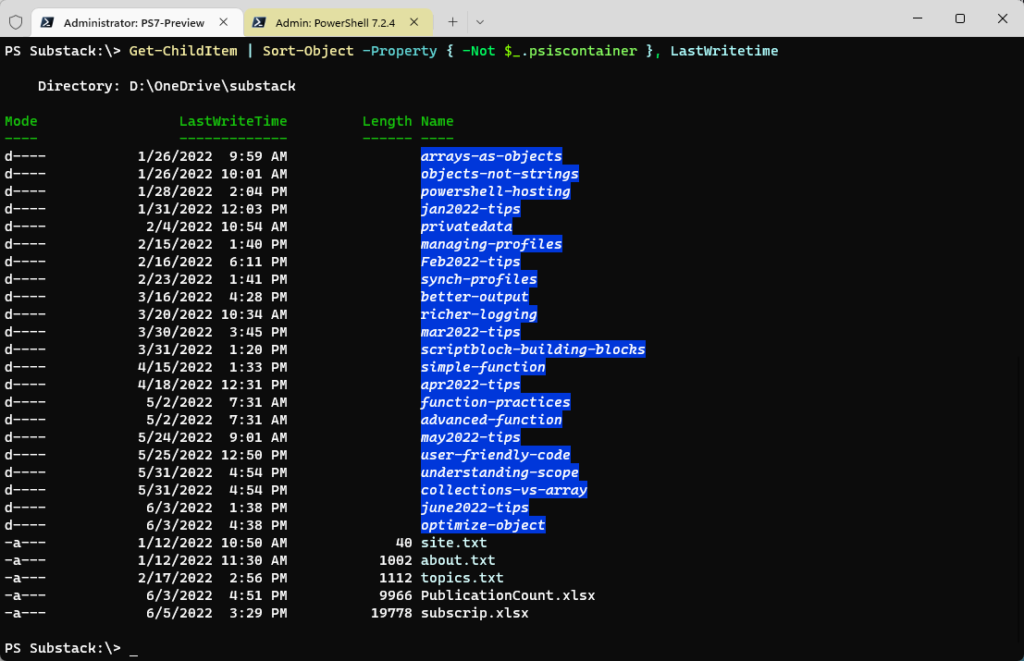
Type-Object is sorting pipeline enter on two properties. The primary is a customized property outlined on-the-fly by the scriptblock. The scriptblock appears on the PSIsContainer property, a Boolean worth. Recordsdata can have a price of True and folders a price of False. I would like folders to show first, so I’m utilizing the -Not operator to invert the worth. After objects are sorted on this property, they’re sorted on the LastWriteTime. This makes my life a lot, a lot simpler. However I’m lazy. I don’t wish to keep in mind to sort all of this, even with auto-completion through PSReadline.
How a few perform?
Perform Get-MyFolderItem {
[cmdletbinding()]
[alias("dl")]
Param(
[Parameter(Position = 0)]
[string]$Path,
[Parameter(Position = 1)]
[string]$Flter,
[switch]$File,
[switch]$Listing,
[string[]]$Exclude,
[string[]]$Embody,
[switch]$Recurse
)
#Run Get-Childitem with no matter parameters are specified.
Get-ChildItem @psboundparameters | Type-Object -Property { -Not $_.psiscontainer }, LastWritetime
}
This perform is nothing greater than a wrapper round Get-ChildItem. My perform parameters mirror these of Get-ChildItem. I’m splatting $PSBoundParameters to Get-ChildItem after which performing my kind.
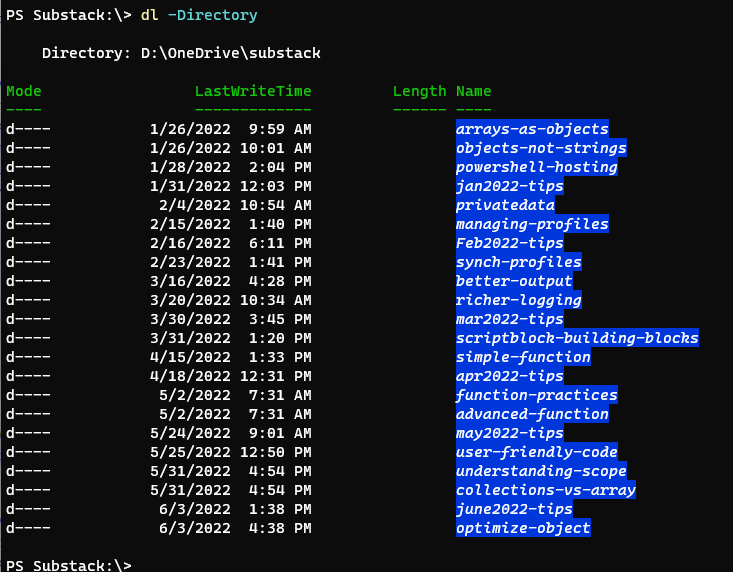
$PSBoundParameters is an intrinsic variable. It’s a specialised hashtable that accommodates each parameter with a price. I additionally added an alias to my perform to make this even simpler to make use of. I can put this perform in my PowerShell profile script, and I’m set.
Itemizing by Proxy
However there may be one other approach I can create this command. I might make a proxy perform. It is a explicit sort of perform. Proxy features typically substitute authentic instructions. Sometimes, you employ proxy features so as to add or take away parameters or customise a command’s habits. You typically see proxy features with Simply Sufficient Administration (JEA) deployments. However I might use a proxy perform constructed on Get-ChildItem.
The best strategy to get began is with Copy-Command from my PSScriptTools module.
Copy-Command Get-Childitem -AsProxy -UseForwardHelp -IncludeDynamic
Run this command within the VS Code built-in editor, and it’ll load a brand new file into the editor.
If you’re constructing proxy features, take note of your PowerShell model. I created my file in PowerShell 7. Happily, the parameters are unchanged in Home windows PowerShell, in order that I can use my proxy perform in each variations. However that will at all times not be the case.
Right here’s the proxy perform with one addition.
#requires -version 5.1
<#
It is a copy of:
CommandType Title Model Supply
----------- ---- ------- ------
Cmdlet Get-ChildItem 7.0.0.0 Microsoft.PowerShell.Administration
Created: 06 June 2022
Writer : Jeff Hicks
Created with Copy-Command from the PSScriptTools module
https://github.com/jdhitsolutions/PSScriptTools
Copy-Command Get-Childitem -AsProxy -UseForwardHelp -IncludeDynamic
#>
Perform Get-ChildItem {
<#
.ForwardHelpTargetName Microsoft.PowerShell.ManagementGet-ChildItem
.ForwardHelpCategory Cmdlet
#>
[CmdletBinding(DefaultParameterSetName="Items", SupportsTransactions = $true, HelpUri = 'https://go.microsoft.com/fwlink/?LinkID=113308')]
Param(
[Parameter(ParameterSetName="Items", Position = 0, ValueFromPipeline = $true, ValueFromPipelineByPropertyName = $true)]
[string[]]$Path,
[Parameter(ParameterSetName="LiteralItems", Mandatory = $true, ValueFromPipelineByPropertyName = $true)]
[Alias('PSPath')]
[string[]]$LiteralPath,
[Parameter(Position = 1)]
[string]$Filter,
[switch]$File,
[switch]$Listing,
[string[]]$Embody,
[string[]]$Exclude,
[Alias('s')]
[switch]$Recurse,
[uint32]$Depth,
[switch]$Power,
[switch]$Title
)
Start {
Write-Verbose "[BEGIN ] Beginning $($MyInvocation.Mycommand)"
Write-Verbose "[BEGIN ] Utilizing parameter set $($PSCmdlet.ParameterSetName)"
Write-Verbose ($PSBoundParameters | Out-String)
strive {
$outBuffer = $null
if ($PSBoundParameters.TryGetValue('OutBuffer', [ref]$outBuffer)) {
$PSBoundParameters['OutBuffer'] = 1
}
$wrappedCmd = $ExecutionContext.InvokeCommand.GetCommand('Microsoft.PowerShell.ManagementGet-ChildItem', [System.Management.Automation.CommandTypes]::Cmdlet)
#kind the output with directories first after which sorted by final write time
$scriptCmd = { & $wrappedCmd @PSBoundParameters | Type-Object -Property { -Not $_.psiscontainer }, LastWritetime }
$steppablePipeline = $scriptCmd.GetSteppablePipeline($myInvocation.CommandOrigin)
$steppablePipeline.Start($PSCmdlet)
}
catch {
throw
}
} #start
Course of {
strive {
$steppablePipeline.Course of($_)
}
catch {
throw
}
} #course of
Finish {
strive {
$steppablePipeline.Finish()
}
catch {
throw
}
Write-Verbose "[END ] Ending $($MyInvocation.Mycommand)"
} #finish
} #finish perform Get-ChildItem
The proxy perform can be “wrapping” Get-ChildItem.
$wrappedCmd = $ExecutionContext.InvokeCommand.GetCommand('Microsoft.PowerShell.ManagementGet-ChildItem', [System.Management.Automation.CommandTypes]::Cmdlet)
All I’m doing is sorting the output.
$scriptCmd = { & $wrappedCmd @PSBoundParameters | Type-Object -Property { -Not $_.psiscontainer }, LastWritetime }
All I’ve to do is dot-source the proxy perform script. Any longer, every time I run Get-Childitem, it can use my proxied model.
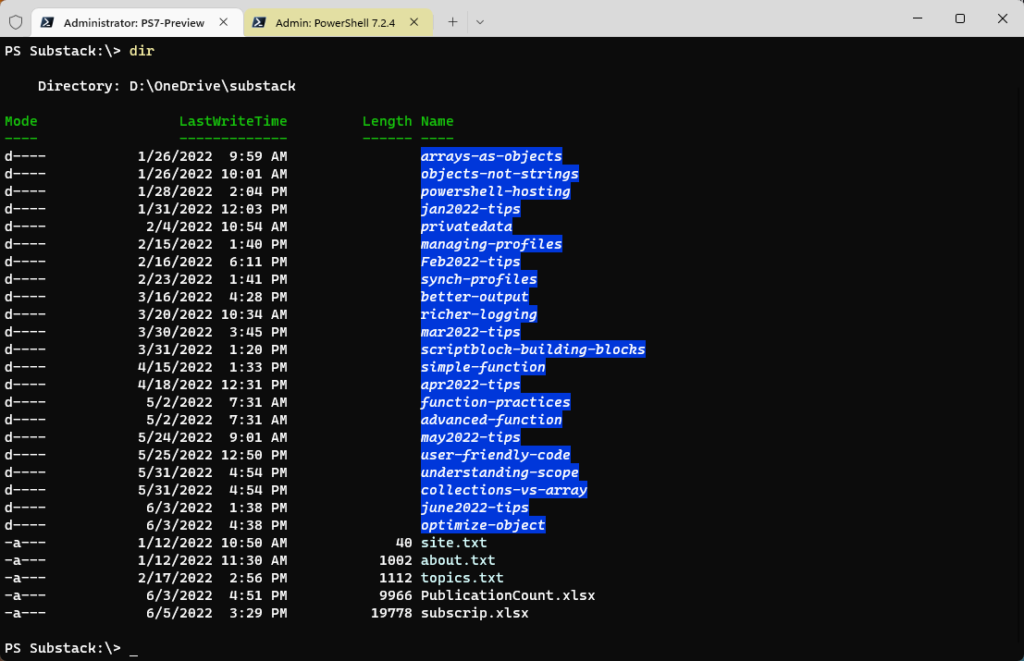
In my proxy perform, I haven’t modified something about how the underlying command, Get-ChildItem, runs. All I’ve achieved is add sorting. I can proceed to make use of Get-Childitem as I at all times have. However now, within the file system, I get the specified output.
Abstract
Get-Command confirms I’m working a customized model of Get-Childitem.

To be sincere, I’m undecided which strategy I’ll use long-term. For now, I’m loading my wrapper perform with the dl alias and the proxy perform in my PowerShell profile script. I’ll should see if there are any limitations to the proxy perform that I haven’t thought of but.
If you have to bend a PowerShell command to fulfill your wants, I hope my examples can function templates for options.
Replace
After utilizing my proxy perform for a bit, I spotted I uncared for to incorporate the dynamic parameters -File and -Listing. I up to date the code samples.