Reverse engineer the Firebase iOS SDK
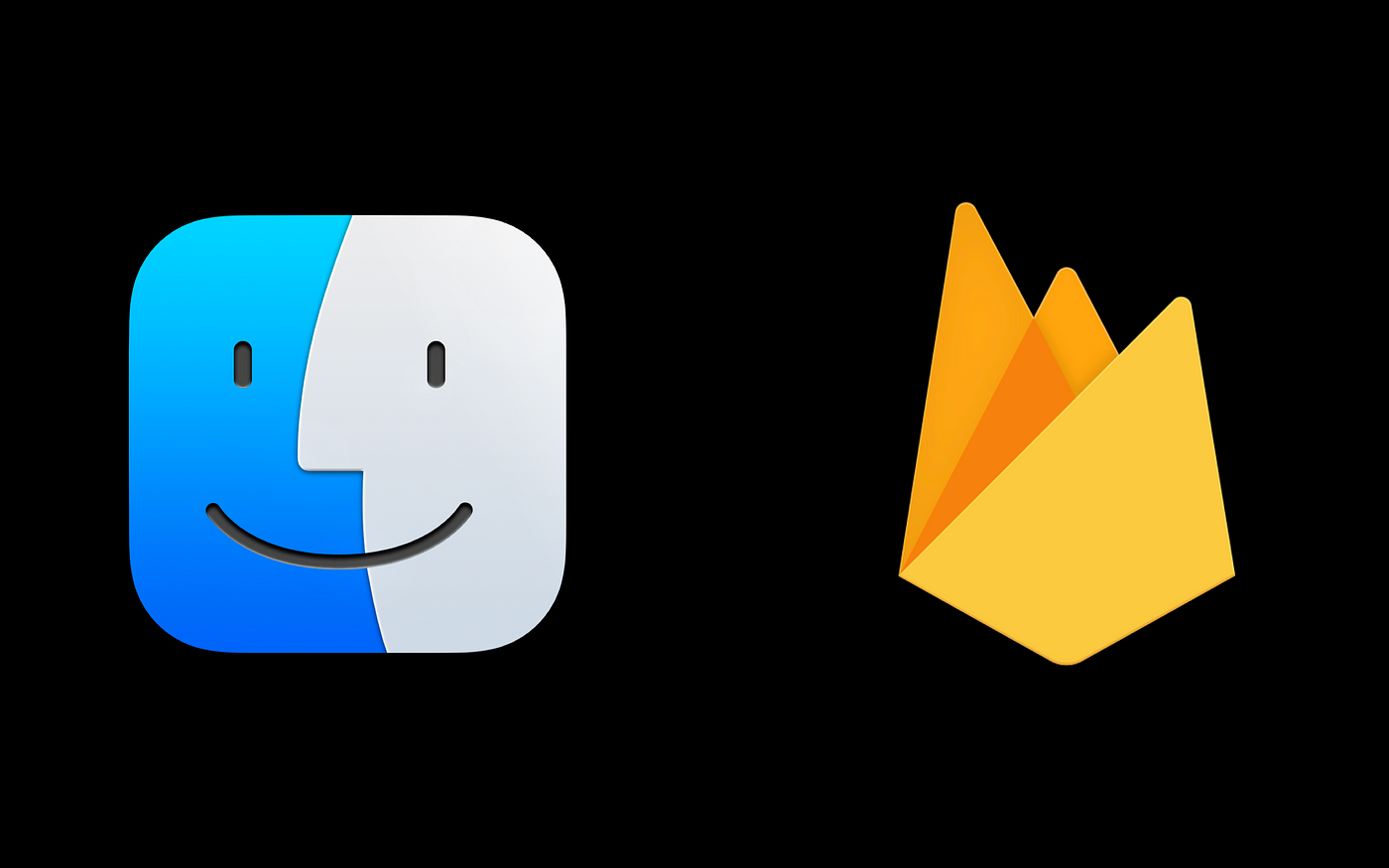
In accordance with their documentation, Firebase Dynamic Hyperlinks are solely supported on iOS. I’ll present you ways I added macOS assist for Dynamic Hyperlinks by reverse-engineering the Firebase iOS SDK.
I used to be fortunately constructing my iOS and macOS cross-platform utility utilizing Firebase till I realized that Firebase Dynamic Hyperlinks solely labored on iOS. I wished to combine on-line periods into my app, and I used Dynamic Hyperlinks as a manner for different customers to affix in a session.
So discovering out Dynamic Hyperlinks weren’t supported on macOS was a disappointment. However I needed to confirm this.

The proof is in Firebase’s Package deal.swift: the Dynamic Hyperlinks goal is simply supported for iOS. Bummer!
However that bought me pondering… why? The core options of Firebase Dynamic Hyperlinks library within the SDK are
- making a Dynamic Hyperlink from the deep hyperlink
- including fallback URL’s, social parameters, minimal variations, and different specifiers to the Dynamic Hyperlink
- shortening the Dynamic Hyperlink
- and dealing with the finished hyperlink.
Clearly, it appears Firebase ought to be dealing with these items. And we belief what they develop of their SDK; absolutely it should work!
However what’s stopping us from making our personal API to get across the macOS challenge? A number of URL requests from a number of Firebase endpoints; some handbook URL parsing and creation… this isn’t too unhealthy!
However earlier than we dive into the code, let’s stand up to hurry on how Dynamic Hyperlinks work.
OK, so that you’ve learn the Dynamic Hyperlinks documentation earlier than, however possibly it’s time for a fast refresher on the related vocabulary. Jargon like “deep hyperlink”, “common hyperlink”, “fallback URL”, and so forth. can get complicated. So, let’s make clear what Google means.

- Within the cellular context, A deep hyperlink makes use of a uniform useful resource identifier (URI) that hyperlinks to a particular location inside a cellular app reasonably than merely launching the app. It may be used to open, as an illustration, the App Retailer in case your app just isn’t put in.
- A Dynamic Hyperlink is a hyperlink hosted and managed by Firebase. It carries details about your app (like bundle ID, and app retailer ID), social meta parameters, and extra. The neat promoting characteristic of Dynamic Hyperlinks is cross-platform compatibility with many platforms, like iOS, Android, Flutter, and so forth. The payload of your Dynamic Hyperlink is your deep hyperlink.
- A common hyperlink is a hyperlink that might be your Dynamic Hyperlink and is dealt with by your app. Firebase will try to resolve a common hyperlink as a Dynamic Hyperlink so it could possibly provide the actual payload: the deep hyperlink.
Dynamic Hyperlinks are generated with a URL Prefix. In Firebase, by default, your URL prefix is generated to be your-firebase-project.web page.hyperlink
.
In the event you use a customized area, you’ll be able to create a URL prefix, corresponding tomy-website.com/hyperlink
.
- A brief Dynamic Hyperlink is a shortened model of your dynamic hyperlink. Like the conventional Dynamic Hyperlink, it begins together with your URL prefix. Nevertheless, the question parameters within the Dynamic Hyperlink are shortened to a random string of 4 (or extra) characters.
These varied hyperlink sorts appear fantastic. Let’s now evaluation how dynamic hyperlinks work in motion:
Creating Hyperlinks
- First, we have to construct our deep hyperlink.
- Seize your web site’s area. For instance, I’ll use
app.com
. - Select a path on your content material. I wish to hyperlink to my person mannequin, so my path will likely be
/person
. Paths are non-compulsory, and you may get inventive as a lot as you’d prefer to customise in-app dealing with. - Add question parameters for further in-app dealing with. As an example, I take advantage of
?id=ABCDEFG
to specify that I will likely be fetching the person with ID “ABCDEFG”. - You now have your deep hyperlink payload. In my instance, that is
https://app.com/person?id=ABCDEFG
. - Subsequent, seize your Area URL Prefix. I’ll use
app.web page.hyperlink
. - From right here, you employ Firebase iOS SDK to create a “lengthy” Dynamic Hyperlink with extra parameters, just like the minimal app model wanted, or your app’s App Retailer ID:
guard let hyperlink = URL(string: "https://app.com/person?id=ABCDEFG") else { return }
let dynamicLinksDomainURIPrefix = "https://app.web page.hyperlink"
let linkBuilder = DynamicLinkComponents(hyperlink: hyperlink, domainURIPrefix: dynamicLinksDomainURIPRefix)
linkBuilder.iOSParameters = DynamicLinkIOSParameters(bundleID: "com.instance.ios")
linkBuilder.androidParameters = DynamicLinkAndroidParameters(packageName: "com.instance.android")guard let longDynamicLink = linkBuilder.url else { return }
print("The lengthy URL is: (longDynamicLink)")
8. Most of the time, your lengthy Dynamic Hyperlink will likely be… lengthy… and also you’ll must shorten it:
DynamicLinkComponents.shortenURL(url: longDynamicLink, choices: nil) { url, warnings, error in
guard let url = url, error != nil else { return }
print("The quick URL is: (url)")
}
9. As soon as shortened, you’ll have your shareable Dynamic Hyperlink. Mine appears to be like like https://app.web page.hyperlink/ABCD
.
Dealing with Hyperlinks
- OK, so we’ve opened the URL in our app. In SwiftUI, this appears to be like like:
MyView()
.onOpenURL { url in
// Deal with common url right here.
}
2. We use the SDK to deal with the (common) URL to resolve the Dynamic Hyperlink:
DynamicLinks.dynamicLinks().handleUniversalLink(url)
{ dynamicLink, error in
// Dynamic hyperlink handed right here
}
3. We get hold of the deep hyperlink URL from the Dynamic Hyperlink:
let deepURL: URL? = dynamicLink?.url
4. That deep hyperlink comprises our payload as described earlier. As an example, I can open a person’s profile web page:
// deepURL = "https://app.com/person?id=ABCDEFG"if deepURL.path == "/person" {
let elements = URLComponents(string: deepURL.absoluteString)
let userID = elements?.queryItems.first?.worth
// Deal with opening person ID right here
}
Increase. We’re completed. Hyperlinks created and dealt with.
However none of this works on macOS. That’s an issue, as I need to have the ability to on the very least create hyperlinks from my desktop app (or every other Swift-running platform).
So, let’s see if we are able to choose aside firebase-ios-sdk
, and the Firebase documentation, to perform our aim.
Let’s begin with creating hyperlinks. The very best place to begin is Firebase’s Dynamic Hyperlink documentation. Usually, we’d head over to the iOS part. Nevertheless, do you discover one other part within the documentation that would assist us with bringing Dynamic Hyperlinks over to macOS?

It’s Handbook URL Development. I discovered this web page whereas exploring the docs, and it’s what initially gave me the hope that this endeavor can be potential on macOS.

Utilizing the standard URL prefix and question parameters, we are able to embed our deep hyperlink payload (?hyperlink=
), and all of our metadata (i.e. &apn=
, &amv=
, &afl=
), all regionally:
var builder = URLComponents()
builder.scheme = "https"
builder.host = "app.web page.hyperlink"
builder.queryItems = [
.init(name: "link", value: "https://app.com/user?id=ABCDEFG"),
.init(name: "ibi", value: "com.myapp.bundleid"),
// add other items, like "isi", "imv", "ofl", "st", "sd"...
]
let longDynamicLink: URL? = builder.url
Swift’s URLComponents
will gloriously convert this right into a properly-formatted URL, which is our lengthy Dynamic Hyperlink.
Now, it’s time to shorten our lengthy Dynamic Hyperlink. That is the place doing REST work ourselves will actually shine.
We all know the shorten technique offered by the SDK has an escaping completion handler, that means one thing backend-related should be happening.
In reality, that’s precisely the case: a POST request is known as. The request comprises details about the lengthy Dynamic Hyperlink and the specified shortened hyperlink of the brand new URL.
Leaping to the definition of Firebase SDK’s shortenURL
technique, we are able to see a URL request is made on line 518:

Digging deeper, we see that the “shortening request” is definitely a long-winded POST request… referred to as it!
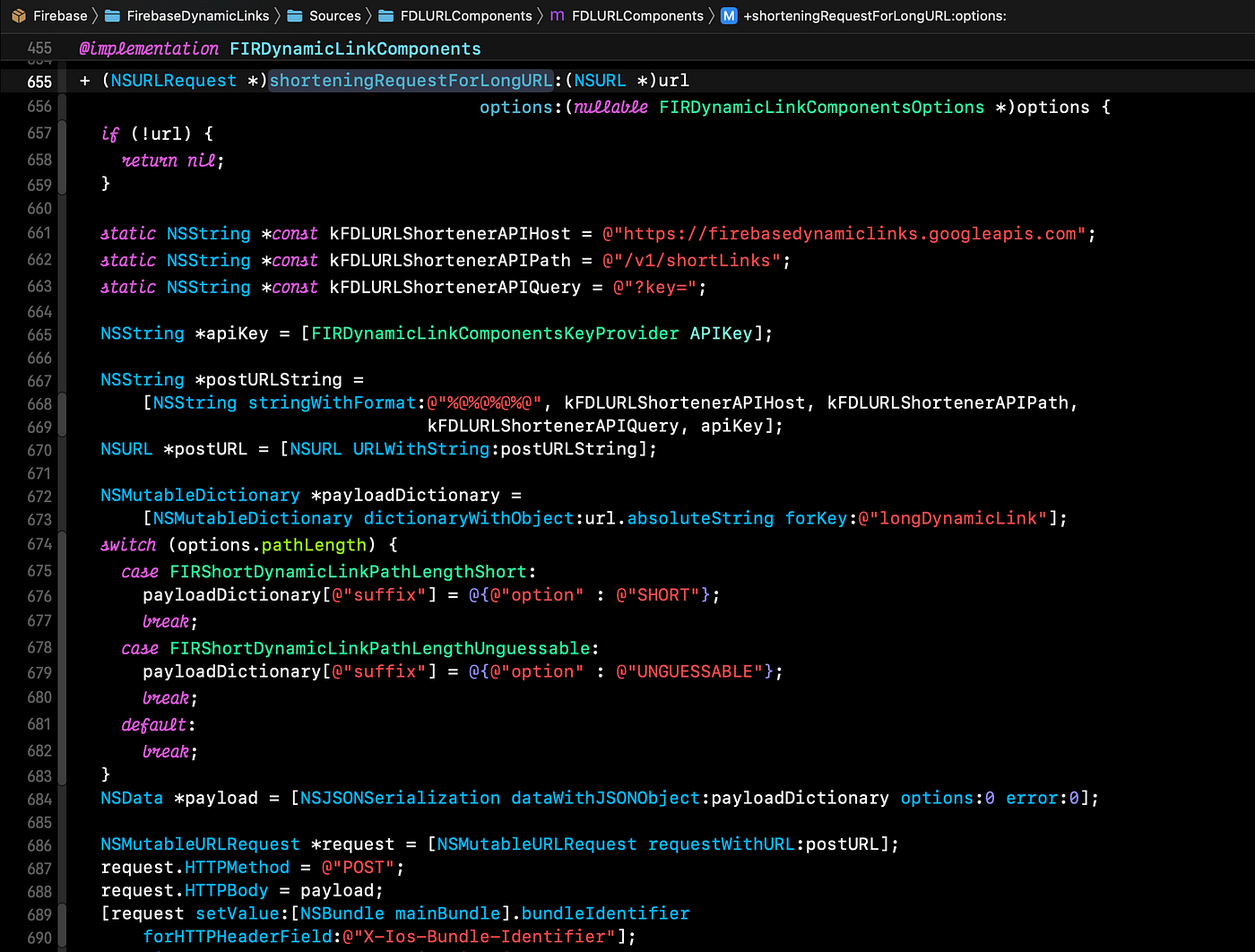
Exploring this technique offers us some key info:
{
"longDynamicLink": longDynamicLink,
"suffix": ["option": "SHORT"]
}
- And, after all, the strategy is POST with JSON content material sort.
We’ve completed our digging, so now it’s time to cheat and take a look at the docs. Heading over to the REST part in Create Dynamic Hyperlinks reveals us the right way to shorten a protracted Dynamic Hyperlink by way of REST:
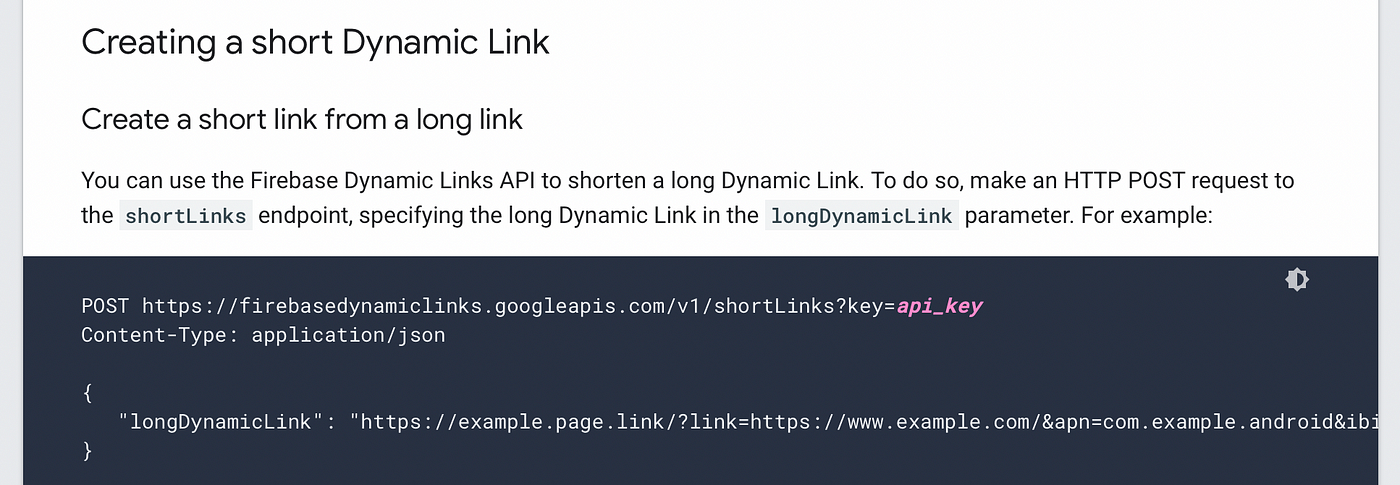
Groovy! This matches what we simply arrange.
Sadly, that is so far as the documentation will take us. On the subject of dealing with URLs on our gadgets, there is no such thing as a details about utilizing URL requests to resolve a Deep Hyperlink, or every other info, from a shortened Dynamic Hyperlink.
The excellent news? We all know the right way to dig by way of the SDK. Let’s examine DynamicLinks.dynamicLinks().handleUniversalLink(_:)
and see what we are able to discover:
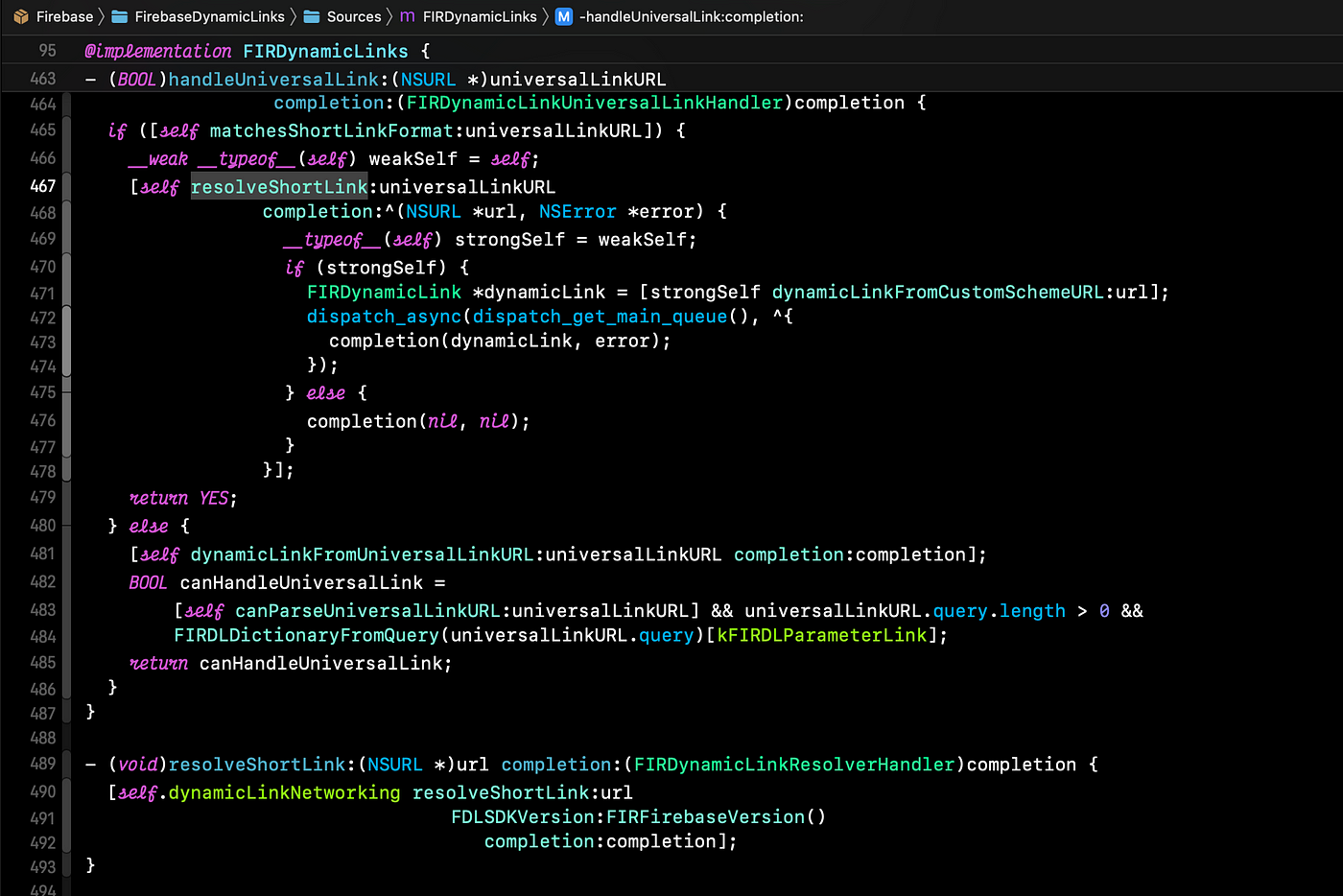
It appears to be like like handleUniversalLink
calls one other technique, resolveShortLink
, on line 467. Leaping to the definition of resolveShortLink
on line 490, we collect extra intel:
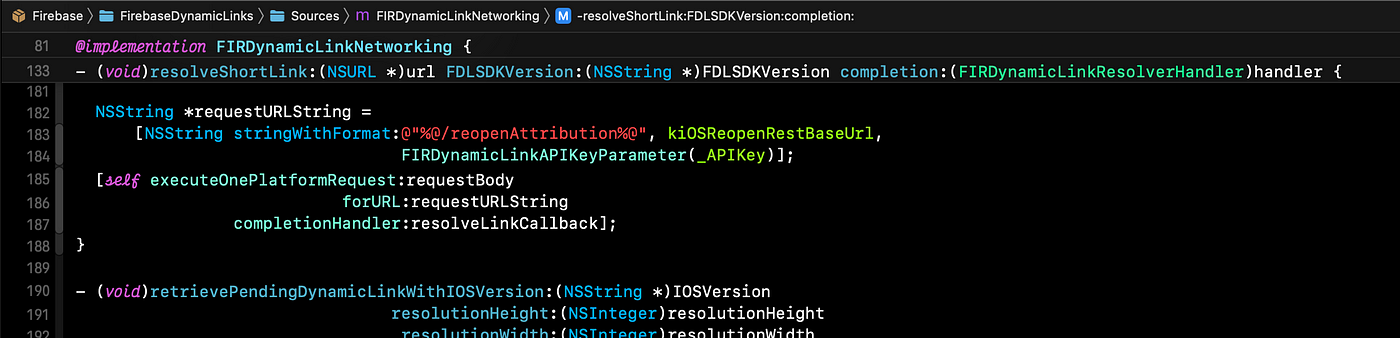
Discover that inspecting “/reopenAttribution”, kiOSReopenRestBaseUrl
, and FIRDynamicLinkAPIKeyParameter(_APIKey)
are all used to kind the fundamental API endpoint wanted to deal with our Dynamic Hyperlink:
As for the POST request physique, let’s scroll down a bit:

We are able to see the POST physique is within the kind:
{
"requestedLink": url.absoluteString,
"bundle_id": "com.app.bundleid",
"sdk_version": "9.0.0"
}
And the callback JSON from the URL request gives us with the deep hyperlink URL:
URLSession.shared.dataTask(with: request) { knowledge, response, error in
guard
let knowledge = knowledge,
let dict = attempt? JSONSerialization.jsonObject(with: knowledge) as? [String: Any],
let deepLink = dict["deepLink"] as? String,
let url = URL(string: deepLink)
else {
completion(nil)
return
}
// Deal with your deep hyperlink URL right here
}.resume()
In fact, on macOS, the choices for dealing with opening URLs in your app are restricted, so here’s a fast workaround suggestion:
- Equip your advertising and marketing web site to open a fallback URL based mostly in your Deep Hyperlink URL if the person is on a macOS system. Right here, the fallback URL will include payload info like a person ID.
- Use JavaScript to deal with the URL and put together a customized URL scheme to be opened. A URL scheme is one thing that native apps working on macOS can deal with, like
my app://additional-information
. - Deal with the URL scheme in-app, immediately accessing the payload knowledge and carrying about your corporation.
In conclusion, it’s essential to do not forget that as builders, it’s as much as us to unravel our personal issues and carve out a path for ourselves, and our friends.
Giving macOS Dynamic Hyperlinks assist as soon as appeared tough, however by way of persistence and just a little digging, we managed to beat the issue.
Retaining a constructive mindset about your studying and analysis means as a developer is vital for having the boldness to sort out tough issues. So, keep affected person and check out your greatest! You’ll be taught extra that manner in any case.
In the event you’ve been studying my articles, you’ll know I’m engaged on EasyFirebase, a Swift Package deal that makes Firebase a lot simpler to make use of. Dynamic Hyperlinks are supported for iOS and macOS within the bundle out-of-the-box, so that you needn’t implement this all your self for those who use my bundle.
To get began, you’ll be able to create hyperlinks like this:
EasyLink.urlPrefix = "https://app.web page.hyperlink"var hyperlink = EasyLink(host: "app.com", path: "/person", question: ("id", "ABCDEFG"))
Then shorten it like this:
hyperlink.shorten { url in
// ...
}
And deal with it like this:
EasyLink.deal with(url) { easyLink in
guard let easyLink = easyLink else { return }
if easyLink.path == "/person" {
if let id = easyLink.question["id"] {
// Deal with person ID right here.
}
}
}