Abstract: You possibly can break up a string utilizing an empty separator utilizing –
(i) listing constructor
(ii) map+lambda
(iii) regex
(iv) listing comprehension
Minimal Instance:
textual content="12345" # Utilizing listing() print(listing(textual content)) # Utilizing map+lambda print(listing(map(lambda c: c, textual content))) # Utilizing listing comprehension print([x for x in text]) # Utilizing regex import re # Strategy 1 print([x for x in re.split('', text) if x != '']) # Strategy 2 print(re.findall('.', textual content))
Drawback Formulation
📜Drawback: Find out how to break up a string utilizing an empty string as a separator?
Instance: Think about the next snippet –
a="abcd" print(a.break up(''))
Output:
Traceback (most up-to-date name final): File "C:UsersSHUBHAM SAYONPycharmProjectsFinxterBlogsFinxter.py", line 2, in <module> a.break up('') ValueError: empty separator
Anticipated Output:
['a', 'b', 'c', 'd']
So, this basically signifies that once you attempt to break up a string through the use of an empty string because the separator, you’ll get a ValueError. Thus, your process is to learn how to get rid of this error and break up the string in a method such that every character of the string is individually saved as an merchandise in a listing.
Now that we’ve a transparent image of the issue allow us to dive into the options to resolve the issue.
Methodology 1: Use listing()
Strategy: Use the listing() constructor and go the given string as an argument inside it because the enter, which is able to break up the string into separate characters.
Notice: listing()
creates a brand new listing object that incorporates objects obtained by iterating over the enter iterable. Since a string is an iterable shaped by combining a gaggle of characters, therefore, iterating over it utilizing the listing constructor yields a single character at every iteration which represents particular person objects within the newly shaped listing.
Code:
a="abcd" print(listing(a)) # ['a', 'b', 'c', 'd']
🌎Associated Learn: Python listing() — A Easy Information with Video
Strategy: Use the map()
to execute a sure lambda perform on the given string. All you want to do is to create a lambda perform that merely returns the character handed to it because the enter to the map object. That’s it! Nevertheless, the map methodology will return a map object, so you should convert it to a listing utilizing the listing()
perform.
Code:
a="abcd" print(listing(map(lambda c: c, a))) # ['a', 'b', 'c', 'd']
Strategy: Use a listing comprehension that returns a brand new listing containing every character of the given string as particular person objects.
Code:
a="abcd" print([x for x in a]) # ['a', 'b', 'c', 'd']
🌎Associated Learn: Listing Comprehension in Python — A Useful Illustrated Information
Methodology 4: Utilizing regex
The re.findall(sample, string)
methodology scans string
from left to proper, trying to find all non-overlapping matches of the sample
. It returns a listing of strings within the matching order when scanning the string from left to proper.
🌎Associated Learn: Python re.findall() – All the pieces You Must Know
Strategy: Use the common expression re.findall('.',a)
that finds all characters within the given string ‘a
‘ and stires them in a listing as particular person objects.
Code:
import re a="abcd" print(re.findall('.',a)) # ['a', 'b', 'c', 'd']
Alternatively, it’s also possible to use the break up methodology of the regex library in a listing comprehension which returns every character of the string and eliminates empty strings.
Code:
import re a="abcd" print([x for x in re.split('',a) if x!='']) # ['a', 'b', 'c', 'd']
🌎Associated Learn: Python Regex Break up
Do you need to grasp the regex superpower? Try my new ebook The Smartest Solution to Study Common Expressions in Python with the revolutionary 3-step strategy for energetic studying: (1) examine a ebook chapter, (2) remedy a code puzzle, and (3) watch an academic chapter video.
Conclusion
Hurrah! We now have efficiently solved the given drawback utilizing as many as 4 (5, to be trustworthy) alternative ways. I hope this article helped you and answered your queries. Please subscribe and keep tuned for extra fascinating articles and options sooner or later.
Joyful coding! 🙂
Regex Humor

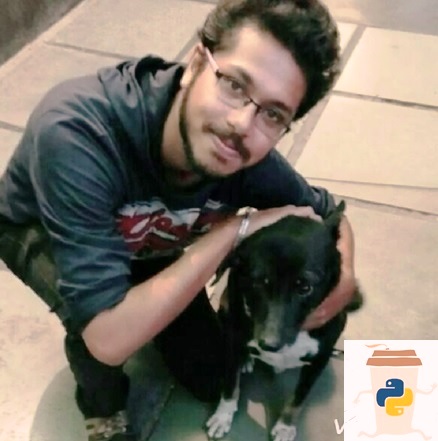
I’m knowledgeable Python Blogger and Content material creator. I’ve revealed quite a few articles and created programs over a time frame. Presently I’m working as a full-time freelancer and I’ve expertise in domains like Python, AWS, DevOps, and Networking.
You possibly can contact me @: