Each state change in React may cause re-rendering which might have an effect on badly on utility.
Largely when the part dimension will get elevated.
useCallback
and useMemo
hooks are used for improvising the efficiency of React utility.
Does useCallback and useMemo do the identical factor?
Although each are used for efficiency optimization,
it could be complicated for builders concerning their use-case.
So let’s perceive them completely.
Earlier than diving deeply into their distinction,
let’s perceive why they’re used.
- As talked about above, each hooks are used for efficiency optimization.
- If our utility is doing frequent re-rendering then primarily based on the use-case we will use one among them.
Let’s see how syntactically completely different they’re:-
useCallback(()=>{
doSomething();
}, [dependencies]);
useMemo(()=>{
doSomething();
}, [dependencies]);
As we will see,
there isn’t any such distinction of their syntax besides of their naming.
Each hooks settle for a perform
and a dependency array.
How is useMemo completely different from useCallback?
The primary distinction between useMemo
and useCallback hook is,
useMemo
returns memoized worth and
useCallback
returns memoised perform.
Nonetheless confused?
No downside. We’ll perceive the distinction by contemplating one instance.
Let’s say we’ve one guardian part,
import { React, useState } from 'react';
import ChildComponent from './ChildComponent'
perform App() {
const [num, setNum] = useState(0);
return(
<div>
<h1>{num}</h1>
<ChildComponent />
<button onClick={() => setNum(num + 1)}> Addition </button>
</div>
);
}
and a toddler part
import { React } from 'react';
perform ChildComponent() {
console.log("little one part rendered")
return(
<div>
<h1>Good day World</h1>
</div>
);
}
export default ChildComponent;
We will observe that the console assertion –
little one part rendered
is getting printed each time we click on on the Addition
button.
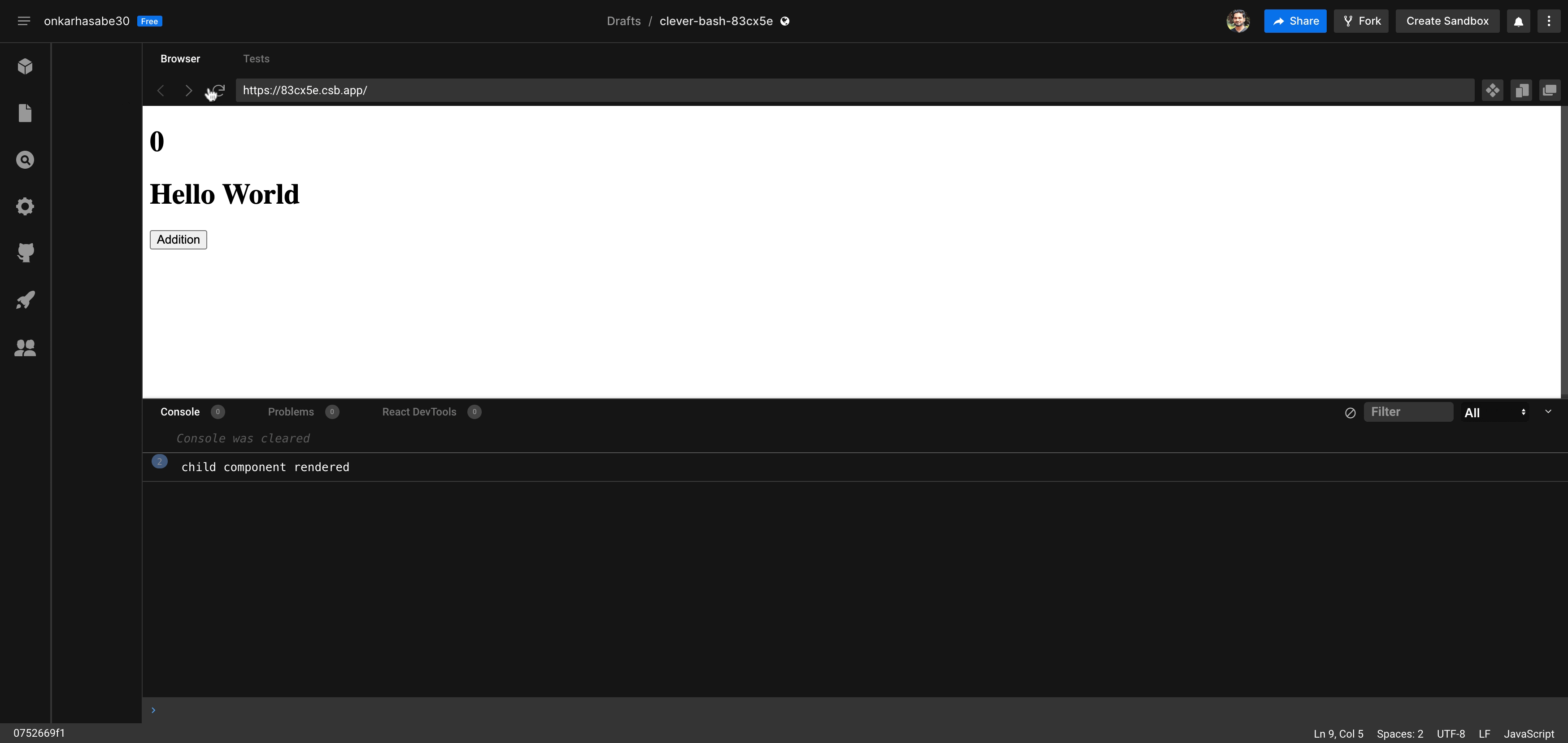
Although the state change is accuring in guardian part solely,
the kid part is re-rendering unnecessarily.
Let’s take a look on how we will keep away from that.
Understanding useMemo
On this state of affairs useMemo
can provide us memoized consequence.
Simply name the kid part by wrapping in useMemo
.
So, the guardian part will appear to be:
import { React, useState, useMemo } from 'react';
import ChildComponent from './ChildComponent'
perform App() {
const [num, setNum] = useState(0);
const getChildComp = useMemo(() => <ChildComponent />, []);
return(
<div>
<h1>{num}</h1>
{getChildComp}
<button onClick={() => setNum(num + 1)}> Addition </button>
</div>
);
}
We will observe right here that,
the console assertion isn’t getting printed as we click on on Addition
button
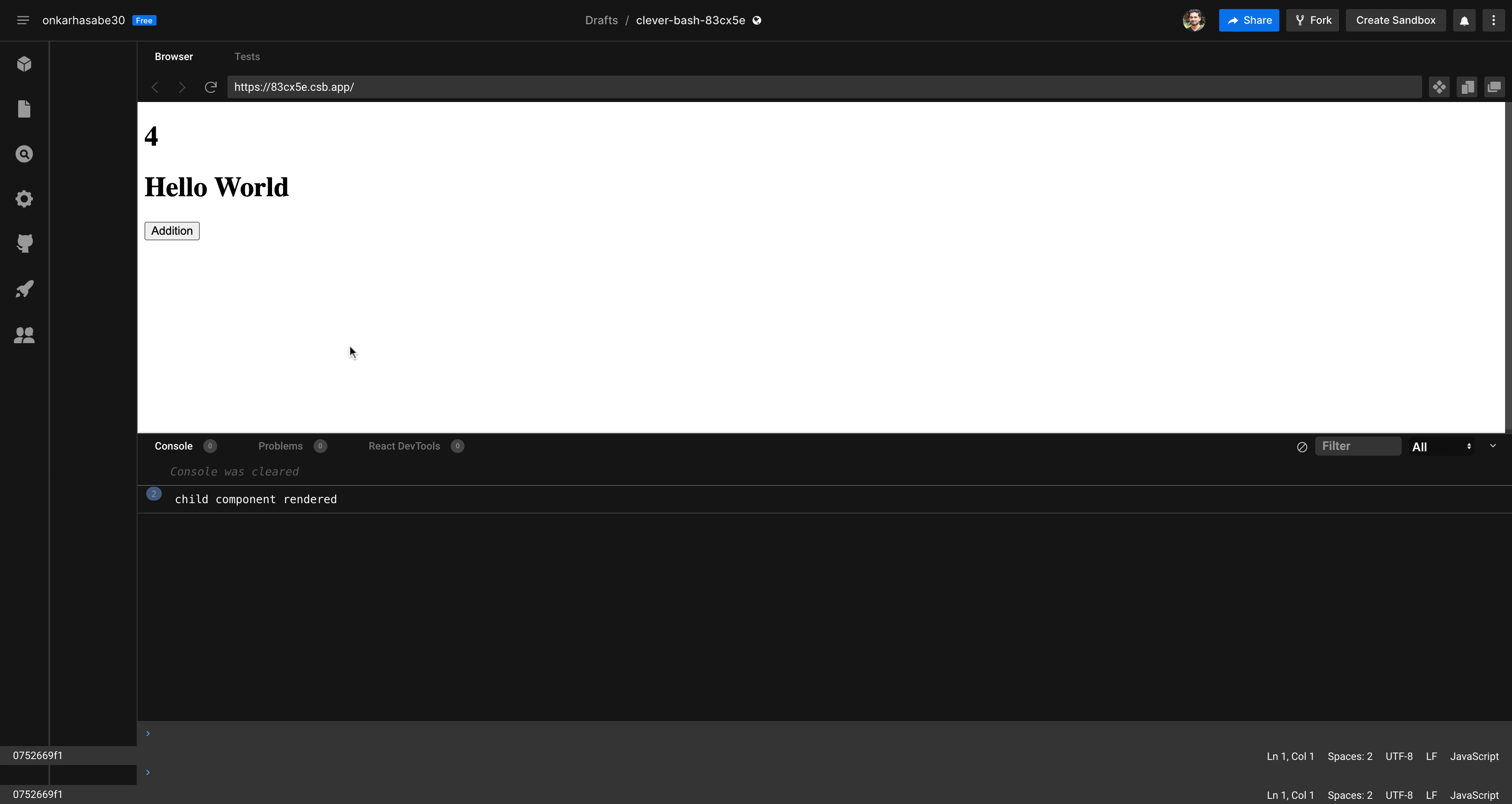
Thus useMemo
helped us in rising efficiency on this state of affairs.
Now, let’s see how useCallback
works.
Understanding useCallback
Simply create a perform within the guardian part and cross it to the kid part.
In order that the guardian part will appear to be,
import { React, useState } from 'react';
import ChildComponent from './ChildComponent'
perform App() {
const [num, setNum] = useState(0);
const handleUpdateNum = () => {
//some code
};
const getChildComp = useMemo(
() => <ChildComponent handleUpdateNum={handleUpdateNum} />,
[handleUpdateNum]
);
return(
<div>
<h1>{num}</h1>
{getChildComp}
<button onClick={() => setNum(num + 1)}> Addition </button>
</div>
);
}
Now, if we attempt to click on on the Addition
button,
we will see little one part rendered
on a console.
So, useMemo
gained’t assist out on this state of affairs.
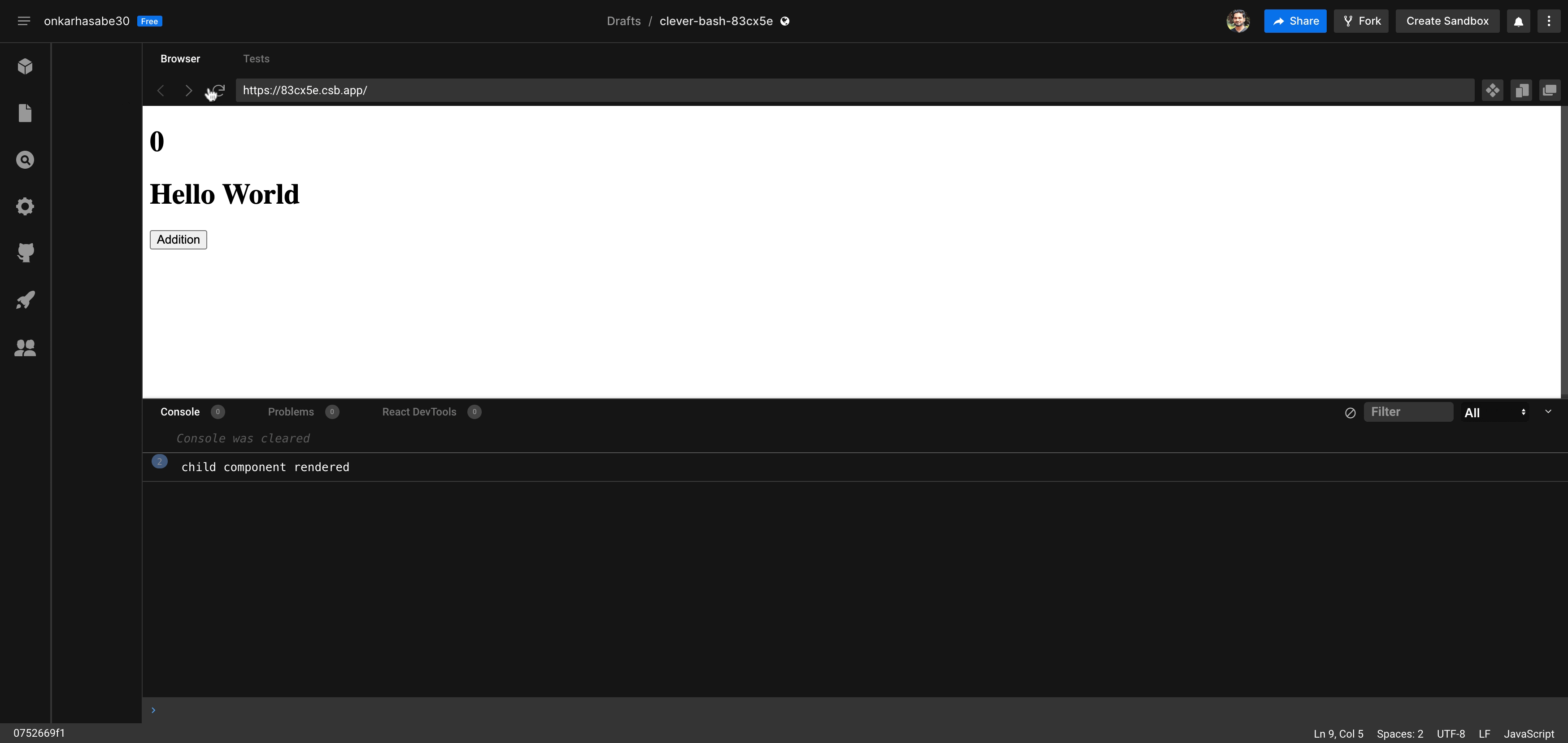
As a result of each time the guardian part will get re-rendered,
handleUpdateNum
perform received re-created.
There useCallback
comes into the image which returns us memoized perform.
Let’s wrap the handleUpdateNum perform by useCallback
hook and see the consequence.
So the code in guardian part will appear to be this,
import { React, useState, useCallback } from 'react';
import ChildComponent from './ChildComponent'
perform App() {
const [num, setNum] = useState(0);
const handleUpdateNum = useCallback(() => {
//some code
}, []);
const getChildComp = useMemo(
() => <ChildComponent handleUpdateNum={handleUpdateNum} />,
[handleUpdateNum]
);
return(
<div>
<h1>{num}</h1>
{getChildComp}
<button onClick={() => setNum(num + 1)}> Addition </button>
</div>
);
}
Now we will see, even after the change within the guardian part’s state,
the kid part isn’t re-rendering.
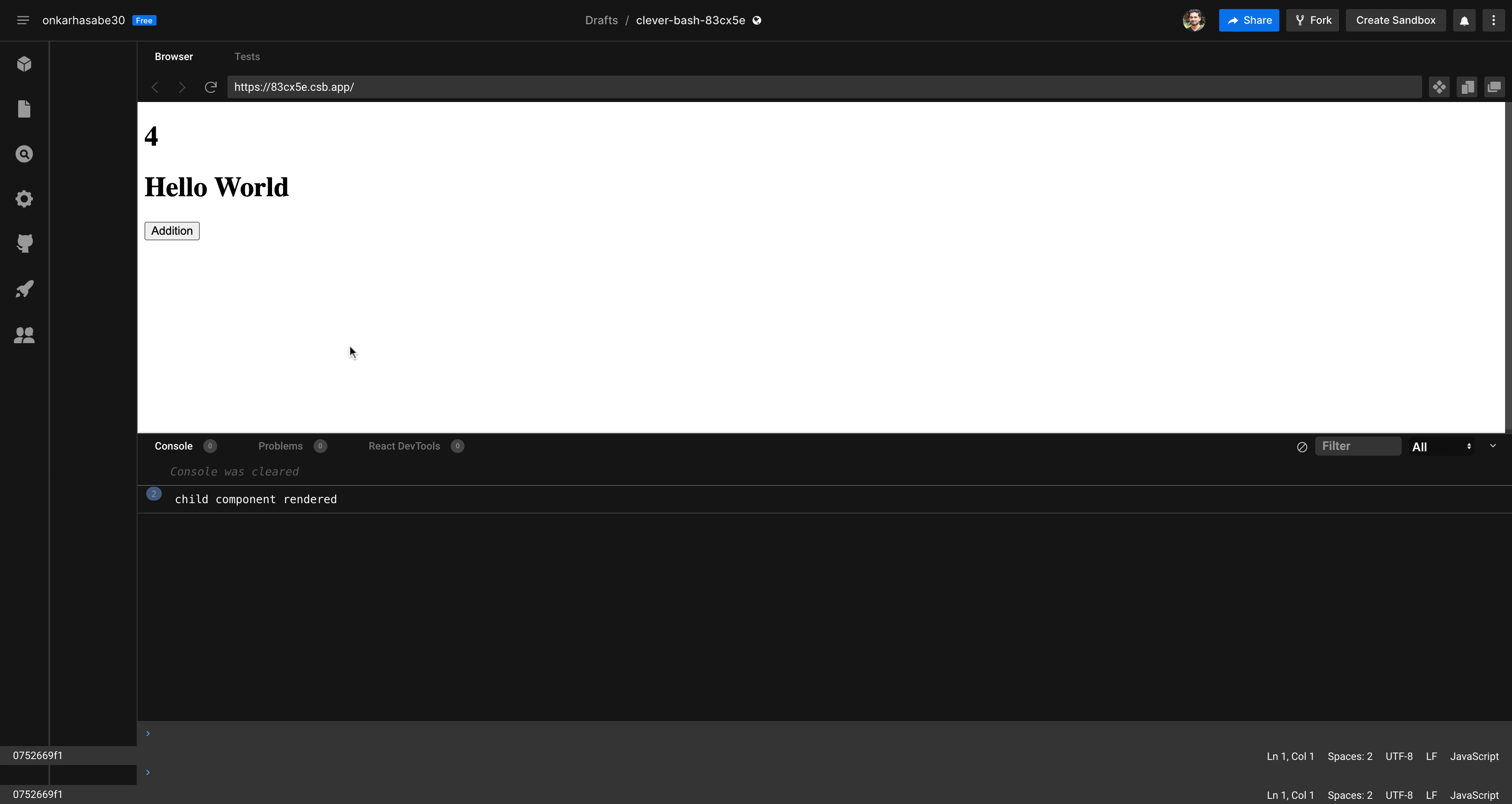
Conclusion
Although we’ve explored useCallback
and useMemo
,
we will make use of those hooks as per our requirement.
Simply take into account these hooks as fine-tuning in React.
It could’t repair badly written code but when every thing is up to speed,
these hooks will present their further advantages.
For reference, please use
this hyperlink.