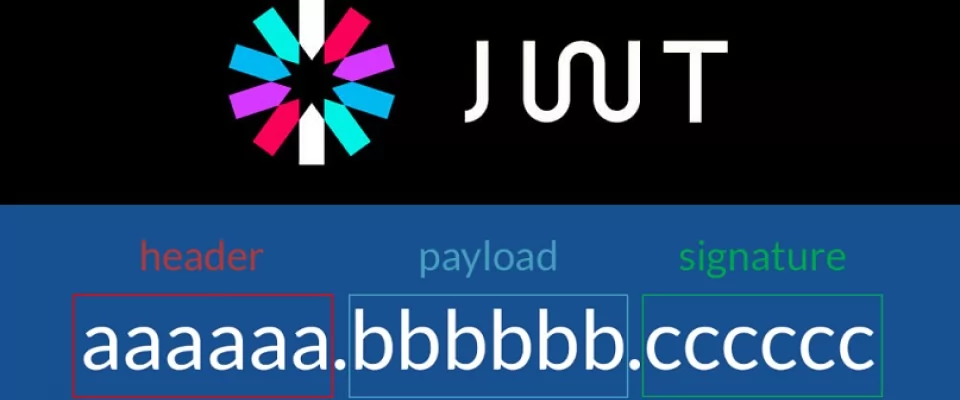
JWT tokens are a typical used to create utility entry tokens, enabling person authentication in net purposes. Particularly, it follows the RFC 7519 commonplace.
What’s a JWT token
A JWT token is a token that offers the person entry to an utility by certifying their identification. This token is returned to the shopper, which is often the person’s browser, sending the token again to the server with every successive request. On this approach, the server is aware of whether or not or not the request comes from a particular person.
The sort of design is quite common when we’ve got a frontend utility that accesses the server via a REST API or GraphQL. The person will authenticate by sending their entry information to the server and the server will create and return a JWT token if the entry information is right. It will be important to not confuse authentication with authorisation, as they’re two completely different processes. The token shall be saved within the person’s browser, for which a cookie is commonly used.
When utilizing a JWT token you’ll need to make use of a safe HTTPS connection. It’s because the tokens are usually not encrypted, they’re merely cryptographically signed by the server. In concept, utilizing a safe connection, no person will have the ability to intercept or modify the token because it travels from its supply to its vacation spot and vice versa.
Find out how to generate a JWT token
The strategy used to generate a JWT token varies relying on the programming language and the features or libraries used for this function. Under we are going to see the best way to generate a JWT token with each JavaScript and PHP.
JWT token with JavaScript
Let’s examine the best way to generate a JWT token with Node.js. The one factor you’ll need is to have Node.js put in in your system and initialize any venture.
Then it’s essential to use the next code, the place it’s essential to create a JSON object wherein the HMAC SHA256 algorithm is ready because the encryption algorithm, though you possibly can use some other that’s supported. Then we generate a Buffer from the article and encode it as a base64 string:
const header = { "alg": "HS256", "typ": "JWT" }; const codedHeader = Buffer.from(JSON.stringify(header)).toString('base64');
You should then add the person’s information, no matter components determine the person. This might be the person’s system person identify or identifier. You possibly can add as a lot information as you contemplate obligatory. The one exception to the weather of the article containing the info are the iss
and exp
keys, that are key phrases that you just won’t be able so as to add and that serve to determine the issuer of the token and its expiry date.
We can even should convert the article right into a Buffer and encode it as a base64 string. Under you may see an instance:
const information = { id: 'id_user' }; const codedData = Buffer.from(JSON.stringify(information)).toString('base64');
Subsequent, we’re going to import the crypto module from Node.js and arrange a secret key to signal the token. You should utilize some other library to do that. Then we are going to generate the signature from the header codedHeader
and the person information information codedData
. We are going to use the signature and the key key to generate a base64 illustration of the encrypted signature.
const crypto = require('crypto'); const secretKey = 'secret_key'; const signature = crypto.createHmac('sha256', secretKey).replace(codedHeader + '.' + codedData).digest('base64');
This prevents the content material from being modified, as this is able to invalidate the signature.
Now we solely must concatenate the encoded header, the encoded information and the signature to generate the JWT token. For this we use a template literal. The completely different components shall be separated by a dot:
const tokenJWT = `${codedHeader}.${codedData}.${firma}`;
JWT token with PHP
Let’s examine the best way to create a JWT authentication token with PHP. For this we might use an current package deal or create the token from scratch. Let us take a look at each circumstances.
To create the token manually, we first should create the JSON object that can include the token header, setting the HMAC SHA256 algorithm because the encryption algorithm, though you possibly can use some other that’s supported. Using typ and alg names is a part of the usual. We are going to then encode the article as a base64 string:
$header = json_encode(['typ' => 'JWT', 'alg' => 'HS256']); $codedHeader = str_replace(['+', '/', '='], ['-', '_', ''], base64_encode($header));
Then we should add the person’s identification information in a JSON object, with the ability to add any key besides the reserved phrases iss
and exp
, used to determine the token issuer and expiry date respectively. We can even should generate a string in base64 format:
$information = json_encode(['id' => 'user_id']); $CodedData = Buffer.from(JSON.stringify(information)).toString('base64');
Subsequent we should create the signature, encrypting the header, the info and the key key with the sha256
algorithm, for which we are going to use the PHP hash_hmac
perform. As well as, we should additionally encode the signature as a base64 string:
$secretKey = 'secret_key'; $signature = hash_hmac('sha256', $CodedHeader. '.' . $codedData, $secretKey, true); $codedSignature = str_replace(['+', '/', '='], ['-', '_', ''], base64_encode($firma));
Lastly, we are going to create the JWT token by becoming a member of the encrypted header, the encrypted information and the encrypted and encrypted signature:
$tokenJWT = $codedHeader . '.' . $codedData . '.' . $codedSignature;
With this we’d have already created the JWT token, though there are different sooner strategies by which we will additionally generate the token.
For instance, we will use one of many many packages that facilitate the creation of JWT tokens. Particularly, we will use the ReallySimpleJWT library, which you’ll be able to set up through composer:
composer require rbdwllr/reallysimplejwt
To create a JWT token within the easiest way, we merely use the create
methodology of the ReallySimpleJWTToken
class, which we are going to use to create the token.
This methodology receives as parameters the person’s identifier, which might be their e-mail, their username or some other information that identifies them. As a second parameter it accepts a secret key that we should configure and, as a 3rd and fourth parameter it accepts the expiry date of the token and the issuer of the token respectively, these final two parameters being elective:
use ReallySimpleJWTToken; $idUser="id_user"; $secretKey = 'secret_key'; $expiration = time() + 3600; $emitter="localhost"; $tokenJWT = Token::create($idUer, $secretKey, $expiration, $emitter);
We might additionally add extra customized information along with the person ID utilizing the customPayload
methodology:
use ReallySimpleJWTToken; $information = [ 'uid' => 'id_user', 'iat' => time(), 'exp' => time() + 3600, 'iss' => 'localhost' ]; $secretKey = 'secret_key'; $token = Token::customPayload($information, $secretKey);
To validate the token when it’s returned by the shopper, we will use the validate
methodology:
use ReallySimpleJWTToken; $consequence = Token::validate($token, $secretKey);
API Authentication with a JWT token
After you have generated a JWT token, you may retailer it in an HttpOnly cookie, which is probably the most safe strategy to keep away from XSS assaults. It’s because JavaScript won’t be able to entry this cookie, being mechanically despatched to the server with every request. The method to be carried out is as follows:
- First the person will ship his entry information to the server.
- The server will generate the JWT token based mostly on this information and retailer it in a cookie.
- In subsequent requests, the shopper will ship the JWT token to the server, thus with the ability to determine itself.
Lastly, in case you wish to retailer session information, it’s strongly advisable that you just use the server periods of a lifetime.
That’s all.
Associated Posts
Template Literals in JavaScript
Template literals, often known as template literals, appeared in JavaScript in its ES6 model, offering a brand new methodology of declaring strings utilizing inverted quotes, providing a number of new and improved prospects.
About…
Find out how to use the endsWith methodology in JavaScript
On this quick tutorial, we’re going to see what the endsWith methodology, launched in JavaScript ES6, is and the way it’s used with strings in JavaScript.
The endsWith methodology is…
Callbacks in JavaScript
Callback features are the identical previous JavaScript features. They haven’t any particular syntax, as they’re merely features which can be handed as an argument to a different perform.
The perform that receives…
Find out how to create PDF with JavaScript and jsPDF
Creating dynamic PDF recordsdata straight within the browser is feasible because of the jsPDF JavaScript library.
Within the final a part of this text we’ve got ready a sensible tutorial the place I…
Node.js and npm: introductory tutorial
On this tutorial we are going to see the best way to set up and use each Node.js and the npm package deal supervisor. As well as, we can even create a small pattern utility. When you…
How to connect with MySQL with Node.js
Let’s examine how one can connect with a MySQL database utilizing Node.js, the favored JavaScript runtime setting.
Earlier than we begin, it is very important notice that it’s essential to have Node.js put in…
JavaScript Programming Types: Greatest Practices
When programming with JavaScript there are particular conventions that it’s best to apply, particularly when working in a staff setting. In reality, it’s common to have conferences to debate requirements…