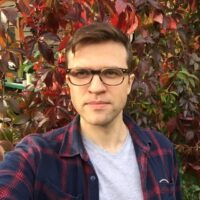
This text was written by an exterior contributor.
Trying to manually create check instances for all doable consumer interactions along with your software program might be daunting, if not unimaginable. Fuzz testing, or fuzzing, is a sort of automated software program testing that may help within the discovery of potential bugs and safety vulnerabilities.
The testing entails injecting random information (or “fuzz”) into the software program being examined. This testing framework might help uncover undefined habits that might result in crashes or different safety points. Whereas it’s not doable to seek out all bugs with fuzz testing, it may be an efficient technique to discover and repair many widespread kinds of points. It’s ceaselessly used to check packages that deal with enter from untrusted sources or which are prone to have surprising inputs.
Fuzz assessments are sometimes automated and can be utilized to check for each practical and safety defects. Purposeful fuzz testing entails feeding invalid information to a program to test for surprising habits. Safety fuzz testing entails feeding malicious information to a program to uncover safety vulnerabilities.
Go has a implausible ecosystem of packages, such because the open supply fuzz testing packages gofuzz and go-fuzz, that are easy to make use of and might be built-in into your testing workflow. Nevertheless, since Go 1.18, native fuzz assist is current within the language, which suggests you not must import any exterior packages. This publish will cowl the benefits and drawbacks of fuzzing, how it may be applied in Go, and completely different fuzzing strategies.
Use Instances of Fuzzing
Fuzzing can be utilized anyplace you might have enter from an untrusted supply, whether or not that’s on the open web or someplace safety delicate. Fuzzing can be utilized to check software program, recordsdata, community insurance policies, purposes, and libraries.
For instance, say you might have an utility that requires the consumer’s identify as enter, and it’s a must to check the applying for invalid information. You may create a check case with fuzzing that makes use of enter with particular characters or values that may result in crashes or end in a reminiscence or buffer overflow.
Different use instances embody:
- Server-client inspection, through which each the shopper and the server are fuzzed to seek out vulnerabilities.
- Packet inspection, through which community sniffers are examined for his or her means to determine vulnerabilities in packet inspection units.
- Testing APIs by operating assessments on every parameter and methodology within the interface to determine weak factors.
This checklist is only a subset of the hundreds of use instances. Something that consumes complicated enter can profit from fuzz testing.
Find out how to Fuzz Check in Go
Let’s take a look at methods to fuzz check a easy utility. To comply with together with this tutorial, you’ll want a working data of Go and a neighborhood set up of the newest steady model of Go. You’ll additionally want a standard or built-in terminal, an surroundings that helps fuzzing (AMD64 or ARM64 structure), and GoLand put in in your machine.
Perform
You need to use fuzz testing on modules, easy capabilities, or something you imagine requires fuzzing. On this tutorial, you’ll use a easy operate that compares two-byte slices and returns false if the slices aren’t equal:
func Equal(a []byte, b []byte) bool { // Verify if the slices are of the identical size if len(a) != len(b) { return false } for i := vary a { // Verify if the weather in the identical index are the identical if a[i] != b[i] { return false } } return true }
Generate Check Instances
GoLand gives a really highly effective utility that lets you generate assessments routinely. It additionally gives an clever technique to generate assessments based mostly on what you’re making an attempt to realize. You need to use the function to create strong check instances, and the steps are as simple as making a operate, file, or bundle after which clicking buttons to generate the check.
First, press Alt+Insert (⌘N) and choose Check for operate to generate the unit check instances. Alternatively, you possibly can right-click the operate identify and choose Generate:
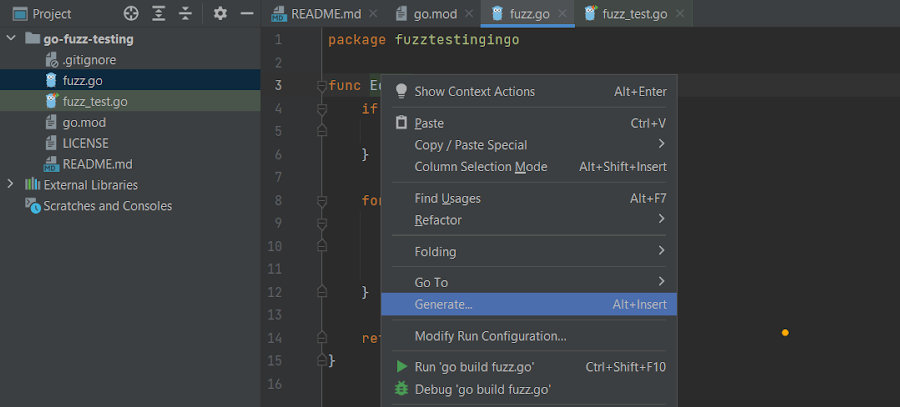
Then, click on Check for operate to generate your check instances:
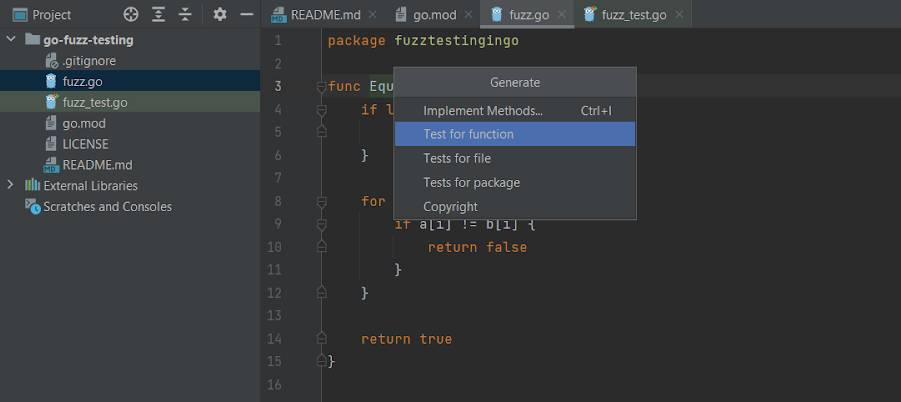
After GoLand generates the primary unit check, you possibly can add your personal assessments if desired. You can too skip the clever check era and create all of the assessments by yourself, although this selection is probably much less efficient.
func TestEqual(t *testing.T) { kind args struct { a []byte b []byte } assessments := []struct { identify string args args need bool }{ { identify: "Slices have the identical size", args: args{a: []byte{102, 97, 108, 99}, b: []byte{102, 97, 108, 99}}, need: true, }, { identify: "Slices don’t have the similar size", args: args{a: []byte{102, 97, 99}, b: []byte{102, 97, 108, 99}}, need: false, }, } for _, tt := vary assessments { t.Run(tt.identify, func(t *testing.T) { if obtained := Equal(tt.args.a, tt.args.b); obtained != tt.need { t.Errorf("Equal() = %v, need %v", obtained, tt.need) } }) } }
As soon as the assessments have been created, there are two methods to run them. You need to use the command go check within the built-in terminal, which can end in a hit or failure standing:
hrittik@hrittik:~/go-fuzz-testing$ go check PASS okay github.com/hrittikhere/go-fuzz-testing 0.003s
Alternatively, you should utilize GoLand to run the assessments by clicking the inexperienced triangle icon within the gutter. The advantage of this strategy is that it additionally supplies you with debugging and recording (out there on Linux machines) capabilities, that are useful for those who’re looking for and perceive a failing check:
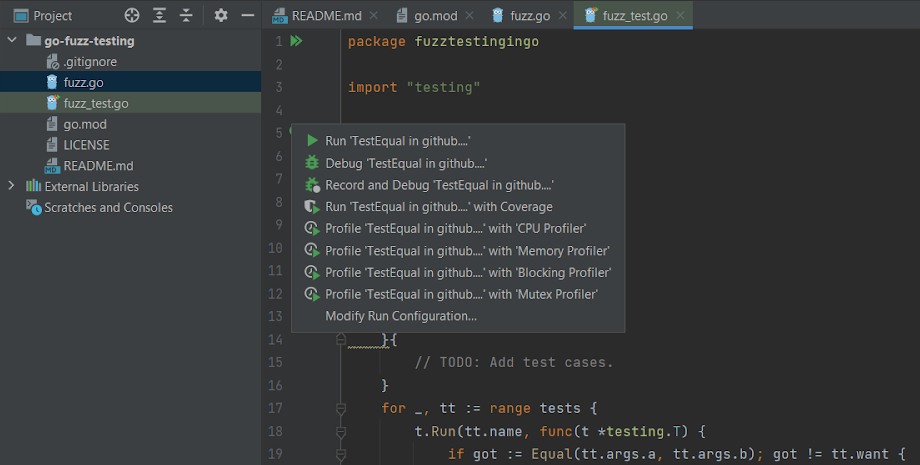
Whenever you use GoLand for testing, the outcomes are proven in a devoted pane with particulars about all assessments that had been run:
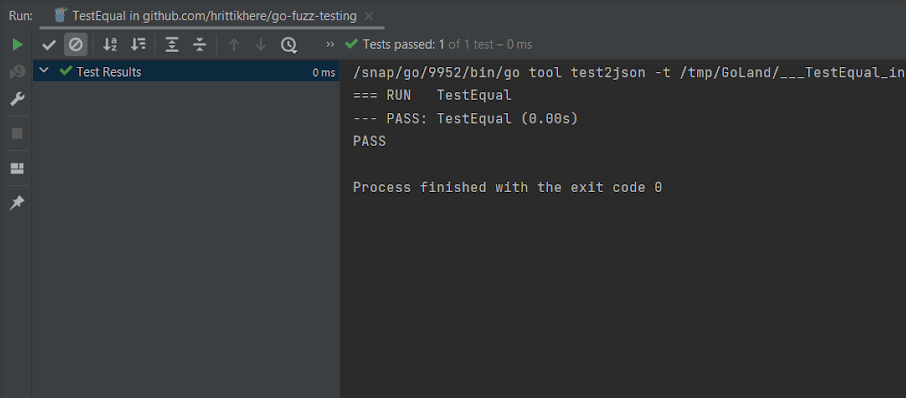
Generate Fuzz Checks
Now that your code has handed a unit check, let’s create a fuzz check. Your check needs to be included within the file you created, and for fuzz assessments you must preserve the next guidelines:
- Your operate identify wants to start with
Fuzz
. - It’s best to settle for the
*testing.F
kind to your operate. - It’s best to solely goal one operate.
You need to use the next code:
func FuzzEqual(f *testing.F) { // Just one operate to be examined f.Fuzz(func(t *testing.T, a []byte, b []byte) { // Worth of a, b will likely be auto generated and handed Equal(a, b) // Equal matches if each auto generated values match and returns False after they do not }) }
Right here, a
and b
are the fuzzing arguments, which will likely be in contrast with Equal
to yield check outcomes. To run the check, you possibly can once more click on the sidebar to open the Run tab, or you possibly can click on the check operate and press Ctrl+Shift+F10 (⌃⇧R).
As fuzz will not be a regular testing methodology, you’ll need to select the second choice, which has the -fuzz
flag, to begin the fuzz check in your IDE:
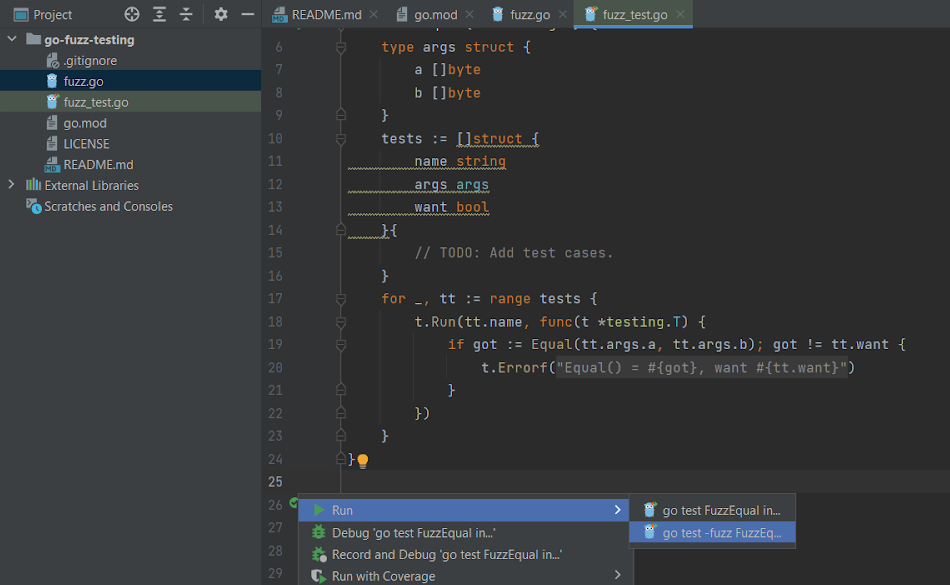
Alternatively, you should utilize go check -fuzz .
, although that methodology doesn’t supply debugging.
As soon as began, fuzz testing will proceed till you manually cease the check. After a number of executions, cease your check by urgent the sq. crimson cease button on the right-hand panel:
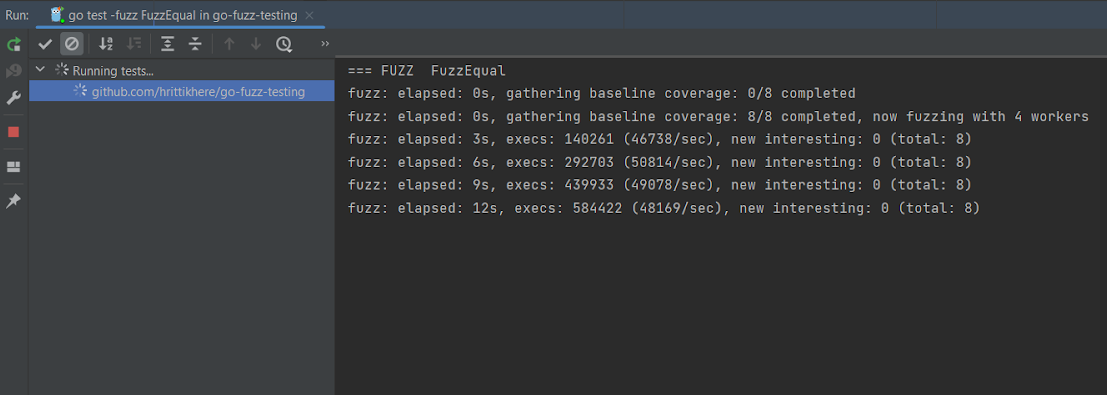
Within the screenshot beneath, after the cease, the check was assessed and reported as having handed, which signifies that all iterations of the check had been profitable:
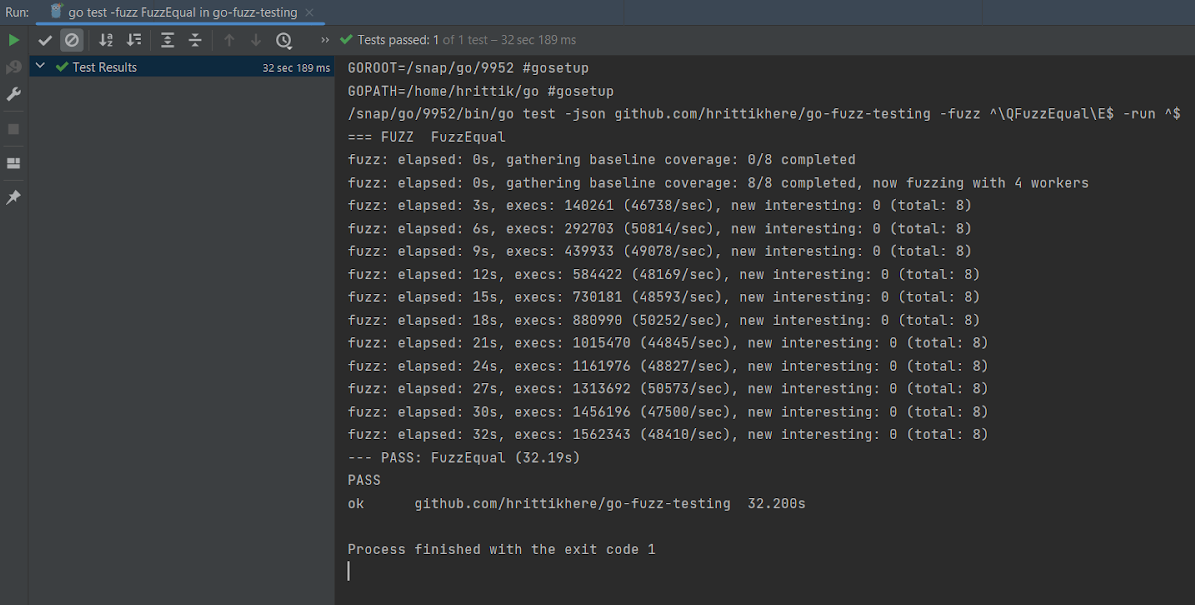
So far as notes go, elapsed is the period of time the check ran for, new attention-grabbing is the variety of inputs added to the corpus that present distinctive outcomes, and execs is the variety of particular person assessments that had been run.
In case your check fails, you should utilize Check Runner to trace your check execution and discover the precise check case that failed, then make modifications to repair your code.
If you wish to see what occurs when your fuzz check in GoLand fails use this instance. If the check fails, the failing seed corpus entry will likely be written to a file and positioned in your bundle listing within the testdata
folder. The trail to this file may even seem within the console as a clickable hyperlink. Should you click on on the hyperlink, the file will likely be opened within the IDE, and you will notice a inexperienced triangle icon on the high of the file. Clicking on this icon will run go check with the failing seed corpus entry.
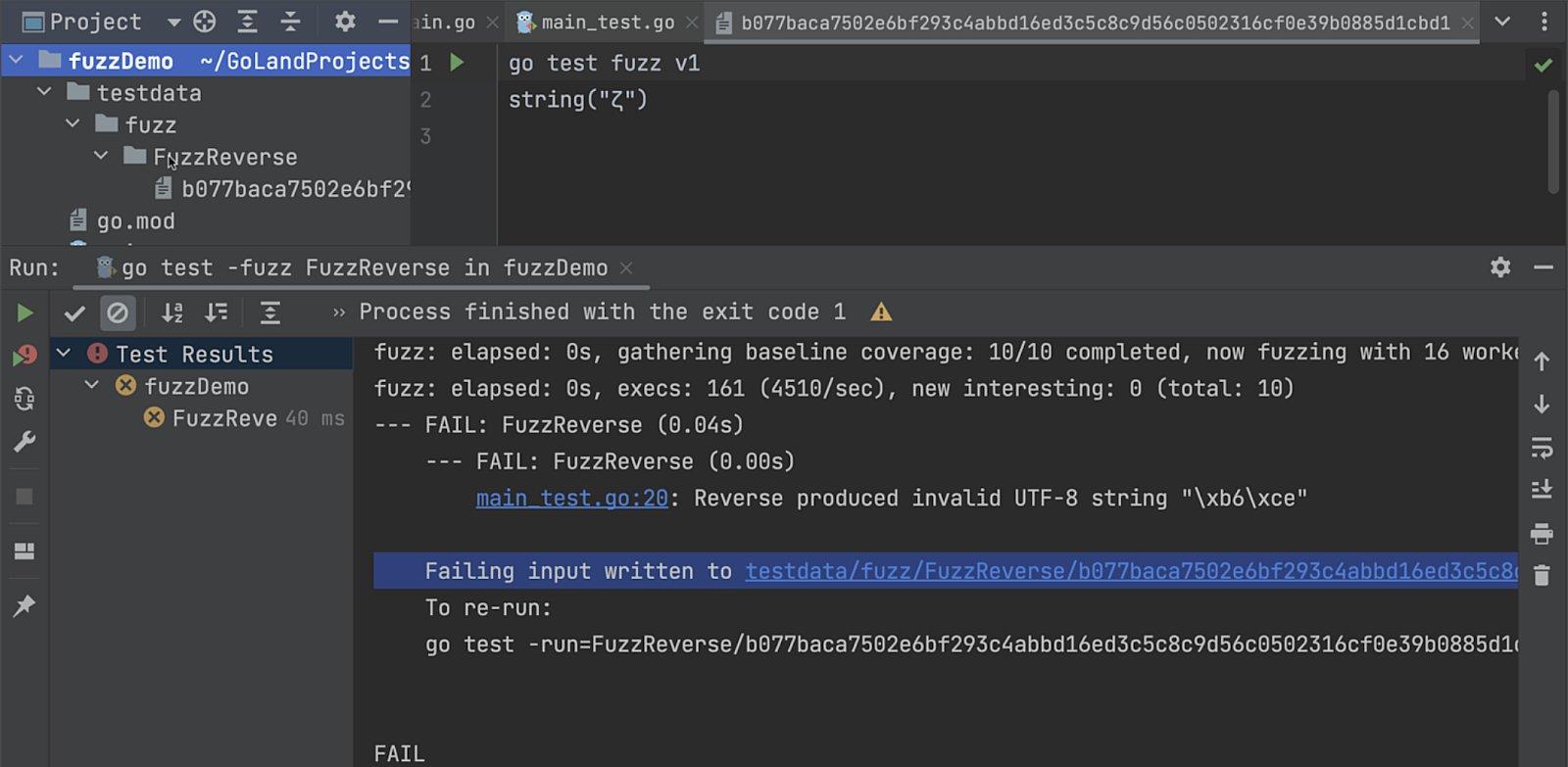
If required, it’s also possible to export the check outcomes to HTML, XML, or a customized XSL template via the Check Runner toolbar.
Superior Methods
Within the earlier part, you checked out a easy fuzz check, however that gained’t be adequate in most manufacturing conditions. For situations that require extra thorough testing, you should utilize the next strategies.
Differential Fuzzing
Differential fuzzing is a fuzzing approach that compares the outcomes of two completely different implementations of a program, every of which receives completely different inputs, to detect bugs that solely happen in sure situations.
One of many largest benefits of differential fuzzing is that it may enable you to uncover bugs that is likely to be hidden when utilizing different fuzzing strategies. A notable instance of that is the flexibility to detect novel HTTP request smuggling strategies.
The primary drawback is that establishing two completely different implementations of this system might be tough. It can be onerous to find out what counts as a “distinction” between them. Moreover, the approach could also be ineffective if the implementations usually are not similar, resembling if one is a debug construct and the opposite is a launch construct.
Spherical-Journey Fuzzing
Spherical-trip fuzzing operates on the premise that if two capabilities with opposing operations exist, the accuracy and integrity of information might be examined by passing the output of the first operate because the enter to the secondary operate, then evaluating the outcomes to the first enter or the unique information.
The advantage of this testing approach is that it may assist discover errors that remoted inputs can miss, like within the case of foreign money conversion, the place a floating level error may not be apparent. Nevertheless, it may additionally introduce new errors if the 2 capabilities usually are not completely inverse, as is commonly the case with rounding errors. Basically, round-trip fuzzing is only when the 2 capabilities are easy and properly understood.
The downside to round-trip fuzzing is that as a result of it’s a must to convert the info twice, it may be tough to arrange, and architecting for complicated programs takes time. Moreover, the completely different information codecs have to be appropriate with each capabilities.
Benefits of Fuzz Testing
Fuzzing’s ease of use and easy implementation make it a necessary testing approach. It expedites the software program improvement lifecycle, making errors simpler to repair and decreasing them within the remaining product.
It is because, in contrast to different testing strategies resembling integration, fuzz testing might be carried out early in improvement, as quickly as any portion of the goal code is written. Fuzz testing lets you goal particular person capabilities whatever the state of the general codebase. It’s additionally distinguished by its means to check a number of elements of an utility on the similar time, which isn’t doable with conventional testing strategies.
Lastly, with fashionable libraries, it’s very simple to implement and catches quite a lot of bugs and safety flaws, making it a obligatory testing approach to have in your arsenal.
Disadvantages of Fuzzing
Fuzz testing is a strong approach for locating software program defects or vulnerabilities, however it has some limitations. It’s not an alternative to conventional testing strategies resembling unit testing, integration testing, and regression testing and needs to be used as a complement to those strategies, not a alternative. Equally, safety updates, pentesting, and compliance with safety requirements have to be continued. Fuzzing will not be the “silver bullet” of software program safety.
One other drawback is that fuzz testing can solely discover defects that end in crashes or different detectable failures.
Lastly, fuzzing tells you that there’s an error, however the tester or developer is liable for debugging and discovering the foundation trigger. This may be simplified by utilizing GoLand, which supplies debugger periods, breakpoints, and core dumps that may enable you to.
Whereas this isn’t essentially a downside, you must also keep in mind that fuzzing is memory- and CPU-intensive, so operating fuzz assessments in your software program supply pipelines will not be advisable.
Conclusion
On this article, you realized about fuzz testing. Fuzzing is a really helpful testing approach and is simple to get began with. The testing methodology is used all through the trade and has assisted Google in catching over fifteen thousand bugs throughout hundreds of instruments and purposes.
Fuzzing is a really efficient and low-cost testing course of. The built-in assist for fuzzing from GoLand makes the implementation even easier.
So what’s subsequent? Extra details about fuzzing might be discovered on the Go Safety weblog.