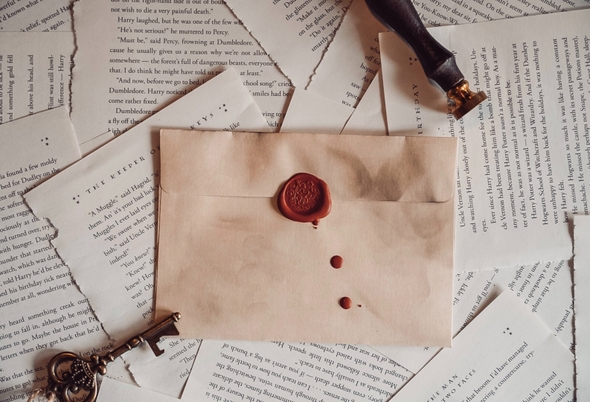
In at the moment’s interconnected digital panorama, file uploads have turn into important to creating net purposes. Whether or not you’re constructing a social media platform, an e-commerce website, or a collaborative doc modifying software, enabling customers to add recordsdata seamlessly is essential.
Angular has been round since 2010, and in accordance with the State of JS analysis, it’s nonetheless the second hottest front-end framework. Nevertheless, you possibly can typically take effort and time to implement a dependable and user-friendly file add performance with Angular. Luckily, with the collaboration of the proper instruments, you possibly can simplify the file add course of and supply a pleasant consumer expertise.
This information will discover the way to deal with file importing with Angular. We’ll construct a easy utility with a user-friendly interface to add single and a number of recordsdata. We’ll additionally determine the way to streamline the event workflow utilizing the Uploadcare widget and implement file-uploading performance in just some minutes.
So, with out additional ado, let’s uncover the way to take your file add performance to the subsequent stage!
Oh, wait! Right here’s what our result’s going to appear like! Wonderful, huh?
Uploadcare Uploader in Angular movement
Organising the Mission
To start, let’s create a brand new Angular venture utilizing the Angular CLI. Should you haven’t put in it but, you are able to do so by following these steps:
- Open your terminal or command immediate.
- Run the command
npm set up -g @angular/cli@newest
to put in the Angular CLI globally in your system. - Run
ng new angular-file-upload
to generate a brand new Angular venture known as “angular-file-upload”.
After the venture is generated, navigate into the venture listing utilizing cd angular-file-upload
.
Implementing the Single File Add Part
Let’s generate a brand new element known as “single-file-upload” to deal with the single-file add performance:
ng generate element single-file-upload
This command will create a number of recordsdata for us, together with single-file-upload.element.html
and single-file-upload.element.ts
, which we’ll deal with on this weblog publish.
Open the single-file-upload.element.html
file and add the next code:
<h2>Single File Add</h2>
<enter kind="file" class="file-input" (change)="onChange($occasion)" #fileUpload />
<div *ngIf="file">
<part class="file-info">
File particulars:
<ul>
<li>Title: {{file.title}}</li>
<li>Kind: {{file.kind}}</li>
<li>Dimension: {{file.dimension}} bytes</li>
</ul>
</part>
<button (click on)="onUpload()">Add the file</button>
<part [ngSwitch]="standing">
<p *ngSwitchCase="'importing'">⏳ Importing...</p>
<p *ngSwitchCase="'success'">✅ Achieved!</p>
<p *ngSwitchCase="'fail'">❌ Error!</p>
<p *ngSwitchDefault>😶 Ready to add...</p>
</part>
</div>
On this code snippet, we’ve added an enter area for file choice, a bit to show file particulars, a button to provoke the file add, and a standing part to point the progress of the add.
Subsequent, open the single-file-upload.element.ts
file and change its contents with the next code:
import { Part } from "@angular/core"
import { HttpClient } from "@angular/frequent/http"
@Part({
selector: "app-single-file-upload",
templateUrl: "./single-file-upload.element.html",
styleUrls: ["./single-file-upload.component.css"],
})
export class SingleFileUploadComponent {
standing: "preliminary" | "importing" | "success" | "fail" = "preliminary"
file: File | null = null
constructor(non-public http: HttpClient) {}
ngOnInit(): void {}
onChange(occasion: any) {
const file: File = occasion.goal.recordsdata[0]
if (file) {
this.standing = "preliminary"
this.file = file
}
}
onUpload() {
}
}
With this TypeScript code, we have now outlined the SingleFileUploadComponent
class. It contains properties to trace the add standing and the chosen file. The onChange
operate is triggered when a file is chosen, updating the file
property with the chosen file. The onUpload
operate is known as when clicking the “Add the file” button. Inside this operate, we’ll create a brand new FormData
object, append the chosen file to it, and ship a POST request utilizing Angular’s HTTP shopper.
To visualise the one file add element, open the src/app/app.element.html file and add the next code:
<h1>Angular File Add</h1>
<app-single-file-upload></app-single-file-upload>
Now, you possibly can run the venture by executing ng serve
within the venture’s root listing. The appliance shall be accessible at http://localhost:4200
, and you need to see the one file add element displayed.
We are able to run ng serve
to view what we constructed on the venture’s root. The outcome ought to appear like this:
Single file add
At this level, we will choose a file for add, and the element will show its particulars. Nevertheless, clicking the “Add the file” button gained’t set off any motion.
Single file add file chosen
We nonetheless want an important element – the precise file-uploading performance. To handle this, we’ll leverage the ability of FormData and Angular’s HTTP shopper capabilities.
Using Angular’s HTTP Consumer and FormData
Angular supplies an distinctive function set, together with its built-in HTTP shopper, which allows easy request dealing with. In our case, we’ll mix this functionality with FormData to perform our file-uploading aims.
To start, let’s incorporate the importing logic into the onUpload
technique situated in single-file-upload.element.ts
:
onUpload() {
if (this.file) {
const formData = new FormData();
formData.append('file', this.file, this.file.title);
const add$ = this.http.publish("https://httpbin.org/publish", formData);
this.standing = 'importing';
add$.subscribe({
subsequent: () => {
this.standing = 'success';
},
error: (error: any) => {
this.standing = 'fail';
return throwError(() => error);
},
});
}
}
On this code snippet, we first validate whether or not a file has been chosen for importing. If a file is current, we create a brand new occasion of FormData
utilizing new FormData()
and assign it to the variable formData
. It allows us to append our file to the formData
object utilizing the formData.append(file)
technique.
Subsequent, we name this.http.publish
with a URL designated for file uploads. In our instance, we make the most of https://httpbin.org/publish
. Nevertheless, you’ll seemingly make use of a backend route or an acceptable third-party service equivalent to Uploadcare. Throughout the POST request, we embody the formData
object as follows:
const add$ = this.http.publish("https://httpbin.org/publish", formData)
Angular’s this.http.publish technique
seamlessly integrates with FormData
, streamlining the file add course of. To make sure the request is distributed, we name the subscribe
technique on the add$
observable. The $
(greenback) suffix is a conference used for observables in Angular. For additional info on Angular’s HTTP Consumer and its RxJS observables, please confer with the official documentation on HTTP server communication. In abstract, invoking add$.subscribe(...)
triggers the execution of the request and initiates its transmission. Moreover, we move an object containing two handlers, subsequent
and error
, to the add$.subscribe()
technique. These handlers enable us to replace the add standing each time they’re invoked. By following these steps, we make sure the seamless supply of the file to the goal URL.
Right here’s the entire code for the element:
import { Part } from "@angular/core"
import { HttpClient } from "@angular/frequent/http"
import { throwError } from "rxjs"
@Part({
selector: "app-single-file-upload",
templateUrl: "./single-file-upload.element.html",
styleUrls: ["./single-file-upload.component.css"],
})
export class SingleFileUploadComponent {
standing: "preliminary" | "importing" | "success" | "fail" = "preliminary"
file: File | null = null
constructor(non-public http: HttpClient) {}
ngOnInit(): void {}
onChange(occasion: any) {
const file: File = occasion.goal.recordsdata[0]
if (file) {
this.standing = "preliminary"
this.file = file
}
}
onUpload() {
if (this.file) {
const formData = new FormData()
formData.append("file", this.file, this.file.title)
const add$ = this.http.publish("https://httpbin.org/publish", formData)
this.standing = "importing"
add$.subscribe({
subsequent: () => {
this.standing = "success"
},
error: (error: any) => {
this.standing = "fail"
return throwError(() => error)
},
})
}
}
}
Right here’s an illustration of how these ties work collectively:
Single file add movement
We choose a file, view its particulars, and click on the “Add the file” button. All through the method, we will monitor the add standing. Upon urgent the “Add the file” button, the standing signifies that the file is at the moment being uploaded. As soon as the add is full, a “Achieved” message is displayed.
Now that we have now lined the basics of displaying a file enter, presenting file particulars, and importing a single file in Angular, let’s discover the method of importing a number of recordsdata.
We choose a file, view its particulars and click on the “Add the file” button. On a regular basis, we will comply with the standing of the add. As soon as we press the “Add the file” button, the standing reveals that the file is importing. As soon as it finishes, we see the “Achieved” message present.
Nice, now that we lined the fundamentals on the way to present file enter, chosen file particulars, and add a single file in Angular – let’s present the way to add a number of recordsdata.
Importing A number of Information in Angular
Many of the code will stay the identical as within the earlier part, the place we demonstrated the way to add a single file. The one half that can change is the part the place customers can select a number of recordsdata to add and the way we deal with them utilizing FormData
. Let’s generate a separate element for a number of file uploads utilizing the ng
CLI software:
ng generate element multiple-file-upload
Subsequent, we’ll start populating the markup in multiple-file-upload.element.html
:
<h2>A number of File Add</h2>
<enter kind="file" class="file-input" a number of (change)="onChange($occasion)" />
<div *ngIf="recordsdata.size" class="file-section">
<part *ngFor="let file of recordsdata">
File particulars:
<ul>
<li>Title: {{file.title}}</li>
<li>Kind: {{file.kind}}</li>
<li>Dimension: {{file.dimension}} bytes</li>
</ul>
</part>
<button (click on)="onUpload()" class="upload-button">Add the file</button>
<part [ngSwitch]="standing">
<p *ngSwitchCase="'importing'">⏳ Importing...</p>
<p *ngSwitchCase="'success'">✅ Achieved!</p>
<p *ngSwitchCase="'fail'">❌ Error!</p>
<p *ngSwitchDefault>😶 Ready to add...</p>
</part>
</div>
The distinction from the one file add is together with the a number of
attribute within the enter
factor. Moreover, we have now an ngIf
part the place we iterate over the chosen recordsdata and show their info. Now, let’s proceed to the element logic in multiple-file-upload.element.ts
:
import { Part } from "@angular/core"
import { HttpClient } from "@angular/frequent/http"
import { throwError } from "rxjs"
@Part({
selector: "app-multiple-file-upload",
templateUrl: "./multiple-file-upload.element.html",
styleUrls: ["./multiple-file-upload.component.css"],
})
export class MultipleFileUploadComponent {
standing: "preliminary" | "importing" | "success" | "fail" = "preliminary"
recordsdata: File[] = []
constructor(non-public http: HttpClient) {}
ngOnInit(): void {}
onChange(occasion: any) {
const recordsdata = occasion.goal.recordsdata
if (recordsdata.size) {
this.standing = "preliminary"
this.recordsdata = recordsdata
}
}
onUpload() {
if (this.recordsdata.size) {
const formData = new FormData()
;[...this.files].forEach((file) => {
formData.append("file", file, file.title)
})
const add$ = this.http.publish("https://httpbin.com/publish", formData)
this.standing = "importing"
add$.subscribe({
subsequent: () => {
this.standing = "success"
},
error: (error: any) => {
this.standing = "fail"
return throwError(() => error)
},
})
}
}
}
On this code, we carry out an identical course of to the SingleFileUploaderComponent
. Nevertheless, on this case, we save the chosen recordsdata
and iterate over them, appending every file to the formData
object as follows:
;[...this.files].forEach((file) => {
formData.append("file", file, file.title)
})
We deconstruct this.recordsdata
to transform the FileList
to an array, enabling us to make the most of array strategies like map
or forEach
.
Now that we have now the whole lot arrange for a number of file importing let’s see the way it works in motion:
A number of file importing movement
That’s all good and dandy, however what if we simplify the implementation additional? Within the subsequent part, we’ll discover the Uploadcare File Uploader, which may make issues even simpler.
Importing Information in Angular with Uploadcare File Uploader
Let’s improve the file importing expertise utilizing the Uploadcare File Uploader. It’s a extremely customizable widget that permits managing recordsdata from numerous sources, storing them by way of exterior CDN, and optimizing them for efficiency wants.
To get began, it’s good to set up the Uploadcare Blocks bundle utilizing the next command:
npm set up @uploadcare/blocks
Moreover, it’s possible you’ll want so as to add an choice to tsconfig.json
to allow the default import from @uploadcare/blocks
. You may simply skip this step.
{
"compilerOptions": {
"allowSyntheticDefaultImports": true
}
}
Subsequent, within the app.module.ts
file, add the CUSTOM_ELEMENTS_SCHEMA
in order that we will use the @uploadcare/blocks
customized components to render the uploader.
import { NgModule, CUSTOM_ELEMENTS_SCHEMA } from "@angular/core"
import { BrowserModule } from "@angular/platform-browser"
import { HttpClientModule } from "@angular/frequent/http"
import { AppComponent } from "./app.element"
@NgModule({
declarations: [
AppComponent,
],
imports: [BrowserModule, HttpClientModule],
suppliers: [],
bootstrap: [AppComponent],
schemas: [CUSTOM_ELEMENTS_SCHEMA],
})
export class AppModule {}
Now, let’s generate a element to deal with the Uploadcare logic utilizing the ng generate
command:
ng generate element uploadcare-upload
Inside uploadcare-upload.element.html
, add the mandatory markup to render the uploader:
<h2>Uploadcare File Uploader</h2>
<div class="wrapper">
<lr-file-uploader-regular
class="uploader-cfg"
css-src="https://unpkg.com/@uploadcare/[email protected]/net/file-uploader-regular.min.css"
>
<lr-data-output
(lr-data-output)="handleUploaderEvent($occasion)"
use-event
hidden
class="uploader-cfg"
></lr-data-output>
</lr-file-uploader-regular>
<div class="output">
<img *ngFor="let file of recordsdata" src="{{ file.cdnUrl }}" width="300" />
</div>
</div>
Right here, we have now two customized components: lr-file-uploader-regular
and lr-data-output
. The lr-file-uploader-regular
factor supplies the UI for importing recordsdata, whereas lr-data-output
permits us to trace the uploaded and eliminated recordsdata within the UI.
Observe that each components have the category attribute with the worth “uploader-cfg” as a result of Uploadcare Blocks are personalized utilizing CSS. The kinds are positioned in uploadcare-upload.element.css
, and right here’s its content material:
.uploader-cfg {
--ctx-name: "uploader";
--cfg-pubkey: "demopublickey";
}
The --ctx-name
variable ensures that each lr-file-uploader-regular
and lr-data-output
are linked. To work appropriately, each components should have the identical --ctx-name
. The --cfg-pubkey
variable is the place you need to place your Uploadcare venture API key. We use the demo public key on this instance, however you need to change it together with your public key.
Subsequent, let’s add the logic to uploadcare-upload.element.ts
:
import { Part } from "@angular/core"
import * as LR from "@uploadcare/blocks"
LR.registerBlocks(LR)
@Part({
selector: "app-uploadcare-upload",
templateUrl: "./uploadcare-upload.element.html",
styleUrls: ["./uploadcare-upload.component.css"],
})
export class UploadcareUploadComponent {
recordsdata: any[] = []
handleUploaderEvent(e: Occasion) {
const { knowledge: recordsdata } = (e as CustomEvent).element
this.recordsdata = recordsdata
}
}
On this code, we import LR
from @uploadcare/blocks
and name registerBlocks(LR)
to register the elements used inside UploadcareUploadComponent
. The handleUploaderEvent
operate shops the uploaded recordsdata within the element in order that we will render and show them to the consumer.
Now that we have now added all the mandatory logic let’s see the way it works in motion:
Uploadcare Uploader in Angular movement
And that’s principally it!
Uploadcare takes care of the importing course of, accelerating it by utilizing its importing community (just like a CDN), monitoring the importing progress, processing recordsdata on the fly, and much more.
You may examine the documentation on File Uploader and see the way to configure it and tailor it to your wants.
Conclusion
So, we lined numerous points of file importing in Angular 2+:
- The way to show a correct file enter and present chosen file particulars;
- The way to work with FormData interface and Angular’s HTTP shopper for importing single or a number of recordsdata;
- The way to present a easy importing standing indicator within the UI;
- The way to iterate over FileList with a neat trick;
- Hnd the way to effectively use Uploadcare File Uploader to simplify file administration.
Hope you loved this information. You’ll find all of the supply code within the GitHub repo and even attempt them out within the dwell playground.
Thanks for studying, and catch you within the subsequent one.