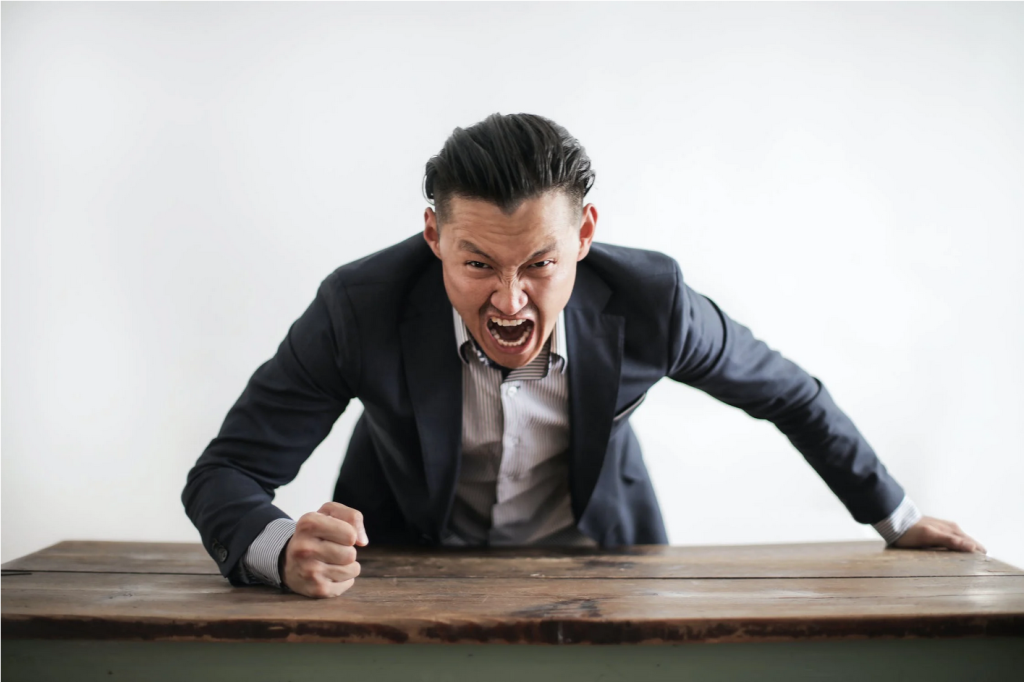
The “TypeError: 'methodology' object is just not subscriptable
” happens while you name a technique utilizing sq. brackets, e.g., object.methodology[3]
. To repair it, name the non-subscriptable methodology solely with parentheses like so: object.methodology(3)
.
How Does the Error Happen (Instance)
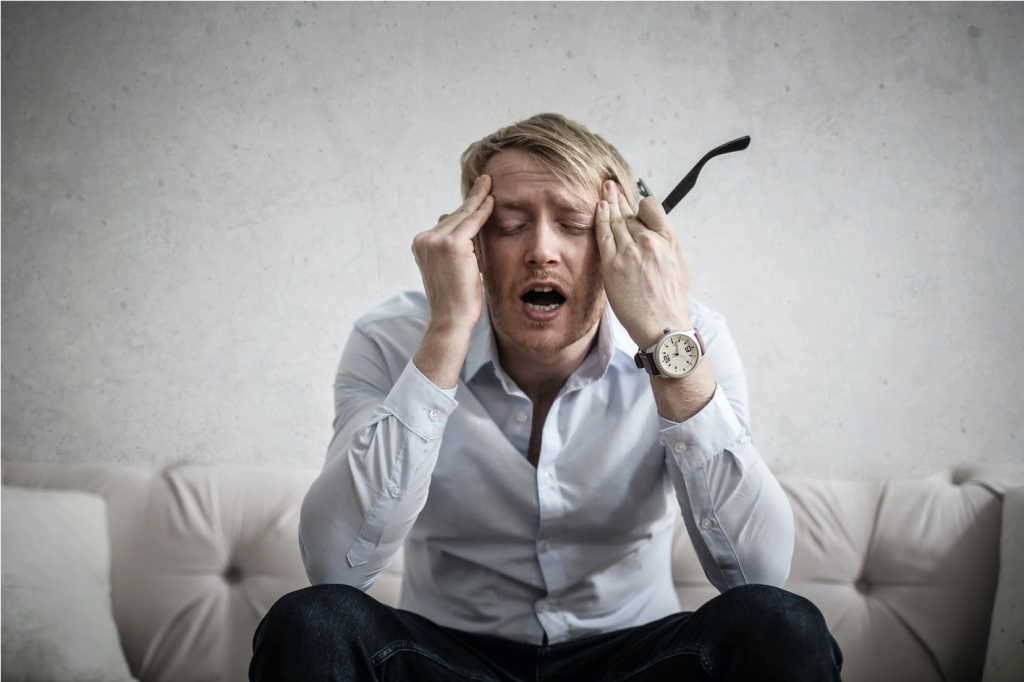
Within the following minimal instance, you try to outline a customized “listing” class and also you attempt to entry the listing aspect at positon 1 by utilizing the tactic name my_list.get[1]
. ⚡
class MyList: def __init__(self, lst): self.lst = lst def get(self, index): return self.lst[index] my_list = MyList(['Alice', 'Bob', 'Carl']) print(my_list.get[1])
Nevertheless, Python raises the next TypeError: 'methodology' object is just not subscriptable
:
Traceback (most up-to-date name final): File "C:UsersxcentDesktopcode.py", line 11, in <module> print(my_list.get[1]) TypeError: 'methodology' object is just not subscriptable
The reason being that Python thinks you are attempting to entry the my_list.get
methodology object at place 1
. Nevertheless, regardless that a technique is an object in Python too, this isn’t what you wish to do. You wish to entry the place 1
of the listing inside the my_list
object!
An instance follows in a minute! ⤵️
The same error would happen for any of the next indexing or slicing calls:
my_list.get[1, 2, 3]
my_list.get[1:3]
my_list.get[:]
my_list.get[-1]
Once more, you can’t name strategies the identical method you’d name lists, i.e., utilizing the sq. bracket notation.
The way to Resolve the Error (Straightforward) + Instance
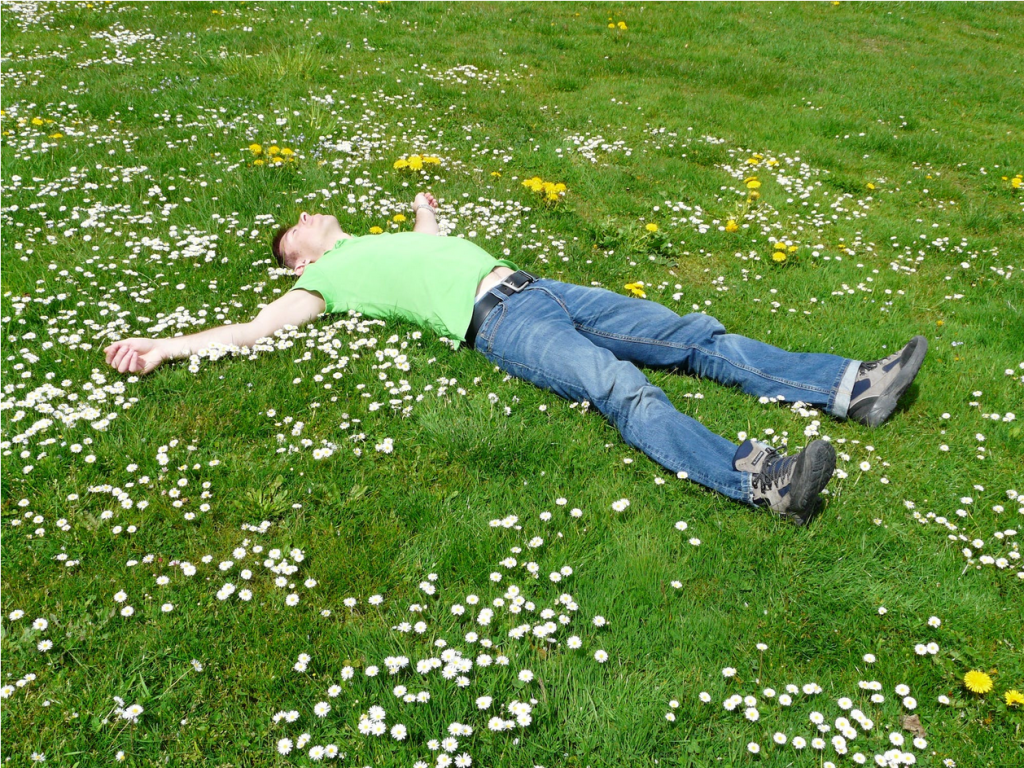
To repair this error within the best doable method, merely don’t name strategies utilizing the sq. bracket notation. Solely use the sq. bracket notation on subscriptable listing or sequence objects (or objects that outline the __getitem__()
methodology, see beneath).
In lots of circumstances, that is merely a matter of utilizing the conventional parentheses that was missed. So, use object.methodology([1])
or object.methodology(1)
as a substitute of object.methodology[1]
.
The next code exhibits appropriately entry the strategies get()
and append_all()
utilizing our customized listing instance:
class MyList: def __init__(self, lst): self.lst = lst def get(self, index): return self.lst[index] def append_all(self, lst): self.lst.prolong(lst) my_list = MyList(['Alice']) my_list.append_all(['Bob', 'Carl']) print(my_list.get(-1)) # Carl
Each methodology calls now work superbly. ❤️
Stick with me for a second as a result of there’s truly a really Pythonic idea that you need to know. It’s known as “magic strategies” or “dunder strategies”. 👇
The way to Resolve the Error (Superior) + Instance
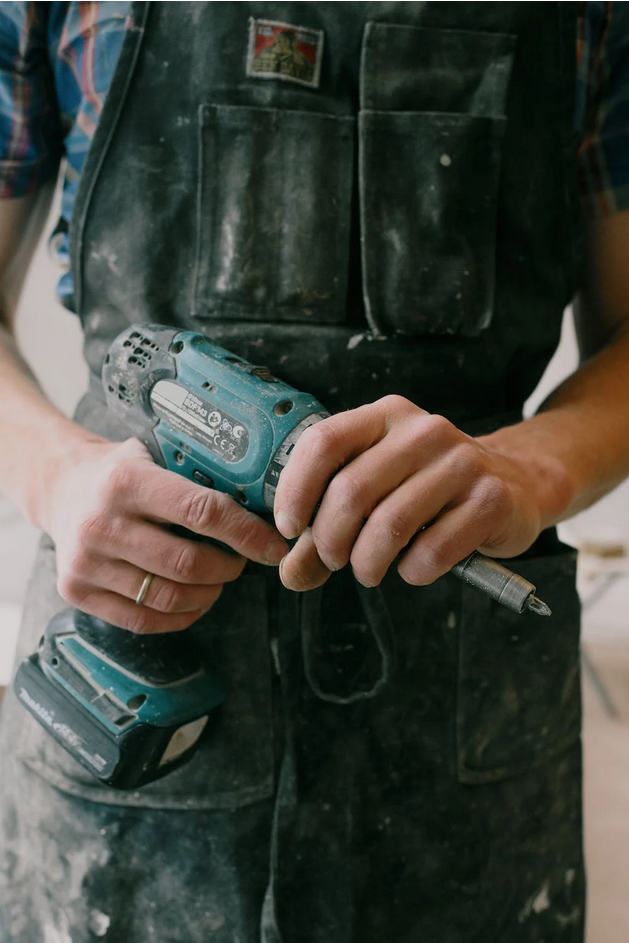
In some circumstances, you may very well wish to make the category subscriptable. I present you ways to do that only for enjoyable and that will help you enhance your expertise. 👍
ℹ️ Python raises the TypeError object is just not subscriptable
should you use indexing with the sq. bracket notation on an object that’s not indexable. That is the case if the article doesn’t outline the __getitem__()
dunder methodology.
Right here’s a minimal instance that defines the dunder methodology and, thereby, making the customized class MyList
subscriptable so you need to use the sq. bracket notation like so: MyList[0]
.
class MyList: def __init__(self, lst): self.lst = lst def __getitem__(self, index): return self.lst[index] my_list = MyList(['Alice', 'Bob', 'Carl']) print(my_list[0]) # Alice
Yay! This manner, you may truly apply the sq. bracket notation to your customized class!
Be at liberty to learn on about this matter in our detailed information right here:
👉 Beneficial Tutorial: Python TypeError: Object is Not Subscriptable (The way to Repair This Silly Bug)
Additionally, be at liberty to affix our free electronic mail academy — it’s enjoyable and we have now cheat sheets!
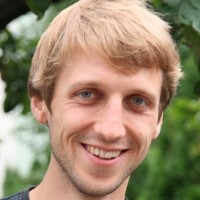
Whereas working as a researcher in distributed techniques, Dr. Christian Mayer discovered his love for educating pc science college students.
To assist college students attain increased ranges of Python success, he based the programming schooling web site Finxter.com. He’s writer of the favored programming e book Python One-Liners (NoStarch 2020), coauthor of the Espresso Break Python collection of self-published books, pc science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his best ardour is to serve aspiring coders by way of Finxter and assist them to spice up their expertise. You’ll be able to be part of his free electronic mail academy right here.