With this text, we proceed our journey by means of the realm of Solidity information varieties.
👉 Beneficial Tutorial: Solidity Primary Information Sorts
This time, we are going to concentrate on mounted level numbers, handle varieties and handle sort members, and examine when, how, and why they’re used.
Historically, our articles are operating alongside the official Solidity documentation and are used to make clear, complement, and clarify the ideas specified by the documentation.
Mounted Level Numbers
⚡ Warning: Solidity nonetheless doesn’t absolutely assist fixed-point numbers, in that it permits solely the declaration, however not the task from and to mounted level variables.
Mounted level numbers exist within the signed (mounted
) and unsigned (ufixed
) variants and sizes.
Key phrases could be written in a common type of ufixedMxN
and fixedMxN
, the place the parameter M
represents the variety of bits that our sort takes, and the parameter N
represents what number of decimal factors are reserved for our sort.
As with the integer sort, the parameter M
begins with the worth 8 and likewise increments within the steps of 8, as much as the worth of 256. The parameter N
could be any integer between 0 and 80 (included).
If we categorical our mounted level sort as mounted
or ufixed
(with out the parameters M
and N
), it’s interpreted as an alias for fixed128x18
and ufixed128x18
.
Mounted Level Operators
Right here we’ll checklist the obtainable operators for mounted level varieties:
- Comparisons:
<=, <, ==, !=, >=, >
(consider to bool) - Arithmetic operators:
+, -, unary -, *, /, %
(modulo)
Mounted vs Floating Level Numbers
We now have to make some extent in regards to the distinction between floating level and mounted level numbers.
Floating level numbers normally correspond to drift and double in lots of programming languages; technically talking, they’re known as IEEE 754 numbers, named after their defining customary IEEE 754.
The principle distinction is that with the mounted level numbers, the variety of bits used for the integer and the fractional half (after the decimal dot) is strictly outlined, whereas with the floating level numbers the variety of bits used for the integer and the fractional half is versatile.
In follow, floating level numbers take up virtually your entire house to signify the quantity, and solely a small variety of bits will probably be used to outline the placement of the decimal level.
Tackle Sort
The handle sort is accessible in two variants, and they’re virtually an identical however don’t fear, we’ll contact on the distinction in a second:
handle
: The kind is related to a 20-byte worth, which is a dimension of an Ethereum handle.handle payable
: The kind is similar ashandle
, the one distinction is that it has extra membersswitch(...)
andship(...)
.
The distinction between the 2 lies in a necessity to tell apart an handle, i.e., to handle payable
we are able to ship Ether, from a plain handle that holds a sensible contract not designed to obtain Ether.
In different phrases, the 2 handle varieties exist to stop sending the foreign money to the improper handle, whereas we have now to explicitly specify the appropriate handle.
Learn how to Convert “handle” to “handle payable” in Solidity?
In fact, in some instances, we’ll have to have the ability to convert the handle
sort to the handle payable
sort, and for that, we use the specific conversion operate payable(<handle>)
. Conversion within the different route, i.e. from the handle payable sort to handle sort is implicit.
Explicitly conversions to and from the handle sort are allowed for uint160
, integer literals (variants int8/uint8
to int256/uint256
), bytes20
, and contract
sort.
Solely variables of sort handle
and contract
sort could be transformed to the sort handle payable
by utilizing the specific conversion operate payable(<handle>)
and payable(this)
. The key phrase this
is a reference to the present contract.
In case we’re changing a contract
sort, the contract should be capable of obtain Ether, i.e. it both has a obtain()
operate or a payable
fallback operate.
Nevertheless, there may be an exception to the rule: the expression payable(0)
.
💡 Be aware: After we’re going through a requirement of needing an handle variable and likewise plan to ship Ether to this handle, we should always declare the handle
sort as handle payable
as quickly as potential to keep away from any pointless conversions.
Tackle Sort Comparability Operators
The operators obtainable to be used with the handle sort are the comparability operators:
<=
Lower than or equal to<
Lower than==
Equal to!=
Not equal to>=
Higher than or equal to>
Higher than
Warning: since there’s a risk to transform a bigger sort, e.g. bytes32
to an handle, the handle will probably be truncated. Solidity creators made a step in lowering this ambiguity since Solidity compiler v0.4.24, forcing us to explicitly truncate the bigger sort variable.
If we take a 32-byte worth
b = 0x111122223333444455556666777788889999AAAABBBBCCCCDDDDEEEEFFFFCCCC
,
we are able to convert it by maintaining the primary 20 bytes, like in
handle(uint160(bytes20(b))) = 0x111122223333444455556666777788889999AAAA
,
or the final 20 bytes, like in
handle(uint160(uint256(b))) = 0x777788889999AAAABBBBCCCCDDDDEEEEFFFFCCCC
.
💡 Be aware: The kinds handle
and handle payable
had been launched with Solidity v0.5.0. Another novelty that this model launched was that the contracts stopped being derived from the handle
sort, though they will nonetheless be explicitly transformed to varieties handle
or handle payable
, however provided that they’ve a obtain()
or payable
fallback operate.
👉 Beneficial Tutorial: What’s Solidity payable
?
Tackle Sort Members
The 2 notable members of an handle
or handle payable
sort are property stability
and performance switch(...)
.
- The property
stability
permits us to test the stability of an handle. - The operate
switch(...)
permits us to ship Ether expressed in items of Wei to apayable
handle.
Each use instances are proven within the instance:
handle payable x = payable(0x123); handle myAddress = handle(this); if (x.stability < 10 && myAddress.stability >= 10) x.switch(10);
The switch operate will fail if the stability on the present contract is smaller than 10 Wei or if the receiving account rejects the switch. In case of a failure, the switch()
operate will revert.
💡 Be aware: If x
within the supply code above holds a contract handle, its supply code, i.e. its operate for receiving Ether or its fallback operate, will probably be executed with the decision of the switch(...)
operate. This conduct is an inherent, non-optional function of EVM. If the execution of those capabilities depletes the connected fuel or fails in another means, the Ether switch will probably be reverted, and the present contract will terminate, elevating an exception.
ℹ️ Information: “The fallback operate is executed on a name to the contract if not one of the different capabilities match the given operate signature, or if no information was equipped in any respect and there’s no obtain Ether operate” (docs).
Talking of foreign money (Ether) switch, the ship(...)
operate represents the low-level variant of the switch(...)
operate. If the ship(...)
operate execution fails, the present contract is not going to terminate throwing an exception, however as a substitute, ship(...)
will return a boolean worth false
.
Warning: The ship(...)
operate additionally brings some risks with it, e.g. the switch will fail if the decision stack depth reached 1024, and the caller can power such an occasion.
Additionally, the switch will fail if the foreign money recipient runs out of fuel. Subsequently, good programming patterns for secure Ether switch embrace checking the return worth of the ship(...)
operate or utilizing the switch(...)
operate, however one of the best sample is the one the place the recipient withdraws the foreign money.
After we need to interface with the contracts that aren’t compliant with the ABI or get direct management over the encoding course of, we’d use the capabilities name(...)
, delegatecall(...)
, and staticcall(...)
.
Their enter parameter is a single bytes reminiscence and the return outcomes are the success situation (bool sort) and the returned information (bytes reminiscence sort, similar because the enter parameter).
The capabilities abi.encode(...)
, abi.encodePacked(...)
, abi.encodeWithSelector(...)
, and abi.encodeWithSignature(...)
can be found for encoding the structured information:
bytes reminiscence payload = abi.encodeWithSignature("register(string)", "MyName"); (bool success, bytes reminiscence returnData) = handle(nameReg).name(payload); require(success);
Warning: since abi.
capabilities talked about above are low-level capabilities, they need to be used with warning, i.e. an unknown contract could also be malicious, and if we name it, we’re giving it management that may be used to name our contract in return.
In that case, we should always put together for contract state modifications with the decision return. The common means of interacting with different contracts is looking a operate uncovered on a contract x
‘s object, e.g. x.f()
.
The quantities of equipped fuel and Ether could be adjusted in the course of the operate name by supplying the fuel and Ether modifiers:
handle(nameReg).name{ fuel: 1000000, worth: 1 ether }(abi.encodeWithSignature("register(string)", "MyName"));
In fact, we might’ve positioned your entire name(...)
operate name in the identical line, however I cut up it into three strains for higher readability.
Much like the name(...)
operate, the delegatecall(...)
operate will also be referred to as, however as talked about a couple of articles earlier than, solely the code of the given handle (contract) will probably be used, and every part else, equivalent to storage, stability, and so on. will probably be disregarded.
Let’s remind ourselves, delegatecall(...)
can solely name the library code saved on this different contract. Nevertheless, it’s as much as us as customers to test if the format of storage in each contracts is appropriate for calling the delegatecall(...)
.
💡 Be aware: Earlier than the EVM model Homestead, solely a restricted variant of the delegatecall(...)
operate named callcode(...)
was obtainable, nevertheless it didn’t present entry to the unique msg.sender
and msg.worth
values. callcode(...)
was eliminated in Solidity v0.5.0.
💡 Additional Be aware: The EVM model Byzantium launched the staticcall(...)
operate. It may be used the identical because the name(...)
operate, nevertheless it’s going to revert if the referred to as operate modifies the contract state.
We should always take into consideration that each one three capabilities, name(...)
, delegatecall(...)
, and staticcall(...)
work on a really low degree and must be used solely when there’s no different strategy to obtain the required performance as a result of using these capabilities violates the type-safety of Solidity.
The fuel
choice is accessible on all three strategies, whereas the worth
choice is just obtainable on the decision(…) operate.
💡 Be aware: The most effective follow is to keep away from utilizing hardcoded fuel values in our sensible contracts, whatever the entry to the state variables.
There are two extra noteworthy handle
sort members within the context of our story: code
and codehash
.
- Member
<handle>.code
permits us to question the deployed code for a sensible contract, i.e. to get the EVM bytecode within the type of bytes reminiscence, which could be empty. - Member
<handle>.codehash
offers us with the Keccak-256 hash of the deployed supply code within the type of bytes32.
We must be conscious that <handle>.codehash
is cheaper than calling the operate keccak256(addr.code)
.
As was talked about a couple of articles in the past, each contract could be transformed to the handle
sort, so getting the contract’s stability is feasible through handle(this).stability
.
Conclusion
With this text, we made three extra crucial steps within the route of attending to know Solidity information varieties, particularly fixed-point numbers, handle, and handle sort members.
What we get by intently realizing Solidity information varieties with the ability to choose the appropriate information sort in a particular state of affairs.
Additionally, this information offers us the combating probability to keep away from widespread errors and pitfalls in the course of the design and coding phases in creating our sensible contracts.
First, we made a well mannered and mild introduction to in the present day’s matters.
Second, we defined what mounted level numbers are, made a brief comparability with the floating level numbers, and jumped on the subsequent, necessary level.
Third, we by no means earlier than received so shut with addresses. Any nearer, and we’d go postal.
Fourth, we jumped into an in depth dialogue about handle sort members (capabilities and properties). It received fairly technical, however there’s no higher means of studying than poppin’ the hood.
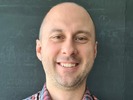
I’m an skilled laptop science engineer and know-how fanatic devoted to understanding how the world works and utilizing my data and skill to advance it. I’m targeted on changing into an knowledgeable in Solidity and crypto know-how, with a ardour for coding, studying, and contributing to the Finxter mission of accelerating the collective intelligence of humanity.