Harness the ability of SwiftUI 4

In a earlier article, I wrote a CSV parser. A pattern utility was used to parse housing knowledge retrieved from Zillow. Over the following couple of chapters, we are going to write an utility to show that knowledge in a desk and chart.
I’ll break this up into small chunks. Chapter 1 will likely be about knowledge parsing. Chapter 2 will have a look at the SwiftUI Desk class. Chapter 3 will likely be all about SwiftUI Charts.
Problem
The housing knowledge is giant. The CSV on the time of writing is 909 rows x 265 columns. The concept is to see what limitations we’ve with the SwiftUI when coping with giant knowledge. The purpose is to make use of solely SwiftUI and no UIKit.
As we go alongside we are going to talk about the boundaries of SwiftUI’s Desk and Chart and design round them.
Fast Specification and Design
The purpose is to have the ability to choose completely different housing areas and examine them on a chart. The housing areas will likely be introduced in a Desk and the chart knowledge will likely be used the SwiftUI 4’s Chart. We’ll hold the design easy with the chart above the desk.

All the information will likely be retrieved from Zillow’s Housing knowledge. We’ll have a look at the median dwelling listing value month by month for the US and main Metro areas.
This mission would require macOS Ventura and XCode 14. Each are presently in beta as this text is being written.
Xcode Venture
- Open XCode 14.
- Create a brand new SwiftUI Venture with Unit Assessments.
Add the CSV Parser
In a earlier article, I wrote a command line CSV parser. I’ve turned that right into a bundle referred to as EasyCVS that can be utilized to parse the CSV file. So as to add the bundle:
- Choose Add Package deal from the file menu
- Put https://github.com/scottandrew/EasyCSV.git into the search field
- Press “Add Package deal”.
CSV Format
RegionID,SizeRank,RegionName,RegionType,StateName,2018-01-31,...
102001,0,United States,Nation,,271600.0,...
394913,1,"New York, NY",Msa,NY,517967.0,...
753899,2,"Los Angeles-Lengthy Seaside-Anaheim, CA",Msa,CA,798670.0,...
- Row 0 is column headers. The information begins on Row 1.
- Column 0 = Integer for a area id
- Column 1 = Integer for Measurement Rank
- Column 2 = Area identify as string
- Column 3 = Area sort as a string
- Column 4 = State identify as a string or null
- Columns 5 by way of the tip a double worth or null representing the median value.
Value Information
Create the PriceData.swift
file to retailer the median value and date for the information. There will be empty columns if there isn’t a knowledge so the worth
property must be elective.
PriceData
must be Identifiable
in order that it’s distinctive for a collection. So the date will likely be used for our id.
Area Information
Create RegionData.swift
and add the next code:
The area knowledge will assist outline row rows within the desk. The PriceData
for every area is what will likely be charted. The initializer will take a row of knowledge and can take the array of dates from the header.
There’s an ColumnIndex
enum to outline the index of the values within the row. Notice that there’s an index of firstDate
that is the index of the primary column to make use of for the worth knowledge. The trickiest half is mapping the dates to the median costs.
To create the PriceData
array we take the values from the dateStartIndex
to the tip of the information array. We’ll use zip
to mix the values after which map the outcome to a PriceData
construction.
The .toDouble
and .toInt
calls on strings are right here for comfort. The helpers will try to create an Int
or a Double
from a string. An error is thrown if it fails. Create a String+Utility.swift
file with the next code:
HousingDataParser
A small parsing engine is required to deal with the conversion from traces of knowledge, which is an array of strings, to an array of HousingData
.
Create a RegionDataParser.swift
file with two features parse(url:)
will likely be used to parse the information out. extractDataColumns(from)
will likely be used to create match values to dates and create the worth array for a area.
The attention-grabbing factor within the code is that the primary column, 0, is the header. We need to extract the dates from the header. If we’re lacking date, or can’t parse a date, an error is thrown. This assumption is that there are the identical variety of dates and median values within the CSV.
Unit Testing the parser
The parser must be examined with some pattern knowledge. I downloaded some pattern knowledge to make use of from Zillow. I renamed the file TestData.json. The unit check will verify:
- Parses the file
- Verifies the variety of areas.
- Validates 2 areas which have some null columns.
- validates the variety of values in every row.
Preview Information
To make the chart work with preview we’d like some knowledge. I’ll create a neighborhood CSV file with a subset of housing knowledge.
- Proper-click on the
Preview Content material
folder within the mission tree - Choose Empty from the Different group within the template chooser.
- Identify the file PreviewData.csv
- Copy the primary 2 traces out of the TestData.csv file.
- Copy the Indianapolis, IN knowledge from TestData.csv. This has null columns so its is sweet preview knowledge.
View Mannequin
I’m going to make use of a light-weight ViewModel
to wrap our parser and handle our knowledge loading. The views must be all about viewing the fetched knowledge. There are a few targets:
- We need to asynchronously load the most recent knowledge from Zillow.
- We need to make view mannequin behave properly with SwifUI’s preview.
I’ll create a brand new HousingDataModel.swift
file:
- Wrap the category in across the
@MainActor
attribute this ensures that the printed knowledge notifications occur on the principle thread. - Test if the code is working in a SwiftUI preview. Whether it is we are going to load the preview CSV file. In any other case, load knowledge from the web.
- To ensure the most recent knowledge is loaded. The URL’s
t
argument is right this moment’s epoch date (date since 1970) as an integer.TimeInterval
is aDouble
to typecast it I’ll create anInt
from theDouble
HousingDataView (1st cross)
I’m going to take a fast cross 1st cross on the view to verify the parsing is working. Earlier than doing that we’d like to verify we are able to obtain knowledge from the web within the utility.
- Navigate to the HousingData goal
- Within the Signing & Capabilities panel verify the Outgoing Connections (Shopper) verify field.
I’m going to rename the ContentView
to HousingDataView
.
- Open the
ContentView.swift
file. - Choose the
ContentView
class identify and proper click on. - Choose Refactor -> Rename from the context menu.
- Kind HousingDataView
This could rename the ContentView.swift
file and think about struct. Now I need to clear up the preview class for consistency. Rename the ContentView_Preview
to HousingDataView_Preview
I’m going to depart the pattern code however change the “Whats up World” textual content discipline in order that it shows the depend of the parsed rows.
- Observe the
HousingModel
for adjustments - Change the “Whats up World” textual content with the variety of parsed rows
- use the
.activity
modifier to asyncronously load our knowledge when the view is loaded. When an error happens “oops” will likely be printed. That may get fastened quickly.
Now when the view is previewed 3 is displayed. When the applying is run, the worth will replace to 908 after the information has been loaded.


SwiftUI Desk
Tables have been in SwiftUI since SwiftUI 3. The limitation with Tables in SwiftUI is they’re restricted to 10 columns. So we cannot construct a scrolling unfold sheet view that shows all of the columns. To work across the limitation the applying will present the rank, area identify, area sort, and state within the desk.
Tables comprise a set of columns which might be constructed utilizing a TableColumnBuilder. TableColumns will be linked on to RegionData properties if the worth is a String
or helps the StringProtocol
. If the worth is elective or doesn’t conform to the StringProtocol
a view will must be created.
- For string knowledge that’s not elective create a desk columns that immediately level to the property within the row’s area.
- For the area’s sort (enum) and rank (int) textual content discipline is inserted with the given worth. We might return any SwiftUI view right here.
- For the state, which is elective, a textual content view must be inserted with both the worth or empty textual content. There should be a view in a column, even when there isn’t a knowledge.
- A set width is given to the all columns however the “Identify” column.
Since preview was setup to work with the information the desk seems in preview with the three check rows.
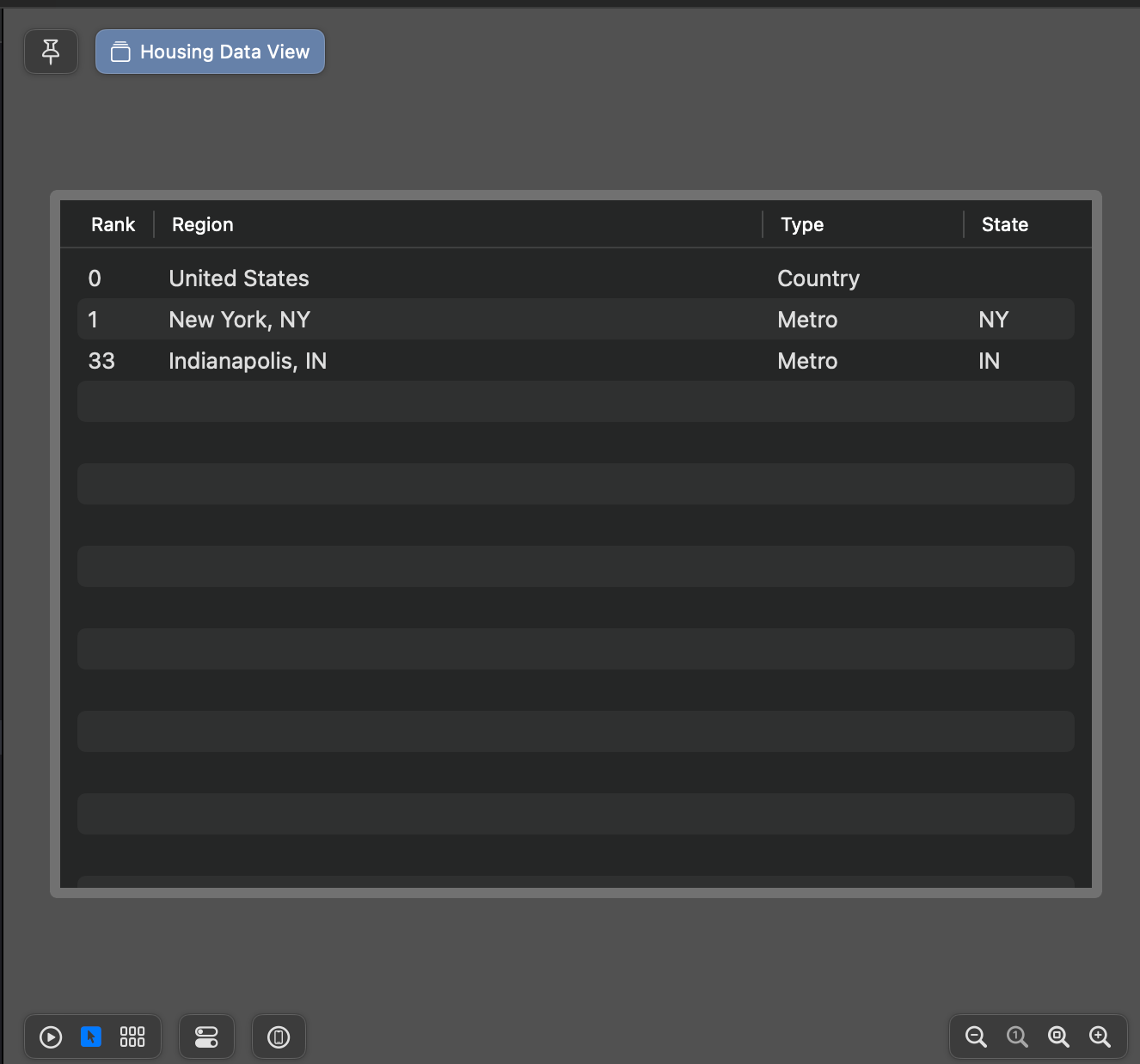
Operating the applying will will let you scroll by way of all of the area knowledge.
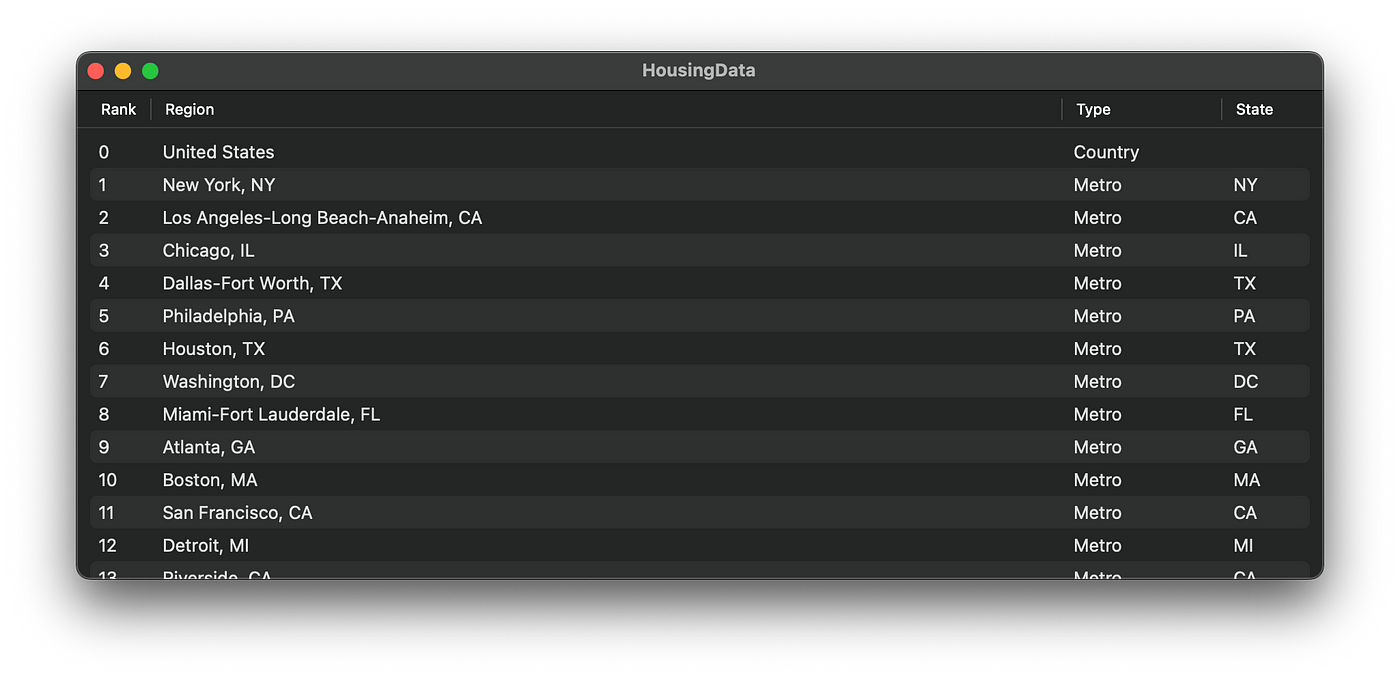
Dealing with thrown errors
To start with of the article I dealt with any errors from the information fetching with a print assertion that printed “oops”. I believe it could be higher to indicate an alert that shows a generic error.
First I’ll add a brand new non-public state variable that triggers the alert’s visibility.
The .activity
modifier must set the showError
flag to true when an error is caught. Simply setting the boolean works because the SwiftUI ensures that the view’s physique
is constructed on the principle thread.
To show the alert I’ll add a .alert
modifier to the VStack
. This can simply present a fundamental alert and dismiss it when the “OK” button is clicked.
The entire HousingDataView
view now seems like the next:
A little bit of refactoring
To wash up the code a bit I’ll transfer the listing and chart code into a few view builders.
Opening up the HousingDataView.swift
file I’ll create a brand new desk
view builder and transfer the desk creation code into there.
I will even create an empty chart
view builder that returns a easyRectangle
for now.
I’ll put these in a VSplitView.
This permits the person to manage how a lot of the tape and graph will be seen by dragging the splitter’s bar up and down. I’ll change the VStack
with the VSplitView
containing a name to chart
and desk
.
Desk Choice and Focus
I can not show all 900+ rows in chart. The chart can be unreadable and SwiftUI crashes when making an attempt to attract numerous knowledge units in a line graph. To get round this, I’ll restrict the variety of alternatives to 10. I’ll add a state variable to gather the chosen merchandise IDs.
An attention-grabbing drawback right here is that the alternatives are in a set, not an array. So the order of the choice can change as a brand new merchandise is added. There isn’t a easy strategy to repair this, with out an enormous quantity of reserving, since a Set
is an ordered assortment. I need to attempt to hold this so simple as potential.
I want to inform the desk the place to retailer the choice. I’ll level the choice to $selectedItems
in order that the desk will replace the chosen objects set every time an merchandise‘s choice has modified.
Yet one more factor that I need to do, after loading, is to verify the desk turns into the targeted view and that the primary merchandise is chosen. First I’ll add a spotlight state variable.
The desk wants a targeted
view modifier that tells the view its focus state based mostly on the inFocus
flag. Right here is the finished Desk
.
After the information is downloaded, I’ll replace the activity
modifier on the VSplitView
to pick out the primary merchandise within the listing and make the desk the targeted view.
Chart
I’ll create a easy line chart that simply attracts the factors for the chosen rows. One factor to recollect the rows are saved in a set which is required by Swift UI’s desk. So the order of the traces drawn might change since a set is a nonordered assortment.
I’ll wrap the rectangle in an if
assertion checking to see if there’s precise knowledge. If there isn’t a knowledge I’ll return an empty Rectangle
that will likely be 300 models excessive. In any other case, I’ll put in a chart with some padding and set the minimal and very best peak to 300 models.
For each chosen merchandise I’ll map every quantity. The X worth is the month and 12 months of the information. The Y worth would be the common dwelling value. I’ll let swift UI robotically coloration every line and legend worth based mostly on the row’s metropolis.
Limiting The Quantity Alternatives
On the prime of the article, I mentioned that I needed to restrict the variety of chosen objects to 10. I must retailer the final chosen rows that can be utilized the person makes an attempt to pick out greater than 10 objects. I’ll add a public lastSelection
property to the HousingDataModel
On the finish of the VStack
I’ll advert a modifier that known as when selectedItems
adjustments. The logic is straightforward:
- If the chosen merchandise depend is lower than, or equal to 10, retailer the choice because the final choice within the mannequin.
- If the chosen merchandise depend is bigger than 10, I restore the choice to the final set that was saved within the mannequin.
Operating the app now will load the most recent knowledge from Zillow and graph the chosen rows in our desk. In the event you see the order of alternatives not matching the order that was picked, keep in mind that is because of the desk choice being saved in an unordered set the place the order isn’t assured.
Even with the desk and chart limitations. It was simple to get a easy utility working that may obtain knowledge, show it in a desk with a number of choice and draw values in a chart. The ultimate mission will be discovered on GitHub.