On this article, we’ll take an in depth take a look at the construction of a wise contract (docs).
💡 We’ll study state variables, capabilities, perform modifiers, occasions, errors, struct sorts, and enum sorts.
We now have acknowledged most of those parts in motion within the earlier articles, particularly within the ones the place we had actual examples of sensible contracts.
Constructing on the beforehand seen context of use, we’ll spend a couple of extra phrases on every of them and supply an extra context of their applicability. It’s all the time helpful to know the proverbial nuts and bolts of the stuff we’re working with, in addition to stretch our creativeness for presumably different makes use of than simply the most typical ones.
Construction of a Contract
For these of us who’ve some expertise with programming with object-oriented languages, OOL, there’s a noticeable similarity between sensible contracts and courses in OOL.
As talked about within the introduction, contracts might include any of the state variables, capabilities, perform modifiers, occasions, errors, struct sorts, and enum sorts.
There’s a helpful Solidity characteristic we haven’t used earlier than, and it permits us to kind a brand new sensible contract primarily based on another, extra normal however helpful contract. This characteristic known as inheritance. We’ll simply contact on the ideas on this article however may even go into additional element in a future one.
Data: “Object-oriented language (OOL) is a high-level pc programming language that implements objects and their related procedures throughout the programming context to create software program packages. Object-oriented language makes use of an object-oriented programming method that binds associated knowledge and capabilities into an object and encourages reuse of those objects throughout the identical and different packages.”
Different particular sorts of contracts are libraries and interfaces.
- 📚 Libraries are contracts which can be deployed solely as soon as at a selected tackle (similar to a brick-and-mortar library in the true world), after which their “data”, i.e. performance and knowledge buildings are reused at any time when wanted.
- 🖥️ Interfaces seem like summary contracts, however they can not implement any capabilities. As an alternative, they include solely the declarations of knowledge buildings and performance signatures, instructing an inheriting contract on how the perform ought to look when it’s carried out.
State Variables
State variables, or extra typically, objects maintain the values which can be completely saved within the contract (EVM) reminiscence space known as storage. As a reminder, there are three reminiscence areas: storage, reminiscence, name knowledge, plus stack.
An instance of a state variable declaration seems to be like this:
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.4.0 <0.9.0; contract SimpleStorage { uint storedData; // State variable // ... }
Features
Features are constructs of a sensible contract that give it the specified conduct, or in different phrases, capabilities are executable items of code.
They are often outlined inside or outdoors a contract, relying on the undertaking construction and conduct necessities.
Right here we now have an instance of a easy helper perform (a continuously used utility perform) that simply takes an argument, multiplies it by 2, and returns the end result to the caller would seem like this:
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.7.1 <0.9.0; contract SimpleAuction { perform bid() public payable { // Operate // ... } } // Helper perform outlined outdoors of a contract perform helper(uint x) pure returns (uint) { return x * 2; }
The perform definition says a couple of extra issues concerning the perform, aside from its conduct. The perform is outlined as pure, which means it can’t entry any of the state variables, i.e. its outer context is made unavailable. the perform returns a results of sort uint
, i.e. the unsigned integer.
Operate calls could be made both from different sensible contracts or shoppers, which we acknowledge as exterior perform calls, or from different same-contract capabilities, which we acknowledge as inside perform calls.
In addition to, capabilities could be outlined with one of many a number of completely different ranges of visibility in direction of different contacts. Generally, capabilities are outlined with (enter) parameters (also called formal parameters, variables, or named placeholders) and settle for arguments of their place (precise parameters, actual values).
With that in thoughts, we see that capabilities talk with one another by accepting arguments and returning values.
Operate Modifiers
Based on the official Solidity documentation, “Operate modifiers can be utilized to amend the semantics of capabilities in a declarative means”.
Because of this the perform definition is supplemented with a perform modifier that moreover defines how a perform can work together with its different context.
ℹ️ Data: Semantics is the department of linguistics and logic involved with which means, branching into two primary areas: logical semantics and lexical semantics. Lexical semantics is anxious with the evaluation of phrase meanings and relations between them.
💡 Data: Declarative means expresses what needs to be completed, or the specified conduct, with out specifying the way it needs to be achieved or carried out (crucial).
The definition above implies that we will use one of many perform modifiers to vary the best way the perform behaves by way of studying and modifying the state variables, solely studying the state variables, or being remoted from the state variables, i.e. working solely with its enter arguments.
Occasions
Occasions are mostly described as comfort interfaces with the EVM logging services.
In case this description appears a bit obscure or dry, let’s put it in different phrases: occasions allow us as builders to deploy sign sending from contract capabilities and use these indicators to watch the contract.
👀 Contract monitoring is beneficial in a number of methods, e.g. we will use them to set off an motion upon receiving the sign, forming a series of actions, throughout the debugging course of, or simply to watch and even learn the way a wise contract works.
Extra on occasions could be discovered following the hyperlink: https://docs.soliditylang.org/en/v0.8.15/contracts.html#occasions.
Errors
Error constructs signify a helpful and easy method that permits us to outline descriptive names and knowledge for circumstances when an error happens.
As proven in earlier articles on Solidity supply code examples, error constructs are generally used with revert statements to help in clarifying the reason for an error and, consequentially, the state reversal.
Through the use of error constructs, we will exactly decide the main points of an error, the error identify, and the information that brought on it. Errors use fewer assets than string descriptions and permit us to encode further knowledge. Much more, we will use NatSpec to explain the error.
An instance of an error assemble is:
// SPDX-License-Identifier: GPL-3.0 pragma solidity ^0.8.4; /// Not sufficient funds for switch. Requested `requested`, /// however solely `accessible` accessible. error NotEnoughFunds(uint requested, uint accessible); contract Token { mapping(tackle => uint) balances; perform switch(tackle to, uint quantity) public { uint steadiness = balances[msg.sender]; if (steadiness < quantity) revert NotEnoughFunds(quantity, steadiness); balances[msg.sender] -= quantity; balances[to] += quantity; // ... } }
Struct Varieties
Struct sorts are Solidity knowledge sorts that we outline once we want a customized, advanced knowledge sort.
Struct sorts are fashioned by grouping variables collectively and utilized by instancing the customized struct sort later within the supply code:
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.4.0 <0.9.0; contract Poll { struct Voter { // Struct uint weight; bool voted; tackle delegate; uint vote; } }
Enum Varieties
Enum sorts are particular customized sorts which can be primarily based on a finite, restricted set of fixed values. In our instance a couple of articles earlier than, we used the Enum sort for preserving monitor of a state our contract is in:
// SPDX-License-Identifier: GPL-3.0 pragma solidity >=0.4.0 <0.9.0; contract Buy { enum State { Created, Locked, Inactive } // Enum }
Conclusion
All through this text, we made an extra step in familiarizing ourselves with the essential parts of the Solidity programming language.
As we advance our data, we’ll get to know every of the talked about parts even higher. To this point, we went by means of a number of, vital sections.
First, we had a short overview of the construction of a wise contract.
Second, we targeted on the state variables and reminded ourselves what they’re.
Third, we formally refreshed our data on the subject of capabilities.
Fourth, a pleasant reminder of the perform modifiers, together with a couple of definitions blazed in entrance of our eyes.
Fifth, though it wasn’t a fancy occasion, it was an fascinating refresher on how can we set them up! Simply keep in mind, gown appropriately 🙂
Sixth, errors are frequent in life, since no person’s good. The right way to deal with errors is a completely completely different factor. Now we all know how and are able to face them!
Seventh, what ought to we do with struct sorts? We realized that trick, let’s arrange a lure and use it to seize customized knowledge construction.
Eighth, we realized what enum sorts are and methods to use them to maintain a report of our sensible contract’s present state.
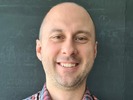
I’m an skilled pc science engineer and expertise fanatic devoted to understanding how the world works and utilizing my data and talent to advance it. I’m targeted on changing into an skilled in Solidity and crypto expertise, with a ardour for coding, studying, and contributing to the Finxter mission of accelerating the collective intelligence of humanity.