Prelude
That is the second publish in a 3 half sequence that can present an understanding of the mechanics and semantics behind the scheduler in Go. This publish focuses on the Go scheduler.
Index of the three half sequence:
1) Scheduling In Go : Half I – OS Scheduler
2) Scheduling In Go : Half II – Go Scheduler
3) Scheduling In Go : Half III – Concurrency
Introduction
Within the first half of this scheduling sequence, I defined features of the operating-system scheduler that I consider are essential in understanding and appreciating the semantics of the Go scheduler. On this publish, I’ll clarify at a semantic stage how the Go scheduler works and deal with the high-level behaviors. The Go scheduler is a posh system and the little mechanical particulars are usually not essential. What’s essential is having a very good mannequin for a way issues work and behave. This may permit you to make higher engineering selections.
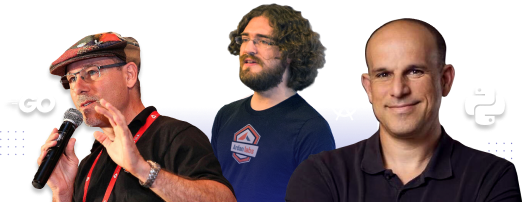
Your Program Begins
When your Go program begins up, it’s given a Logical Processor (P) for each digital core that’s recognized on the host machine. In case you have a processor with a number of {hardware} threads per bodily core (Hyper-Threading), every {hardware} thread can be introduced to your Go program as a digital core. To raised perceive this, check out the system report for my MacBook Professional.
Determine 1
You possibly can see I’ve a single processor with 4 bodily cores. What this report will not be exposing is the variety of {hardware} threads I’ve per bodily core. The Intel Core i7 processor has Hyper-Threading, which suggests there are 2 {hardware} threads per bodily core. This may report back to the Go program that 8 digital cores can be found for executing OS Threads in parallel.
To check this, contemplate the next program:
Itemizing 1
bundle principal
import (
"fmt"
"runtime"
)
func principal() {
// NumCPU returns the variety of logical
// CPUs usable by the present course of.
fmt.Println(runtime.NumCPU())
}
Once I run this program on my native machine, the results of the NumCPU() perform name would be the worth of 8. Any Go program I run on my machine can be given 8 P’s.
Each P is assigned an OS Thread (“M”). The ‘M’ stands for machine. This Thread remains to be managed by the OS and the OS remains to be chargeable for inserting the Thread on a Core for execution, as defined within the final publish. This implies after I run a Go program on my machine, I’ve 8 threads accessible to execute my work, every individually connected to a P.
Each Go program can also be given an preliminary Goroutine (“G”), which is the trail of execution for a Go program. A Goroutine is actually a Coroutine however that is Go, so we change the letter “C” with a “G” and we get the phrase Goroutine. You possibly can consider Goroutines as application-level threads and they’re much like OS Threads in some ways. Simply as OS Threads are context-switched on and off a core, Goroutines are context-switched on and off an M.
The final piece of the puzzle is the run queues. There are two completely different run queues within the Go scheduler: the World Run Queue (GRQ) and the Native Run Queue (LRQ). Every P is given a LRQ that manages the Goroutines assigned to be executed throughout the context of a P. These Goroutines take turns being context-switched on and off the M assigned to that P. The GRQ is for Goroutines that haven’t been assigned to a P but. There’s a course of to maneuver Goroutines from the GRQ to a LRQ that we are going to focus on later.
Determine 2 offers a picture of all these elements collectively.
Determine 2
Cooperating Scheduler
As we mentioned within the first publish, the OS scheduler is a preemptive scheduler. Basically which means you’ll be able to’t predict what the scheduler goes to do at any given time. The kernel is making selections and the whole lot is non-deterministic. Purposes that run on prime of the OS haven’t any management over what is going on contained in the kernel with scheduling except they leverage synchronization primitives like atomic directions and mutex calls.
The Go scheduler is a part of the Go runtime, and the Go runtime is constructed into your utility. This implies the Go scheduler runs in person house, above the kernel. The present implementation of the Go scheduler will not be a preemptive scheduler however a cooperating scheduler. Being a cooperating scheduler means the scheduler wants well-defined person house occasions that occur at secure factors within the code to make scheduling selections.
What’s sensible concerning the Go cooperating scheduler is that it appears and feels preemptive. You possibly can’t predict what the Go scheduler goes to do. It’s because choice making for this cooperating scheduler doesn’t relaxation within the fingers of builders, however within the Go runtime. It’s essential to think about the Go scheduler as a preemptive scheduler and because the scheduler is non-deterministic, this isn’t a lot of a stretch.
Goroutine States
Similar to Threads, Goroutines have the identical three high-level states. These dictate the position the Go scheduler takes with any given Goroutine. A Goroutine might be in one in every of three states: Ready, Runnable or Executing.
Ready: This implies the Goroutine is stopped and ready for one thing as a way to proceed. This could possibly be for causes like ready for the working system (system calls) or synchronization calls (atomic and mutex operations). These kind of latencies are a root trigger for dangerous efficiency.
Runnable: This implies the Goroutine needs time on an M so it could possibly execute its assigned directions. In case you have a whole lot of Goroutines that need time, then Goroutines have to attend longer to get time. Additionally, the person period of time any given Goroutine will get is shortened as extra Goroutines compete for time. This kind of scheduling latency can be a explanation for dangerous efficiency.
Executing: This implies the Goroutine has been positioned on an M and is executing its directions. The work associated to the appliance is getting completed. That is what everybody needs.
Context Switching
The Go scheduler requires well-defined user-space occasions that happen at secure factors within the code to context-switch from. These occasions and secure factors manifest themselves inside perform calls. Operate calls are vital to the well being of the Go scheduler. At this time (with Go 1.11 or much less), in the event you run any tight loops that aren’t making perform calls, you’ll trigger latencies throughout the scheduler and rubbish assortment. It’s critically essential that perform calls occur inside affordable timeframes.
Observe: There’s a proposal for 1.12 that was accepted to use non-cooperative preemption methods contained in the Go scheduler to permit for the preemption of tight loops.
There are 4 lessons of occasions that happen in your Go applications that permit the scheduler to make scheduling selections. This doesn’t imply it’ll all the time occur on one in every of these occasions. It means the scheduler will get the chance.
- The usage of the key phrase
go
- Rubbish assortment
- System calls
- Synchronization and Orchestration
The usage of the key phrase go
The key phrase go
is the way you create Goroutines. As soon as a brand new Goroutine is created, it provides the scheduler a possibility to make a scheduling choice.
Rubbish assortment
Because the GC runs utilizing its personal set of Goroutines, these Goroutines want time on an M to run. This causes the GC to create a whole lot of scheduling chaos. Nonetheless, the scheduler could be very sensible about what a Goroutine is doing and it’ll leverage that intelligence to make sensible selections. One smart move is context-switching a Goroutine that wishes to the touch the heap with those who don’t contact the heap throughout GC. When GC is working, a whole lot of scheduling selections are being made.
System calls
If a Goroutine makes a system name that can trigger the Goroutine to dam the M, generally the scheduler is able to context-switching the Goroutine off the M and context-switch a brand new Goroutine onto that very same M. Nonetheless, generally a brand new M is required to maintain executing Goroutines which can be queued up within the P. How this works can be defined in additional element within the subsequent part.
Synchronization and Orchestration
If an atomic, mutex, or channel operation name will trigger the Goroutine to dam, the scheduler can context-switch a brand new Goroutine to run. As soon as the Goroutine can run once more, it may be re-queued and finally context-switched again on an M.
Asynchronous System Calls
When the OS you might be working on has the power to deal with a system name asynchronously, one thing referred to as the community poller can be utilized to course of the system name extra effectively. That is achieved by utilizing kqueue (MacOS), epoll (Linux) or iocp (Home windows) inside these respective OS’s.
Networking-based system calls might be processed asynchronously by most of the OSs we use in the present day. That is the place the community poller will get its title, since its major use is dealing with networking operations. By utilizing the community poller for networking system calls, the scheduler can stop Goroutines from blocking the M when these system calls are made. This helps to maintain the M accessible to execute different Goroutines within the P’s LRQ with out the necessity to create new Ms. This helps to scale back scheduling load on the OS.
One of the best ways to see how this works is to run via an instance.
Determine 3
Determine 3 exhibits our base scheduling diagram. Goroutine-1 is executing on the M and there are 3 extra Goroutines ready within the LRQ to get their time on the M. The community poller is idle with nothing to do.
Determine 4
In determine 4, Goroutine-1 needs to make a community system name, so Goroutine-1 is moved to the community poller and the asynchronous community system name is processed. As soon as Goroutine-1 is moved to the community poller, the M is now accessible to execute a unique Goroutine from the LRQ. On this case, Goroutine-2 is context-switched on the M.
Determine 5
In determine 5, the asynchronous community system name is accomplished by the community poller and Goroutine-1 is moved again into the LRQ for the P. As soon as Goroutine-1 might be context-switched again on the M, the Go associated code it’s chargeable for can execute once more. The large win right here is that, to execute community system calls, no further Ms are wanted. The community poller has an OS Thread and it’s dealing with an environment friendly occasion loop.
Synchronous System Calls
What occurs when the Goroutine needs to make a system name that may’t be completed asynchronously? On this case, the community poller can’t be used and the Goroutine making the system name goes to dam the M. That is unlucky however there’s no technique to stop this from taking place. One instance of a system name that may’t be made asynchronously is file-based system calls. If you’re utilizing CGO, there could also be different conditions the place calling C features will block the M as properly.
Observe: The Home windows OS does have the aptitude of constructing file-based system calls asynchronously. Technically when working on Home windows, the community poller can be utilized.
Let’s stroll via what occurs with a synchronous system name (like file I/O) that can trigger the M to dam.
Determine 6
Determine 6 is displaying our fundamental scheduling diagram once more however this time Goroutine-1 goes to make a synchronous system name that can block M1.
Determine 7
In determine 7, the scheduler is ready to establish that Goroutine-1 has triggered the M to dam. At this level, the scheduler detaches M1 from the P with the blocking Goroutine-1 nonetheless connected. Then the scheduler brings in a brand new M2 to service the P. At that time, Goroutine-2 might be chosen from the LRQ and context-switched on M2. If an M already exists due to a earlier swap, this transition is faster than having to create a brand new M.
Determine 8
In determine 8, the blocking system name that was made by Goroutine-1 finishes. At this level, Goroutine-1 can transfer again into the LRQ and be serviced by the P once more. M1 is then positioned on the aspect for future use if this situation must occur once more.
Work Stealing
One other side of the scheduler is that it’s a work-stealing scheduler. This helps in a couple of areas to maintain scheduling environment friendly. For one, the very last thing you need is an M to maneuver right into a ready state as a result of, as soon as that occurs, the OS will context-switch the M off the Core. This implies the P can’t get any work completed, even when there’s a Goroutine in a runnable state, till an M is context-switched again on a Core. The work stealing additionally helps to steadiness the Goroutines throughout all of the P’s so the work is best distributed and getting completed extra effectively.
Let’s run via an instance.
Determine 9
In determine 9, we’ve got a multi-threaded Go program with two P’s servicing 4 Goroutines every and a single Goroutine within the GRQ. What occurs if one of many P’s providers all of its Goroutines rapidly?
Determine 10
In determine 10, P1 has no extra Goroutines to execute. However there are Goroutines in a runnable state, each within the LRQ for P2 and within the GRQ. It is a second the place P1 must steal work. The principles for stealing work are as follows.
Itemizing 2
runtime.schedule() {
// only one/61 of the time, examine the worldwide runnable queue for a G.
// if not discovered, examine the native queue.
// if not discovered,
// attempt to steal from different Ps.
// if not, examine the worldwide runnable queue.
// if not discovered, ballot community.
}
So based mostly on these guidelines in Itemizing 2, P1 must examine P2 for Goroutines in its LRQ and take half of what it finds.
Determine 11
In determine 11, half the Goroutines are taken from P2 and now P1 can execute these Goroutines.
What occurs if P2 finishes servicing all of its Goroutines and P1 has nothing left in its LRQ?
Determine 12
In determine 12, P2 completed all its work and now must steal some. First, it’ll take a look at the LRQ of P1 but it surely received’t discover any Goroutines. Subsequent, it’ll take a look at the GRQ. There it’ll discover Goroutine-9.
Determine 13
In determine 13, P2 steals Goroutine-9 from the GRQ and begins to execute the work. What’s nice about all this work stealing is that it permits the Ms to remain busy and never go idle. This work stealing is taken into account internally as spinning the M. This spinning has different advantages that JBD explains properly in her work-stealing weblog publish.
Sensible Instance
With the mechanics and semantics in place, I need to present you ways all of this comes collectively to permit the Go scheduler to execute extra work over time. Think about a multi-threaded utility written in C the place this system is managing two OS Threads which can be passing messages backwards and forwards to one another.
Determine 14
In determine 14, there are 2 Threads which can be passing a message backwards and forwards. Thread 1 will get context-switched on Core 1 and is now executing, which permits Thread 1 to ship its message to Thread 2.
Observe: How the message is being handed is unimportant. What’s essential is the state of the Threads as this orchestration proceeds.
Determine 15
In determine 15, as soon as Thread 1 finishes sending the message, it now wants to attend for the response. This may trigger Thread 1 to be context-switched off Core 1 and moved right into a ready state. As soon as Thread 2 is notified concerning the message, it strikes right into a runnable state. Now the OS can carry out a context change and get Thread 2 executing on a Core, which it occurs to be Core 2. Subsequent, Thread 2 processes the message and sends a brand new message again to Thread 1.
Determine 16
In determine 16, Threads context-switch as soon as once more because the message by Thread 2 is obtained by Thread 1. Now Thread 2 context-switches from an executing state to a ready state and Thread 1 context-switches from a ready state to a runnable state and eventually again to an executing state, which permits it to course of and ship a brand new message again.
All these context switches and state adjustments require time to be carried out which limits how briskly the work can get completed. With every context-switching potential incurring a latency of ~1000 nanoseconds, and hopefully the {hardware} executing 12 directions per nanosecond, you’re looking at 12k directions, roughly, not executing throughout these context switches. Since these Threads are additionally bouncing between completely different Cores, the possibilities of incurring extra latency as a consequence of cache-line misses are additionally excessive.
Let’s take this similar instance however use Goroutines and the Go scheduler as a substitute.
Determine 17
In determine 17, there are two Goroutines which can be in orchestration with one another passing a message backwards and forwards. G1 will get context-switched on M1, which occurs to be working on Core 1, which permits G1 to be executing its work. The work is for G1 to ship its message to G2.
Determine 18
In determine 18, as soon as G1 finishes sending the message, it now wants to attend for the response. This may trigger G1 to be context-switched off M1 and moved right into a ready state. As soon as G2 is notified concerning the message, it strikes right into a runnable state. Now the Go scheduler can carry out a context change and get G2 executing on M1, which remains to be working on Core 1. Subsequent, G2 processes the message and sends a brand new message again to G1.
Determine 19
In determine 19, issues context-switch as soon as once more because the message despatched by G2 is obtained by G1. Now G2 context-switches from an executing state to a ready state and G1 context-switches from a ready state to a runnable state and eventually again to an executing state, which permits it to course of and ship a brand new message again.
Issues on the floor don’t look like any completely different. All the identical context switches and state adjustments are occuring whether or not you utilize Threads or Goroutines. Nonetheless, there’s a main distinction between utilizing Threads and Goroutines which may not be apparent at first look.
Within the case of utilizing Goroutines, the identical OS Thread and Core is getting used for all of the processing. Because of this, from the OS’s perspective, the OS Thread by no means strikes right into a ready state; not as soon as. Because of this all these directions we misplaced to context switches when utilizing Threads are usually not misplaced when utilizing Goroutines.
Basically, Go has turned IO/Blocking work into CPU-bound work on the OS stage. Since all of the context switching is going on on the utility stage, we don’t lose the identical ~12k directions (on common) per context change that we have been dropping when utilizing Threads. In Go, those self same context switches are costing you ~200 nanoseconds or ~2.4k directions. The scheduler can also be serving to with features on cache-line efficiencies and NUMA. For this reason we don’t want extra Threads than we’ve got digital cores. In Go, it’s doable to get extra work completed, over time, as a result of the Go scheduler makes an attempt to make use of much less Threads and do extra on every Thread, which helps to scale back load on the OS and the {hardware}.
Conclusion
The Go scheduler is basically superb in how the design takes under consideration the intricacies of how the OS and the {hardware} work. The flexibility to show IO/Blocking work into CPU-bound work on the OS stage is the place we get an enormous win in leveraging extra CPU capability over time. For this reason you don’t want extra OS Threads than you will have digital cores. You possibly can moderately anticipate to get your whole work completed (CPU and IO/Blocking sure) with only one OS Thread per digital core. Doing so is feasible for networking apps and different apps that don’t want system calls that block OS Threads.
As a developer, you continue to want to know what your app is doing when it comes to the sorts of labor you might be processing. You possibly can’t create an infinite variety of Goroutines and anticipate superb efficiency. Much less is all the time extra, however with the understanding of those Go-scheduler semantics, you may make higher engineering selections. Within the subsequent publish, I’ll discover this concept of leveraging concurrency in conservative methods to achieve higher efficiency whereas nonetheless balancing the quantity of complexity chances are you’ll want so as to add to the code.