Ruby-on-Rails has no built-in authentication mechanism. So let’s choose the well-known gem Devise on this tutorial.
Rails authentication
Ruby-on-Rails lack of inside, built-in authentication mechanism is one thing typically claimed by developer for the subsequent Rails model.
Devise appears the go-to, default gem for authentication, regardless of being closely mentioned on boards (like this Reddit dialogue).
For bootrails we now have chosen Rodauth, which is absolutely good.
However this text, I’ll attempt to current you the performance of the Devise gem through a standard check case: a fast authentication with username and password
Setup an empty Rails app
We assume you’re utilizing an Ubuntu Linux distribution with the next put in:
So let’s create an empty Rails utility.
Conditions
To comply with this text you’ll need to test you probably have put in the conditions, if one thing is lacking that you must set up it first to proceed.
$/workspace> ruby -v
ruby 3.1.2p20 // you need to have no less than model 3
$/workspace> rails -v
Rails 7.0.3.1
$/workspace> bundle -v
Bundler model 2.3.7 // you need to have no less than model 2
How do I get arrange with Rails?
Since this can be a hands-on tutorial we must always begin by creating an empty Rails utility and we will construct upon it.
Let’s assume that we’ll have one controller in our utility, the PagesController, which is able to deal with all requests coming for our two-page web site:
As you’ll be able to think about we have to management the entry to the “Unique content material” to solely the registered customers whereas the house web page will likely be accessible by anybody.
// Create a brand new Rails app
$/workspace> rails new my-app
// Enter the newly created folder
$/workspace> cd my-app
// Create our 'PagesController' controller with the 'unique' view
$/workspace/my-app> bin/rails generate controller PagesController unique
How do I get arrange with Devise?
Firstly open up along with your editor your Gemfile and add this:
gem 'devise'
then run these:
// add the gem to the dependencies
$/workspace/my-app> bundle set up
// set up the gem
$/workspace/my-app> bin/rails g devise:set up
// create the Consumer mannequin
$/workspace/my-app> bin/rails g devise consumer
// Run the DB Migration
$/workspace/my-app> bin/rails db:migrate
Verify the work to this point
Run the event server
// begin the Puma built-in internet server for testing
$/workspace/my-app> bin/rails server
and level your browser to the next URL: http://localhost:3000. It is best to see one thing like this:
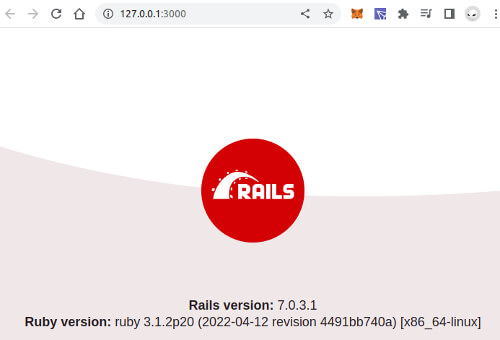
Attempt to go to additionally the Unique content material web page http://localhost:3000/pages/unique, you’ll be able to see it with none drawback, proper?
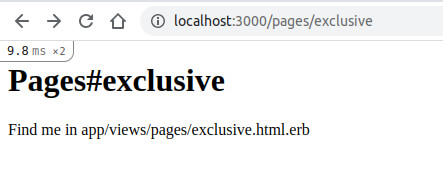
// shut the online server
$/workspace/my-app> Ctr-C
Welcome to the Devise world!
The Devise gem will create all of the required code (Mannequin, DB migrations) and routes to create consumer accounts, sign up, signal out, reset passwords, and so forth.
What choices do I’ve with Devise
The next primary routes have already been created:
You even have a Consumer mannequin able to help the dealing with of the customers of your utility.
class Consumer < ApplicationRecord
# Embrace default devise modules. Others obtainable are:
# :confirmable, :lockable, :timeoutable, :trackable and :omniauthable
devise :database_authenticatable, :registerable,
:recoverable, :rememberable, :validatable
finish
Devise will present additionally the choice:
- to confirm if a consumer is signed in through the use of the next helper: user_signed_in?
- to entry the present signed-in consumer through the use of: current_user
- or to entry the session through: user_session
Create a consumer for our testing
Level your browser at: http://localhost:3000/customers/sign_up
and create a brand new consumer. A easy kind like this will likely be introduced:
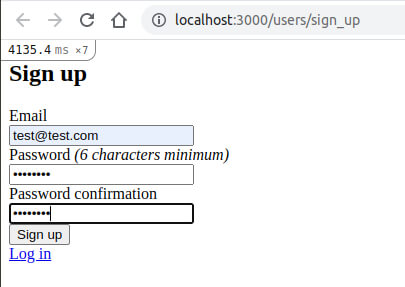
simply set a demo e mail and a password for authentication. For instance:
consumer: check@check.com
go: password
Let’s add additionally the principle hyperlinks of SignUp, SignIn, and Logout
Along with your editor open the format file: my-app/app/views/layouts/utility.html.rb
and add this:
<div>
<% if user_signed_in? %>
Logged in as <robust><%= current_user.e mail %></robust>.
<%= link_to "Logout", destroy_user_session_path, methodology: :delete, :class => 'navbar-link' %>
<% else %>
<%= link_to "Enroll", new_user_registration_path, :class => 'navbar-link' %> |
<%= link_to "Sign up", new_user_session_path, :class => 'navbar-link' %>
<% finish %>
</div>
Like this:
<!DOCTYPE html>
<html>
<head>
<title>MyApp</title>
<meta identify="viewport" content material="width=device-width,initial-scale=1">
<%= csrf_meta_tags %>
<%= csp_meta_tag %>
<%= stylesheet_link_tag 'utility', media: 'all', 'data-turbolinks-track': 'reload' %>
<%= javascript_pack_tag 'utility', 'data-turbolinks-track': 'reload' %>
</head>
<physique>
<div>
<% if user_signed_in? %>
Logged in as <robust><%= current_user.e mail %></robust>.
<%= link_to "Logout", destroy_user_session_path, methodology: :delete, :class => 'navbar-link' %>
<% else %>
<%= link_to "Enroll", new_user_registration_path, :class => 'navbar-link' %> |
<%= link_to "Sign up", new_user_session_path, :class => 'navbar-link' %>
<% finish %>
</div>
<%= yield %>
</physique>
</html>
A easy consumer authentication
As we wrote earlier the web page with the “Unique content material” accommodates some content material that have to be accessible solely to registered customers. A easy Register and Login/Logout performance is ample. So The place do we start?
You guessed appropriately! No want to put in writing any further code for the widespread performance above.
Open along with your editor the my-app/app/controllers/pages_controller.rb
and add this line:
before_action :authenticate_user!
class PagesController < ApplicationController
before_action :authenticate_user!
def unique
finish
finish
Attempt to go to now the Unique content material web page http://localhost:3000/pages/unique, you can’t see it now, proper? It redirects you to the login web page.
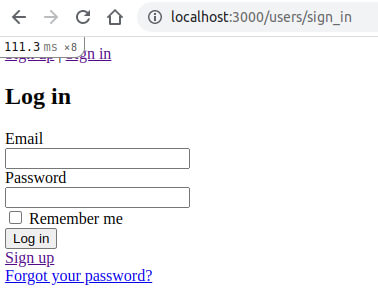
Use the consumer credentials you simply created and you’ll be redirected to the unique content material. You possibly can see now on the high the Logout possibility.
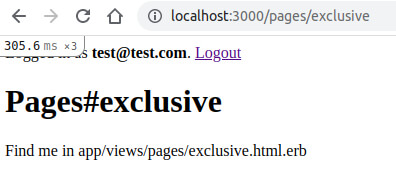
The place you’ll be able to go from right here
You possibly can all the time enhance and broaden on current code, listed here are some concepts:
- change the Consumer mannequin and add your code to reinforce it.
- add Roles and Permissions and join them with the consumer Mannequin.
- change the styling and the construction of the scaffolded varieties to fill your necessities.
The above check case is an easy one however certainly you’ll face extra advanced conditions in real-life internet functions. These days the necessities are greater and internet functions are getting extra advanced. Contemplate that:
- you’ll be able to implement a 2FA Authentication
- or implement authentication as properly for API’s particular endpoints.
These might be supported by the Devise gem ( you’ll be able to be taught extra in regards to the Devise gem by studying their documentation ) however we are going to write about them in future articles! 😉
Conclusion
Congratulations! You have got simply created an internet utility with consumer authentication in a couple of minutes. Keep in mind that you’ve got simply tasted a small piece of the capabilities of the devise gem. Continue to learn!
As you’ll be able to see with just a few instructions you’ll be able to construct a really highly effective authentication system. The Devise ecosystem supplies the flexibleness to a developer to neglect about trivial issues like consumer authentication and focus extra on the core functionalities of the online utility.
Get pleasure from!!