💡 Drawback Formulation: In numerous programming duties, we could also be required to type a listing of strings not solely based mostly on alphabetical order but additionally by the size of every string.
Suppose we have now a listing of strings like ["banana", "apple", "cherry", "blueberry"]
.
We wish to manage this listing in order that it’s sorted by the size of every string and, for strings of the identical size, type them alphabetically.
The specified output for this listing could be ["apple", "banana", "cherry", "blueberry"]
.
For those who solely wish to type by size, try my article right here:
👉 Python Type Listing of Strings by Size
Methodology 1: Utilizing a Customized Sorting Perform
Python’s type()
technique can take a customized sorting operate by its ‘key’ parameter. This operate is usually a lambda operate that returns a tuple the place the primary factor is the size of the string, and the second is the string itself, establishing a form precedence.
🖨️ Really useful Tech Product: Try this insane 3D printer for lower than $200: Amazon Affiliate Hyperlink, >20k five-star scores ⭐⭐⭐⭐⭐
Right here’s an instance:
fruits = ["banana", "apple", "cherry", "blueberry"] fruits.type(key=lambda merchandise: (len(merchandise), merchandise)) print(fruits)
This code snippet types the listing of fruits by size after which alphabetically. The lambda
operate returns a tuple the place the primary factor is the size of the string, guaranteeing major sorting by size, and the second factor is the string, which types the same-length strings alphabetically.
Methodology 2: Utilizing the sorted() Perform with a Customized Key
The sorted()
operate in Python works equally to the type()
technique however returns a brand new sorted listing. You possibly can present a key operate that, once more, returns a tuple, first of the size, then the string itself.
Right here’s an instance:
fruits = ["banana", "apple", "cherry", "blueberry"] sorted_fruits = sorted(fruits, key=lambda merchandise: (len(merchandise), merchandise)) print(sorted_fruits)
On this code snippet, the listing of fruits is sorted by size and alphabetically with out altering the unique listing, because the sorted()
operate creates a brand new sorted listing. The customized key operate operates the identical approach as in Methodology 1.
Methodology 3: Utilizing an Inline Perform Definition
As an alternative of a lambda, you possibly can outline an inline operate utilizing def
, which may enhance readability when the sorting standards are advanced or if you happen to’re avoiding lambda capabilities for stylistic causes.
Right here’s an instance:
fruits = ["banana", "apple", "cherry", "blueberry"] def sort_key(merchandise): return len(merchandise), merchandise fruits.type(key=sort_key) print(fruits)
The code snippet above defines a sorting key operate sort_key
that returns a tuple based mostly on the string’s size after which the string itself. The type()
technique types the listing in-place utilizing this operate.
Methodology 4: Utilizing Operator.itemgetter for Combined Sorting
For circumstances the place you’re sorting objects or tuples and never simply strings, operator.itemgetter
might be helpful. It may be utilized in mixture with sorted()
or type()
to type the strings by size after which alphabetically if you happen to first convert strings to tuples containing their size.
Right here’s an instance:
from operator import itemgetter fruits = ["banana", "apple", "cherry", "blueberry"] tuples = [(len(fruit), fruit) for fruit in fruits] sorted_tuples = sorted(tuples, key=itemgetter(0, 1)) sorted_fruits = [fruit for _, fruit in sorted_tuples] print(sorted_fruits)
This snippet first converts the fruit strings into tuples of (size, string)
, types the listing of tuples, after which extracts the sorted strings. itemgetter(0, 1)
specifies the tuple indices by which to type.
Bonus One-Liner Methodology 5: Combining sorted() and Listing Comprehension
For those who fancy one-liners, you possibly can mix sorted()
with a listing comprehension to attain the identical outcome, albeit much less readable for some.
Right here’s an instance:
fruits = ["banana", "apple", "cherry", "blueberry"] print([fruit for _, fruit in sorted((len(fruit), fruit) for fruit in fruits)])
This advanced one-liner creates a generator that produces tuples (size, string)
, types them, after which extracts the second factor of every tuple to kind the sorted listing of strings.
Python One-Liners E book: Grasp the Single Line First!
Python programmers will enhance their laptop science expertise with these helpful one-liners.
Python One-Liners will train you the best way to learn and write “one-liners”: concise statements of helpful performance packed right into a single line of code. You’ll discover ways to systematically unpack and perceive any line of Python code, and write eloquent, powerfully compressed Python like an skilled.
The guide’s 5 chapters cowl (1) ideas and methods, (2) common expressions, (3) machine studying, (4) core knowledge science matters, and (5) helpful algorithms.
Detailed explanations of one-liners introduce key laptop science ideas and increase your coding and analytical expertise. You’ll find out about superior Python options equivalent to listing comprehension, slicing, lambda capabilities, common expressions, map and cut back capabilities, and slice assignments.
You’ll additionally discover ways to:
- Leverage knowledge buildings to resolve real-world issues, like utilizing Boolean indexing to search out cities with above-average air pollution
- Use NumPy fundamentals equivalent to array, form, axis, sort, broadcasting, superior indexing, slicing, sorting, looking, aggregating, and statistics
- Calculate primary statistics of multidimensional knowledge arrays and the Okay-Means algorithms for unsupervised studying
- Create extra superior common expressions utilizing grouping and named teams, detrimental lookaheads, escaped characters, whitespaces, character units (and detrimental characters units), and grasping/nongreedy operators
- Perceive a variety of laptop science matters, together with anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, looking, and algorithmic sorting
By the top of the guide, you’ll know the best way to write Python at its most refined, and create concise, lovely items of “Python artwork” in merely a single line.
Get your Python One-Liners on Amazon!!
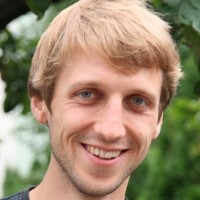
Whereas working as a researcher in distributed methods, Dr. Christian Mayer discovered his love for instructing laptop science college students.
To assist college students attain increased ranges of Python success, he based the programming training web site Finxter.com that has taught exponential expertise to thousands and thousands of coders worldwide. He’s the writer of the best-selling programming books Python One-Liners (NoStarch 2020), The Artwork of Clear Code (NoStarch 2022), and The E book of Sprint (NoStarch 2022). Chris additionally coauthored the Espresso Break Python collection of self-published books. He’s a pc science fanatic, freelancer, and proprietor of one of many high 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his best ardour is to serve aspiring coders by Finxter and assist them to spice up their expertise. You possibly can be part of his free e mail academy right here.