Python lists are used to retailer a number of information in a single variable.
Create a Python Record
We create an inventory by inserting components inside sq. brackets []
, separated by commas. For instance,
# an inventory of three components
ages = [19, 26, 29]
print(ages)
# Output: [19, 26, 29]
Right here, the ages
record has three gadgets.
In Python, lists can retailer information of various information sorts.
# an inventory containing strings and numbers
scholar = ['Jack', 32, 'Computer Science']
print(scholar)
# an empty record
empty_list = []
print(empty_list)
We are able to use the built-in record() operate to transform different iterables (strings, dictionaries, tuples, and so forth.) to an inventory.
x = "axz"
# convert to record
end result = record(x)
print(end result) # ['a', 'x', 'z']
Record Traits
Lists are:
- Ordered – They preserve the order of components.
- Mutable – Gadgets could be modified after creation.
- Permit duplicates – They’ll include duplicate values.
Entry Record Components
Every ingredient in an inventory is related to a quantity, often known as a index.
The index all the time begins from 0. The primary ingredient of an inventory is at index 0, the second ingredient is at index 1, and so forth.

Entry Components Utilizing Index
We use index numbers to entry record components. For instance,
languages = ['Python', 'Swift', 'C++']
# entry the primary ingredient
print(languages[0]) # Python
# entry the third ingredient
print(languages[2]) # C++

Extra on Accessing Record Components
Python additionally helps damaging indexing. The index of the final ingredient is -1, the second-last ingredient is -2, and so forth.
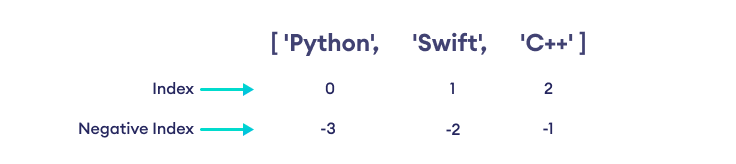
Adverse indexing makes it simple to entry record gadgets from final.
Let’s have a look at an instance,
languages = ['Python', 'Swift', 'C++']
# entry merchandise at index 0
print(languages[-1]) # C++
# entry merchandise at index 2
print(languages[-3]) # Python
In Python, it’s doable to entry a piece of things from the record utilizing the slicing operator :
. For instance,
my_list = ['p', 'r', 'o', 'g', 'r', 'a', 'm']
# gadgets from index 2 to index 4
print(my_list[2:5])
# gadgets from index 5 to finish
print(my_list[5:])
# gadgets starting to finish
print(my_list[:])
Output
['o', 'g', 'r'] ['a', 'm'] ['p', 'r', 'o', 'g', 'r', 'a', 'm']
To study extra about slicing, go to Python program to slice lists.
Be aware: If the required index doesn’t exist in an inventory, Python throws the IndexError
exception.
Add Components to a Python Record
We use the append() methodology so as to add a component to the tip of a Python record. For instance,
fruits = ['apple', 'banana', 'orange']
print('Authentic Record:', fruits)
# utilizing append methodology
fruits.append('cherry')
print('Up to date Record:', fruits)
Output
Authentic Record: ['apple', 'banana', 'orange'] Up to date Record: ['apple', 'banana', 'orange', 'cherry']
The insert() methodology provides a component on the specified index. For instance,
fruits = ['apple', 'banana', 'orange']
print("Authentic Record:", fruits)
# insert 'cherry' at index 2
fruits.insert(2, 'cherry')
print("Up to date Record:", fruits)
Output
Authentic Record: ['apple', 'banana', 'orange'] Up to date Record: ['apple', 'banana', 'cherry', 'orange']
We use the prolong() methodology so as to add components to an inventory from different iterables. For instance,
numbers = [1, 3, 5]
print('Numbers:', numbers)
even_numbers = [2, 4, 6]
# including components of 1 record to a different
numbers.prolong(even_numbers)
print('Up to date Numbers:', numbers)
Output
Numbers: [1, 3, 5] Up to date Numbers: [1, 3, 5, 2, 4, 6]
Change Record Gadgets
We are able to change the gadgets of an inventory by assigning new values utilizing the =
operator. For instance,
colours = ['Red', 'Black', 'Green']
print('Authentic Record:', colours)
# altering the third merchandise to 'Blue'
colours[2] = 'Blue'
print('Up to date Record:', colours)
Output
Authentic Record: ['Red', 'Black', 'Green'] Up to date Record: ['Red', 'Black', 'Blue']
Right here, we’ve changed the ingredient at index 2: 'Inexperienced'
with 'Blue'
.
Take away an Merchandise From a Record
We are able to take away an merchandise from an inventory utilizing the take away() methodology. For instance,
numbers = [2,4,7,9]
# take away 4 from the record
numbers.take away(4)
print(numbers)
# Output: [2, 7, 9]
The del assertion removes a number of gadgets from an inventory. For instance,
names = ['John', 'Eva', 'Laura', 'Nick', 'Jack']
# deleting the second merchandise
del names[1]
print(names)
# deleting gadgets from index 1 to index 3
del names[1: 4]
print(names) # Error! Record would not exist.
Output
['John', 'Laura', 'Nick', 'Jack'] ['John']
Be aware: We are able to additionally use the del
assertion to delete your complete record. For instance,
names = ['John', 'Eva', 'Laura', 'Nick']
# deleting your complete record
del names
print(names)
Python Record Size
We are able to use the built-in len() operate to seek out the variety of components in an inventory. For instance,
automobiles = ['BMW', 'Mercedes', 'Tesla']
print('Complete Components: ', len(automobiles))
# Output: Complete Components: 3
Iterating By way of a Record
We are able to use a for loop to iterate over the weather of an inventory. For instance,
fruits = ['apple', 'banana', 'orange']
# iterate by way of the record
for fruit in fruits:
print(fruit)
Output
apple banana orange
Python Record Strategies
Python has many helpful record strategies that make it very easy to work with lists.
Technique | Description |
---|---|
append() | Provides an merchandise to the tip of the record |
prolong() | Provides gadgets of lists and different iterables to the tip of the record |
insert() | Inserts an merchandise on the specified index |
take away() | Removes merchandise current on the given index |
pop() | Returns and removes merchandise current on the given index |
clear() | Removes all gadgets from the record |
index() | Returns the index of the primary matched merchandise |
rely() | Returns the rely of the required merchandise within the record |
kind() | Kinds the record in ascending/descending order |
reverse() | Reverses the merchandise of the record |
copy() | Returns the shallow copy of the record |
Extra on Python Lists
Record Comprehension is a concise and stylish method to create an inventory. For instance,
# create an inventory with sq. values
numbers = [n**2 for n in range(1, 6)]
print(numbers)
# Output: [1, 4, 9, 16, 25]
To study extra, go to Python Record Comprehension.
We use the in
key phrase to test if an merchandise exists within the record. For instance,
fruits = ['apple', 'cherry', 'banana']
print('orange' in fruits) # False
print('cherry' in fruits) # True
Right here,
- orange shouldn’t be current in
fruits
, so,'orange' in fruits
evaluates toFalse
. - cherry is current in
fruits
, so,'cherry' in fruits
evaluates toTrue
.
Additionally Learn