In C Programming, operators are symbols or key phrases used to carry out operations on values and variables. These are basic to performing varied operations in C programming and are important for constructing complicated algorithms and packages. We are able to use operators to carry out a variety of duties, together with arithmetic calculations, logical operations, and comparisons.
Desk of Contents
- 1) Task Operators ( =, +=, -=, *=, /=, %= )
- 2) Arithmetic Operators (+, -, /, * and %)
- 3) Unary Increment or Decrement Operators (++ and –)
- 4) Relational or Comparability Operators (==, !=, >, <, >= and <=)
- 5) Logical Operators ( !, && and ||)
- 6) Bit-wise Operators (~, &, |, ^, >> and <<)
- 7) Pointer Operators (*, & and ->)
- 8) Conditional Operators (? 🙂
- 9) Comma Operator ( , )
- Operators Diagram (Infographics)
1) Task Operators ( =, +=, -=, *=, /=, %= )
The task operator is the one equals signal (=).
i = 6; i = i + 1; |
The task operator copies the worth from its proper hand facet to the variable on its left hand facet. The task additionally acts as an expression which returns the newly assigned worth. Some programmers will use that characteristic to jot down issues like the next.
y = (x = 2 * x); // double x, and in addition put x’s new worth in y |
Different Task Operators (>>=, <<=, &=, |= and ^=)
Along with the plain = operator, C contains many shorthand operators which represents variations on the fundamental =. For instance “+=” provides the appropriate hand facet to the left hand facet. x = x + 10; may be decreased to x += 10;. That is most helpful if x is a protracted expression corresponding to the next, and in some instances it could run a little bit quicker.
individual -> family.mother.numChildren += 2; // enhance kids by 2 |
Right here’s the listing of task shorthand operators…
+=, -= Increment or decrement by RHS *=, /= Multiply or divide by RHS %= Mod by RHS >>= Bitwise proper shift by RHS (divide by energy of two) <<= Bitwise left shift RHS (multiply by energy of two) &=, |=, ^= Bitwise and, or, xor by RHS |
2) Arithmetic Operators (+, -, /, * and %)
C contains the same old binary and unary arithmetic operators. These are used to carry out fundamental arithmetic operations corresponding to addition (+), subtraction (-), multiplication (*), division (/), and the rest after division (%) modulo division.
Personally, I simply use parenthesis liberally to keep away from any bugs attributable to a misunderstanding of priority. The operators are delicate to the kind of the operands. So division (/) with two integer arguments will do integer division. If both argument is a float, it does floating level division. So (6/4) evaluates to 1 whereas (6/4.0) evaluates to 1.5 — the 6 is promoted to six.0 earlier than the division.
+ Addition – Subtraction / Division * Multiplication % The rest after division( modulo division) |
3) Unary Increment or Decrement Operators (++ and –)
The unary ++ and — operators increment or decrements the worth in a variable. There are “pre” and “submit” variants for each operators which do barely various things (defined under)
var++ increment “submit” variant ++var increment “pre” variant var– decrement “submit” variant –var decrement “pre” variant int i = 42; i++; // increment on i // i is now 43 i–; // decrement on i // i is now 42 |
4) Relational or Comparability Operators (==, !=, >, <, >= and <=)
These function on integer or floating level values and return a 0 or 1 boolean worth to indicate the comparisons between two operands.
== Equal != Not Equal > Better Than < Much less Than >= Better or Equal <= Much less or Equal To see if x equals three, write one thing like: if (x == 3) … |
Utilizing each task (=) and comparability (==) operators collectively
A fully basic pitfall is to jot down task (=) if you imply comparability (==). This might not be such an issue, besides the wrong task model compiles wonderful as a result of the compiler assumes you imply to make use of the worth returned by the task. That is not often what you need
This doesn’t check if x is 3. This units x to the worth 3, after which returns the three to the if for testing. 3 will not be 0, so it counts as “true” each time. That is most likely the one commonest error made by starting C programmers.
The issue is that the compiler is not any assist — it thinks each kinds are wonderful, so the one protection is excessive vigilance when coding.
5) Logical Operators ( !, && and ||)
In Logical operators in C, the worth 0 is fake, the rest is true. The operators consider left to proper and cease as quickly as the reality or falsity of the expression may be deduced. (Such operators are referred to as “brief circuiting”) In ANSI C, these are moreover assured to make use of 1 to characterize true, and never just a few random non-zero bit sample.
! Boolean not (unary) && Boolean and || Boolean or |
6) Bit-wise Operators (~, &, |, ^, >> and <<)
C contains operators to govern reminiscence on the bit degree. That is helpful for writing low degree {hardware} or working system code the place the extraordinary abstractions of numbers, characters, pointers, and many others… are inadequate — an more and more uncommon want. Bit manipulation code tends to be much less “transportable”. Code is “transportable” if with no programmer intervention it compiles and runs appropriately on several types of computer systems. The bit-wise operations are usually used with unsigned varieties. Specifically, the shift operations are assured to shift 0 bits into the newly vacated positions when used on unsigned values.
~ Bitwise Negation (unary) – flip 0 to 1 and 1 to 0 all through & Bitwise And | Bitwise Or ^ Bitwise Unique Or >> Proper Shift by proper hand facet (RHS) (divide by energy of two) << Left Shift by RHS (multiply by energy of two) |
Don’t confuse the Bit-wise operators with the logical operators. The bit-wise connectives are one character broad (&, |) whereas the Boolean connectives are two characters broad (&&, ||). The bit-wise operators have increased priority than the Boolean operators. The compiler won’t ever make it easier to out with a kind error for those who use & if you meant &&. So far as the sort checker is worried, they’re similar– they each take and produce integers since there isn’t a distinct Boolean sort.
7) Pointer Operators (*, & and ->)
In C, pointer operators are operators which might be used together with pointers. There are a number of pointer operators in C, together with:
- Tackle-of operator (
&
): Returns the reminiscence tackle of a variable. When used with a variable, the&
operator returns the tackle of the variable in reminiscence. - Dereference operator (
*
): Accesses the worth saved at a reminiscence tackle. When used with a pointer, the*
operator returns the worth saved on the tackle pointed to by the pointer. - Indirection operator (
->
): Accesses a member of a construction via a pointer. The->
operator is used to entry members of a construction when a pointer to the construction is used.
8) Conditional Operators (? 🙂
The conditional operator (often known as the ternary operator ) is a shorthand method of writing an if
assertion. It permits you to conditionally execute an expression based mostly on the worth of a situation. The syntax of the ternary operator is as follows:
situation ? expression1 : expression2 |
9) Comma Operator ( , )
The comma operator ,
in C is used to separate expressions. It evaluates every expression from left to proper and returns the worth of the rightmost expression. It’s typically utilized in locations the place a number of expressions are syntactically allowed however just one is predicted.
For instance within the following instance, the expressions a++
and b++
are evaluated first after which the expression a + b
is evaluated, and the result’s assigned to the variable c
.
int predominant() {
int a = 5, b = 10, c;
// The comma operator is used right here to mix a number of expressions
c = (a++, b++, a + b);
printf(“a = %d, b = %d, c = %dn”, a, b, c);
return 0;
}
#embrace <stdio.h>
int predominant() { int a = 5, b = 10, c;
// The comma operator is used right here to mix a number of expressions c = (a++, b++, a + b);
printf(“a = %d, b = %d, c = %dn”, a, b, c);
return 0; } |
Operators Diagram (Infographics)
The next diagram reveals an inventory of operators and their classes utilized in C.
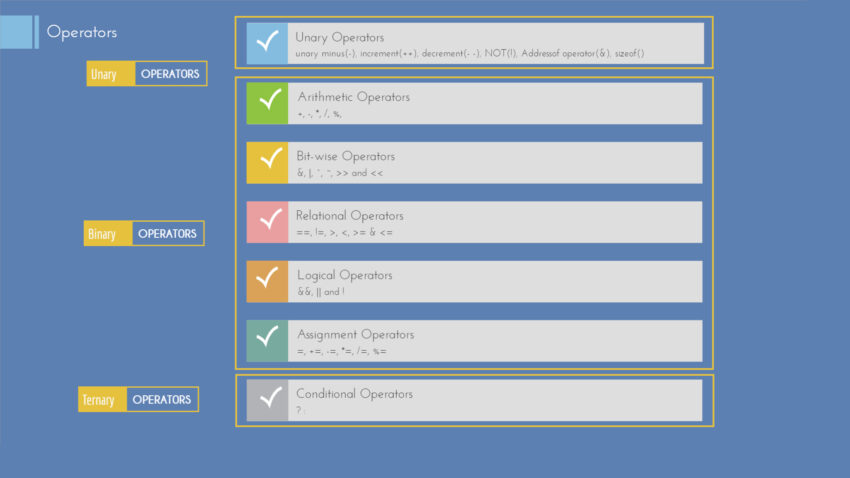
If you wish to see these operators in motion, have a look at the next C Applications: