💬 Query: Given a Boolean worth True
or False
. Methods to convert it to a string "True"
or "False"
in Python?
Be aware that this tutorial doesn’t concern “concatenating a Boolean to a string”. If you wish to do that, take a look at our in-depth article on the Finxter weblog.
Easy Bool to String Conversion
To transform a given Boolean worth to a string in Python, use the str(boolean)
perform and move the Boolean worth into it. This converts Boolean True
to string "True"
and Boolean False
to string "False"
.
Right here’s a minimal instance:
>>> str(True) 'True' >>> str(False) 'False'
Python Boolean Kind is Integer
Booleans are represented by integers in Python, i.e., bool
is a subclass of int
. Boolean worth True
is represented with integer 1
. And Boolean worth False
is represented with integer 0
.
Right here’s a minimal instance:
>>> True == 1 True >>> False == 0 True
Convert True to ‘1’ and False to ‘0’
To transform a Boolean worth to a string '1'
or '0'
, use the expression str(int(boolean))
. For example, str(int(True))
returns '1'
and str(int(False))
returns '0'
. That is due to Python’s use of integers to signify Boolean values.
Right here’s a minimal instance:
>>> str(int(True)) '1' >>> str(int(False)) '0'
Convert Checklist of Boolean to Checklist of Strings
To transform a Boolean to a string checklist, use the checklist comprehension expression [str(x) for x in my_bools]
assuming the Boolean checklist is saved in variable my_bools
. This converts every Boolean x
to a string utilizing the built-in str()
perform and repeats it for all x
within the Boolean checklist.
Right here’s a easy instance:
my_bools = [True, True, False, False, True] my_strings = [str(x) for x in my_bools] print(my_strings) # ['True', 'True', 'False', 'False', 'True']
Convert String Again to Boolean
What if you wish to convert the string illustration 'True'
and 'False'
(or: '1'
and '0'
) again to the Boolean illustration True
and False
?
👉 Advisable Tutorial: String to Boolean Conversion
Right here’s the brief abstract:
You possibly can convert a string worth s
to a Boolean worth utilizing the Python perform bool(s)
.
For instance, bool('True')
and bool('1')
return True
.
Nevertheless, bool('False')
and bool('0')
return False
as properly which can come sudden to you.
💡 It is because all Python objects are “truthy”, i.e., they’ve an related Boolean worth. As a rule of thumb: empty values return Boolean True
and non-empty values return Boolean False
. So, solely bool('')
on the empty string ''
returns False
. All different strings return True
!
You possibly can see this within the following instance:
>>> bool('True') True >>> bool('1') True >>> bool('2') True >>> bool('False') True >>> bool('0') True >>> bool('') False
Okay, what to do about it?
Simple – first move the string into the eval()
perform after which move the consequence into the bool()
perform. In different phrases, the expression bool(eval(my_string))
converts a string to a Boolean mapping 'True'
and '1'
to Boolean True
and 'False'
and '0'
to Boolean False
.
Lastly – this conduct is as anticipated by many coders simply beginning out.
Right here’s an instance:
>>> bool(eval('False')) False >>> bool(eval('0')) False >>> bool(eval('True')) True >>> bool(eval('1')) True
Be at liberty to go over our detailed information on the perform:
👉 Advisable Tutorial: Python eval()
deep dive
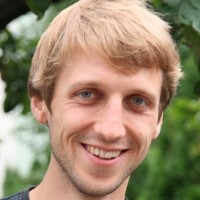
Whereas working as a researcher in distributed techniques, Dr. Christian Mayer discovered his love for instructing pc science college students.
To assist college students attain greater ranges of Python success, he based the programming training web site Finxter.com. He’s writer of the favored programming ebook Python One-Liners (NoStarch 2020), coauthor of the Espresso Break Python sequence of self-published books, pc science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his biggest ardour is to serve aspiring coders by means of Finxter and assist them to spice up their abilities. You possibly can be a part of his free e-mail academy right here.