Whereas growing an utility, efficient communication and preservation of knowledge throughout parts are very important, particularly when navigating between pages. Whereas passing data between parts is a standard strategy, state upkeep could also be extra optimum for dealing with advanced eventualities.
This weblog elucidates the steps to implement state upkeep within the Syncfusion Blazor Tabs element.
Understanding state in Blazor
In Blazor, the person’s state resides within the server’s reminiscence inside a circuit. State administration entails preserving the app state even after a connection loss or disconnection. The state inside a person’s circuit encompasses varied facets:
- UI-related knowledge, together with element cases and rendered output.
- Property/discipline values of element cases.
- Dependency injection service occasion knowledge.
Notice that by default, Blazor can solely handle inside states, which means the variables are intently tied to the element the place they’re outlined. Consequently, the related variables will likely be misplaced when a element is deleted. A easy web page navigation serves for example for this state of affairs.
Necessity for state administration
In Blazor, an app is related to the server by means of a circuit holding the person’s state. This connection might be disrupted or misplaced attributable to causes corresponding to:
- Many customers accessing the server trigger elevated reminiscence utilization and circuit disconnection.
- Customers reload the app or webpage, resulting in circuit disconnection.
These disruptions can lead to the lack of the person’s state, probably inflicting important knowledge loss for processes like:
- Multistep webforms.
- Buying carts.
Lack of knowledge after finishing multistep processes or making a buying checklist can result in pointless time consumption. In Blazor, knowledge is saved within the native cache to counteract this, guaranteeing that the state persists even within the occasion of circuit disconnection.
Persisting state in Blazor Tabs
Rendering content material inside the Blazor Tabs presents flexibility. The content material rendering of tabs might be achieved in 3 ways:
This part will elaborate on sustaining the state for the Blazor Tabs throughout these three content-rendering modes.
Dynamic rendering
Dynamic rendering is the default rendering mode. It initially hundreds the chosen tab’s content material and makes it accessible within the DOM. Should you select one other tab, the content material within the DOM is changed with that tab’s content material. Whereas this boosts web page loading efficiency by rendering solely the at present energetic tab content material, the draw back is that tabs don’t retain their present state, since every tab hundreds recent content material when chosen.
On this mode, state administration should be dealt with on the utility finish. Sustaining state in tab content material might be achieved utilizing dependency injection service occasion knowledge.
Observe these steps to protect the state in Blazor Tabs in dynamic rendering mode.
Step 1: Making a state administration service
To handle the state in reminiscence (or within the circuit for Blazor Server), we have to set up a devoted service for state dealing with. Confer with the next code instance.
FormService.cs
public class FormService { public string? UserName { get; set; } public string? MailAddress { get; set; } }
Then, register the service within the Program.cs file.
var builder = WebApplication.CreateBuilder(args); // Add providers to the container. builder.Companies.AddRazorPages(); builder.Companies.AddServerSideBlazor(); builder.Companies.AddSingleton<WeatherForecastService>(); builder.Companies.AddSingleton<FormService, FormService>(); builder.Companies.AddSyncfusionBlazor();
Step 2: Configure dynamic rendering mode in Blazor Tabs
Inject the FormService and carry out knowledge binding instantly on the Index.razor web page. Lastly, render the Syncfusion Blazor Tabs element with the dynamic rendering mode.
Index.razor
@utilizing BlazorStateMaintenance.Knowledge @inject FormService kind @utilizing Syncfusion.Blazor.Navigations @utilizing Syncfusion.Blazor.Inputs @utilizing Syncfusion.Blazor.Buttons <SfTab @bind-SelectedItem="@selectedTab"> <TabItems> <TabItem> <HeaderTemplate>Sign up</HeaderTemplate> <ContentTemplate> <div id="Person particulars" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox Placeholder="E-mail" @bind-Worth="kind.MailAddress" Kind="InputType.E-mail"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSignin">Sign up</SfButton> <SfButton @onclick="@OnSkip">Skip</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Syncfusion Blazor</HeaderTemplate> <ContentTemplate> <p>You'll be able to try our Syncfusion Blazor demo right here - https://blazor.syncfusion.com/demos/ </p> <br /> <p>Additionally, the person information will likely be accessible right here - https://blazor.syncfusion.com/documentation/introduction/</p> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Suggestions</HeaderTemplate> <ContentTemplate> <div id="Feedback_Form" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="kind.MailAddress" Placeholder="E-mail" Kind="InputType.E-mail"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="feedback" Placeholder="Share your feedback"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSubmit">Submit</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> </TabItems> </SfTab> @code { non-public string feedback { get; set; } non-public int selectedTab { get; set; } = 0; non-public Boolean emptyField = false; non-public void OnSignin() { if (kind.UserName == null || kind.MailAddress == null) { emptyField = true; } else { emptyField = false; this.selectedTab = 1; } } non-public void OnSkip() { this.selectedTab = 1; } non-public void OnSubmit() { if (kind.UserName == null || kind.MailAddress == null || this.feedback == null) { emptyField = true; } else { emptyField = false; kind.UserName = null; kind.MailAddress = null; this.feedback = null; } } }
On this illustration, the FormService is seamlessly injected into the Index.razor web page, establishing a direct binding between its properties and the content material inside the tabs. You’ll be able to simply customise the bindings and content material to align with the precise necessities of your app.
The FormService ensures persistence in in-memory storage even when the Blazor Tabs element undergoes destruction and recreation.
Confer with the next output picture. In it, the username and e mail deal with are retained inside the tab gadgets, even throughout navigation.
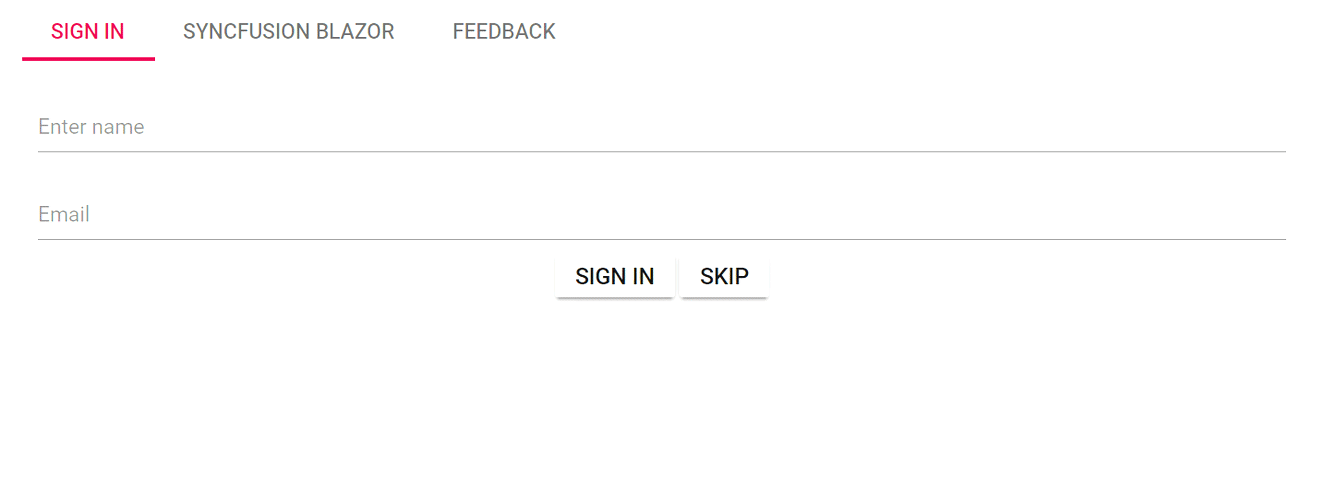
On-demand rendering or lazy loading
We are able to allow on-demand rendering or lazy loading for the Blazor Tabs element by configuring the ContentLoad.Demand property to LoadOn. On this mode, solely the content material of the chosen tab will likely be loaded initially and rendered upon choice. The content material of the tabs loaded as soon as will likely be maintained within the DOM. This strategy ensures the persistence of tab states, corresponding to scroller place and kind values.
Confer with the next code instance. The info entered within the first tab persists even when navigating to the third tab.
Index.razor
@utilizing BlazorStateMaintenance.Knowledge @inject FormService kind @utilizing Syncfusion.Blazor.Navigations @utilizing Syncfusion.Blazor.Inputs @utilizing Syncfusion.Blazor.Buttons <SfTab LoadOn="ContentLoad.Demand" @bind-SelectedItem="@selectedTab"> <TabItems> <TabItem> <HeaderTemplate>Sign up</HeaderTemplate> <ContentTemplate> <div id="Person particulars" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox Placeholder="E-mail" @bind-Worth="kind.MailAddress" Kind="InputType.E-mail"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSignin">Sign up</SfButton> <SfButton @onclick="@OnSkip">Skip</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Syncfusion Blazor</HeaderTemplate> <ContentTemplate> <p>You'll be able to try our Syncfusion Blazor demo right here - https://blazor.syncfusion.com/demos/ </p> <br /> <p>Additionally, the person information will likely be accessible right here - https://blazor.syncfusion.com/documentation/introduction/</p> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Suggestions</HeaderTemplate> <ContentTemplate> <div id="Feedback_Form" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="kind.MailAddress" Placeholder="E-mail" Kind="InputType.E-mail"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="feedback" Placeholder="Share your feedback"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSubmit">Submit</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> </TabItems> </SfTab> @code { non-public string feedback { get; set; } non-public int selectedTab { get; set; } = 0; non-public Boolean emptyField = false; non-public void OnSignin() { if (kind.UserName == null || kind.MailAddress == null) { emptyField = true; } else { emptyField = false; this.selectedTab = 1; } } non-public void OnSkip() { this.selectedTab = 1; } non-public void OnSubmit() { if (kind.UserName == null || kind.MailAddress == null || this.feedback == null) { emptyField = true; } else { emptyField = false; kind.UserName = null; kind.MailAddress = null; this.feedback = null; } } }
Alter the content material inside every tab as wanted. The carried out logic ensures that person inputs within the first tab persist when navigating to the third tab.

On-initial rendering
We are able to activate the preliminary rendering mode for Blazor Tabs by configuring the ContentLoad.Init property to LoadOn. On this mode, the content material of all tabs will likely be rendered through the preliminary load and retained within the DOM. Moreover, this mode permits entry to the references of parts rendered in different tabs. Make the most of this mode when coping with a restricted variety of tabs the place sustaining tab state is essential.
Within the following code instance, all three tabs are rendered through the preliminary load, and the info entered within the first tab persists even when the second or third tab is energetic. This mode is especially helpful when working with fewer tabs containing minimal content material.
Index.razor
@utilizing BlazorStateMaintenance.Knowledge @inject FormService kind @utilizing Syncfusion.Blazor.Navigations @utilizing Syncfusion.Blazor.Inputs @utilizing Syncfusion.Blazor.Buttons <SfTab LoadOn="ContentLoad.Init" @bind-SelectedItem="@selectedTab"> <TabItems> <TabItem> <HeaderTemplate>Sign up</HeaderTemplate> <ContentTemplate> <div id="Person particulars" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox Placeholder="E-mail" @bind-Worth="kind.MailAddress" Kind="InputType.E-mail"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSignin">Sign up</SfButton> <SfButton @onclick="@OnSkip">Skip</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Syncfusion Blazor</HeaderTemplate> <ContentTemplate> <p>You'll be able to try our Syncfusion Blazor demo right here - https://blazor.syncfusion.com/demos/ </p> <br /> <p>Additionally, the person information will likely be accessible right here - https://blazor.syncfusion.com/documentation/introduction/</p> </ContentTemplate> </TabItem> <TabItem> <HeaderTemplate>Suggestions</HeaderTemplate> <ContentTemplate> <div id="Feedback_Form" model="padding:10px"> <kind id="formId"> <div class="form-group"> <div class="e-float-input"> <SfTextBox Placeholder="Enter title" @bind-Worth="kind.UserName"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="kind.MailAddress" Placeholder="E-mail" Kind="InputType.E-mail"></SfTextBox> </div> <div class="e-float-input"> <SfTextBox @bind-Worth="feedback" Placeholder="Share your feedback"></SfTextBox> </div> </div> </kind> <div model="text-align: middle"> <SfButton @onclick="@OnSubmit">Submit</SfButton> @if (emptyField) { <div class="Error">* Please fill all fields</div> } </div> </div> </ContentTemplate> </TabItem> </TabItems> </SfTab> @code { non-public string feedback { get; set; } non-public int selectedTab { get; set; } = 0; non-public Boolean emptyField = false; non-public void OnSignin() { if (kind.UserName == null || kind.MailAddress == null) { emptyField = true; } else { emptyField = false; this.selectedTab = 1; } } non-public void OnSkip() { this.selectedTab = 1; } non-public void OnSubmit() { if (kind.UserName == null || kind.MailAddress == null || this.feedback == null) { emptyField = true; } else { emptyField = false; kind.UserName = null; kind.MailAddress = null; this.feedback = null; } } }
Confer with the next output picture.

GitHub references
Discover the practical instance of state upkeep in Blazor Tabs by visiting this GitHub repository.
Abstract
We recognize the time spent on this weblog, the place we defined the steps for sustaining the state within the Syncfusion Blazor Tabs element. We hope you discovered the knowledge precious. Take a hands-on strategy by following the outlined steps and share your ideas within the feedback under.
For our present Syncfusion prospects, the brand new model of Important Studio is offered on the License and Downloads web page. Should you’re not but a part of our group, join a 30-day free trial to judge these options.
For questions, you’ll be able to contact us by means of our assist boards, assist portal, or suggestions portal. Your satisfaction is our precedence, and we stay up for helping you in your Syncfusion journey!