The .NET MAUI Scheduler offers all of the scheduling functionalities to create and handle skilled and private appointments. This weblog explains learn how to load appointments on demand within the .NET MAUI Scheduler management through net providers utilizing JSON knowledge.
Let’s get began!
Creating an internet knowledge mannequin
First, we have to create a .NET MAUI software and an internet knowledge mannequin to get the appointment info from an internet service.
Seek advice from the next code instance.
public class WebData { public string Topic { get; set; } public string StartTime { get; set; } public string EndTime { get; set; } }
Creating an internet API service and fetching knowledge from it
We will get the web JSON knowledge with the assistance of the GetStringAsync methodology and deserialize it as a listing of WebData fashions. We are going to use the hosted service for instance.
Seek advice from the next code instance to deserialize JSON net knowledge.
SchedulerViewModel.cs
personal async Process<Checklist<WebData>> GetJsonWebData() { var httpClient = new HttpClient(); var response = await httpClient.GetStringAsync("https://providers.syncfusion.com/js/manufacturing/api/schedule"); return JsonConvert.DeserializeObject<Checklist< WebData >>(response); }
Load appointments on demand through net service in .NET MAUI Scheduler
Let’s load the deserialized knowledge into the Syncfusion .NET MAUI Scheduler management on demand.
Word: Earlier than continuing, please learn the getting began with .NET MAUI Scheduler documentation.
Step 1: Register the handler
We register the handler for Syncfusion core within the MauiProgram.cs file.
Public static MauiApp CreateMauiApp() { var builder = MauiApp.CreateBuilder(); builder.ConfigureSyncfusionCore();
}
Step 2: Creating an appointment mannequin class
Subsequent, we create the appointment Occasion knowledge mannequin class containing primary info to generate the appointments.
Seek advice from the next code instance.
Occasion.cs
public class Occasion : INotifyPropertyChanged { personal DateTime from; personal DateTime to; personal bool isAllDay; personal string eventName; personal Brush background; /// <abstract> /// Will get or units the worth to show the beginning date. /// </abstract> public DateTime From { get { return this.from; } set { this.from = worth; this.OnPropertyChanged(nameof(From)); } } /// <abstract> /// Will get or units the worth to show the top date. /// </abstract> public DateTime To { get { return this.to; } set { this.to = worth; this.OnPropertyChanged(nameof(To)); } } /// <abstract> /// Will get or units the worth indicating whether or not the appointment is all day or not. /// </abstract> public bool IsAllDay { get { return this.isAllDay; } set { this.isAllDay = worth; this.OnPropertyChanged(nameof(IsAllDay)); } } /// <abstract> /// Will get or units the worth to show the topic. /// </abstract> public string EventName { get { return this.eventName; } set { this.eventName = worth; } } /// <abstract> /// Will get or units the worth to show the background. /// </abstract> public Brush Background { get { return this.background; } set { this.background = worth; } } public occasion PropertyChangedEventHandler? PropertyChanged; personal void OnPropertyChanged(string propertyName) { this.PropertyChanged?.Invoke(this, new PropertyChangedEventArgs(propertyName)); } }
Step 3: Create the appointments
Following that, we’ll have a look at creating an Occasions assortment from on-line knowledge retrieved through the online service.
You may load appointments on demand with a loading indicator primarily based on the Scheduler’s seen date vary. We will enhance the appointment loading efficiency utilizing the QueryAppointmentsCommand property.
Seek advice from the next code instance to retrieve net appointments within the view mannequin class.
SchedulerViewModel.cs
public ICommand QueryAppointmentsCommand { get; set; } personal ObservableCollection<Occasion> occasions; public ObservableCollection<Occasion> Occasions { get { return this.occasions; } set { this.occasions = worth; } } public SchedulerViewModel() { this.QueryAppointmentsCommand = new Command<object>(LoadAppointments); } personal async void LoadAppointments(object obj) { //// Get appointment particulars from the online service. if (jsonWebData == null) jsonWebData = await GetJsonWebData(); Random random = new Random(); var visibleDates = ((SchedulerQueryAppointmentsEventArgs)obj).VisibleDates; this.Occasions = new ObservableCollection<Occasion>(); this.ShowBusyIndicator = true; DateTime visibleStartDate = visibleDates.FirstOrDefault(); DateTime visibleEndDate = visibleDates.LastOrDefault(); foreach (var knowledge in jsonWebData) { DateTime startDate = Convert.ToDateTime(knowledge.StartTime); DateTime endDate = Convert.ToDateTime(knowledge.EndTime); if ((visibleStartDate <= startDate.Date && visibleEndDate >= startDate.Date) || (visibleStartDate <= endDate.Date && visibleEndDate >= endDate.Date)) { Occasions.Add(new Occasion() { EventName = knowledge.Topic, From = startDate, To = endDate, Background = this.colours[random.Next(this.colors.Count)] }); } } if (jsonWebData != null) await Process.Delay(1000); this.ShowBusyIndicator = false; }
Step 4: Bind appointments to the Scheduler
Let’s initialize the .NET MAUI Scheduler management.
MainPage.xaml
xmlns:scheduler="clr-namespace:Syncfusion.Maui.Scheduler;meeting=Syncfusion.Maui.Scheduler" <scheduler:SfScheduler/>
Subsequent, we bind the customized knowledge Occasions to the .NET MAUI Scheduler’s AppointmentsSource utilizing the appointment mapping function.
Seek advice from the next code instance to bind the QueryAppointmentsCommand and AppointmentsSource properties from the SchedulerViewModel class.
MainPage.xaml
<scheduler:SfScheduler View="Month" QueryAppointmentsCommand="{Binding QueryAppointmentsCommand}" AppointmentsSource="{Binding Occasions}"> <scheduler:SfScheduler.AppointmentMapping> <scheduler:SchedulerAppointmentMapping Topic="EventName" StartTime="From" EndTime="To" Background="Background" IsAllDay="IsAllDay" /> </scheduler:SfScheduler.AppointmentMapping> <scheduler:SfScheduler.BindingContext> <native:SchedulerViewModel/> </scheduler:SfScheduler.BindingContext> </scheduler:SfScheduler>
After executing the earlier code examples, we’ll get output like within the following picture.
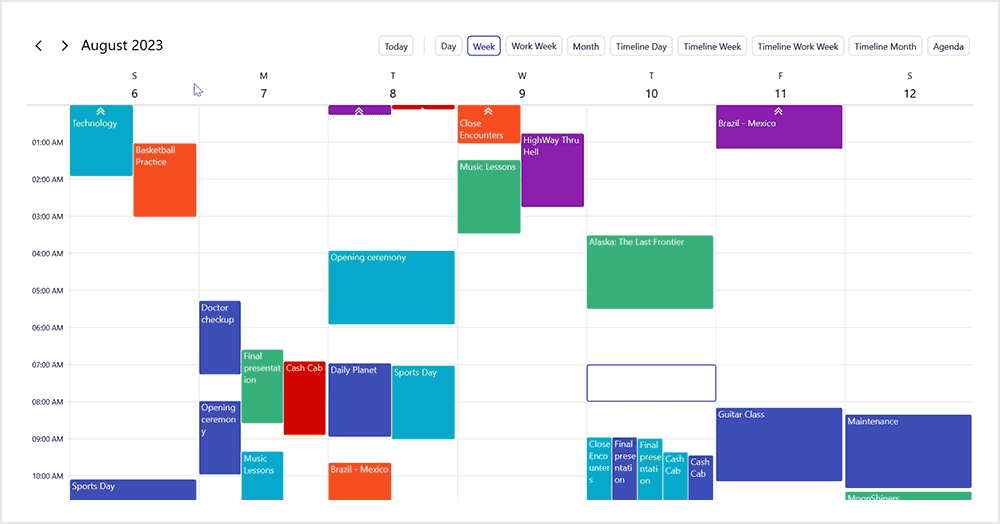
GitHub reference
For extra particulars, discuss with the instance for loading appointments on demand through net providers in .NET MAUI Scheduler utilizing JSON knowledge on GitHub.
Conclusion
Thanks for studying! On this weblog, we discovered learn how to load appointments on demand within the .NET MAUI Scheduler management through net providers. With this, you possibly can load solely the required appointments on demand and significantly improve the loading efficiency of your app. Check out the steps and go away your suggestions within the feedback part beneath!
For present Syncfusion clients, the latest model of Important Studio is accessible from the license and downloads web page. If you’re not a buyer but, attempt our 30-day free trial to take a look at these new options.
You can even contact us by way of our help discussion board, help portal, or suggestions portal. We’re all the time completely happy to help you!