GoLand 2023.3 comes with help for knowledge stream evaluation (DFA). On this put up, we’ll introduce the characteristic, clarify the way it works, and present some real-world examples of how DFA can detect bugs on the fly!
Because of the CLion workforce for serving to us by porting their highly effective DFA engine. For now, the GoLand engine solely implements a restricted variety of DFA options, however extra will likely be added in subsequent releases. The CLion workforce has additionally lined quite a lot of different matters, together with a deeper dive into implementation specifics, on their weblog.
What’s knowledge stream evaluation?
DFA is a sort of static code evaluation that analyzes how knowledge flows by means of a program. In fundamental phrases, it calculates the doable values of variables at totally different factors in this system’s execution. With this data, yow will discover varied potential bugs, reminiscent of nil
dereferences, infinite loops, fixed situations, and different incorrect or atypical program habits.
Let’s have a look at a simple instance of DFA in motion within the type of a easy perform:
func dummy(initializeResource bool) { 1 var useful resource *Useful resource = nil 2 init := false 3 4 if initializeResource { 5 useful resource = new(Useful resource) 6 init = true 7 } 8 9 r := useful resource 10 _ = r.Title }
A typical method to carry out DFA begins with constructing a management stream graph (CFG) of the analyzed perform. The CFG of this dummy perform is offered under. For readability, all statements (strains of code) are numbered in response to their corresponding code snippet.
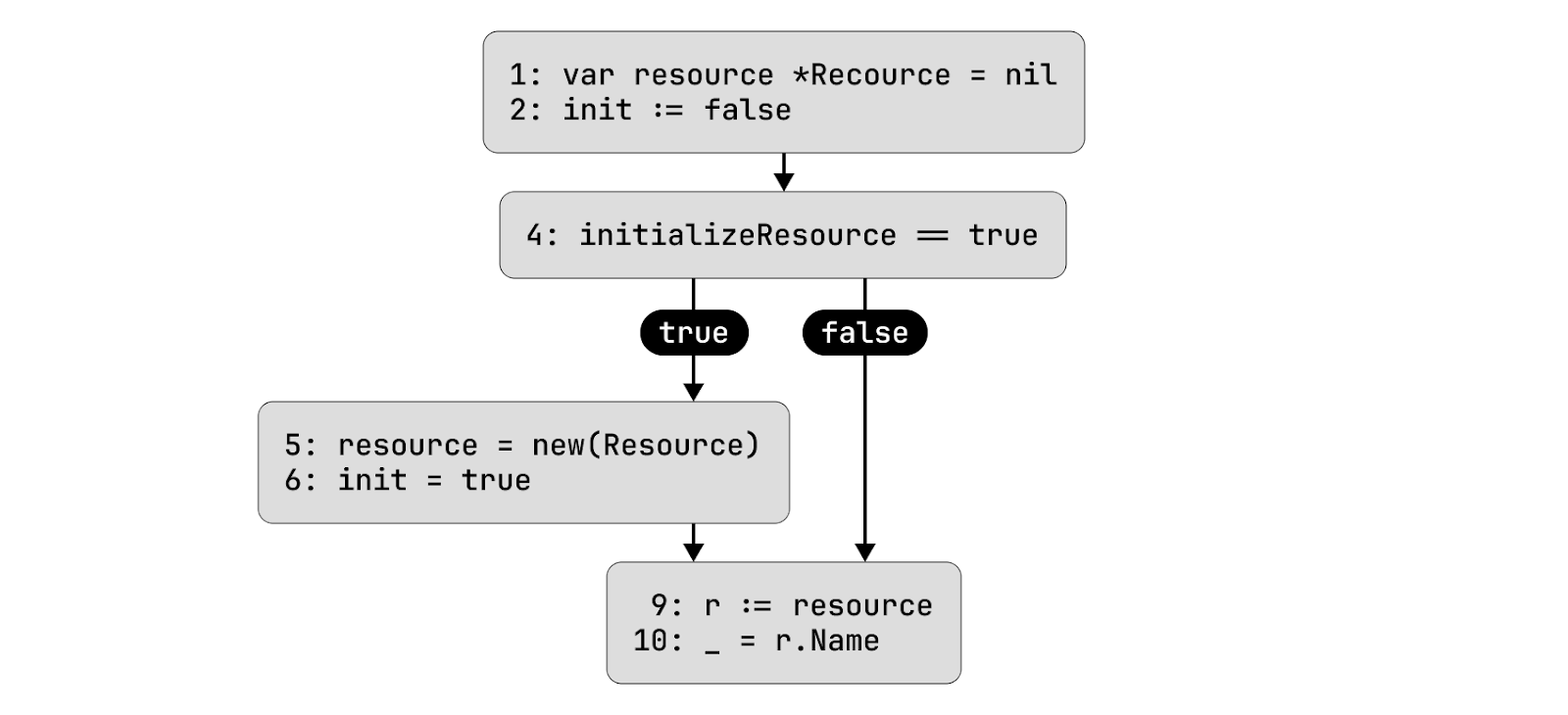
You possibly can take into consideration CFG as a easy graph that displays the perform’s execution. The graph nodes correspond to code blocks, and the sides replicate conditional and unconditional jumps between them. You don’t have to know the precise formal definition of a CFG or the right way to construct them for this text, however should you’d wish to find out about CFGs, you’ll be able to go to this hyperlink.
As soon as the CFG has been constructed, the principle stage of the evaluation can start. Throughout this stage, the DFA computes the entire doable values of variables that may observe every perform assertion. Roughly talking, this may be executed by propagating values by means of statements (reminiscent of assignments) and the sides of the CFG, taking into consideration the reachability of the CFG’s nodes.
For instance, the doable worth set of the variable r after assertion 9 is {nil, new(Useful resource)}
. The nil
worth is obtained from assertion 1
by propagating it by means of the trail 1 -> 2 -> 4 -> 9. The new(Useful resource)
worth is obtained by propagating it by means of the trail 1 -> 2 -> 4 -> 5 -> 6 -> 9, taking into consideration assignments on strains 5 and 9.
We will use the data obtained about variable values to search out potential bugs within the corresponding packages. For instance, since we all know that, after assertion 9, the variable r
will be nil
, which means there could be a nil
dereference on line 10.
Challenges of information stream evaluation
As an introduction to the potential pitfalls and difficulties related to implementing knowledge stream evaluation, let’s check out a barely modified model of our earlier instance perform:
func dummy(initializeResource bool) { 1 var useful resource *Useful resource = nil 2 init := false 3 4 if initializeResource { 5 useful resource = new(Useful resource) 6 init = true 7 } 8 9 r := useful resource 10 if init { 11 _ = r.Title 12 } 13 }
The corresponding CFG appears like this:
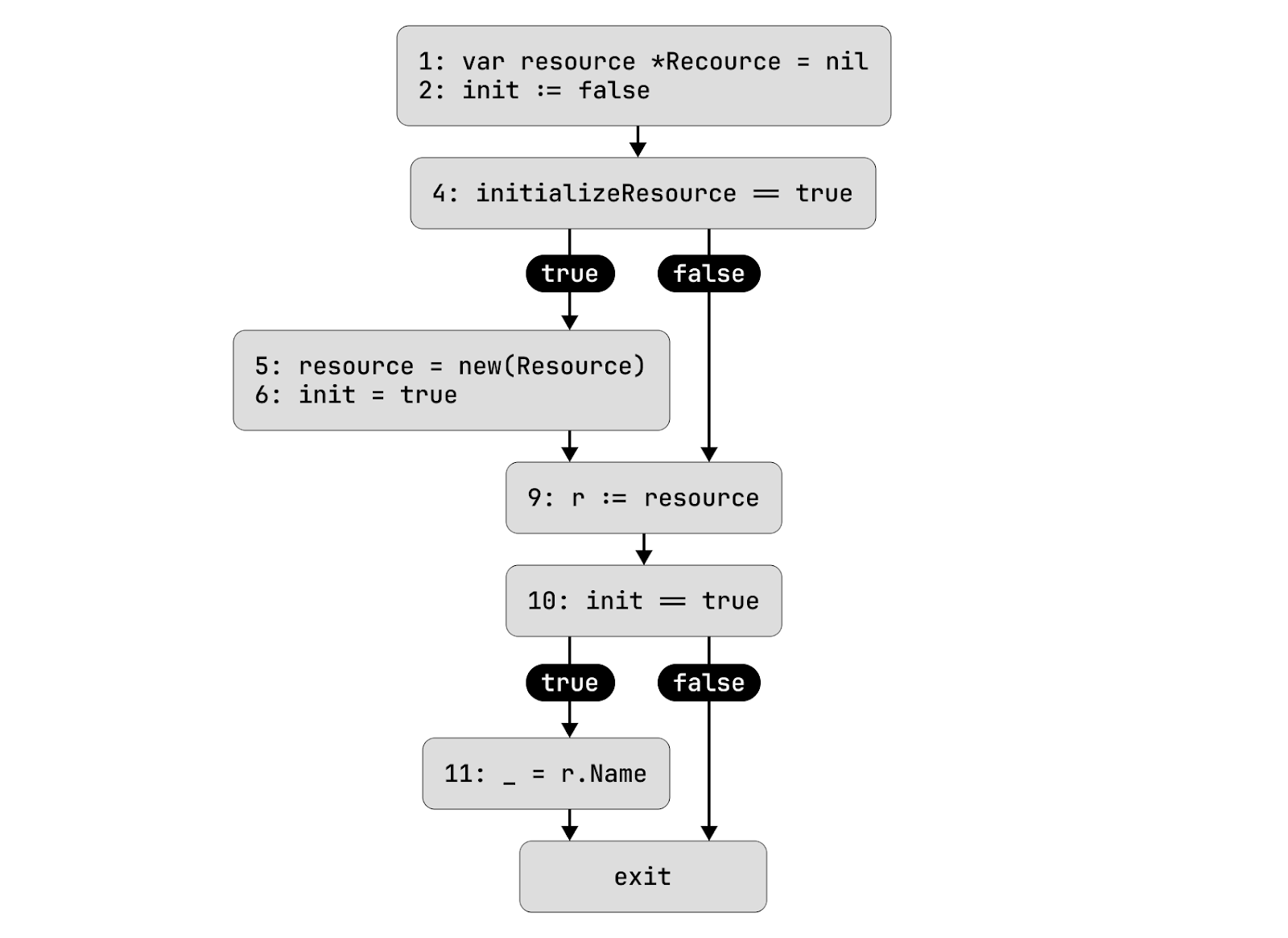
DFA, like different static evaluation instruments, over-approximates the habits of packages. In our case, because of this DFA computes the higher sure of doable variable values. On this instance, knowledge stream evaluation infers that the doable worth set of the variable init in assertion 10 is {false, true}
. Therefore, each the branches of the situation on line 10 are reachable, which implies an execution can attain assertion 11. In assertion 11, the worth set of variable r
is {nil, new(Useful resource)}
. Thus, we are able to infer that there’s a potential nil
dereference on line 11.
However that’s probably not true. In truth, the variable init
can take each true
and false
values at assertion 10. Nonetheless, the reachability of the situation init == true
additionally relies on the situation initializeResource == true
. If the latter is met, then init
can solely take the worth true, and if it isn’t met, then init
can solely be false. To determine such instances, we should use contexts. Let’s assume that we’re analyzing a perform in two totally different contexts. The primary context corresponds to a case the place initializeResource
is true
, the second corresponds to a case the place initializeResource
is false
. These contexts are most simply described as a clone of the CFG:
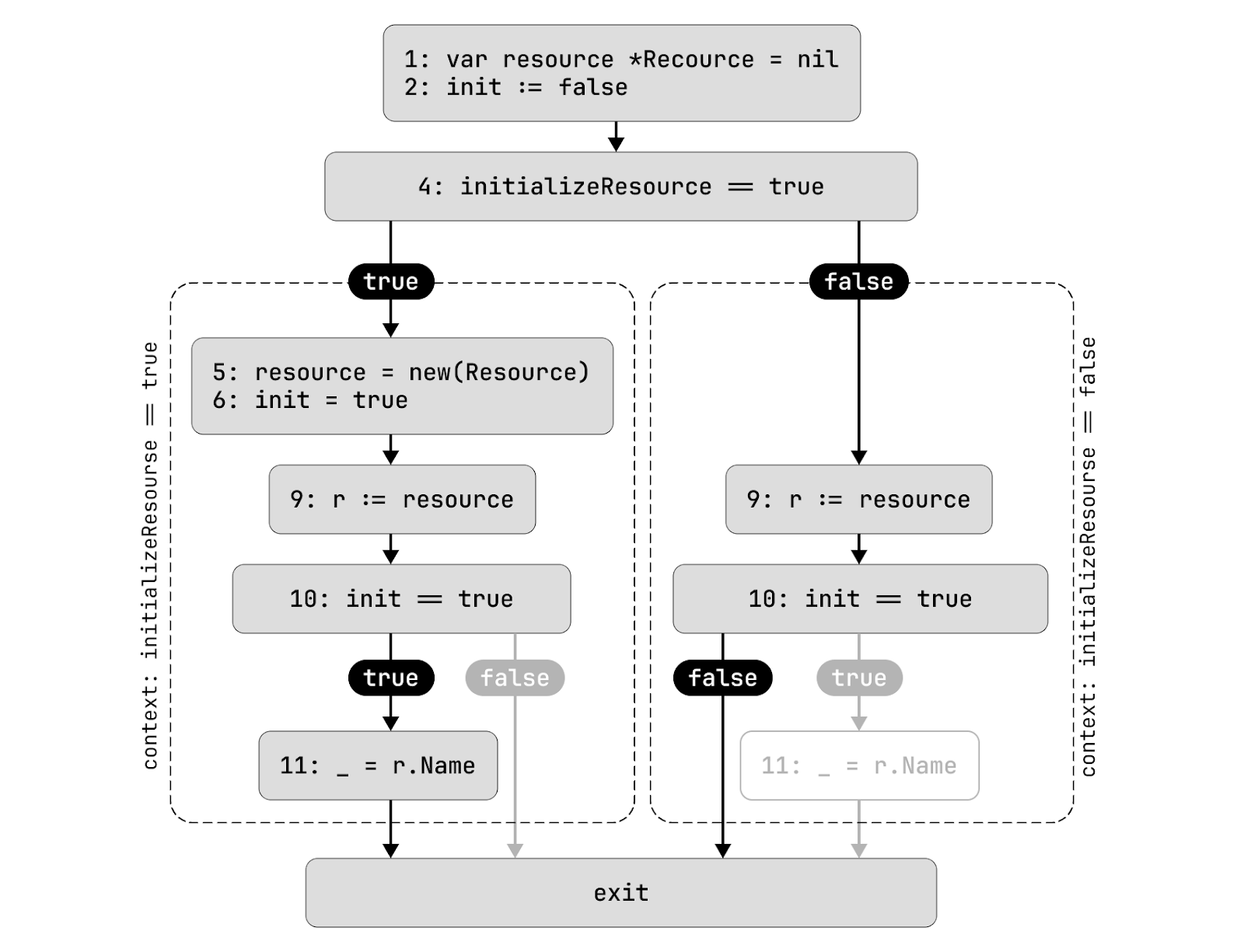
As you’ll be able to see, there are two totally different contexts (surrounded by a dashed border). In every context, we are able to analyze statements 9, 10, and 11 in several methods. For instance, in assertion 10 of the context initializeResource == true
, the variable init can solely take a true
worth, and variable r
can solely take a new(Useful resource)
worth. Due to this fact, on this context, assertion 11 is reachable, however a nil
dereference isn’t doable. In assertion 10 of the context initializeResource == false
, variable r
can take a nil
worth. Because the worth of init
can solely be false
, assertion 11 will not be reachable on this context and due to this fact a nil
dereference isn’t doable. As such, a nil
dereference can not happen in both context.
Utilizing contexts in static evaluation permits us to enhance the standard of the evaluation and weed out false-positives. To help this, exit statements of the if assertion are break up into two totally different contexts, duplicating the following nodes of the management stream graph and analyzing them independently to determine all of the doable knowledge paths.
The capabilities of information stream evaluation in GoLand
Fixed situations detection. Fixed situations symbolize an important sort of information stream inspection. The fixed situation inspection makes use of the DFA execution knowledge to find out if sure situations are fixed. Listed here are two examples:
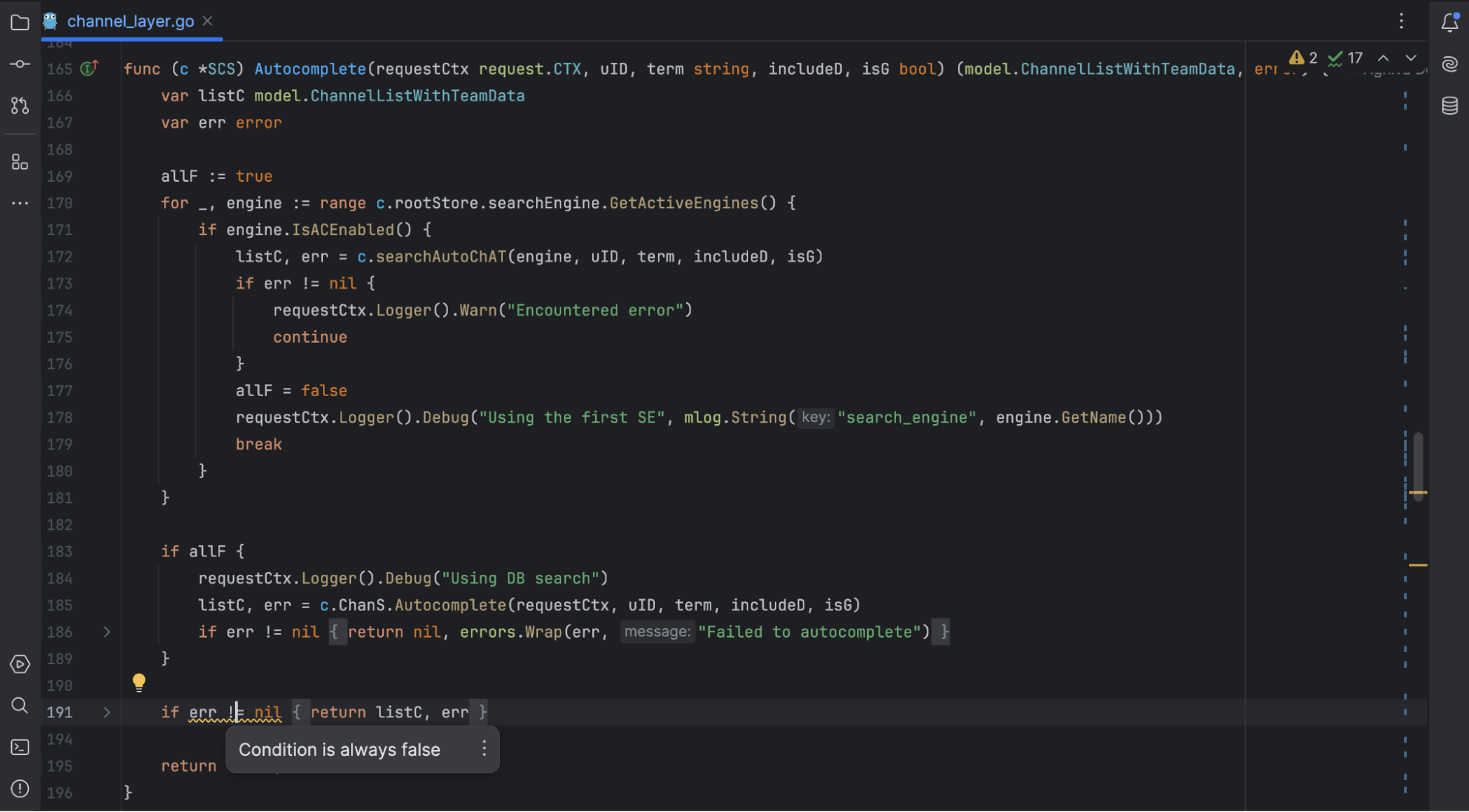
Instance 1: On this instance, DFA has deduced that the situation err != nil
is all the time false
. To point out that that is certainly the case, let’s take into account what values the err
variable can take within the situation on line 191. There are two foremost instances – when allF
is true and when allF
is fake after the for
loop is executed. If allF
is true
after the for
loop is executed, then err
will likely be nil
on line 191, in any other case there will likely be a return
from the perform on line 186. The remaining eventuality, when allF
is false
after the for
loop is executed, can solely occur if line 177 is reachable. After line 177 is executed, we will be positive of two issues: firstly, that err
is nil
(in any other case the execution of the loop would have continued on line 175), and secondly, that the execution of the loop has been interrupted, and due to this fact the variable err
received’t be assigned every other worth. Therefore, in instances the place allF
is false
, the variable err
can solely ever be nil
. Thus, the situation err != nil
is all the time false
.

Instance 2: Here’s a easier instance wherein DFA deduces that the situation r0k != nil
on line 193 is all the time true
. This occurs as a result of the implicit dereference r0k.License
is current on line 191, after which the variable r0k
can’t be nil
. Though the derived fixed situation inspection doesn’t precisely present an actual drawback within the code, it reveals unusual habits in this system. In truth, points might change into obvious at runtime after the potential nil dereference of the variable r0k
on line 191, because the writer of the code implies that r0k
will be nil
.
These examples present how the fixed situation inspection can will let you determine peculiar factors or unusual habits in this system’s code.
Potential nil
dereference. DFA can detect a nil
dereference for a variable even in code that appears completely regular to the bare eye. Let’s see how that is doable:
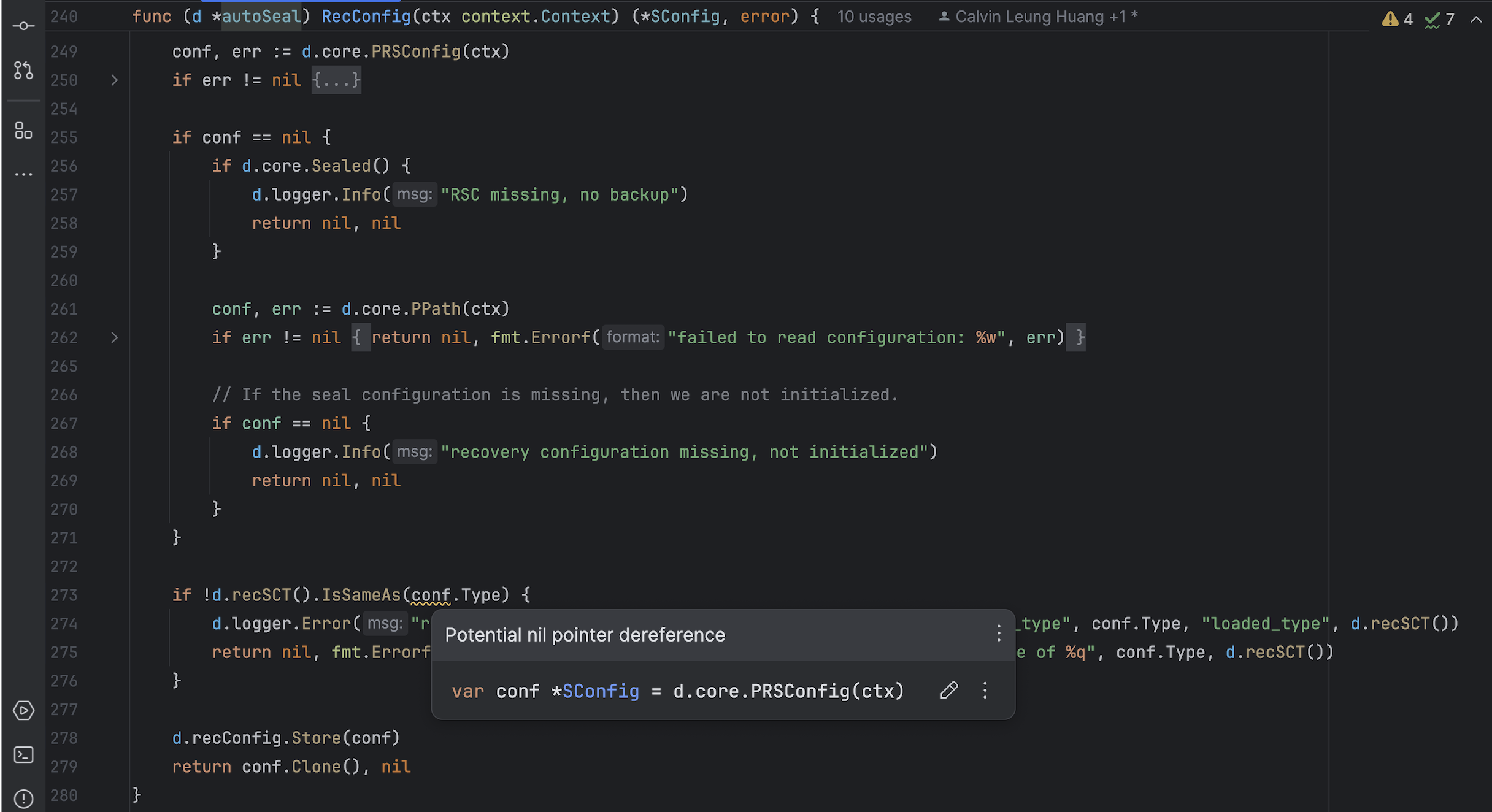
Instance 3: On this instance, DFA infers that there could be a nil
dereference of the variable conf on line 273. This may occasionally appear unusual as a result of the code takes under consideration instances the place the variable conf
is nil
. In such a case, there needs to be a return from the perform on line 269, and so dereference of the conf
variable shouldn’t be reachable. Nonetheless, there are literally two totally different conf
variables. The primary one is asserted on line 249 and the second on line 261. Thus, the final declaration shadows the earlier one.
This could result in a 0 dereference. Let’s assume that the primary conf
variable (line 249) is nil
however the second conf variable (line 261) will not be nil
, and let’s additionally assume that the corresponding error
variable is nil
. On this case, there isn’t any return
from the perform on strains 262 and 269, which implies we attain the conf
dereference on line 273. This dereference corresponds to the primary conf
variable and causes points at runtime. For that reason, we needs to be cautious with nested quick variable declarations!
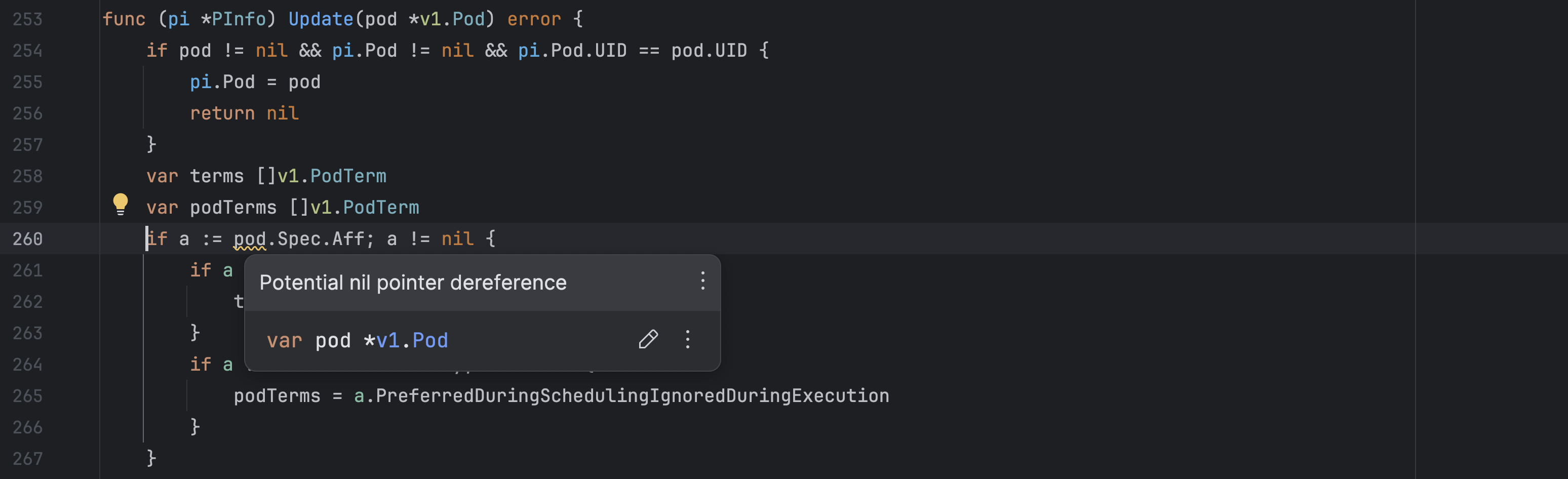
Instance 4: DFA deduces a possible nil
dereference of the variable pod
on line 260. This perform takes under consideration the nilablility of the variable pod
(there’s a situation pod != nil
on line 254), so the writer of the code implies that the variable pod
will be nil
inside the if
assertion. However on this case, there will likely be a nil
dereference on line 260.
Error could also be not nil
. This inspection experiences instances wherein variables may need nil
or an surprising worth due to the related error that’s not checked for being non-nil
.
The evaluation is at the moment intra-procedural and doesn’t take into account user-imposed contracts on the perform. Due to this fact, in particular conditions, there could also be false positives. For instances reminiscent of these, you need to use a quick-fix to ask the DFA to not analyze or report these errors.
func _() { file, err := os.Open("file.txt") // Error test is omitted right here title := file.Title() print(title, err) }
Within the instance offered, the variable file might both have the worth nil or an surprising worth if err will not be nil.
Attempt DFA for your self!
You possibly can check out these enhancements now within the GoLand 2024.1 Launch Candidate or await the 2024.1 model. It’s additionally obtainable for 2023.3 customers in early entry, however is disabled by default. To allow it, go to Settings | Editor | Inspections | Go | Information Circulate Evaluation (experimental).