Fast reply: How you can put a easy if-then-else assertion in a single line of Python code?
To place an if-then-else assertion in a single line, use Python’s ternary operator x if c else y
. This returns the results of expression x
if the Boolean situation c
evaluates to True
. In any other case, the ternary operator returns the choice expression y
.
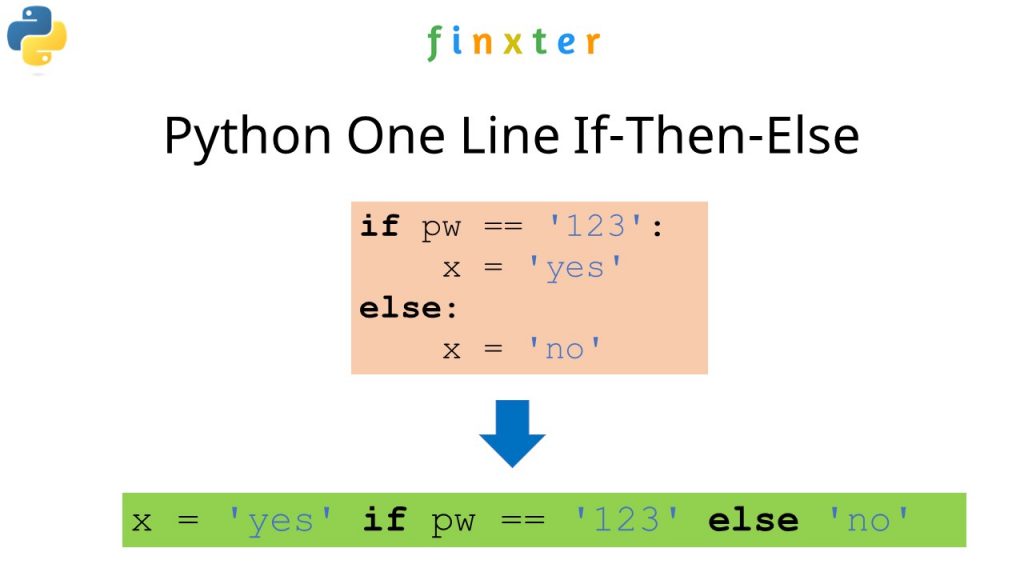
When you learn by the article to spice up your one-liner energy, you possibly can hearken to my detailed video clarification:
Right here’s one other minimal instance the place you come 21
if the situation 3>2
evaluates to True
, in any other case, you come 42
:
var = 21 if 3<2 else 42 # var == 42
The output 42
is saved within the variable var
.
Introduction and Overview
Python is so highly effective, which you can even compress complete algorithms in a single line of code.
(I’m so fascinated about this that I even wrote a complete e book with NoStarch on Python One-Liners:)
However earlier than we transfer on, I’m excited to current you my new Python e book Python One-Liners (Amazon Hyperlink).
Should you like one-liners, you’ll LOVE the e book. It’ll train you every part there may be to learn about a single line of Python code. Nevertheless it’s additionally an introduction to laptop science, information science, machine studying, and algorithms. The universe in a single line of Python!
The e book was launched in 2020 with the world-class programming e book writer NoStarch Press (San Francisco).
Hyperlink: https://nostarch.com/pythononeliners
So the pure query arises: are you able to write conditional if-then-else statements in a single line of code?
This text explores this mission-critical query in all element.
Are you able to write the if-then-else assertion in a single line of code?
Sure, you possibly can write most if
statements in a single line of Python utilizing any of the next strategies:
- Write the if assertion with out else department as a Python one-liner:
if 42 in vary(100): print("42")
. - If you wish to set a variable, use the ternary operator:
x = "Alice" if "Jon" in "My identify is Jonas" else "Bob"
. - If you wish to conditionally execute a operate, nonetheless use the ternary operator:
print("42") if 42 in vary(100) else print("21")
.
Within the earlier paragraph, you’ve unwillingly realized in regards to the ternary operator in Python.
The ternary operator is one thing you’ll see in most superior code bases so be certain that to grasp it completely by studying the next part of this text.
I drew this image to point out visually how the ternary operator works:
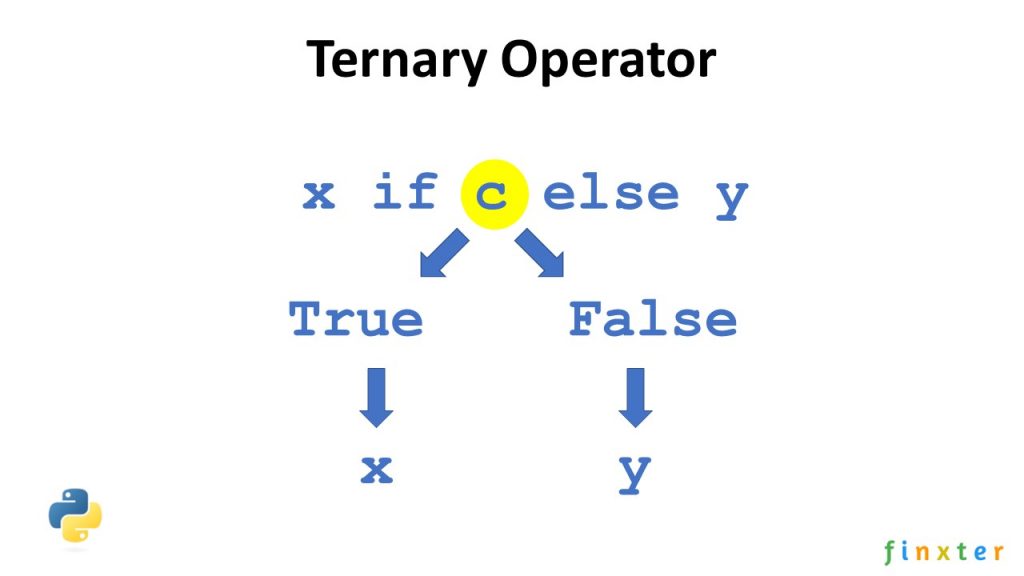
Let’s dive into the three alternative ways to write the if-then-else assertion as a Python one-liner.
Associated articles: Python One Line Ternary
How you can Write the If-Then-Else Assertion as a Python One-Liner?
Let’s take a look in any respect the methods how one can write the if-then-else assertion in a single line.
The trivial reply is to only write it in a single line—however provided that you don’t have an else department:
Case 1: You Don’t Have an Else Department
Take into account the next code snippet the place you examine for the quantity 42 whether or not it falls in a variety of numbers:
>>> if 42 in vary(100): >>> print("42") 42
This code snippet will certainly print the output as a result of the integer 42 falls into the vary of numbers from 0 to 99.
However how can we write this if
assertion in a single line of code?
Simply use a single line like this:
>>> if 42 in vary(100): print("42") 42
The 2 statements are similar so that is the best way to do it—for those who can write the conditional physique in a single line of code.
Nevertheless, for those who attempt to change into too fancy and you utilize a nested if
physique, it received’t work:
>>> if 42 in vary(100): print("42") else print("21") # "Error: invalid syntax"
Python can not deal with this anymore: the interpreter throws an “invalid syntax” error as a result of the assertion grew to become ambiguous.
However don’t fear, there’s a workaround: the ternary operator.
Case 2: You Have an Else Department And You Need to Conditionally Assign a Worth
In case you may have an else department and also you need to conditionally assign a price to a variable, the ternary operator is your buddy.
Say, you need to write the next if-then-else assertion in a single line of code:
>>> if "Jon" in "My identify is Jonas": >>> x = "Alice" >>> else: >>> x = "Bob" >>> print(x) Alice
Because the string "Jon"
seems within the string "My identify is Jonas"
, the variable x
will take worth "Alice"
.
Can we write it in a single line? Certain—through the use of the ternary operator.
x = "Alice" if "Jon" in "My identify is Jonas" else "Bob"
The ternary operator could be very intuitive. Simply learn it from left to proper and also you’ll perceive its that means.
We assign the worth "Alice"
to the variable x
in case the next situation holds: "Jon"
in "My identify is Jonas"
. In any other case, we assign the string "Bob"
to the variable x
.
Ternary Operator Syntax: The three operands are written as x if c else y
which reads as “return x
if c
else return y
“. Let’s write this extra intuitively as:
<OnTrue> if <Situation> else <OnFalse>
Operand | Description |
---|---|
<OnTrue> |
The return expression of the operator in case the situation evaluates to True |
<Situation> |
The situation determines whether or not to return the <On True> or the <On False> department. |
<OnFalse> |
The return expression of the operator in case the situation evaluates to False |
Case 3: What If You Don’t Need to Assign Any Worth However You Have an Else Department?
Effectively, there’s a fast and soiled hack: simply ignore the return worth of the ternary operator.
Say, we need to compress the next if-then-else assertion in a single line of code:
if 42 in vary(100): print("42") else: print("21")
The issue is that we don’t have a return worth. So can we nonetheless use the ternary operator?
Because it seems, we will. Let’s write this if-then-else assertion in a single line:
>>> print("42") if 42 in vary(100) else print("21") 42
We use the ternary operator. The return worth of the print()
operate is solely None
. However we don’t actually care in regards to the return worth, so we don’t retailer it in any variable.
We solely care about executing the print
operate in case the if
situation is met.
How you can Write an If-Elif-Else Assertion in a Single Line of Python?
Within the earlier paragraphs, you’ve realized that we will write the if-else assertion in a single line of code.
However are you able to one-linerize an elif
expression with a number of circumstances?
In fact, you possibly can!
(Heuristic: Should you’re unsure about whether or not you are able to do XYZ in a single line of Python, simply assume which you can. See right here.)
Say, you need to write the next if-then-else situation in a single line of code:
>>> x = 42 >>> if x > 42: >>> print("no") >>> elif x == 42: >>> print("sure") >>> else: >>> print("possibly") sure
The elif
department wins! We print the output "sure"
to the shell.
However the right way to do it in a single line of code? Simply use the ternary operator with an elif
assertion received’t work (it’ll throw a syntax error).
The reply is easy: nest two ternary operators like so:
>>> print("no") if x > 42 else print("sure") if x == 42 else print("possibly") sure
If the worth x
is bigger than 42
, we print "no"
to the shell.
In any other case, we execute the rest of the code (which is a ternary operator by itself). If the worth x
is the same as 42
, we print "sure"
, in any other case "possibly"
.
So by nesting a number of ternary operators, we will significantly enhance our Python one-liner energy!
Earlier than you and I transfer on, let me current our brand-new Python e book Python One-Liners.
Should you like one-liners, you’ll LOVE the e book. It’ll train you every part there may be to learn about a single line of Python code. Nevertheless it’s additionally an introduction to laptop science, information science, machine studying, and algorithms. The universe in a single line of Python!

The e book is launched in 2020 with the world-class programming e book writer NoStarch Press (San Francisco).
Associated Questions
Let’s rapidly deal with a bunch of associated questions:
How you can Write If With out Else in One Line?
We’ve already seen an instance above: we merely write the if
assertion in a single line with out utilizing the ternary operator: if 42 in vary(100): print("42")
.
Python is completely in a position to perceive a easy if assertion with out an else department in a single line of code.
How you can Write Elif in One Line?
We can not straight write the elif
department in a single line of Python code. However we will nest two ternary operators as an alternative:
>>> 100 if x > 42 else 42 if x == 42 else 0 42
Python If-Else One-Liner: What Does It Return?
The ternary operator at all times returns the results of the conditional analysis. Once more, the code snippet 100 if x>42 else 42
returns the integer worth 42.
Should you solely execute capabilities throughout the ternary operator, it’ll return the None
worth.
Associated Video Tutorial
Grasp the ability of the only line of code—get your Python One-Liners e book now! (Amazon Hyperlink)
The place to Go From Right here
Realizing small Python one-liner methods such because the ternary operator is significant on your success within the Python language. Each professional coder is aware of them by coronary heart—in spite of everything, that is what makes them very productive.
If you wish to study the language Python by coronary heart, be part of my free Python electronic mail course. It’s 100% based mostly on free Python cheat sheets and Python classes. It’s enjoyable, straightforward, and you’ll go away anytime. Strive it!
Python One-Liners Ebook: Grasp the Single Line First!
Python programmers will enhance their laptop science abilities with these helpful one-liners.
Python One-Liners will train you the right way to learn and write “one-liners”: concise statements of helpful performance packed right into a single line of code. You’ll discover ways to systematically unpack and perceive any line of Python code, and write eloquent, powerfully compressed Python like an professional.
The e book’s 5 chapters cowl (1) ideas and methods, (2) common expressions, (3) machine studying, (4) core information science matters, and (5) helpful algorithms.
Detailed explanations of one-liners introduce key laptop science ideas and increase your coding and analytical abilities. You’ll study superior Python options similar to record comprehension, slicing, lambda capabilities, common expressions, map and scale back capabilities, and slice assignments.
You’ll additionally discover ways to:
- Leverage information constructions to resolve real-world issues, like utilizing Boolean indexing to seek out cities with above-average air pollution
- Use NumPy fundamentals similar to array, form, axis, kind, broadcasting, superior indexing, slicing, sorting, looking out, aggregating, and statistics
- Calculate primary statistics of multidimensional information arrays and the Okay-Means algorithms for unsupervised studying
- Create extra superior common expressions utilizing grouping and named teams, damaging lookaheads, escaped characters, whitespaces, character units (and damaging characters units), and grasping/nongreedy operators
- Perceive a variety of laptop science matters, together with anagrams, palindromes, supersets, permutations, factorials, prime numbers, Fibonacci numbers, obfuscation, looking out, and algorithmic sorting
By the tip of the e book, you’ll know the right way to write Python at its most refined, and create concise, lovely items of “Python artwork” in merely a single line.
Get your Python One-Liners on Amazon!!
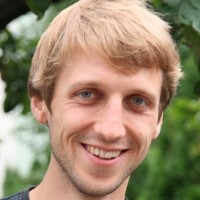
Whereas working as a researcher in distributed methods, Dr. Christian Mayer discovered his love for instructing laptop science college students.
To assist college students attain larger ranges of Python success, he based the programming training web site Finxter.com. He’s creator of the favored programming e book Python One-Liners (NoStarch 2020), coauthor of the Espresso Break Python collection of self-published books, laptop science fanatic, freelancer, and proprietor of one of many prime 10 largest Python blogs worldwide.
His passions are writing, studying, and coding. However his best ardour is to serve aspiring coders by Finxter and assist them to spice up their abilities. You possibly can be part of his free electronic mail academy right here.