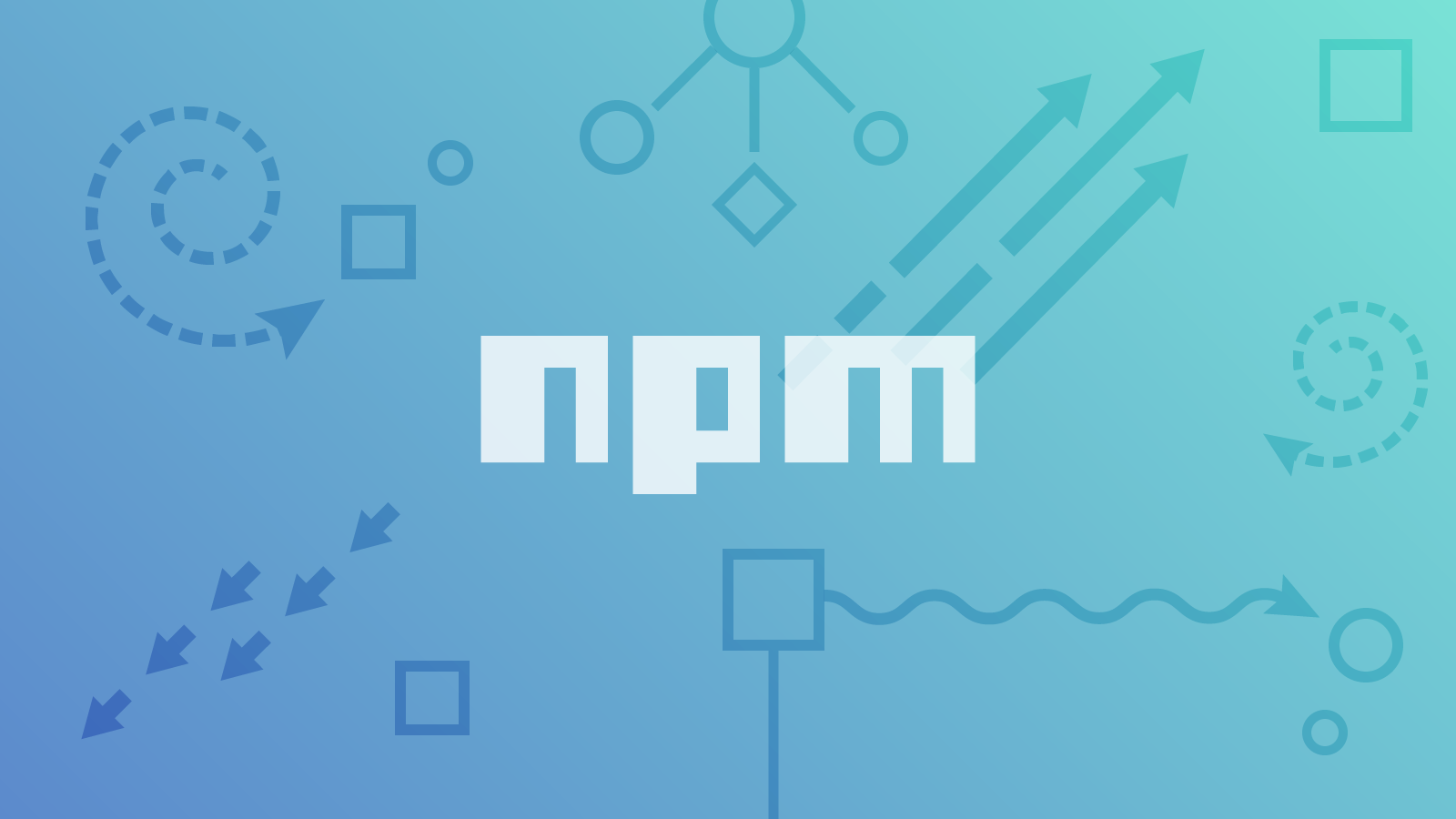
On the subject of constructing a easy front-end challenge, how do you get began? What are the instruments you want? I believe everybody could have a distinct reply. Do you begin with a (JS or CSS) framework, or off-the-shelf boilerplate? Maybe you employ a activity runner (like Gulp to orchestrate your challenge’s wants. Or do you begin easy, with simply HTML and a CSS file?
The front-end tooling panorama might be complicated, and at instances overwhelming – and whenever you’re dedicating your time to studying HTML, CSS and Javascript, it appears like one more factor it’s essential to make time to be taught. On this collection of articles I need to assist builders perceive among the instruments and methodologies which have turn out to be commonplace for constructing internet tasks. Over the subsequent three articles we’ll construct a easy challenge starter (or boilerplate) collectively. We’ll cowl:
- An introduction to utilizing NPM scripts (this text) for compiling Sass, working a server and reside reloading.
- Getting up and working with Parcel, a minimal-config utility bundler.
- Constructing out a reusable Sass structure
Be happy to skip over the components you’re already aware of.
Why do we’d like a challenge starter repository?
I’ve written beforehand on this weblog about conserving issues easy and constructing dependency-free — and for a primary, minimal web site, this strategy has so much to advocate it. However the overwhelming majority of my tasks would profit from a bit extra tooling. In any given challenge, it’s seemingly that on the very least I’ll need to:
- Run a neighborhood server
- Compile SCSS to CSS, and minify the output
- Stay reload (present adjustments within the browser with out the necessity for handbook refresh)
- Optimise photographs
- Create SVG icon sprites
In bigger tasks, there are a lot extra tooling choices we might add into the combo to assist us construct performant, accessible web sites. We would need module bundling, code splitting and transpiling. On the CSS facet, maybe we’d prefer to inline our crucial CSS, or purge unused selectors.
If you happen to don’t know what a few of these phrases imply, you’re not alone! Entrance-end improvement has obtained much more advanced lately, and it may be arduous to maintain abreast of the fixed adjustments to finest practices. One article that has actually helped me perceive the huge tooling panorama that nowadays falls into the realm of front-end improvement is Fashionable Javascript Defined For Dinosaurs. Though a few years outdated, this text remains to be extraordinarily related, and explains succinctly how Javascript has developed to turn out to be such a significant a part of our workflow.
All this takes time to arrange and configure, and to do it from scratch each time we begin a brand new challenge wouldn’t be perfect. Which is why it’s helpful to have a starter repository that we will clone or obtain, with the whole lot we have to begin coding immediately.
Selecting our instruments
I’m not an individual who loves spending time organising advanced tooling. I need my instruments to demand as little time from me as doable, in order that I can think about the issues I really like doing! While I’ve used Gulp up to now, it now appears a much less essential a part of the toolchain: just about all dependencies might be put in through NPM and configuring them with NPM scripts isn’t any tougher than configuring them with Gulp. So utilizing a activity runner appears a bit redundant, and would solely add an additional dependency to the challenge.
The instruments I’ve chosen listed below are a private desire, and go well with the sort of tasks I prefer to construct. They’re not essentially everybody’s selection, and there are many alternative ways to do issues. However I hope this tutorial will aid you get a bit extra aware of among the instruments which have turn out to be fashionable amongst builders, as a way to make your personal decisions.
With that in thoughts, let’s start constructing our challenge starter, and be taught concerning the instruments we’ll be utilizing alongside the best way. Be happy to skip over any components you’re already aware of.
Putting in Node.js
The very very first thing we have to do to get our challenge set as much as work with NPM scripts is to verify we’ve got Node.js put in globally. This sounds easy sufficient, however already issues begin to get a little bit extra difficult once we realise there are a variety of various methods to do that:
- Obtain the newest model from the web site and observe the directions for set up
- Use a bundle supervisor like Homebrew for Mac, which permits us to replace our node model with a easy command:
brew improve node
. - Utilizing NVM (Node Model Supervisor).
NVM is my most well-liked possibility, because it permits us to simply improve our node model, see which model we’re at the moment working, checklist different put in variations or swap to a different model utilizing single instructions. Nevertheless it requires further steps to put in relying in your setup, which is past the scope of this explicit article.
Upon getting Node put in (by whichever technique fits you), you’ll be able to examine the at the moment put in model by working node -v
. (You may need to improve to the newest model.) If you happen to’re utilizing NVM you would (optionally) create a .nvmrc config file to make sure you at all times run the proper Node model in your challenge.
NPM
Putting in Node additionally installs NPM (Node Bundle Supervisor). That is principally an enormous library of open supply Javascript improvement instruments (or packages) that anybody can publish to. We have now direct entry to this library of instruments and (for higher or worse!) can set up any of them in our tasks.
NPM or Yarn?
Yarn is another bundle supervisor, much like NPM, and nearly as fashionable. Actually, many individuals take into account it an enchancment. It may be utilized in an analogous approach, to put in dependencies. If you happen to desire to make use of Yarn over NPM, you’ll be able to safely substitute NPM instructions with the Yarn equal anyplace they’re used on this article.
Initialising the challenge
First, let’s create a brand new challenge folder, which we’ll (imaginitively) name new-project. Open the terminal, and inside that folder run:
npm init
Operating this command brings up a number of steps for initialising our challenge within the command line, akin to including a reputation and outline. You possibly can hit Enter to skip by every of those for those who don’t need to full them immediately – we’ll have the ability to edit them in a while. You’ll then see {that a} bundle.json file has been created, which ought to look one thing like this:
{
"title": "project-starter",
"model": "1.0.0",
"description": "",
"essential": "index.js",
"scripts": {
"take a look at": "echo "Error: no take a look at specified" && exit 1"
},
"writer": "",
"license": "ISC"
}
This file incorporates all of the details about our challenge, and is the place we will edit the main points that we simply skipped by.
Any packages that we set up from NPM will probably be robotically listed within the bundle.json file. It’s additionally the place we’ll configure the scripts that may construct and run our challenge. We’ll set up some packages and configure these shortly, however first we’ll want a primary challenge structure, and a few information to work with.
Venture construction
We’ll begin with a folder construction that appears like this:
new-project
index.html
src
icons
photographs
js
scss
node_modules
bundle.json
We’ve already generated the node_modules
listing and bundle.json within the root of the challenge. We simply have to create a listing referred to as src, containing directories for photographs, JS, SCSS and icons, plus an index.html file.
Creating our folder construction from the command line
You could possibly create the above folder construction manually, both in your textual content editor of selection or in your pc’s file system. However if you wish to save time, you would do it from the terminal as an alternative. Within the root of the challenge, you would run:
contact index.html
mkdir src && cd src
mkdir js scss photographs icons
cd ../
Line by line, this code:
- Creates a brand new index.html file
- Creates a brand new src listing and strikes us into the newly-created listing
- Creates directories inside src referred to as js, scss, photographs and icons, and a file referred to as index.html.
- Brings us again as much as the challenge root.
Now let’s add the next to our index.html file in order that we will see our web site within the browser:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta title="viewport" content material="width=device-width, initial-scale=1.0" />
<meta http-equiv="X-UA-Appropriate" content material="ie=edge" />
<title>Venture starter</title>
<hyperlink rel="stylesheet" kind="textual content/css" href="dist/css/types.css" />
</head>
<physique>
<h1>Hey world!</h1>
</physique>
</html>
Putting in dependencies
Now that we’ve got our primary folder construction, we will begin to set up some packages and write some NPM scripts that may allow us to construct and examine our web site. The scripts we’re going to jot down will:
- Run a neighborhood server
- Compile Sass to CSS
- Look ahead to adjustments and reload the web page at any time when we replace our HTML or CSS
Let’s set up the node-sass bundle from NPM, which compiles .scss information to CSS. Within the terminal run:
npm set up node-sass --save-dev
As soon as this command has completed working, it is best to see a few new issues:
- A listing referred to as
node_modules
has been created - Within the bundle.json file,
node-sass
is now listed in “devDependencies”. - Provides a package-lock.json file. This isn’t one thing we should always ever want to the touch.
Including a .gitignore
The node_modules
listing is the place the code for all of our challenge dependencies will reside. The contents of this folder ought to not be dedicated to Github (or your favorite repository host), as putting in just some dependencies might lead to a whole lot of 1000’s of information! So the subsequent factor we should always do is add a .gitignore file within the challenge root:
contact .gitignore && echo "node_modules" >> .gitignore
This command creates the .gitignore file and provides node_modules
to it. (Once more, you are able to do this manually for those who desire.) Now we’re protected within the information that our packages is not going to be dedicated.
If we’re not committing these information, then how can we share our dependencies with different customers? Properly, this all the way down to the bundle.json file. It tells us the title and model variety of any dependencies we’ve got put in. Anybody who clones or forks the challenge (together with us, once we use it to start out a brand new challenge) can merely run npm set up
and all of the related dependencies will probably be fetched and downloaded from NPM.
Forms of dependencies
Once we put in node-sass we ran the set up
command with the --save-dev
flag. This installs the challenge as a “dev dependency”. Different packages could not require this command, and save a bundle underneath “dependencies” as an alternative. The distinction is that common dependencies are runtime dependencies, whereas dev dependencies are buildtime dependencies. node-sass is required to construct your challenge, so it’s a dev dependency. However one thing like, say, a carousel plugin, or framework that must be downloaded on the shopper facet, (like React) would must be an everyday dependency.
Now we’ll additionally set up Browsersync as a dev dependency. Browsersync will run a neighborhood server and reload the browser when our information change.
npm set up browser-sync --save-dev
Writing NPM scripts
Now it’s time to jot down some scripts to run our challenge. We’re going to jot down these within the “scripts” part of our bundle.json.
Sass to CSS
NPM scripts encompass a key (the title of the script, which is what we’d kind within the terminal with a purpose to run it) and a worth – the script itself, which will probably be executed once we run the command. First we’ll write the script which compiles Sass to CSS. We’ll give it the title scss (we might title it something we like) and add it to our “scripts” part:
"scripts": {
"scss": "node-sass --output-style compressed -o dist/css src/scss",
}
The node-sass bundle incorporates some choices, a few of which we’re defining right here. We’re specifying the output fashion (“compressed”), the output listing (dist/css) and the supply listing (src/scss), which is at the moment empty. Let’s create a supply .scss file from the terminal:
contact src/scss/types.scss
Add a number of types to the newly-created file, then return to the terminal and run:
npm run scss
You need to then see a brand new listing referred to as dist has been created, containing your compiled CSS. Now, each time you make adjustments to your types.scss file, you’ll be able to run the script and people adjustments will probably be compiled.
Stay reloading
Our first script is working nice, but it surely’s not very helpful but, as each time we make adjustments to our code we have to obtained again to the terminal and run the script once more. What we’d be a lot better it to run a neighborhood server and see our adjustments mirrored instantaneously within the browser. In an effort to do this we’ll write a script that makes use of Browsersync, which we’ve already put in.
First, let’s write the script that runs the server, which we’ll name serve:
"scripts": {
"scss": "node-sass --output-style compressed -o dist/css src/scss",
"serve": "browser-sync begin --server --files 'dist/css/*.css, **/*.html'"
}
Within the --files
possibility we’re itemizing the information that Browsersync ought to monitor. It’s going to reload the web page when any of those change. If we run this script now (npm run serve
), it would begin a neighborhood server and we will preview our internet web page by going to http://localhost:3000 within the browser.
Expecting adjustments
At present we nonetheless have to run our scss script once we need to compile our Sass. What we’d like our scripts to do is:
- Watch our src/scss listing for adjustments.
- When a change happens, compile this to CSS in dist/css.
- When dist/css is up to date, reload the web page.
First we have to set up an NPM bundle referred to as onchange, to look at for adjustments to the supply information:
npm set up onchange --save-dev
We are able to write NPM scripts that run different scripts. Let’s add the script that watches for adjustments and triggers our scss command to run:
"scripts": {
"scss": "node-sass --output-style compressed -o dist/css src/scss",
"serve": "browser-sync begin --server --files 'dist/css/*.css, **/*.html'",
"watch:css": "onchange 'src/scss' -- npm run scss",
}
The watch:css script watches for adjustments utilizing the onchange bundle (src/scss) and runs our scss script when adjustments happen.
Combining scripts
Now we have to run two instructions in parallel: The serve command to run our server, and the watch:css command to compile our Sass to CSS, which is able to set off the web page reload. Utilizing NPM scripts we will simply run instructions consecutively utilizing the && operator:
"scripts": {
/*...*/
"begin": "npm run serve && npm run scss"
}
Nonetheless, this gained’t obtain what we would like, because the script will wait till after the serve script has completed working earlier than it begins working the scss script. If we go forward and write this script, then run it within the terminal (npm begin
), then npm run scss
gained’t be triggered till we’ve stopped the server.
To allow us to run instructions in parallel, we have to set up one other bundle. NPM has a number of choices to select from. The one I’ve picked is npm-run-all:
npm set up npm-run-all --save-dev
The primary choices on this bundle (or a minimum of, those we care about) are run-s and run-p. The previous is for working sequentially, the latter is for working instructions in parallel. As soon as we’ve got put in this bundle, we will use it to jot down the script that runs each our serve and watch:css instructions in parallel. (We’ll name it begin.)
"scripts": {
"scss": "node-sass --output-style compressed -o dist/css src/scss",
"serve": "browser-sync begin --server --files 'dist/css/*.css, **/*.html'",
"watch:css": "onchange 'src/scss' -- npm run scss",
"begin": "run-p serve watch:css"
}
We now have a really primary starter challenge. We’ve written some scripts that permit us to easily kind the command npm begin
to run a server, look ahead to adjustments, compile Sass to CSS and reload the web page. An instance repository might be discovered right here.
We might now go forward and set up some packages and write scripts to automate a few of our different duties, akin to optimising photographs, creating SVG sprites and uglifying JS. This CSS Tips article has an excellent rundown of some extra scripts you may like so as to add, in addition to a starter repository. (Bear in mind, one or two of the packages included within the instance have since been deprecated. You might want to go looking NPM for substitutes.)
This may occasionally serve us completely nicely for small tasks, however the extra duties we need to run, the extra scripts we’ll want to jot down, and orchestrating all of them turns into extra advanced. So, within the subsequent article we’ll have a look at how Parcel, an utility bundler, can automate lots of these duties for us with minimal configuration, and supply the tooling we’d like with a purpose to construct bigger tasks.