Introduction
One of many first issues I discovered about in Go was utilizing an uppercase or lowercase letter as the primary letter when naming a sort, variable or operate. It was defined that when the primary letter was capitalized, the identifier was public to any piece of code that needed to make use of it. When the primary letter was lowercase, the identifier was personal and will solely be accessed throughout the package deal it was declared.
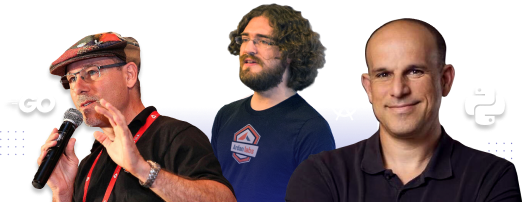
I’ve come to appreciate that using the language private and non-private is actually not correct. It’s extra correct to say an identifier is exported or unexported from a package deal. When an identifier is exported from a package deal, it means the identifier may be immediately accessed from some other package deal within the code base. When an identifier is unexported from a package deal, it might probably’t be immediately accessed from some other package deal. What we are going to quickly be taught is that simply because an identifier is unexported, it doesn’t imply it might probably’t be accessed outdoors of its package deal, it simply means it might probably’t be accessed immediately.
Direct Identifier Entry
Let’s begin with a easy instance of an exported sort:
Right here we outline a named sort referred to as AlertCounter contained in the package deal counters. This kind is an alias for the built-in sort int, however in Go AlertCounter shall be thought of a singular and distinct sort. We’re utilizing the capital letter ‘A’ as the primary letter for the identify of the kind, which implies this sort is exported and accessible by different packages.
Now let’s entry our AlertCounter sort in the principle program:
For the reason that AlertCounter sort has been exported, this code builds tremendous. Once we run this system we get the worth of 10.
Now let’s change the exported AlertCounter sort to be an unexported sort by altering the identify to alertCounter and see what occurs:
After making the adjustments to the counters and major packages, we try and construct the code once more and get the next compiler error:
./major.go:11: can’t confer with unexported identify counters.alertCounter
./major.go:11: undefined: counters.alertCounter
As anticipated we will’t immediately entry the alertCounter sort as a result of it’s unexported. Though we can’t entry the alertCounter sort immediately anymore, there’s a approach for us to create and use variables of this unexported sort in the principle package deal:
Within the counters package deal we add an exported operate referred to as NewAlertCounter. This operate creates and returns values of the alertCounter sort. In the principle program we use this operate and the programming logic stays the identical.
What this instance reveals is that an identifier that’s declared as unexported can nonetheless be accessed and utilized by different packages. It simply can’t be accessed immediately.
Utilizing Structs
Defining exported and unexported members for our structs work in the very same approach. If a area or methodology identify begins with a capital letter, the member is exported and is accessible outdoors of the package deal. If a area or methodology begins with a lowercase letter, the member is unexported and doesn’t have accessibility outdoors of the package deal.
Right here is an instance of a struct with each exported and unexported fields. The principle program has a compiler error as a result of it makes an attempt to entry the unexported area immediately:
Right here is the error from the compiler:
./major.go:14: unknown animal.Canine area ‘age’ in struct literal
As anticipated the compiler doesn’t let the principle program entry the age area immediately.
Let’s have a look at an attention-grabbing instance of embedding. We begin with two struct varieties the place one sort embeds the opposite:
We added a brand new exported sort referred to as Animal with two exported fields referred to as Title and Age. Then we embed the Animal sort into the exported Canine sort. Because of this the Canine sort now has three exported fields, Title, Age and BarkStrength.
Let’s have a look at the implementation of the principle program:
In major we use a composite literal to create and initialize a price of the exported Canine sort. Then we show the construction and values of the canine worth.
To make issues extra attention-grabbing, let’s change the Animal sort from exported to unexported by altering the primary letter of the kind’s identify to a lowercase letter ‘a’:
The animal sort stays embedded within the exported Canine sort, however now as an unexported sort. We hold the Title and Age fields throughout the animal sort as exported fields.
In the principle program we simply change the identify of the kind from Animal to animal:
As soon as once more now we have a major program that may’t compile as a result of we try to entry the unexported sort animal from contained in the composite literal:
./major.go:11: can’t confer with unexported identify animals.animal
./major.go:14: unknown animals.Canine area ‘animal’ in struct literal
We will repair the compiler error by initializing the exported fields from the unexported embedded sort outdoors of the composite literal:
Now the principle program builds once more. The exported fields that had been embedded into the Canine sort from the animal sort are accessible, despite the fact that they got here from an unexported sort. The exported fields hold their exported standing when the kind is embedded.
Customary Library
The exported Time sort from the time package deal is an effective instance of a sort from the usual library that gives no entry to its internals:
The language designers are utilizing the unexported fields to maintain the internals of the Time sort personal. They’re “hiding” the data so we will’t do something opposite to how the time knowledge works. With that being mentioned, we nonetheless can use the unexported fields by means of the interface they supply. With out the power to make use of and entry unexported fields not directly, we’d not be capable to copy values of this sort or embed this sort into our personal struct varieties.
Conclusion
A strong understanding of the right way to disguise and supply entry to knowledge from our packages is necessary. There’s much more to exporting and unexporting identifiers than meets the attention. In getting down to write this submit, I although a few examples would do the trick. Then I noticed how concerned the subject can get as soon as we begin embedding unexported varieties into our personal varieties.
The flexibility to make use of exported or unexported identifiers is an implementation element, one which Go give us flexibility to make use of in our packages. The usual library has nice examples of utilizing unexported identifiers to cover and defend knowledge. We checked out one instance with the time.Time sort. Take the time to have a look at the usual library to be taught extra.