This text was written by an exterior contributor.
Go is understood for its simplicity, efficiency, and full customary library. Out of the field, Go’s library helps many frequent operations that many different languages want third-party libraries to carry out or might not even help in any respect. These operations are contained in numerous packages that deal with options similar to concurrency, networking, I/O, and textual content processing.
database/sql is an ordinary library package deal liable for connectivity and interplay with databases (largely SQL relational ones). It gives generic interfaces, sorts, and strategies for SQL-like interactions. Although it helps many important fashionable database options, similar to transactions and ready statements, it additionally has some limitations. For instance, it suffers from a kind limitation through which massive uint64 values can’t be handed to statements as parameters.
This text compares the database/sql package deal with 3 different Go packages, particularly sqlx, sqlc, and GORM. The comparability of those 4 instruments focuses on 3 major areas:
- Options – Core options are explored, together with what makes the instruments distinctive and what they lack.
- Ease of use – Comparative ease of use is demonstrated utilizing code samples performing the identical operations for every software.
- Efficiency/velocity – Efficiency is measured by benchmarking a number of database operations utilizing the instruments from the usual library’s testing package deal.
Options
This part appears on the options supplied by every package deal. This comparability makes use of the bottom options of the database/sql package deal because the minimal requirement when it comes to options, as all of the packages have been created to increase the performance supplied by the default package deal.
database/sql
database/sql was created with simplicity in thoughts, configured to help probably the most primary however mandatory performance for interacting with SQL-like databases.
To work together with database administration programs, database packages want the suitable drivers. Presently, database/sql helps a listing of over 50 database drivers, protecting the most well-liked DBMSs similar to SQLite, MySQL/MariaDB, PostgreSQL, Oracle, and MS SQL Server.
This package deal additionally helps options similar to primary CRUD operations, database transactions, named parameters, returning a number of consequence units, cancellable queries, SQL sort help, connection pool administration, parameterized queries, and ready statements.
sqlx
sqlx was created to increase the options of the usual library database package deal. Because it depends on the database/sql package deal, the entire options supplied by which can be additionally out there, together with help for a similar set of database applied sciences and drivers.
Exterior of those core options, sqlx shines within the following methods:
- Ready statements with named parameters – These allow you to make use of the names of struct fields and map keys to bind variables in a ready assertion or question.
- Struct scanning – This lets you scan question outcomes straight into structs for a single row with out having to individually scan every discipline or column, as is the case with database/sql. It additionally helps scanning into embedded structs.
Choose
andGet
– These are handy strategies for dealing with queries anticipated to return a number of data or a single file right into a slice of a struct or a single struct, respectively. No have to loop on consequence units!- Help for IN queries – This lets you bind a slice of values as a single parameter to an IN question. The slice is unfold out with the identical variety of bindvars within the anticipated locations, in comparison with database/sql treating the slice as a single worth.
- Named queries – This binds the names of struct fields to column names, avoiding the necessity for positional references to column names when assigning values to bindvars.
- Error-free consequence units: Consequence units don’t return with errors, which permits for chainable operations on returned outcomes, similar to scanning outcomes straight right into a struct. This may be seen within the following snippet:
var p Place err := db.QueryRowx("SELECT metropolis, telcode FROM place LIMIT 1").StructScan(&p)
These are just a few examples of the various options of the sqlx package deal that guarantee a greater working expertise than database/sql.
sqlc
sqlc is an SQL compiler bundled as an executable binary file and might generate type-safe code on your uncooked SQL schema and queries. So, you don’t have to jot down any boilerplate code apart from your precise SQL statements.
In response to the documentation, it might probably generate code for PostgreSQL, MySQL/MariaDB, and SQLite. Nonetheless, the generated code additionally works with the usual library’s SQL package deal; subsequently, it might probably use all supported drivers however not essentially supported databases. Other than supported databases and drivers, the next are a few of its different options:
- Question annotations – These are numerous annotations that mean you can outline the title of the operate for every question and the kind of consequence anticipated from the question. These are used throughout code era to find out the title and signature of the operate.
- JSON tags – sqlc helps the era of JSON tags for structs or sorts that will probably be marshaled and despatched to shoppers as JSON.
- Schema modifications – sqlc has help for studying numerous codecs of migration recordsdata to change schema and generate code to replicate these modifications.
- Struct naming – sqlc gives choices for naming schemes used to generate struct names from desk names.
In case you’re good with SQL statements and like to not use a lot code to carry out database operations and deal with the info, that is positively your package deal.
GORM
GORM is a full-featured object-relational mapper (ORM) library for the Go language. It permits you to write queries as Go code by calling numerous strategies on objects, which GORM interprets into SQL queries when interacting with the database. It gives a wealthy set of options for database interactions, a few of that are listed under:
- Databases and drivers – In response to the official documentation, GORM helps MySQL/MariaDB, PostgreSQL, SQLite, SQL Server, and ClickHouse. It additionally gives the drivers for these database programs. All the identical, chances are you’ll go on an current database/sql connection to it, opening it as much as potential compatibility with all different databases and drivers supported by the database/sql package deal.
- Migrations – GORM helps database migration administration for modifying schemas or reverting modifications. It makes numerous provisions for that, similar to with the ability to create tables from structs.
- Relationships – You’ll be able to outline database relationships utilizing fashions or structs with annotations in tags and nested structs. One-to-one, many-to-many, and one-to-many relationships could be achieved.
- Transactions – GORM gives help for transactions, nested transactions, committing and rolling again transactions, and so forth.
- Database resolver – This lets you entry a number of database and schema sources in a single software. This additionally permits connection switching (computerized and guide) relying on the struct you’re accessing or database availability and cargo balancing.
- SQL builder – The supplied SQL builder helps numerous types of SQL and SQL clauses. These embrace however usually are not restricted to CRUD operations, subqueries, ready statements, named arguments, upserts, SQL expressions, joins, and so forth.
- Hooks – These are interfaces that may be carried out to undertake sure actions earlier than or after a DML assertion is executed.
It is a non-exhaustive listing of this package deal’s options; it has a variety of different options that guarantee it performs properly in virtually any use case.
Ease of Use
To maximise productiveness, it’s essential {that a} library is simple to make use of for each skilled builders and newbies. This part appears at how simple it’s to get began with every library, contemplating the out there documentation, tutorials (textual and video), and group help. This part additionally appears at code samples for a number of frequent operations and the training curve concerned.
The code samples for this part are supplied in examples
listing in this repository on GitHub. To run the code samples, you may hook up with a database in GoLand. You need to use MySQL or MariaDB for this. Just remember to have both of these put in.
To connect with a database, open the Database software window, click on on the plus icon, and select Add knowledge supply.
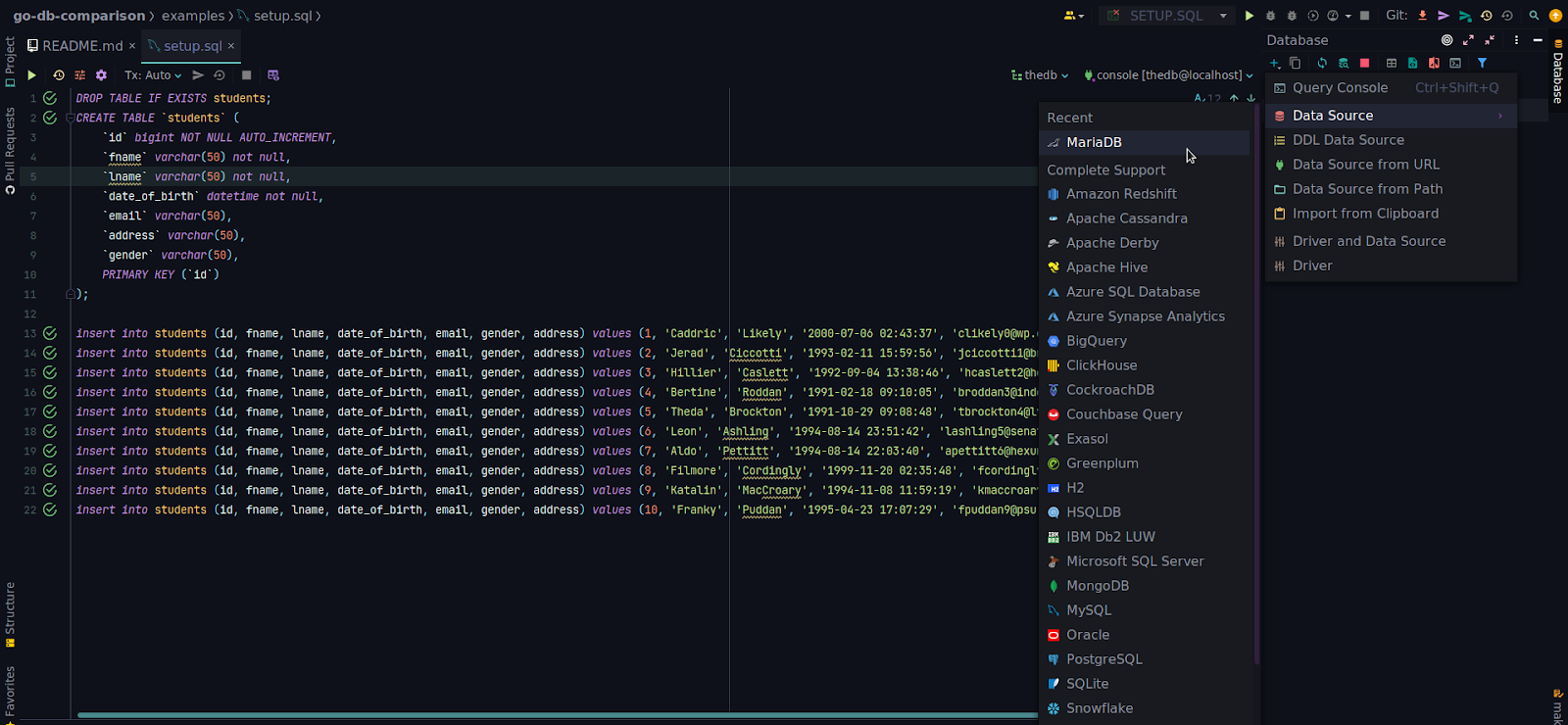
Present a reputation for this database connection you’re establishing and all the mandatory credentials on your setup. This instance makes use of localhost because the host, default MySQL/MariaDB port 3306, a consumer known as theuser, a hidden password of worth thepass, and a database named thedb. GoLand will counsel downloading the mandatory drivers in the event that they haven’t been downloaded earlier than. Lastly, click on on the Take a look at Connection hyperlink on the bottom-left of the display. This can validate the credentials by making an attempt to determine a connection to the desired database utilizing the consumer and password credentials you supplied.
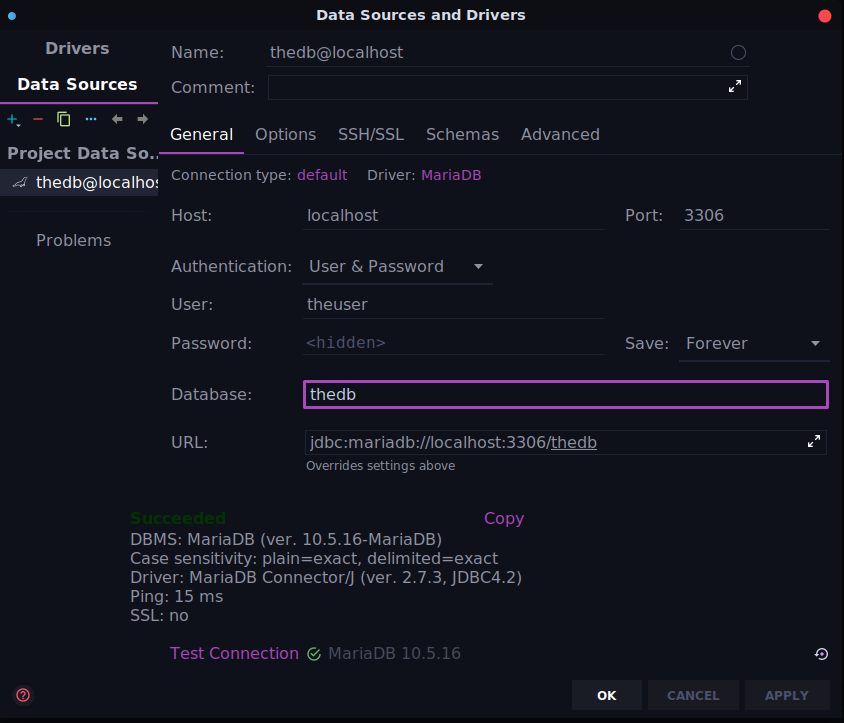
Hopefully, you might have linked to your database. Now you may run the setup.sql
script – simply open it and press on the inexperienced arrow on the prime left nook or press ⌘⏎ /Ctrl+Enter to execute statements.
If the whole lot went easily, you will notice your desk within the tables
listing. You’ll be able to click on on the college students desk twice to see the rows.
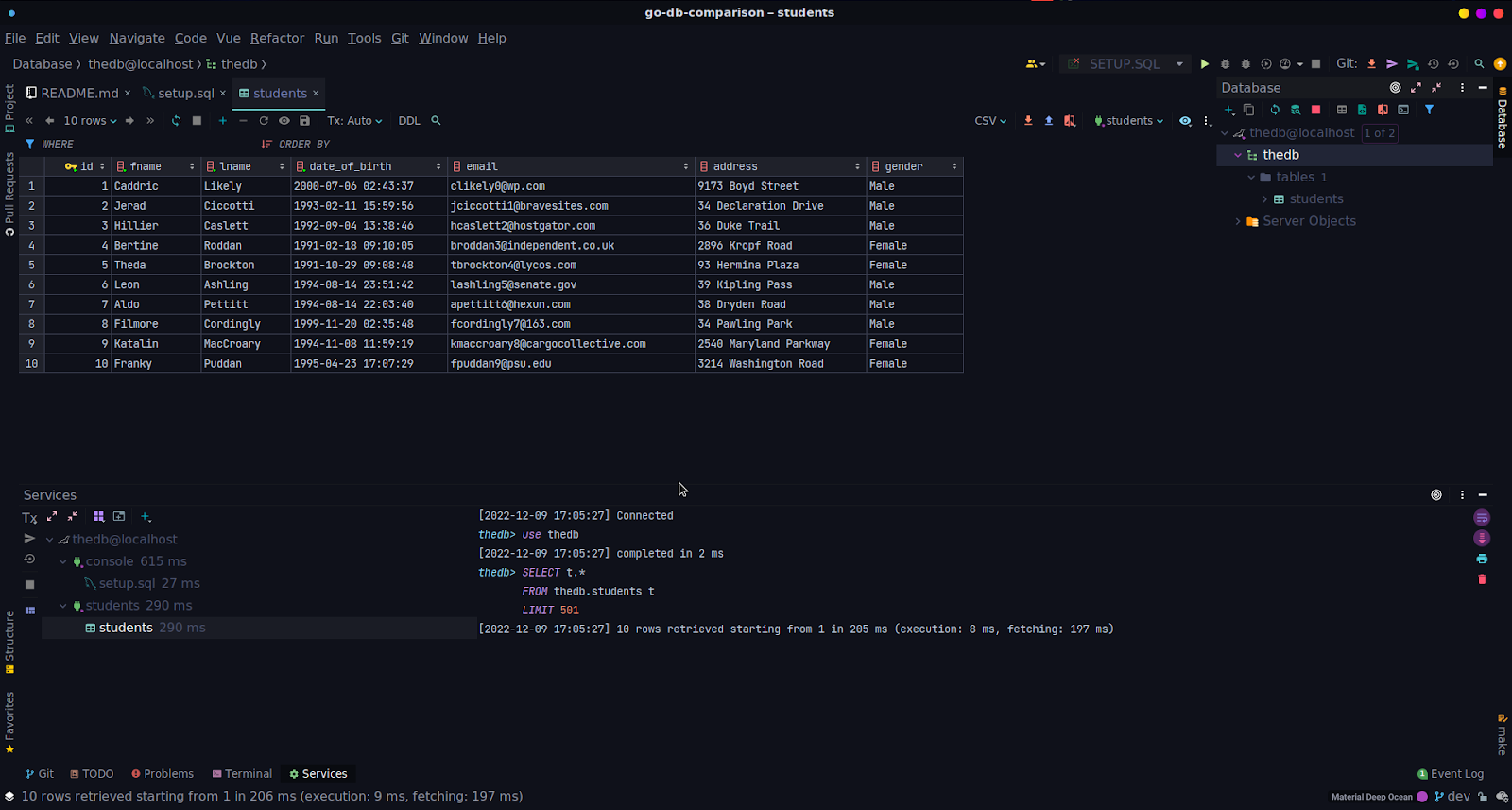
database/sql
For knowledgeable builders, database/sql has detailed, technically superior documentation. Moreover, there’s a nice tutorial for newcomers. The syntax is kind of easy, however you’ll have to examine for errors typically, which implies extra code. Because the go-to package deal in the usual library, it additionally has an enormous following and powerful help from your complete Go dev group.
In case you’re comfy writing your individual SQL queries, this package deal is an efficient choice, particularly for primary queries and primary sorts or structs with a small set of fields.
The next snippet demonstrates the right way to use the database/sql package deal with the MySQL driver to insert a file:
// Operate so as to add a file and return the file ID if profitable, if not then an error func addStudent(s Scholar) (int64, error){ // Insert assertion itemizing all of the fields and offering their respective values question := "insert into college students (fname, lname, date_of_birth, e mail, gender, tackle) values (?, ?, ?, ?, ?, ?);" consequence, err := db.Exec(question, s.Fname,s.Lname, s.DateOfBirth, s.E mail, s.Gender, s.Tackle) if err != nil { return 0, fmt.Errorf("addStudent Error: %v", err) } // Verify to get the ID of the inserted file id, err := consequence.LastInsertId() if err != nil { return 0, fmt.Errorf("addStudent Error: %v", err) } return id, nil }
As you may see, the insert operation is similar to writing direct SQL statements. You’ll additionally see that you should sort every discipline and its related worth individually. Sadly, sustaining the code in massive structs or advanced sorts turns into cumbersome over time and will increase the possibility of introducing errors, which can solely be caught throughout runtime.
The snippet under demonstrates the right way to retrieve a number of data utilizing the database/sql package deal:
// Operate to fetch a number of data func fetchStudents() ([]Scholar, error) { // A slice of College students to carry knowledge from returned rows var college students []Scholar rows, err := db.Question("SELECT * FROM college students") if err != nil { return nil, fmt.Errorf("fetchStudents %v", err) } defer rows.Shut() // Loop by way of rows, utilizing Scan to assign column knowledge to struct fields for rows.Subsequent() { var s Scholar if err := rows.Scan(&s.ID, &s.Fname, &s.Lname, &s.DateOfBirth, &s.E mail, &s.Tackle, &s.Gender ); err != nil { return nil, fmt.Errorf("fetchStudents %v", err) } college students = append(college students, s) } if err := rows.Err(); err != nil { return nil, fmt.Errorf("fetchStudents %v", err) } return college students, nil }
Within the snippet above, after fetching the data, you loop by way of them, use row.Scan()
to individually scan every discipline of every file right into a struct, then append the struct to a slice. You might want to watch out, because the variety of fields within the struct supplied should equal the variety of fields within the data returned. You also needs to notice that it’s troublesome to work with queries utilizing the IN clause, because it treats IN bindvar values as a single worth and never a number of values.
sqlx
sqlx additionally gives detailed technical documentation. Because it’s primarily an extension of the Go database/sql package deal, there’s additionally a complete information on utilizing this package deal with database/sql.
On GitHub, sqlx boasts over 12,800 stars, indicating a powerful following and a really lively subject listing.
In case you’re coming from a database/sql background, you’ll have a easy transition, as queries are related in syntax and appropriate. From the snippets under, you’ll discover various similarities with the database/sql code seen earlier, particularly the insert assertion:
// Add inserting pupil file utilizing the sqlx package deal func addStudent(s Scholar) (int64, error){ question := "insert into college students (fname, lname, date_of_birth, e mail, gender, tackle) values (?, ?, ?, ?, ?, ?);" consequence := db.MustExec(question, s.Fname,s.Lname, s.DateOfBirth, s.E mail, s.Gender, s.Tackle) id, err := consequence.LastInsertId() if err != nil { return 0, fmt.Errorf("addStudent Error: %v", err) } return id, nil }
There are fewer strains of code than with database/sql, because of the decreased want for error checking utilizing the db.MustExec()
technique.
The code under reveals the right way to retrieve a number of data utilizing the sqlx comfort technique:
// Operate to fetch a number of data func fetchStudents() ([]Scholar, error) { // A slice of College students to carry knowledge from returned rows var college students []Scholar err := db.Choose(&college students,"SELECT * FROM college students LIMIT 10") if err != nil { return nil, fmt.Errorf("fetchStudents %v", err) } return college students, nil }
As you may see, in comparison with utilizing db.Question()
to jot down syntax appropriate with database/sql and looping by way of the outcomes, sqlx gives a lot less complicated and cleaner code to attain the identical purpose utilizing db.Choose()
. It even gives a greater means of dealing with queries with the IN
clause, as defined within the Options part above.
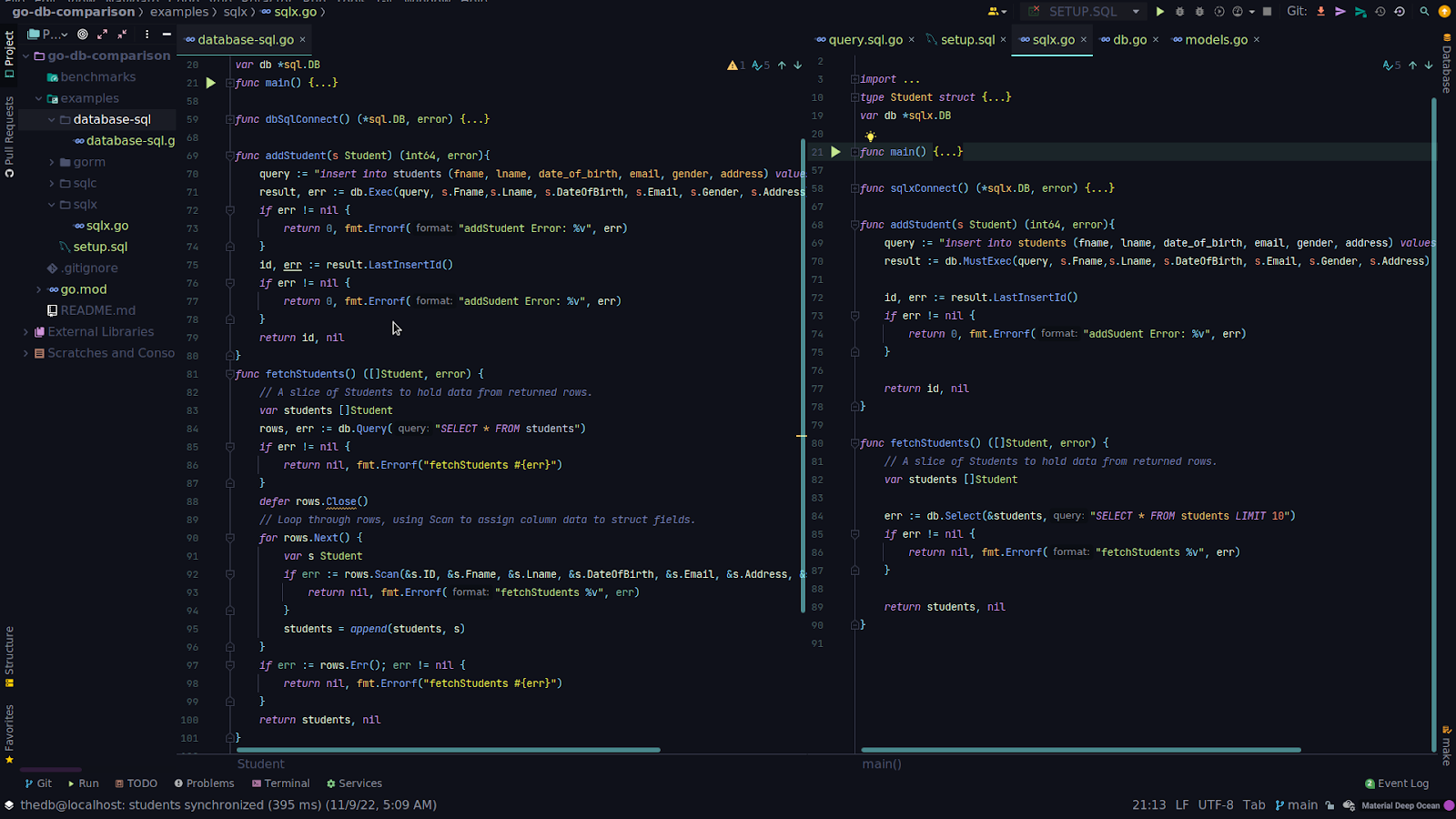
sqlc
sqlc’s beforehand talked about means to generate type-safe code is one other profit for ease of use, because it reduces the quantity of Go code you should write on your database operations, saving you effort and time.
With an lively and rising group of over 6,800 stars, sqlc additionally boasts sturdy engagement and group help.
The documentation gives a step-by-step walkthrough from set up to the era of code, and there are additionally concise video tutorials should you’d like a extra interactive method. So, getting began with sqlc couldn’t be simpler, as you’ll see for your self under.
After putting in the binary, write your config file in sqlc.yaml, which ought to seem like the snippet under:
model: 1 packages: - path: "./" title: "major" engine: "mysql" schema: "schema.sql" queries: "question.sql"
Now all you should do is write your plain outdated SQL in 2 recordsdata, simply as within the picture under, and run the command sqlc generate
in your working listing:
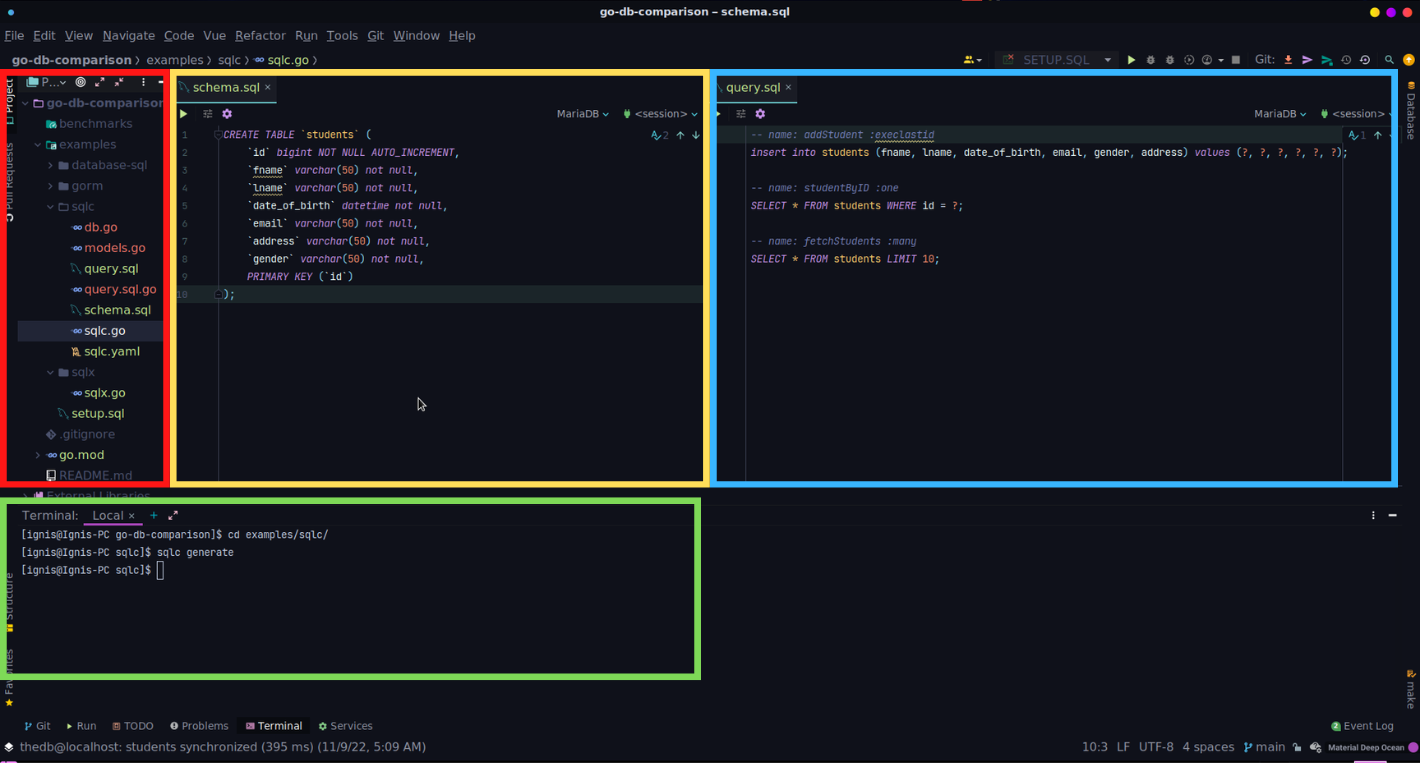
The next listing explains the weather of the above picture:
- The crimson field reveals 3 new generated recordsdata:
fashions.go
for structs,question.sql.go
, anddb.go
for different database-related code. - The yellow field highlights the
schema.sql
file containing your schema definition. - The blue field highlights the
question.sql
file, which accommodates all of the SQL statements on your software’s database operations together with some metadata for producing the capabilities. - The inexperienced field highlights the run that sqlc generated within the working listing within the terminal.
Now, you solely want to supply a database connection utilizing the database/sql package deal, then name your required strategies with out modifying the generated code, as you may see within the following snippet:
func major() { // Create a brand new database connection conn, err := sql.Open("mysql", "theuser:thepass@tcp(localhost:3306)/thedb?parseTime=true") if err != nil { log.Deadly(err) } fmt.Println("Related!") db := New(conn) // Initialize file to be inserted newSt := addStudentParams{ Fname: "Leon", Lname: "Ashling", DateOfBirth: time.Date(1994, time.August, 14, 23, 51, 42, 0, time.UTC), E mail: "lashling5@senate.gov", Gender: "Male", Tackle: "39 Kipling Cross", } // Insert the file sID, err := db.addStudent(context.Background(), newSt) if err != nil { log.Deadly(err) } fmt.Printf("addStudent id: %v n", sID) // Fetch the data college students, err := db.fetchStudents(context.Background()) if err != nil { log.Println(err) } fmt.Printf("fetchStudents rely: %v n", len(college students)) }
Offered you might be good at writing your SQL statements, this package deal’s era of type-safe code makes it a greater choice, particularly if you must work together with many schemas of various sorts.
GORM
GORM has very complete but easy documentation and guides on getting began. Sadly, GORM takes extra of a code-based method to interacting with the database, which means there’s a steep studying curve at first.
Utilizing the GORM syntax, you may spend a whole lot of time initially setting up code much like your uncooked SQL queries. Nonetheless, when you’re accustomed to the syntax, you’ll have a clear codebase with uncommon interplay with uncooked SQL.
GORM is the second greatest Go database package deal (after database/sql) on GitHub, with over 30,400 stars, which implies there needs to be no scarcity of group help.
The next instance demonstrates how GORM can be used to implement the identical insert assertion, and a number of data question as earlier than:
func major() { // Open a database connection db, err := gorm.Open(mysql.Open("theuser:thepass@tcp(127.0.0.1:3306)/thedb?charset=utf8mb4&parseTime=True&loc=Native")) if err != nil { log.Deadly(err) } fmt.Println("Related!") // Initialize file to be inserted s := Scholar{ Fname: "Leon", Lname: "Ashling", DateOfBirth: time.Date(1994, time.August, 14, 23, 51, 42, 0, time.UTC), E mail: "lashling5@senate.gov", Tackle: "39 Kipling Cross", Gender: "Male", } // Add pupil file and return the ID into the ID discipline db.Create(&s) fmt.Printf("addStudent id: %v n", s.ID) // Choose a number of data var college students []Scholar db.Restrict(10).Discover(&college students) fmt.Printf("fetchStudents rely: %v n", len(college students)) }
As you may see within the above snippet, the code is comparatively easy, with only a few strains. When you get previous the steep preliminary studying curve, GORM is definitely extra environment friendly for writing queries than the opposite 3 choices.
Efficiency and Pace
The efficiency and velocity of database operations are essential to the general efficiency of data-centric purposes. Essentially the most appropriate database package deal is very depending on efficiency, particularly should you’re creating a low-latency software.
This part compares the efficiency of all 4 database packages. To undertake this benchmark, a MySQL/MariaDB database was arrange with 15,000 pupil data. Since most use instances for these packages are queries for fetching data, the benchmark captured the efficiency of all 4 packages fetching 1, 10, 100, 1000, 10,000, and 15,000 data and scanning them into structs:
================================== BENCHMARKING 1 RECORDS ====================================== goos: linux goarch: amd64 pkg: github.com/rexfordnyrk/go-db-comparison/benchmarks cpu: Intel(R) Core(TM) i7-8550U CPU @ 1.80GHz Benchmark/database/sql_limit:1_-8 11128 105370 ns/op Benchmark/sqlx_limit:1_-8 10000 111365 ns/op Benchmark/sqlc_limit:1_-8 10000 108700 ns/op Benchmark/gorm_limit:1_-8 16742 70535 ns/op ================================================================================================ ================================== BENCHMARKING 10 RECORDS ====================================== Benchmark/database/sql_limit:10_-8 9727 108236 ns/op Benchmark/sqlx_limit:10_-8 9043 116293 ns/op Benchmark/sqlc_limit:10_-8 10000 113071 ns/op Benchmark/gorm_limit:10_-8 16965 75148 ns/op ================================================================================================ ================================== BENCHMARKING 100 RECORDS ====================================== Benchmark/database/sql_limit:100_-8 10000 107338 ns/op Benchmark/sqlx_limit:100_-8 10000 114617 ns/op Benchmark/sqlc_limit:100_-8 10000 114440 ns/op Benchmark/gorm_limit:100_-8 16810 71593 ns/op ================================================================================================ ================================== BENCHMARKING 1000 RECORDS ====================================== Benchmark/database/sql_limit:1000_-8 10000 111443 ns/op Benchmark/sqlx_limit:1000_-8 9792 111644 ns/op Benchmark/sqlc_limit:1000_-8 10000 114245 ns/op Benchmark/gorm_limit:1000_-8 16417 71042 ns/op ================================================================================================ ================================== BENCHMARKING 10000 RECORDS ====================================== Benchmark/database/sql_limit:10000_-8 10000 106227 ns/op Benchmark/sqlx_limit:10000_-8 9711 111548 ns/op Benchmark/sqlc_limit:10000_-8 10000 113653 ns/op Benchmark/gorm_limit:10000_-8 16928 74733 ns/op ================================================================================================ ================================== BENCHMARKING 15000 RECORDS ====================================== Benchmark/database/sql_limit:15000_-8 11377 108174 ns/op Benchmark/sqlx_limit:15000_-8 10000 110007 ns/op Benchmark/sqlc_limit:15000_-8 9697 107933 ns/op Benchmark/gorm_limit:15000_-8 16348 73725 ns/op =================================================================================================== PASS okay github.com/rexfordnyrk/go-db-comparison/benchmarks 33.163s
For the needs of uniformity and equity, the benchmark was run on the identical {hardware}, and the check ensured the same code construction by putting every motion in a separate operate and measuring their efficiency individually. This benchmark could be replicated utilizing the benchmarks
code listing of this repo on GitHub.
Every consequence set consists of three columns. The primary is the title of the benchmark technique that was run, the second is the variety of occasions the benchmark was run till a dependable time was generated, and the third represents the time (in nanoseconds) it took every time the benchmark was executed.
GORM carried out considerably higher in all of the checks, taking about 71,000 to 75,000 nanoseconds throughout the 6 benchmarks. database/sql follows, hovering largely underneath 110,000 nanoseconds. Lastly, sqlc and sqlx take turns trailing, with related figures, largely round 111,000 nanoseconds.
Conclusion
Whereas database/sql is the default Go package deal, whether or not it is best to use it or not is determined by your wants as a developer. On this article, you might have examine some great benefits of every package deal.
GORM is one of the best package deal should you want superior querying, full help capabilities from underlying database applied sciences, and a clear codebase with out having to fret about efficiency.
In case you solely want primary queries and are comfy writing your individual SQL, then the database/sql or sqlx packages will do exactly high quality.
Lastly, sqlc is greatest fitted to backend builders who work extensively with databases and who want to jot down many queries in Go code underneath tight deadlines. It lets you write your uncooked SQL queries and generate the code wanted for these queries with out having to fret about sorts, scanning, or anything that will get in the way in which of productiveness.
Subscribe to Weblog updates