Within the very early days of contemporary Angular, you would import a Element after which present it as a declaration for use inside one other Element. Then, Angular converted to utilizing NgModule
, which turned the de facto packaging and configuration container for the final 5-or-6 years. Now, in an effort to supply a extra streamlined developer expertise, Angular is as soon as once more permitting Elements to be consumed with out an middleman NgMogule
container. This new-old characteristic is named “Standalone Elements”, or “Non-obligatory Modules”. I have not written an excessive amount of Angular currently (been centered closely on Lucee CFML); so, I assumed this could be a very good probability to mud off my Angular expertise.
View this code in my Plate Weight Calculator undertaking on GitHub.
This submit is not trying to be a tutorial on utilizing Standalone Elements in Angular. The Angular docs have already got a good tutorial that it’s best to try if you’d like extra element. This submit is simply me having enjoyable and attempting to make use of this new characteristic to construct one thing with real-world worth.
As luck would have it, I used to be simply experiencing some real-world friction that can be utilized as a test-bed for this very characteristic: determining the overall weight that’s on a bit fitness center gear. To calculate the overall weight, I would wish so as to add up the varied plates (45 lbs, 25 lbs, 10 lbs, and so on) loaded on the machine; after which, add any base-weight for the machine itself.
So, for instance, an Olympic barbell with two 45-lb plates can be:
45 (base bar weight) + ( 2 * 45 ) = 135-lbs
The great factor about that is that the idea is comparatively easy; however, it is simply advanced sufficient to require a variety of totally different Angular options which might be touched by the brand new standalone elements / optionally available module adjustments:
- Bootstrapping a root part.
- Consuming a nested part.
- Injecting a service.
Earlier than we have a look at the code, let us take a look at a GIF to get a way of what the UI appears like and the way it operates. Within the following UI, I am offering a collection of “Add” and “Take away” controls for a given plate weight. As every plate is added to or faraway from the calculator, I am updating the overall weight and syncing the state of the UI to the URL:
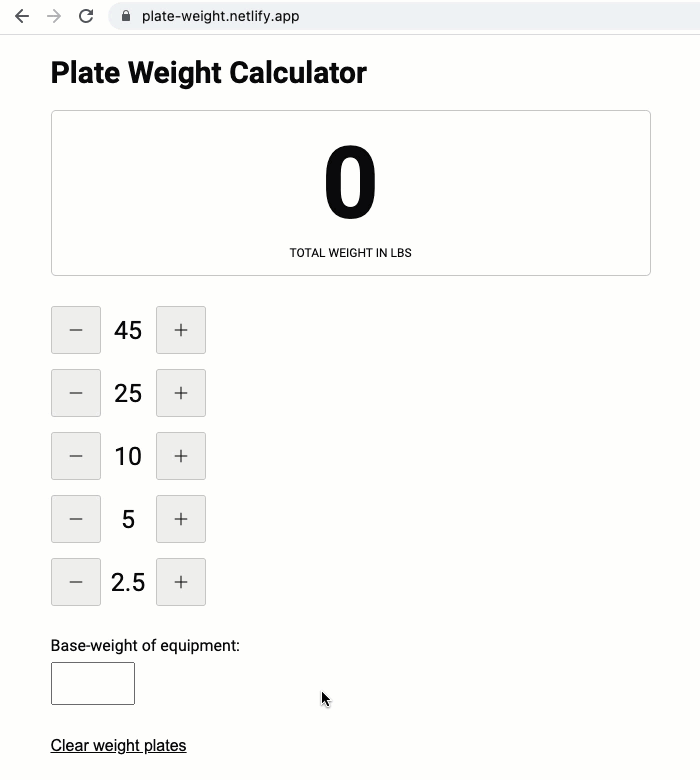
The majority of the UI is outlined within the AppComponent
. The black circles that present up subsequent to every management is the MeterComponent
. And, the service that syncs the state of the plate weight calculator to the URL is the UrlStateSevice
.
First, let us take a look at bootstrapping this software within the foremost.ts
. In contrast to a conventional bootstrapping course of that makes use of a module, right here I’m merely utilizing the basis part:
// Import core Angular modules.
import { bootstrapApplication } from "@angular/platform-browser";
import { enableProdMode } from "@angular/core";
// Import software modules.
import { AppComponent } from "./app/app.part";
import { surroundings } from "./environments/surroundings";
// ----------------------------------------------------------------------------------- //
// ----------------------------------------------------------------------------------- //
// NOTE: The module decision for this file adjustments relying on the construct. See the
// "fileReplacements" possibility within the angular.json configuration file.
if ( surroundings.manufacturing ) {
enableProdMode();
}
bootstrapApplication( AppComponent ).catch(
( error ) => {
console.warn( "There was an issue bootstrapping the Angular software." );
console.error( error );
}
);
On this case, I am simply calling bootstrapApplication( AppComponent )
with a single argument – my root part; however, this operate invocation can take a second argument which configures the applying suppliers. On this demo, since I haven’t got any routes or dependent modules, there’s nothing else to do.
My AppComponent
holds all the conventional UI and state administration that you’d discover in any run-of-the-mill Angular part. Solely, along with the conventional @Element()
decorator info, I’ll additionally outline:
The standalone
flag tells Angular that this can be a standalone / module-free part. And, the imports
assortment tells Angular which elements, directives, and pipes can be utilized as selectors inside the present part template. This contains the core Angular directives. And, since we’ve got no root module (on this demo) offering world declarations, my AppComponent
is accountable for explicitly import
‘ing the CommonModule
and FormsModule
in order that I can use primary directives like *ngIf
, *ngFor
, and [(ngModel)]
inside the AppComponent
template.
This is the total code for AppComponent
; although, the one elements actually related to the standalone-component idea are within the first 50-lines. Be aware that my imports
assortment accommodates each core and nested elements:
// Import core Angular modules.
import { CommonModule } from "@angular/frequent";
import { Element } from "@angular/core";
import { FormsModule } from "@angular/kinds";
// Import software modules.
import { MeterComponent } from "./meter.part";
import { UrlStateService } from "./url-state.service";
// ----------------------------------------------------------------------------------- //
// ----------------------------------------------------------------------------------- //
interface Section {
title: string;
weight: quantity;
rely: quantity;
}
@Element({
standalone: true,
selector: "app-root",
// NOTE: As a result of I'm not utilizing a root module, there's nothing that inherently tells
// Angular that I anticipate the core Angular directives to be accessible within the templates.
// As such, I've to explicitly embrace the CommonModule, which gives directives
// like `ngIf` and `ngForOf`, on this part's imports.
imports: [
CommonModule,
FormsModule,
// OH SNAP, I'm pulling in local, application components as well!
MeterComponent
],
templateUrl: "./app.part.html",
styleUrls: [ "./app.component.less" ]
})
export class AppComponent {
non-public urlStateService: UrlStateService;
public baseWeight: quantity;
public kind: {
baseWeight: string;
};
public segments: Section[];
public whole: quantity;
// I initialize the basis part.
constructor( urlStateService: UrlStateService ) {
this.urlStateService = urlStateService;
this.baseWeight = 0;
this.segments = [
this.buildSegment( 45 ),
this.buildSegment( 25 ),
this.buildSegment( 10 ),
this.buildSegment( 5 ),
this.buildSegment( 2.5 )
];
this.whole = 0;
this.kind = {
baseWeight: ""
};
}
// ---
// PUBLIC METHODS.
// ---
/**
* I add a single plate to the given phase.
*/
public addPlate( phase: Section ) : void {
phase.rely++;
this.setTotal();
this.statePushToUrl();
}
/**
* I apply the present base-weight kind worth to the general weight whole.
*/
public applyBaseWeight() : void 0 );
this.setTotal();
this.statePushToUrl();
/**
* I take away all the plates from all the segments.
*/
public clearPlates() : void {
for ( var phase of this.segments ) {
phase.rely = 0;
}
this.setTotal();
this.statePushToUrl();
}
/**
* I get known as as soon as after the part inputs have been sure for the primary time.
*/
public ngOnInit() : void {
this.statePullFromUrl();
}
/**
* I take away a single plate from the given phase.
*/
public removePlate( phase: Section ) : void {
if ( phase.rely ) {
phase.count--;
this.setTotal();
this.statePushToUrl();
}
}
// ---
// PRIVATE METHODS.
// ---
/**
* I create an empty phase for the given weight-class.
*/
non-public buildSegment( weight: quantity ) : Section {
return({
title: String( weight ),
weight: weight,
rely: 0
});
}
/**
* I calculate and retailer the overall primarily based on the present view-model.
*/
non-public setTotal() : void {
this.whole = this.segments.scale back(
( discount, phase ) => {
return( discount + ( phase.weight * phase.rely ) );
},
this.baseWeight
);
}
/**
* I pull state from the URL (fragment, hash), and use it to replace the view-model.
* This permits the present weight plate affirmation to be shareable with others.
*/
non-public statePullFromUrl() : void
/**
* I persist the present plate configuration to the URL fragment in order that the present
* state might be shared.
*/
non-public statePushToUrl() : void {
this.urlStateService.set( this.baseWeight, this.segments );
}
}
As you’ll be able to see, the AppComponent
is importing the MeterComponent
. That is one other tiny standalone part that interprets a [value]
enter binding right into a collection of iterative discs (meant as an example the plates on the fitness center gear). That is starting to look extra like React, the place you’ll be able to import a part after which eat it inside your JSX. In fact, Angular then layers on highly effective constructs like Dependency-Injection, so it isn’t an apples-to-apples comparability. However, it appears like we’re beginning to get the most effective of each worlds on this Angular 14 replace!
Within the following code for MeterComponent
, discover that this it additionally has to import the CommonModule
as a way to use core Angular directives, like *ngForOf
, even supposing the AppComponent
can also be importing mentioned module. That is the entire level of the standalone-component idea: you explicitly pull in what you want; the part turns into the organizational unit.
// Import core Angular modules.
import { ChangeDetectionStrategy } from "@angular/core";
import { CommonModule } from "@angular/frequent";
import { Element } from "@angular/core";
// ----------------------------------------------------------------------------------- //
// ----------------------------------------------------------------------------------- //
@Element({
standalone: true,
selector: "app-meter",
inputs: [ "value" ],
changeDetection: ChangeDetectionStrategy.OnPush,
imports: [ CommonModule ],
templateUrl: "./meter.part.html",
styleUrls: [ "./meter.component.less" ]
})
export class MeterComponent {
public readings: quantity[];
public worth!: quantity;
// I initialize the meter part.
constructor() {
this.readings = [];
}
// ---
// PUBLIC METHODS.
// ---
/**
* I get known as when the inputs bindings are first sure or up to date.
*/
public ngOnChanges() : void {
this.setReadings();
}
// ---
// PRIVATE METHODS.
// ---
/**
* I populate the readings primarily based on the present view-model.
*/
non-public setReadings() : void {
this.readings = [];
for ( var i = 1 ; i <= this.worth ; i++ ) {
this.readings.push( i );
}
}
}
My AppComponent
additionally injects a UrlStateService
. This service is basically untouched by any standalone-component adjustments. I’m nonetheless adorning it with @Injectable()
; and, it’s nonetheless being offered within the root injector (now, apparently, being known as an “Atmosphere Injector” – see documentation).
// Import core Angular modules.
import { Injectable } from "@angular/core";
// ----------------------------------------------------------------------------------- //
// ----------------------------------------------------------------------------------- //
export interface State {
[ key: string ]: quantity;
}
export interface Section {
weight: quantity;
rely: quantity
}
@Injectable({
providedIn: "root"
})
export class UrlStateService {
/**
* I break up the placement fragment right into a dictionary of key:worth pairs by which the
* values are all numbers.
*/
public get() : State {
var state = Object.create( null );
for ( var setting of window.location.hash.slice( 1 ).break up( ";" ) ) 0 );
state[ name ] = worth;
return( state );
}
/**
* I retailer the given weights into the placement fragment.
*/
public set(
baseWeight: quantity,
segments: Section[]
) : void {
window.location.hash = segments.scale back(
( discount, phase ) => {
return( `${ discount };${ phase.weight }:${ phase.rely }` );
},
`baseWeight:${ baseWeight }`
);
}
}
For the sake of simplicity, I’m foregoing using the Router module and I am simply consuming the native Location
API hash
to retailer the state.
The part templates aren’t affected in any respect by the standalone part updates in Angular 14. That is the gorgeous factor about utilizing a selector primarily based template mannequin: the templates do not care the place the elements and directives are coming from so long as there’s something offering them.
That mentioned, I will present the part templates for completeness. This is the one of many AppComponent
. You may see that it makes use of <app-meter>
, which is the selector for the imported MeterComponent
:
<h1 class="title">
Plate Weight Calculator
</h1>
<determine class="whole">
<div class="total__value">
{{ whole }}
</div>
<figcaption class="total__label">
Complete Weight in LBS
</figcaption>
</determine>
<ul class="segments">
<li *ngFor="let phase of segments" class="segments__segment phase">
<div class="segment__controls controls">
<button
aria-describedby="add-plate-icon"
(click on)="removePlate( phase )"
class="controls__button">
<svg position="img" class="controls__icon">
<title id="add-plate-icon">
Take away {{ phase.weight }}-pound Plate
</title>
<use xlink:href="#icon-minus"></use>
</svg>
</button>
<span aria-hidden="true" class="controls__label">
{{ phase.weight }}
</span>
<button
aria-describedby="remove-plate-icon"
(click on)="addPlate( phase )"
class="controls__button">
<svg position="img" class="controls__icon">
<title id="remove-plate-icon">
Add {{ phase.weight }}-pound Plate
</title>
<use xlink:href="#icon-plus"></use>
</svg>
</button>
</div>
<app-meter
[value]="phase.rely"
class="segment__meter">
</app-meter>
</li>
</ul>
<div class="base-weight">
<label for="base-weight-input" class="base-weight__label">
Base-weight of apparatus:
</label>
<enter
id="base-weight-input"
sort="quantity"
[(ngModel)]="kind.baseWeight"
(enter)="applyBaseWeight()"
class="base-weight__input"
/>
</div>
<button (click on)="clearPlates()" class="clear">
Clear weight plates
</button>
And, this is the tiny template for the MeterComponent
:
<span *ngFor="let studying of readings" class="studying">
{{ studying }}
</span>
From what I can see, Standalone elements / optionally available modules is not essentially altering the best way Angular works. It is actually simply altering the developer ergonomics of structuring your software. Finally, you continue to have to inform Angular about all the constructing blocks that go right into a part. It is simply that in lots of circumstances, we’ll be capable to colocate that configuration info with the elements as an alternative of getting to create separate, considerably superfluous modules.
Wish to use code from this submit?
Take a look at the license.