On this article we are going to see the right way to Encrypt and Decrypt a string with a Secret Key in C#
we’re going to use Symmetric encryption to encrypt and decrypt a string, Symmetric encryption is a sort of encryption the place just one key (a secret key) is used to each encrypt and decrypt data. The entities speaking through symmetric encryption should change the key in order that it may be used within the decryption course of.
see beneath picture which exhibits how ecryption and decryption work with a key
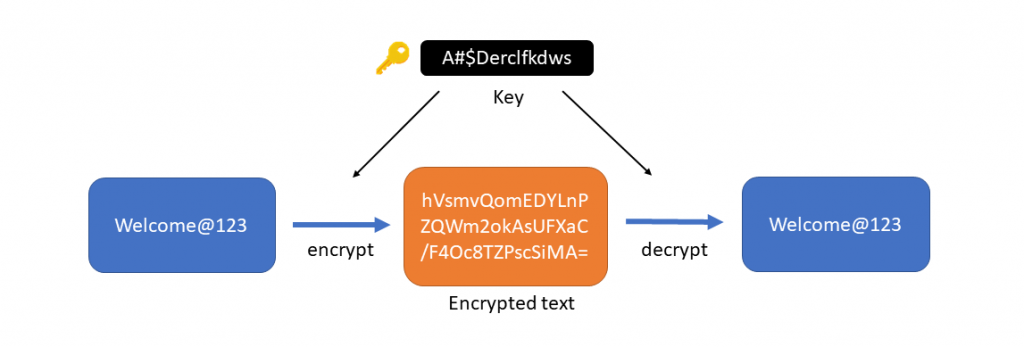
as you see “Welcome@123” is a plain textual content that’s encrypted utilizing a secret key (A#$Derclfkdws
) and decrypted with the identical key returned output “Welcome@123”.
let’s transfer to the code half, I’ve created a easy console utility that accommodates the “static void Primary()
” perform and added two strategies encrypt
and decrypt
in the identical class (Program
) the place “Primary()
” exists so I can entry each strategies straight.
beneath namespaces shall be require for encrypt/decrypt
Encryption/Decryption Instance
utilizing System.IO;
utilizing System.Safety.Cryptography;
utilizing System.Textual content;
public static string Encrypt(string clearText)
{
string EncryptionKey = "A#$Derclfkdws"; // SecurityKey
byte[] clearBytes = Encoding.Unicode.GetBytes(clearText);
utilizing(Aes encryptor = Aes.Create()) {
Rfc2898DeriveBytes pdb = new Rfc2898DeriveBytes(EncryptionKey, new byte[] {
0x49,0x76,0x61,0x6e,0x20,0x4d,0x65,0x64,0x76,0x65,0x64,0x65,0x76
});
encryptor.Key = pdb.GetBytes(32);
encryptor.IV = pdb.GetBytes(16);
utilizing(MemoryStream ms = new MemoryStream()) {
utilizing(CryptoStream cs = new CryptoStream(ms, encryptor.CreateEncryptor(), CryptoStreamMode.Write)) {
cs.Write(clearBytes, 0, clearBytes.Size);
cs.Shut();
}
clearText = Convert.ToBase64String(ms.ToArray());
}
}
return clearText;
}
public static string Decrypt(string cipherText)
{
strive
{
string EncryptionKey = "A#$Derclfkdws"; // SecurityKey
cipherText = cipherText.Exchange(" ", "+");
byte[] cipherBytes = Convert.FromBase64String(cipherText);
utilizing(Aes encryptor = Aes.Create()) {
Rfc2898DeriveBytes pdb = new Rfc2898DeriveBytes(EncryptionKey, new byte[] {
0x49,0x76,0x61,0x6e,0x20,0x4d,0x65,0x64,
0x76,0x65,0x64,0x65,0x76
});
encryptor.Key = pdb.GetBytes(32);
encryptor.IV = pdb.GetBytes(16);
utilizing(MemoryStream ms = new MemoryStream()) {
utilizing(CryptoStream cs = new CryptoStream(ms, encryptor.CreateDecryptor(), CryptoStreamMode.Write)) {
cs.Write(cipherBytes, 0, cipherBytes.Size);
cs.Shut();
}
cipherText = Encoding.Unicode.GetString(ms.ToArray());
}
}
return cipherText;
} catch (Exception ex) {
return "";
}
}
static void Primary(string[] args)
{
bool a = true;
whereas (a) {
Console.Write("Enter textual content :");
var textual content = Console.ReadLine();
var encryption = Encrypt(textual content);
Console.WriteLine("your encrypted string is :" + encryption);
Console.Write("enter encrypted textual content to decrypt: ");
var userInput = Console.ReadLine();
var plaintext = Decrypt(userInput);
Console.WriteLine("Your decrypted string is :" + plaintext);
Console.ReadLine();
}
}
Output :
Enter Textual content : Welcome@123
Your encrypted string is : hVsmvQomEDYLnPZQWm2okAsUFXaC/F4Oc8TZPscSiMA=
Enter encrypted textual content to decrypt : hVsmvQomEDYLnPZQWm2okAsUFXaC/F4Oc8TZPscSiMA=
Your decrypted string is : Welcome@123