This text continues on the Solidity Good Contract Examples sequence, which implements a easy, however the helpful means of protected distant buy.
Right here, we’re strolling via an instance of a blind public sale (docs).
- We’ll first lay out the complete good contract instance with out the feedback for readability and improvement functions.
- Then we’ll dissect it half by half, analyze it and clarify it.
- Following this path, we’ll get a hands-on expertise with good contracts, in addition to good practices in coding, understanding, and debugging good contracts.
Good Contract – Protected Distant Buy
// SPDX-License-Identifier: GPL-3.0 pragma solidity ^0.8.4; contract Buy { uint public worth; handle payable public vendor; handle payable public purchaser; enum State { Created, Locked, Launch, Inactive } State public state; modifier situation(bool condition_) { require(condition_); _; } error OnlyBuyer(); error OnlySeller(); error InvalidState(); error ValueNotEven(); modifier onlyBuyer() { if (msg.sender != purchaser) revert OnlyBuyer(); _; } modifier onlySeller() { if (msg.sender != vendor) revert OnlySeller(); _; } modifier inState(State state_) { if (state != state_) revert InvalidState(); _; } occasion Aborted(); occasion PurchaseConfirmed(); occasion ItemReceived(); occasion SellerRefunded(); constructor() payable { vendor = payable(msg.sender); worth = msg.worth / 2; if ((2 * worth) != msg.worth) revert ValueNotEven(); } perform abort() exterior onlySeller inState(State.Created) { emit Aborted(); state = State.Inactive; vendor.switch(handle(this).stability); } perform confirmPurchase() exterior inState(State.Created) situation(msg.worth == (2 * worth)) payable { emit PurchaseConfirmed(); purchaser = payable(msg.sender); state = State.Locked; } perform confirmReceived() exterior onlyBuyer inState(State.Locked) { emit ItemReceived(); state = State.Launch; purchaser.switch(worth); } perform refundSeller() exterior onlySeller inState(State.Launch) { emit SellerRefunded(); state = State.Inactive; vendor.switch(3 * worth); } }
Code breakdown and evaluation
// SPDX-License-Identifier: GPL-3.0 pragma solidity ^0.8.4; contract Buy {
The state variables for recording the worth, vendor, and purchaser addresses.
uint public worth; handle payable public vendor; handle payable public purchaser;
For the first time, we’re introducing the enum
information construction that symbolically defines the 4 potential states of our contract. The states are internally listed from 0
to enum_length - 1
.
enum State { Created, Locked, Launch, Inactive }
The variable state retains monitor of the present state. Our contract begins by default within the created state and might transition to the Locked, Launch, and Inactive state.
State public state;
The situation
modifier guards a perform towards executing with out beforehand satisfying the situation, i.e. an expression given alongside the perform definition.
modifier situation(bool condition_) { require(condition_); _; }
The error definitions are used with the suitable, equally-named modifiers.
error OnlyBuyer(); error OnlySeller(); error InvalidState(); error ValueNotEven();
The onlyBuyer
modifier guards a perform towards executing when the perform caller is just not the customer.
modifier onlyBuyer() { if (msg.sender != purchaser) revert OnlyBuyer(); _; }
The onlySeller
modifier guards a perform towards executing when the perform caller differs from the vendor.
modifier onlySeller() { if (msg.sender != vendor) revert OnlySeller(); _; }
The inState
modifier guards a perform towards executing when the contract state differs from the required state_
.
modifier inState(State state_) { if (state != state_) revert InvalidState(); _; }
The occasions that the contract emits to acknowledge the capabilities abort()
, confirmPurchase()
, confirmReceived()
, and refundSeller()
had been executed.
occasion Aborted(); occasion PurchaseConfirmed(); occasion ItemReceived(); occasion SellerRefunded();
The constructor is said as payable
, that means that the contract deployment (synonyms creation, instantiation) requires sending a price (msg.worth
) with the contract-creating transaction.
constructor() payable {
The vendor
state variable is about to msg.sender
handle, forged (transformed) to payable.
vendor = payable(msg.sender);
The worth state variable is about to half the msg.worth
, as a result of each the vendor and the customer should put twice the worth of the merchandise being offered/purchased into the contract as an escrow settlement.
💡 Information: “Escrow is a authorized association through which a 3rd celebration quickly holds cash or property till a selected situation has been met (such because the success of a purchase order settlement).” (supply)
In our case, our escrow is our good contract.
worth = msg.worth / 2;
If the worth is just not equally divided, i.e. the msg.worth
is just not an excellent quantity, the perform will terminate. For the reason that vendor will all the time
if ((2 * worth) != msg.worth) revert ValueNotEven(); }
Aborting the distant protected buy is allowed solely within the Created
state and solely by the vendor.
The exterior
key phrase makes the perform callable solely by different accounts / good contracts. From the enterprise perspective, solely the vendor can name the abort()
perform and solely earlier than the customer decides to buy, i.e. earlier than the contract enters the Locked
state.
perform abort() exterior onlySeller inState(State.Created) {
Emits the Aborted
occasion, the contract state transitions to inactive, and the stability is transferred to the vendor.
emit Aborted(); state = State.Inactive;
💡 Observe: “Previous to model 0.5.0, Solidity allowed handle members to be accessed by a contract occasion, for instance, this.stability. That is now forbidden and an specific conversion to handle have to be executed: handle(this).stability.” (docs).
In different phrases, this key phrase lets us entry the contract’s inherited members.
Each contract inherits its members from the handle kind and might entry these members by way of handle(this).<a member>
(docs).
vendor.switch(handle(this).stability); }
The confirmPurchase()
perform is obtainable for execution solely within the Created
state.
It enforces the rule {that a} msg.worth
have to be twice the worth of the acquisition.
The confirmPurchase()
perform can be declared as payable
, that means the caller, i.e. the customer has to ship the foreign money (msg.worth
) with the perform name.
perform confirmPurchase() exterior inState(State.Created) situation(msg.worth == (2 * worth)) payable {
The occasion PurchaseConfirmed()
is emitted to mark the acquisition affirmation.
emit PurchaseConfirmed();
The msg.sender
worth is forged to payable and assigned to the customer variable.
💡 Information: Addresses are non-payable by design to forestall unintended funds; that’s why we now have to forged an handle to a payable earlier than having the ability to switch a cost.
purchaser = payable(msg.sender);
The state is about to Locked
as vendor and purchaser entered the contract, i.e., our digital model of an escrow settlement.
state = State.Locked; }
The confirmReceived()
perform is obtainable for execution solely within the Locked
state, and solely to the customer.
For the reason that purchaser deposited twice the worth quantity and withdrew solely a single worth quantity, the second worth quantity stays on the contract stability with the vendor’s deposit.
perform confirmReceived() exterior onlyBuyer inState(State.Locked) {
Emits the ItemReceived()
occasion.
emit ItemReceived();
Modifications the state to Launch.
state = State.Launch;
Transfers the deposit to the customer.
purchaser.switch(worth); }
The refundSeller()
perform is obtainable for execution solely within the Launch
state, and solely to the vendor.
For the reason that vendor deposited twice the worth quantity and earned a single worth quantity from the acquisition, the contract transfers three worth quantities from the contract stability to the vendor.
perform refundSeller() exterior onlySeller inState(State.Launch) {
Emits the SellerRefunded()
occasion.
emit SellerRefunded();
Modifications the state to Inactive
.
state = State.Inactive;
Transfers the deposit of two worth quantities and the one earned worth quantity to the vendor.
vendor.switch(3 * worth); } }
Our good contract instance of a protected distant buy is a pleasant and easy instance that demonstrates how a purchase order could also be carried out on the Ethereum blockchain community.
The protected distant buy instance reveals two events, a vendor and a purchaser, who each enter a buying and selling relationship with their deposits to the contract stability.
Every deposit quantities to twice the worth of the acquisition, that means that the contract stability will maintain 4 instances the acquisition worth at its highest level, i.e. within the Locked
state.
The peak of deposits is meant to stimulate the decision of any potential disputes between the events, as a result of in any other case, their deposits will keep locked and unavailable within the contract stability.
When the customer confirms that he obtained the products he bought, the contract will transition to the Launch
state, and the acquisition worth can be launched to the customer.
The vendor can now withdraw his earned buy worth with the deposit, the contract stability drops to 0 Wei, the contract transitions to the Inactive
state, and the protected distant buy concludes with execution.
The Contract Arguments
This part incorporates further data for working the contract. We must always count on that our instance accounts could change with every refresh/reload of Remix.
Our contract creation argument is the deposit (twice the acquisition worth). We’ll assume the acquisition worth to be 5 Wei, making the contract creation argument quite simple:
10
Contract Check State of affairs
- Account
0x5B38Da6a701c568545dCfcB03FcB875f56beddC4
deploys the contract with a deposit of 10 Wei, successfully changing into a vendor. - Account
0xAb8483F64d9C6d1EcF9b849Ae677dD3315835cb2
confirms the acquisition by calling theconfirmPurchase()
perform and enters the commerce with a deposit of 10 Wei, successfully changing into a purchaser. - The client confirms receiving the order by calling the
confirmReceived()
perform. - The vendor concludes the commerce by calling the
refundSeller()
perform.
Conclusion
We continued our good contract instance sequence with this text that implements a protected distant buy.
First, we laid out clear supply code (with none feedback) for readability functions.
Second, we dissected the code, analyzed it, and defined every probably non-trivial phase.
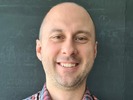
I’m an skilled laptop science engineer and know-how fanatic devoted to understanding how the world works and utilizing my information and talent to advance it. I’m targeted on changing into an professional in Solidity and crypto know-how, with a ardour for coding, studying, and contributing to the Finxter mission of accelerating the collective intelligence of humanity.